CSS Cursors Tutorial with Examples
1. CSS Cursor
CSS cursor allows you to specify cursor type showing to user when the cursor is over an element.
/* Keyword value */
cursor: pointer;
cursor: auto;
.....
/* Global values */
cursor: inherit;
cursor: initial;
cursor: unset;
cursor-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS cursor</title>
<meta charset="UTF-8"/>
<style>
.container {
display: grid;
grid-template-columns: auto auto auto;
}
.child {
padding: 5px;
margin: 5px;
border: 1px solid gray;
}
</style>
</head>
<body>
<h3>CSS cursor</h3>
<p style="color:blue;">
Move the cursor over the elements to see the results.
</p>
<div class="container">
<div class="child" style = "cursor:alias">alias</div>
<div class="child" style = "cursor:all-scroll">all-scroll</div>
<div class="child" style = "cursor:auto">auto</div>
<div class="child" style = "cursor:copy">copy</div>
<div class="child" style = "cursor:crosshair">crosshair</div>
<div class="child" style = "cursor:default">default</div>
<div class="child" style = "cursor:help">help</div>
<div class="child" style = "cursor:inherit">inherit</div>
<div class="child" style = "cursor:move">move</div>
<div class="child" style = "cursor:pointer">pointer</div>
<div class="child" style = "cursor:progress">progress</div>
<div class="child" style = "cursor:text">text</div>
<div class="child" style = "cursor:vertical-text">vertical-text</div>
<div class="child" style = "cursor:wait">wait</div>
<div class="child" style = "cursor:no-drop">no-drop</div>
<div class="child" style = "cursor:grab">grab</div>
<div class="child" style = "cursor:grabbing">grabbing</div>
<div class="child" style = "cursor:e-resize">e-resize</div>
<div class="child" style = "cursor:n-resize">n-resize</div>
<div class="child" style = "cursor:s-resize">s-resize</div>
<div class="child" style = "cursor:w-resize">w-resize</div>
<div class="child" style = "cursor:col-resize">col-resize</div>
<div class="child" style = "cursor:row-resize">row-resize</div>
<div class="child" style = "cursor:ne-resize">ne-resize</div>
<div class="child" style = "cursor:nw-resize">nw-resize</div>
<div class="child" style = "cursor:se-resize">se-resize</div>
<div class="child" style = "cursor:sw-resize">sw-resize</div>
<div class="child" style = "cursor:nesw-resize">nesw-resize</div>
<div class="child" style = "cursor:nwse-resize">nwse-resize</div>
<div class="child" style = "cursor:zoom-in">zoom-in</div>
<div class="child" style = "cursor:zoom-out">zoom-out</div>
</div>
</body>
</html>
The predefined values of CSS cursor and their corresponding shapes are easy to understand. You can see the following illustration:
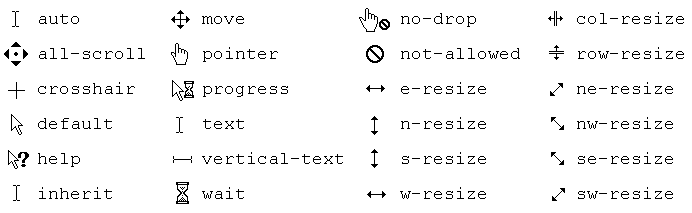
CSS {cursor:auto}
Cursor shape corresponding to the auto value depends on the context of the area which the cursor is over. For example, the cursor will have a hand shape when it stands over a link, etc.
CSS {cursor:default}
The cursor shape corresponding to the default value depends on platform, specifically, on operating system and browser, usually an arrow shape.
In addition to the values defined above, you can create a cursor type based on an image.
/* URL and coordinates, with a keyword fallback */
cursor: url(cursor1.png) 4 12, auto;
cursor: url(cursor1.png), url(cursor2.png) 2 2, pointer;
Url(..)
You can provide one or more url(..) values, which are separated by commas (,). The first value will be given priority to be used. The following values are backup. They are used in case the browser does not support a certain image format. The final backup value should be a predefined value (See the above list).
x, y
The (x,y) value is the coordinates of the image and is optional. They are non-negative integers and are less than 32.
Example:
custom-cursor-example.html
<!DOCTYPE html>
<html>
<head>
<title>CSS cursor</title>
<meta charset="UTF-8"/>
<style>
.my-div {
height: 150px;
width: 300px;
padding: 5px;
border: 1px solid gray;
cursor: url('../images/my-cursor.png'), pointer;
}
</style>
</head>
<body>
<h3>CSS Custom cursor</h3>
<div class="my-div">
Move the cursor over me!
</div>
</body>
</html>
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More