CSS Padding Tutorial with Examples
1. CSS Padding
CSS Padding property is used to create a space surrounding the content of element and located in the element border like the following illustration:
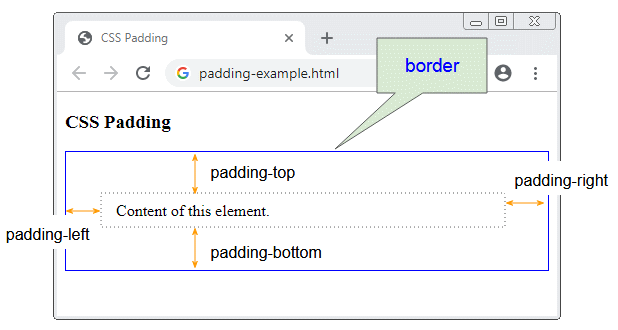
You can provide 4 values for CSS padding:
/* Syntax: */
padding: padding-top padding-right padding-bottom padding-left;
/* Example: */
padding: 5px 20px 30px 40px;
Instead of providing 4 values for CSS padding you can use 4 properties: CSS padding-top, padding-right, padding-bottom, padding-left.
padding-top: 5px;
padding-right: 20px;
padding-bottom: 30px;
padding-right: 40px;
If you provide 2 values for CSS padding, the first value is assigned to padding-top & padding-bottom, and the second value is assigned to padding-right & padding-left.
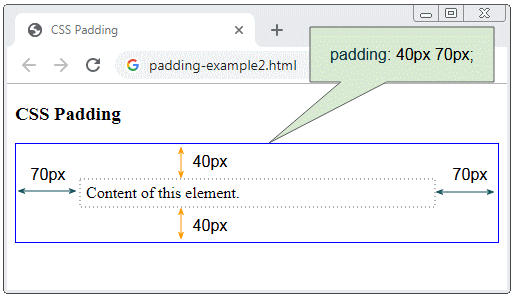
If you provide 3 values for CSS padding, the first value is assigned to padding-top, the second value is assigned to padding-right & padding-left, and the third value is assigned to padding-bottom.
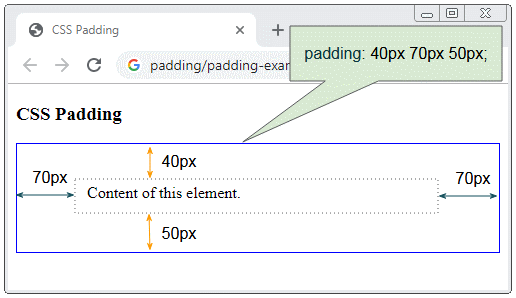
CSS padding accepts specific values in px, pt, cm, ... or percent ( % ).
/* Apply to all four sides */
padding: 1em;
/* vertical | horizontal */
padding: 5% 10%;
/* top | horizontal | bottom */
padding: 1em 2em 2em;
/* top | right | bottom | left */
padding: 5px 1em 0 2em;
/* Global values */
padding: inherit;
padding: initial;
padding: unset;
2. Padding makes an element bigger
The presence of CSS padding makes an element larger than itself without establishing CSS padding.
Please see the following example:
padding-and-width-height.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Padding</title>
<meta charset="UTF-8"/>
</head>
<body>
<h3>CSS Padding</h3>
<div style="width:200px; height:50px; border: 1px solid blue;">
width:200px; height:80px;
</div>
<br/>
<div style="width:200px; height:50px; padding: 20px; border: 1px solid red;">
width:200px; height:80px; padding: 20px;
</div>
</body>
</html>
The actual size of the element you see is calculated by the following formula:
realWidth = contentWidth + cssBorderWidthLeft + cssBorderWidthRight + cssPaddingLeft + cssPaddingRight
realHeight = contentHeight + cssBorderWidthTop + cssBorderWidthBottom + cssPaddingTop + cssPaddingBottom
In case, by default contentWidth = cssWidth and contentHeight = cssHeight.
// By Default:
contentWidth = cssWidth
contentHeight = cssHeight
Using CSS box-sizing:border-box will not increase element size when you use CSS padding.
3. Padding with percentage value
CSS padding accepts percentage value (%). This value is percentage compared to the width of Containing Block. Of which, the Containing Block is the nearest ancestor block-level element that contains the current element.
padding-percent-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS Padding</title>
<meta charset="UTF-8"/>
<script>
function changeParentSize() {
var blockDiv = document.getElementById("blockDiv");
// offsetWidth = cssWidth + padding + border
var offsetWidth = blockDiv.offsetWidth;
if(offsetWidth > 300) {
offsetWidth = 200;
}
blockDiv.style.width = (offsetWidth+1) + "px";
}
</script>
</head>
<body>
<h3>CSS Padding Percentage</h3>
<div id="blockDiv" style="width:200px; height: 150px; border: 1px solid blue">
I am a div (Block-Level Element)
<span style="background-color: yellow;">
I am a span (Inline Element)
<div id= "redDiv" style="width:100px; padding:10%; border:1px solid red;">
width:100px; padding:10%;
</div>
</span>
</div>
<br/>
<button onClick="changeParentSize()">Change size of 'blockDiv'</button>
</body>
</html>
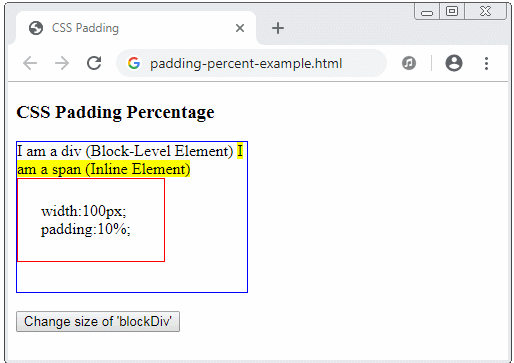
- HTML javascript width height
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More