CSS Display Tutorial with Examples
1. Overview of CSS display
CSS display is used in the following situations:
1 - CSS {display: none}
CSS {display:none} is used to hide an element and release the space that it occupies.
2 - CSS {display: block | inline | inline-block}
Use CSS display with one of the block, inline, inline-block values to make the element treated as a Block element, inline element, or inline-block element.
3 - CSS {display: grid | flex | ... }
Use CSS display with other values (grid, inline-grid, flex, ...) to set the layout for the children of the current element. For example, CSS {display: grid | inline-grid} dividing the surface of the current element into a grid, consisting of many rows and columns, its children will be arranged on these grid cells.
2. CSS {display: none}
CSS {display:none} is used to hide an element and release the space that it occupies. To redisplay this element, use CSS {display:block | inline | inline-block} or remove CSS display.
display-none-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {display:none}</title>
<meta charset="UTF-8"/>
<script>
function showHideImage(event) {
var myImage = document.getElementById("myImage");
var displayValue = myImage.style.display;
if(!displayValue) {
myImage.style.display = "none";
} else {
// Remove CSS display.
myImage.style.display = null;
// OR Set to: block|inline|inline-block.
}
}
</script>
</head>
<body>
<h2>CSS {display:none}</h2>
<button onClick="showHideImage(event)">Show/Hide Image</button>
<div style="padding:5px; margin-top:10px; background:#eee;">
<img src="../images/flower.png" id= "myImage"/>
Apollo 11 was the spaceflight that landed the first humans,
Americans Neil Armstrong and Buzz Aldrin,
on the Moon on July 20, 1969, at 20:18 UTC.
Armstrong became the first to step onto the lunar
surface 6 hours later on July 21 at 02:56 UTC.
</div>
</body>
</html>
CSS {visibility:hidden} makes an element become invisible, but it doesn't release the space that the element occupies.
3. CSS {display:block}
Use CSS {display:block} for an element to make it treated as a Block-level element.
- Some default elements are a block element, for example <div>, <hr>, ...
- Some other elements are by default Inline elements, for example <span>, your can use CSS {display:block} for them to turn it into a block element.
A block element is always displayed as a rectangle. The browser automatically adds line breaks before and after of this element.
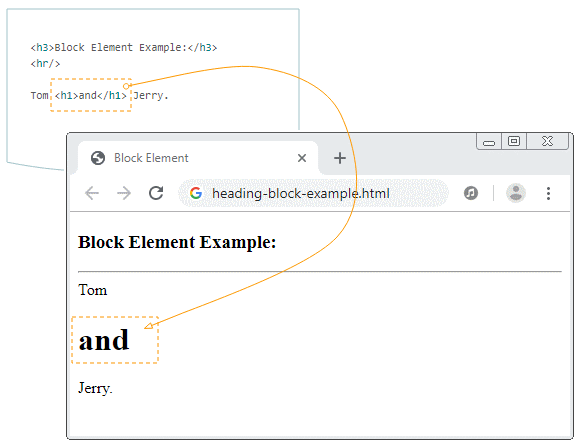
Example: Apply CSS {display:block} to the <span> element to turn it into a block element:
display-block-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {display:block}</title>
<meta charset="UTF-8"/>
<script>
function setDisplayBlock() {
var mySpan = document.getElementById("mySpan");
mySpan.style.display = "block";
}
// Use default display.
function removeDisplay() {
var mySpan = document.getElementById("mySpan");
mySpan.style.display = null;
}
</script>
</head>
<body>
<h2>CSS {display:block}</h2>
<button onClick="setDisplayBlock()">Set {display:block}</button>
<button onClick="removeDisplay()">Remove display property</button>
<div style="padding:5px; margin-top:10px; background:#eee;">
<img src="../images/flower.png" id= "myImage"/>
<span id="mySpan" style="background:yellow">Apollo 11</span> was the
spaceflight that landed the first humans,
Americans Neil Armstrong and Buzz Aldrin,
on the Moon on July 20, 1969, at 20:18 UTC.
Armstrong became the first to step onto the lunar
surface 6 hours later on July 21 at 02:56 UTC.
</div>
</body>
</html>
4. CSS {display: inline}
Use CSS {display:inline} for an element to make it treated as a Inline element.
- Some elements are by default inline elements, for example, <span>, <strong>, ...
- Some other elements are by default block elements, for example, <div>, for which you can use CSS {display:inline} to turn them into inline elements.
An inline element, in many cases, are not a rectangle. It may lie on multiple lines.
Apollo 11 was the spaceflight that landed the first humans,
Americans Neil Armstrong and Buzz Aldrin,
on the Moon on July 20, 1969, at 20:18 UTC.
Armstrong
<span style="background:yellow;">
became the first to step onto the lunar
surface 6 hours later on July 21 at 02:56 UTC.
</span>
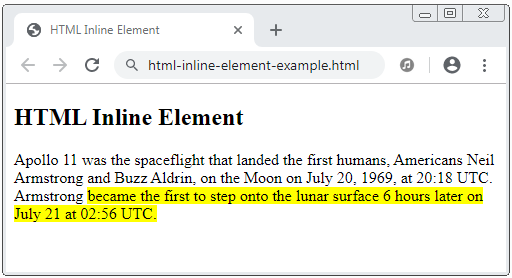
Example, apply CSS {display:inline} to a <div> element to turn it into an inline element.
display-inline-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {display:inline}</title>
<meta charset="UTF-8"/>
<script>
function setDisplayInline() {
var myDiv = document.getElementById("myDiv");
myDiv.style.display = "inline";
}
// Use default display.
function removeDisplay() {
var myDiv = document.getElementById("myDiv");
myDiv.style.display = null;
}
</script>
</head>
<body>
<h2>CSS {display:inline}</h2>
<button onClick="setDisplayInline()">Set {display:inline}</button>
<button onClick="removeDisplay()">Remove display property</button>
<div style="padding:5px; margin-top:10px; background:#eee;">
<img src="../images/flower.png" />
<div id="myDiv" style="background:yellow">
Apollo 11 was the spaceflight that landed the first humans,
Americans Neil Armstrong and Buzz Aldrin,
on the Moon on July 20, 1969, at 20:18 UTC.
</div>
Armstrong became the first to step onto the lunar
surface 6 hours later on July 21 at 02:56 UTC.
</div>
</body>
</html>
5. CSS {display: inline-block}
Use CSS {display:inline-block} for element to make it treated as an Inline-block element.
"Inline-block element" is a rectangle. The browser will not automatically add line breaks before and after this element, which makes inline-block elements possible on the same line.
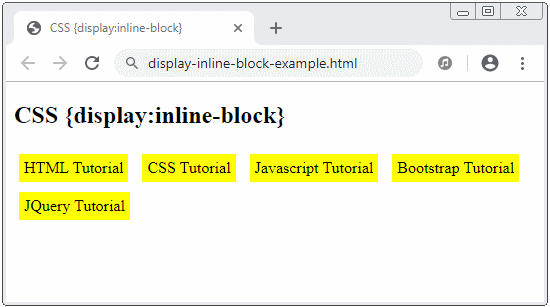
display-inline-block-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS {display:inline-block}</title>
<meta charset="UTF-8"/>
<style>
div {
background: yellow;
padding: 5px;
margin: 5px;
}
</style>
</head>
<body>
<h2>CSS {display:inline-block}</h2>
<div style="display:inline-block">
HTML Tutorial
</div>
<div style="display:inline-block">
CSS Tutorial
</div>
<div style="display:inline-block">
Javascript Tutorial
</div>
<div style="display:inline-block">
Bootstrap Tutorial
</div>
<div style="display:inline-block">
JQuery Tutorial
</div>
</body>
</html>
6. CSS {display: grid | inline-grid}
CSS {display:grid | inline-grid} is applied to an element to divide its surface into a grid, consisting of rows and columns. Its child elements will be arranged on grid cells. It makes it easier to design pages instead of using CSS float or element positioning techniques.
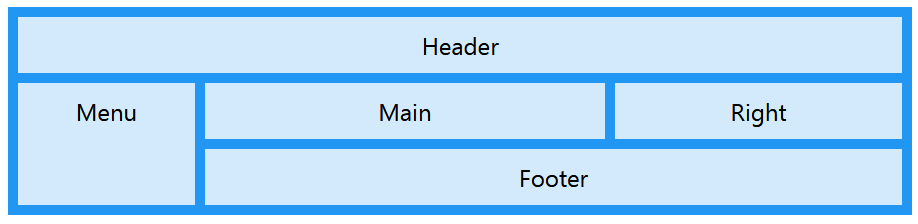
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More