CSS Borders Tutorial with Examples
1. CSS Border Overview
The CSS Border property is used to establish a border for an element, namely border width, border style, and border color.
The simplest syntax to establish a border for an element:
border: border-width border-style border-color;
/* Example: */
border: 40px solid LightGray;
Example:
<div style="border: 40px solid LightGray; padding:10px;">
This is a div <br/>
border: 40px solid LightGray;
</div>
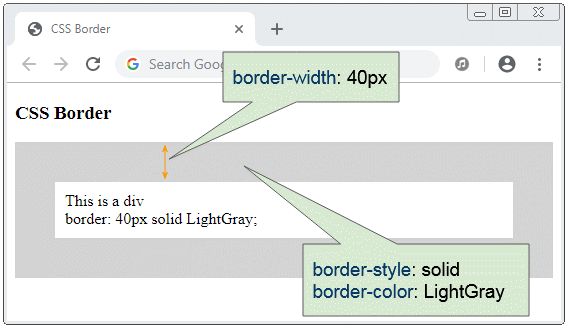
border-width | Optional; the default value is medium. |
border-style | Mandatory. |
border-color | Optional; its default value depends on user's graphic environment |
<div style="border: 1px solid LightGray; ">
3 values (border-width, border-style, border-color):
<p>border: 1px solid LightGray;</p>
</div>
<div style="border: solid LightGray; ">
2 values (border-style, border-color):
<p>border: solid LightGray;</p>
</div>
<div style="border: solid; ">
1 values (border-style):
<p>border: solid;</p>
</div>
Instead of using CSS border you can use the 3 properties: CSS border-width & CSS border-style & CSS border-color.
<div style="border-width: 40px; border-style: solid; border-color: LightGray;">
<p>border-width: 40px; border-style: solid; border-color: LightGray;</p>
</div>
2. CSS border-width
The CSS border-width property is used for establishing border width for an element. You can provide 4 values for it, including top side width, right side width, bottom side width, left side width.
border-width: border-top-width border-right-width border-bottom-width border-left-width;
/* Example: */
boder-width: 10px 20px 30px 40px;
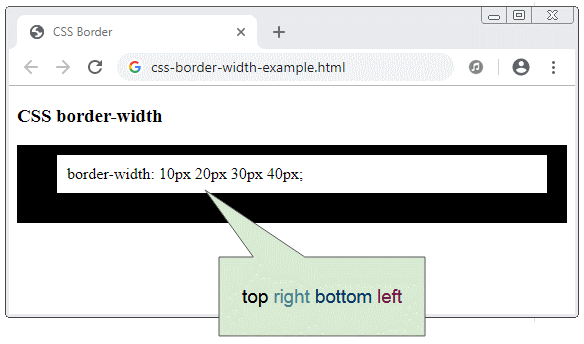
If you provide 2 values for CSS border-width, the first value applies to top side and bottom side. The second value applies to left side and right side.
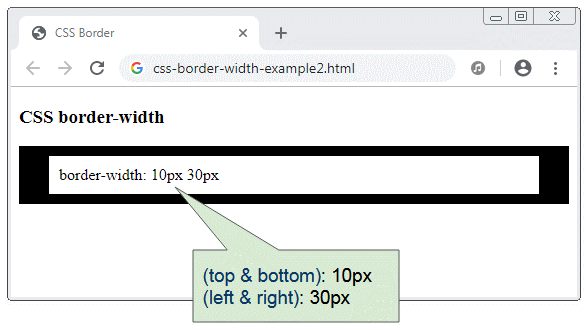
If you provide 3 values for CSS border-width, the first value will apply to top side and the second side will apply to left side and right side and the third value shall apply to the bottom side.
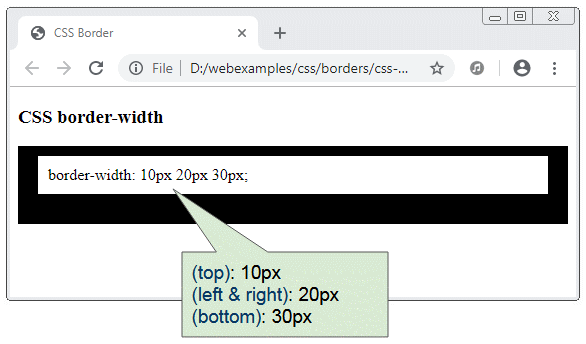
If you provide 1 value for CSS border-width, it will apply to all of the 4 sides of the element.
/* Keyword values */
border-width: thin;
border-width: medium;
border-width: thick;
/* <length> values */
border-width: 4px;
border-width: 1.2rem;
/* vertical | horizontal */
border-width: 2px 1.5em;
/* top | horizontal | bottom */
border-width: 1px 2em 1.5cm;
/* top | right | bottom | left */
border-width: 1px 2em 0 4rem;
/* Global keywords */
border-width: inherit;
border-width: initial;
border-width: unset;
Instead of using CSS border-width, you can use CSS border-top-width, CSS border-right-width, CSS border-bottom-width, and CSS border-left-width.
css-border-width-example5.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Border</title>
<meta charset="UTF-8"/>
<style>
.my-div {
padding:10px;
border-top-width: thin;
border-bottom-width:thick;
border-left-width: 1px;
border-right-width: 10px;
border-style: solid;
}
</style>
</head>
<body>
<h3>CSS border-width</h3>
<div class="my-div">
border-top-width: thin; <br/>
border-bottom-width:thick; <br/>
border-left-width: 1px; <br/>
border-right-width: 10px; <br/>
</div>
</body>
</html>
3. CSS border-style
The CSS border-style property is used to setting up border style for an element. You can provide 4 values for it. They are style for top side, right side, bottom side and left side.
border-style: border-top-style border-right-style border-bottom-style border-left-style;
/* Example: */
border-style: dotted dashed solid double;
css-border-style-example.html
<div style="border-width: 5px; border-style: dotted dashed solid double;">
border-style: dotted dashed solid double;
</div>
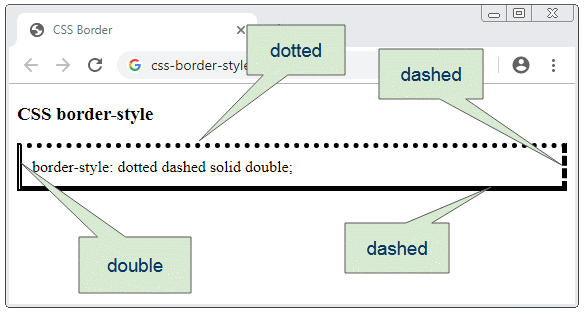
If you provide 2 values for CSS border-style, the first value will apply to top side and bottom side and the second value will apply to left side and right side.
<div style="border-width: 5px; border-style: dotted dashed; ">
border-style: dotted dashed;
</div>
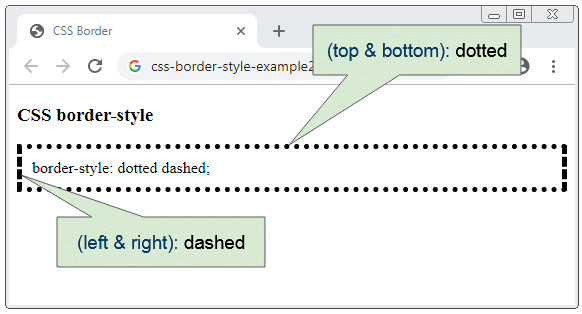
If you provide 3 values for CSS border-style, the first value will appy to the top side, and the second value will apply to the left side and right side and the third value will apply to the bottom side.
css-boder-style-example3.html
<div style="border-width: 5px; 10px 20px 30px; border-style: dotted dashed solid; ">
border-style: dotted dashed solid;
</div>
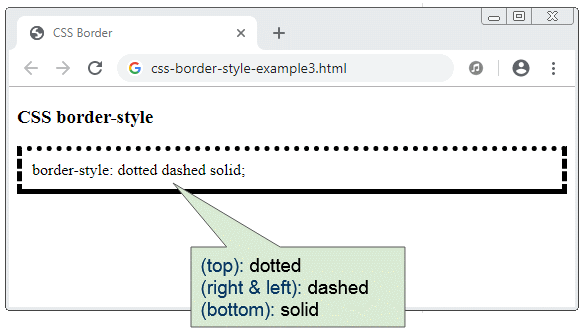
If you provide 1 value for CSS border-style, it will apply to all the sides of the element.
Possible values of CSS border-style:
- dotted
- dashed
- solid
- double
- groove
- ridge
- inset
- outset
- none
- hidden
<div style="border-width:5px; border-style:dotted">border-style:dotted</div>
<div style="border-width:5px; border-style:dashed">border-style:dashed</div>
<div style="border-width:5px; border-style:solid">border-style:solid</div>
<div style="border-width:5px; border-style:double">border-style:double</div>
<div style="border-width:5px; border-style:groove">border-style:groove</div>
<div style="border-width:5px; border-style:ridge">border-style:ridge</div>
<div style="border-width:5px; border-style:inset">border-style:inset</div>
<div style="border-width:5px; border-style:outset">border-style:outset</div>
<div style="border-width:5px; border-style:none">border-style:none</div>
<div style="border-width:5px; border-style:hidden">border-style:hidden</div>
Instead of using CSS border-style, You can use CSS border-top-style, CSS border-right-style, CSS border-bottom-style, CSS border-left-style.
css-boder-style-example5.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Border</title>
<meta charset="UTF-8"/>
<style>
.my-div {
padding:10px;
border-width: 5px;
border-top-style: dotted;
border-right-style: dashed;
border-bottom-style: solid;
border-left-style: double;
}
</style>
</head>
<body>
<h3>CSS border-style</h3>
<div class="my-div">
border-top-style: dotted; <br/>
border-right-style: dashed; <br/>
border-bottom-style: solid; <br/>
border-left-style: double;
</div>
</body>
</html>
4. CSS border-style: none vs hidden
CSS border-style:none and CSS border-style:hidden are the same. They are different when they are used for a collapsed table. You can see the explanation in the following post:
5. CSS border-color
The CSS border-color property is used for setting up border color for an element. You can provide 4 values for it, which are colors for top side, right side, bottom side and left side.
<div style="border-color: green red purple yellow;border-width: 5px; border-style: solid; ">
border-color: green red purple yellow;
</div>
If you provide 2 values for CSS border-color, the first value applies to the top side and bottom side and the second value applies to left side and right side.
<div style="border-color: green red;border-width: 5px; border-style: solid;">
border-color: green red;
</div>
If you provide 3 values for CSS border-color, the first value will apply to the top side, and the second value will apply to the left side and right side and the third value will apply to the bottom side.
css-border-color-example3.html
<div style="border-color: green red blue;border-width: 5px; border-style: solid;">
border-color: green red blue;
</div>
Instead of using CSS border-color, you can use CSS border-top-color, CSS border-right-color, CSS border-bottom-color, CSS border-left-color.
border-top-color: blue;
border-right-color: red;
border-bottom-color: purple;
border-left-color: yellow;
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More