CSS Links Tutorial with Examples
1. CSS Link States
Link is one of the important components of a page. CSS helps you to set up the style for link and highlights the link's status.
A link has 5 states:
- Link (Unvisited)
- Visited
- Hover
- Focus
- Active
Link (unvisited)
The unvisited status is also referred to as link status, which the default status of a link. It shows that a user never visits the address given by this link.
If a user has ever visited an address, it will save a log in browser's History. However, browsers also allow users to delete all these history logs.
Use the pseudo :link class to set up the style of this status.
Visited
This status indicates that the user has visited the address given by the link, in other words, a record of this visit in the browser's History exists.
Use the pseudo :visited class to set up the style for this status.
Hover
This is the state when a user moves the mouse pointer over the link. Use the pseudo :hover class to set up style for this state.
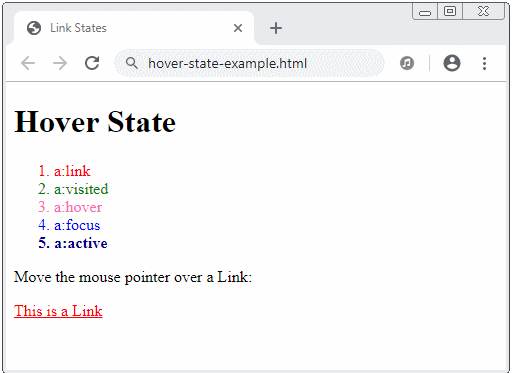
Focus
Status when a user focuses on a link, for example, the user presses TAB to focus to this link or call HTMLElement.focus() to focus to this link. Use the pseudo :focus class to set up the style for this status.
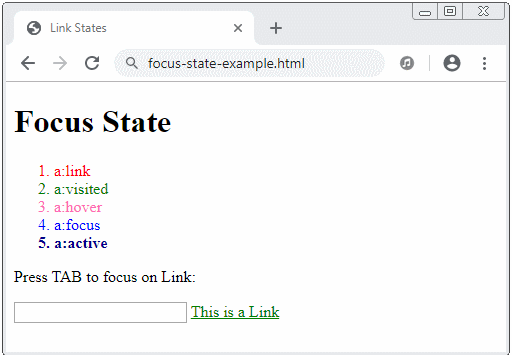
Active
The status of a link when it is being activated, specifically, the user presses the mouse on the link but does not release the mouse. It is noted that after the user releases the mouse, the link switch to the Focus status.
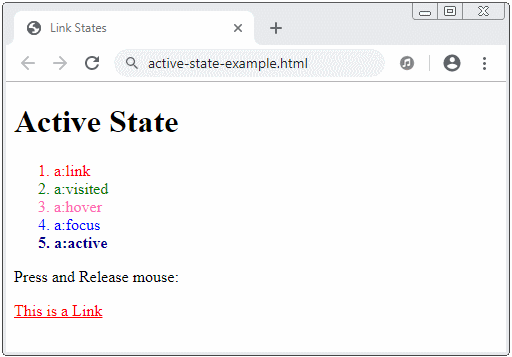
See the full example of the five states of a link:
page.css
/* unvisited link */
a:link, .link {
color: red;
}
/* visited link */
a:visited, .visited {
color: green;
}
/* mouse over link */
a:hover, .hover {
color: hotpink;
}
/* focus link */
a:focus, .focus {
color: blue;
font-weight: none;
}
/* selected link */
a:active, .active {
color: darkblue;
font-weight: bold;
}
page1.html
<!DOCTYPE html>
<html>
<head>
<title>Link States</title>
<meta charset="UTF-8"/>
<link rel="stylesheet" href="page.css" />
</head>
<body>
<h1>page1.html</h1>
<h3>CSS link states:</h3>
<ol>
<li class="link">a:link</li>
<li class="visited">a:visited</li>
<li class="hover">a:hover</li>
<li class="focus">a:focus</li>
<li class="active">a:active</li>
</ol>
<a href="page2.html">
Go to page2.html
</a>
</body>
</html>
page2.html
<!DOCTYPE html>
<html>
<head>
<title>Link States</title>
<meta charset="UTF-8"/>
<link rel="stylesheet" href="page.css" />
</head>
<body>
<h1>page2.html</h1>
<h3>CSS link states:</h3>
<ol>
<li class="link">a:link</li>
<li class="visited">a:visited</li>
<li class="hover">a:hover</li>
<li class="focus">a:focus</li>
<li class="active">a:active</li>
</ol>
<a href="page1.html">
Go to page1.html
</a>
</body>
</html>
Additional simple example of the five states of a link:
link-states-example.css
a {
outline: none;
text-decoration: none;
padding: 2px 1px 0;
}
a:link {
color: #265301;
}
a:visited {
color: #437A16;
}
a:focus {
border-bottom: 1px solid;
background: #BAE498;
}
a:hover {
border-bottom: 1px solid;
background: #CDFEAA;
}
a:active {
background: #265301;
color: #CDFEAA;
}
link-states-example.html
<!DOCTYPE html>
<html>
<head>
<title>Link States</title>
<meta charset="UTF-8"/>
<link rel="stylesheet" href="link-states-example.css" />
</head>
<body>
<h3>CSS Link State Example:</h3>
<p>There are several browsers available, such as
<a href="#">Mozilla Firefox</a>,
<a href="#">Google Chrome</a>, and
<a href="#">Microsoft Edge</a>.
</p>
</body>
</html>
2. CSS text-decoration
By default, links usually have an "underline". We often use CSS text-decoration with the none value to remove this "underline".
The possible values of CSS text-decoration:
- overline
- line-through
- underline
- none
text-decoration-example.css
a:link {
text-decoration: none;
}
a:visited {
text-decoration: none;
}
a:hover {
text-decoration: underline;
background-color: #BAE498;
}
a:focus {
text-decoration: underline;
font-weight: normal;
}
a:active {
text-decoration: underline;
color: red;
font-weight: bold;
}
text-decoration-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Link text-decoration</title>
<meta charset="UTF-8"/>
<link rel="stylesheet" href="text-decoration-example.css" />
</head>
<body>
<h3>CSS text-decoration</h3>
<a href="#">
This is a Link
</a>
</body>
</html>
See also:
3. CSS Link Button
Below is an advanced example, combining several CSS properties to make a link look like a button.
link-button-example.css
a {
display: inline-block;
background-color: #eaf1dd;
color: #060;
text-decoration: none;
padding: 6px 4px;
margin: 4px;
border-right: 2px solid #999999;
border-bottom: 2px solid #999999;
border-top-width: 0px;
border-left-width: 0px;
}
a:hover
{
color: #030;
border: 1px solid #9999ff;
}
a:active
{
color: #aca;
border-left: 2px solid #030;
border-top: 2px solid #030;
border-right-width: 0px;
border-bottom-width: 0px;
}
link-button-example.html
<!DOCTYPE html>
<html>
<head>
<title>Link Button</title>
<meta charset="UTF-8"/>
<link rel="stylesheet" href="link-button-example.css" />
</head>
<body>
<h1>Link Button</h1>
<div>
<a href="#">Apple</a>
<a href="#">Facebook</a>
<a href="#">Google</a>
</div>
</body>
</html>
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More