CSS Overflow Tutorial with Examples
1. CSS Overflow
When the content of an element is larger than the space provided by the element, the content may overflow. CSS overflow allows you to set up element behavior in this case.
Note: CSS overflow works with only the block elements that are specified a specific height.
The possible values of CSS overflow:
- visible
- hidden
- scroll
- auto
2. CSS {overflow: visible}
CSS {overflow: visible}: (Default). If the content of the element is larger than the space provided by the element, it will overflow, which is the default behavior.
overflow-visible-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS overflow</title>
<meta charset="UTF-8"/>
<style>
div {
background-color: #d6eaf8;
padding: 5px;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>CSS {overflow:visible} (Default)</h1>
<hr/>
<div style="width: 200px; height: 100px; overflow: visible">
Michaelmas term lately over, and the Lord Chancellor
sitting in Lincoln's Inn Hall. Implacable November weather.
As much mud in the streets as if the waters
had but newly retired from the face of the earth.
</div>
</body>
</html>
3. CSS {overflow: hidden}
CSS {overflow: hidden}: The content overflowing the space of element will be hidden.
overflow-hidden-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS overflow</title>
<meta charset="UTF-8"/>
<style>
div {
background-color: #d6eaf8;
padding: 5px;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>CSS {overflow:hidden}</h1>
<hr/>
<div style="width: 200px; height: 100px; overflow: hidden">
Michaelmas term lately over, and the Lord Chancellor
sitting in Lincoln's Inn Hall. Implacable November weather.
As much mud in the streets as if the waters
had but newly retired from the face of the earth.
</div>
</body>
</html>
4. CSS {overflow: scroll}
CSS {overflow: scroll}: The browser will create a scroll bar for element. Users can use the scroll bar to view the remaining contents.
Note: For most browsers and operating systems, an element's scroll bar always appears even when the content of the element is less than the space provided by the element. Exceptions occur with Mac OSX Lion, scroll bars appear only when necessary.
overflow-scroll-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS overflow</title>
<meta charset="UTF-8"/>
<style>
div {
background-color: #d6eaf8;
padding: 5px;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>CSS {overflow:scroll}</h1>
<hr/>
<div style="width: 200px; height: 100px; overflow: scroll">
Michaelmas term lately over, and the Lord Chancellor
sitting in Lincoln's Inn Hall. Implacable November weather.
As much mud in the streets as if the waters
had but newly retired from the face of the earth.
</div>
</body>
</html>
5. CSS {overflow: auto}
CSS {overflow: auto}: Similar to 'scroll', but scroll bar appears only when the content is larger than the space that the element provides.
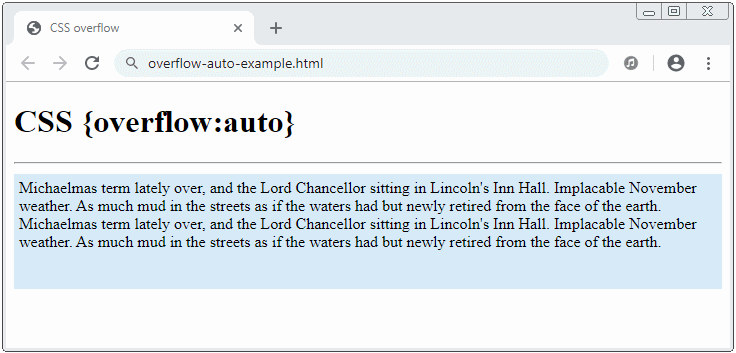
overflow-auto-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS overflow</title>
<meta charset="UTF-8"/>
<style>
div {
background-color: #d6eaf8;
padding: 5px;
margin-top: 10px;
}
</style>
</head>
<body>
<h1>CSS {overflow:auto}</h1>
<hr/>
<div style="height: 105px; overflow: auto">
Michaelmas term lately over, and the Lord Chancellor
sitting in Lincoln's Inn Hall. Implacable November weather.
As much mud in the streets as if the waters
had but newly retired from the face of the earth.
<br/>
Michaelmas term lately over, and the Lord Chancellor
sitting in Lincoln's Inn Hall. Implacable November weather.
As much mud in the streets as if the waters
had but newly retired from the face of the earth.
</div>
</body>
</html>
6. CSS overflow-x, overflow-y
Instead of using CSS overflow you can use CSS overflow-x and CSS overflow-y. The possible values of CSS overflow-x, CSS overflow-y are the same as CSS overflow.
- visible
- hidden
- scroll
- auto
CSS overflow-x
CSS overflow-x is used to set up element behavior when its content overflows horizontally.
CSS overflow-y
CSS overflow-y is used to set up element behavior when its content overflows vertically.
Note: You can specify a value pair for (overflow-x,overflow-y), but the browser will recalculate this value pair, because the value pair specified by you can not be real. For example, (visible, hidden) will be recalculated into (scroll, hidden).
The following table contains 2 columns. The first column contains the value pairs you specify. The second column contains the value pairs that the browser has recalculated.
Specified values | Computed values |
(visible, visible) | (visible, visible) |
(visible, hidden) | (scroll, hidden) |
(visible, scroll) | (scroll, scroll) |
(visible, auto) | (scroll, auto) |
(hidden, visible) | (hidden, scroll) |
(hidden, hidden) | (hidden, hidden) |
(hidden, scroll) | (hidden, scroll) |
(hidden, auto) | (hidden, auto) |
(scroll, visible) | (scroll, scroll) |
(scroll, hidden) | (scroll, hidden) |
(scroll, scroll) | (scroll, scroll) |
(scroll, auto) | (scroll, auto) |
(auto, visible) | (auto, scroll) |
(auto, hidden) | (auto, hidden) |
(auto, scroll) | (auto, scroll) |
(auto, auto) | (auto, auto) |
overflow-x-y-example.js
function changeOverflowX(event) {
value = event.target.value;
var myDiv = document.getElementById("myDiv");
myDiv.style.overflowX = value;
}
function changeOverflowY(event) {
value = event.target.value;
var myDiv = document.getElementById("myDiv");
myDiv.style.overflowY = value;
}
overflow-x-y-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS overflow</title>
<meta charset="UTF-8"/>
<style>
#myDiv {
background-color: #d6eaf8;
padding: 5px;
margin-top: 15px;
}
</style>
<script src="overflow-x-y-example.js"> </script>
</head>
<body>
<h2>CSS overflow-x, overflow-y</h2>
<hr/>
<div style="display: inline-block; width: 150px;">
<p>Overflow-x:</p>
<input type="radio" name="overflowX" value="visible" onClick="changeOverflowX(event)" checked/> Visible <br/>
<input type="radio" name="overflowX" value="hidden" onClick="changeOverflowX(event)"/> Hidden <br/>
<input type="radio" name="overflowX" value="scroll" onClick="changeOverflowX(event)"/> Scroll <br/>
<input type="radio" name="overflowX" value="auto" onClick="changeOverflowX(event)"/> Auto <br/>
</div>
<div style="display: inline-block; width: 150px;">
<p>Overflow-y:</p>
<input type="radio" name="overflowY" value="visible" onClick="changeOverflowY(event)" checked/> Visible <br/>
<input type="radio" name="overflowY" value="hidden" onClick="changeOverflowY(event)"/> Hidden <br/>
<input type="radio" name="overflowY" value="scroll" onClick="changeOverflowY(event)"/> Scroll <br/>
<input type="radio" name="overflowY" value="auto" onClick="changeOverflowY(event)"/> Auto <br/>
</div>
<div id="infoDiv">
</div>
<div id="myDiv" style="height: 50px; width:200px; overflow-x: visible;">
The value of Pi is <br/>
3.1415926535897932384626433832795029.
The value of e is <br/>
2.718281828459045235360287471352662
</div>
</body>
</html>
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More