CSS Tables Tutorial with Examples
1. Table borders
Basically, a table has 2 types of border that is table border and cell borders. It is similar to the following illustration:
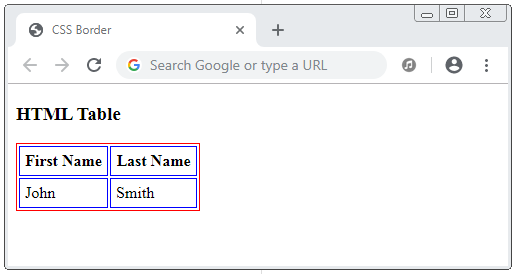
By default, adjacent cells do not use the same border. They have separate borders. And the table borders are also separate from the cell borders.
css-table-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Table Border</title>
<meta charset="UTF-8"/>
<style>
.table1 {
border: 1px solid red;
}
.table1 th {
border: 1px solid blue;
padding: 5px;
}
.table1 td {
border: 1px dashed green;
padding: 5px;
}
</style>
</head>
<body>
<h3>HTML Table Boder</h3>
<table class="table1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr>
<td>John</td>
<td>Smith</td>
</tr>
</table>
</body>
</html>
border-spacing
CSS border-spacing applies to <table> to specify the distance between the borders of adjacent cells.
/* <length> */
border-spacing: 2px;
/* horizontal <length> | vertical <length> */
border-spacing: 1cm 2em;
/* Global values */
border-spacing: inherit;
border-spacing: initial;
border-spacing: unset;
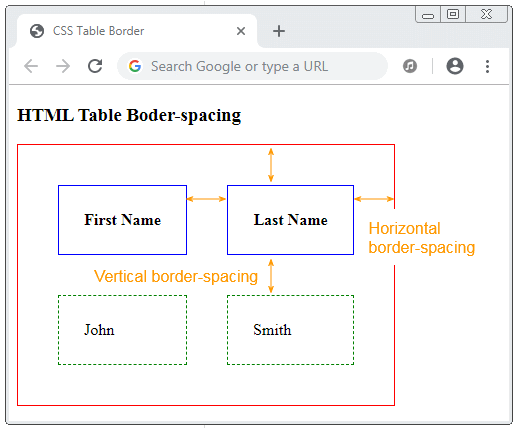
border-spacing-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Table Border</title>
<meta charset="UTF-8"/>
<style>
.table1 {
border: 1px solid red;
border-spacing: 40px;
}
.table1 th {
border: 1px solid blue;
padding: 25px;
}
.table1 td {
border: 1px dashed green;
padding: 25px;
}
</style>
</head>
<body>
<h3>HTML Table Boder-spacing</h3>
<table class="table1">
<tr>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr>
<td>John</td>
<td>Smith</td>
</tr>
</table>
</body>
</html>
Another example with CSS border-spacing:
table {
border-spacing: 1em .5em;
padding: 0 2em 1em 0;
border: 1px solid orange;
}
td {
width: 1.5em;
height: 1.5em;
background: #d2d2d2;
text-align: center;
vertical-align: middle;
}
<table>
<tr>
<td>1</td><td>2</td><td>3</td>
</tr>
<tr>
<td>4</td><td>5</td><td>6</td>
</tr>
<tr>
<td>7</td><td>8</td><td>9</td>
</tr>
</table>
2. Collapsed Table borders
Use CSS border-collapse:collapse for the table, if you want that adjacent cells share a border, and share a border with the table. The default value of CSS border-collapse is separate.
table {
border-collapse: collapse;
border: 1px solid black;
}
table th {
border: 1px solid black;
padding: 5px;
}
table td {
border: 1px solid black;
padding: 5px;
}
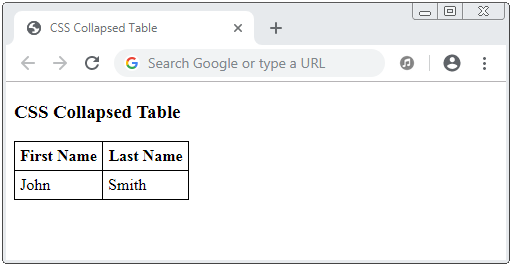
Because each cell can use a different border style. Thus, for collapsed tables conflict situations will occur because 2 adjacent cells will share a border. The question herein is which border style will be used in conflict case?
If borders have the same width, the priority order will be:
- hidden
- double
- solid
- dashed
- dotted
- ridge
- outset
- groove
- inset
- none
Note: CSS border-style:none and CSS border-style:hidden are the same. They make a element borderless. They are different only when applying to a collapsed table. When resolving conflicts CSS border-style:hidden has the highest priority, while CSS border-style:none has the lowest priority.
css-collapse-table-example2.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Collapsed Table</title>
<meta charset="UTF-8"/>
<style>
table {
border-collapse: collapse;
border: 1px solid black;
}
th, td {
padding: 10px;
}
</style>
</head>
<body>
<h3>CSS Collapsed Table</h3>
<table>
<tr>
<th style="border-style:solid;border-width:1px;border-color:blue;">
border-style:solid;<br/>
border-width:1px;<br/>
border-color:blue;
</th>
<th style="border-style:dashed;border-width:1px;border-color:red;">
border-style:dashed;<br/>
border-width:1px;<br/>
border-color:red;
</th>
</tr>
<tr>
<td style="border-style:hidden;border-width:1px;border-color:green;">
border-style:hidden;<br/>
border-width:1px;<br/>
border-color:green;
</td>
<td style="border-style:none;border-width:1px;border-color:green;">
border-style:none;<br/>
border-width:1px;<br/>
border-color:green;
</td>
</tr>
</table>
</body>
</html>
3. CSS Table Width/Height
The CSS width, CSS height property is used for establishing width and height for a table, or a row or a column.
Note: Some browsers only consider <thead>, <tbody>, <tfoot> as containers, therefore, CSS height doesn't work when you apply it to these elements. If you want to set up the height for a table row you need to apply CSS height to <th> or <td>.
table {
width: 100%;
border-collapse: collapse;
border: 1px solid black;
}
th {
height: 50px;
border: 1px solid black;
}
td {
border: 1px solid black;
}
In a table with multiple columns, the columns are numbered 1, 2, ... Use CSS th:nth-child(N) to apply styles to the Nth column of the table.
For example: A table with 3 columns, establish width:40% for 2 first columns and width:20% for the 3rd column.
table {
width: 100%;
border-collapse: collapse;
border: 1px solid black;
}
th:th:nth-child(1), th:th:nth-child(2) {
width: 40%;
}
th:th:nth-child(3) {
width: 20%;
}
th, td {
border: 1px solid black;
}
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More