Basic CSS Selectors Tutorial with Examples
1. What is CSS Selector?
CSS Selector is used to search (or select) elements in an HTML document to apply styles to them.
For a simple example:
/** Select all elements with class contains abc */
.abc
/** Select element with ID = abc */
#abc
CSS Selectors can be divided into 3 types as follows:
Basic Selectors
Basic Selectors help you select elements based on their Name, ID, Class or Attribute.
The basic Selector will be mentioned in this article.
Combining selectors
Select elements based on the relationship among them.
Combinator | Syntax | Example |
Descendant combinator | AB | div span
div .item |
Child combinator | A> B | ul > li |
General sibling combinator | A~ B | p ~ span |
Adjacent sibling combinator | A+ B | h2 + p |
Column combinator | A|| B | col || td |
Pseudo selectors
Pseudo | Syntax | Example |
Pseudo classes | A:B | a:visited |
Pseudo elements | A::B | p::first-line |
- CSS Pseudo Selector
2. Universal selector
Universal selector: Select all the elements or all the elements of a namespace.
Syntax:
Selector | Description |
* | Select all the elements. |
*|* | Select all the elements. |
ns|* | Select all the elements in a namespace called ns. |
|* | Select all the elements with an undeclared namespace. |
For example, select all the elements and set a blue border for them.
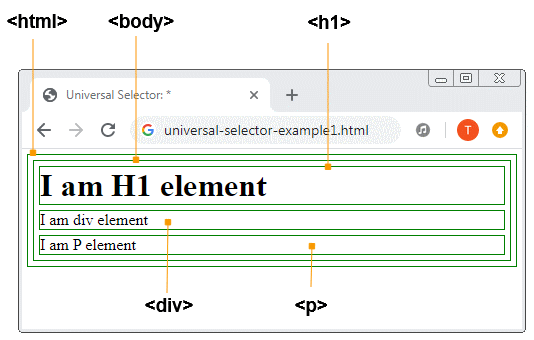
universal-selector-example1.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Universal Selector: *</title>
<style>
* {
border: 1px solid green;
margin: 5px;
}
</style>
</head>
<body>
<h1>I am H1 element</h1>
<div>I am div element</div>
<p>I am P element</div>
</body>
</html>
3. CSS Type Selector
CSS Type Selector helps you find the elements by name.
For example, select all of the <div> elements:
div {
color: red;
}
Another example, select all of the <span> elements:
type-selector-example1.css
span {
background-color: skyblue;
}
type-selector-example1.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Universal Selector: ns|*</title>
<link rel="stylesheet" type="text/css" href="type-selector-example1.css" />
</head>
<body>
<span>I am span element</span>
<p>I am P element</p>
<span>I am span element</span>
</body>
</html>
If CSS selectors have the same rule, you can write them briefly. CSS selectors will be separated by commas and follow the same rule.
type-selector-example2.css
/** Select H1 or H2 */
h1, h2 {
color: blue;
}
type-selector-example2.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Type Selector</title>
<link rel="stylesheet" type="text/css" href="type-selector-example2.css" />
</head>
<body>
<h1>I am H1 element</h1>
<h2>I am H2 element</h2>
<h3>I am H3 element</h3>
</body>
</html>
4. CSS Class Selector
CSS Class Selector selects elements based on the value of the class attribute.
For example, select all the elements with the class = "txt-green" or "txt-green" included.
class-selector-example1.css
.txt-green {
color: green;
}
.bg-yellow {
background-color: yellow;
}
class-selector-example1.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Class Selector</title>
<link rel="stylesheet" type="text/css" href="class-selector-example1.css" />
</head>
<body>
<h1 class ="txt-green">I am H1 with class = 'txt-green'</h1>
<p>I am P element</p>
<h2 class="txt-green bg-yellow">I am H2 with class = 'txt-green bg-yellow'</h2>
</body>
</html>
Example:
class-selector-example2.css
/* <p> with class 'txt-green' or includes 'text-green' */
p.txt-green {
color: green;
}
.bg-yellow {
background-color: yellow;
}
/* <div> with class inculudes both 'text-green' and 'bg-yellow' */
div.txt-green.bg-yellow {
color: green;
font-style: italic;
}
class-selector-example2.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>Class Selector</title>
<link rel="stylesheet" type="text/css" href="class-selector-example2.css" />
</head>
<body>
<h1 class ="txt-green">I am H1 with class = 'txt-green'</h1>
<p class ="txt-green">I am P element with class='txt-green'</p>
<div class="txt-green bg-yellow">I am DIV with class = 'txt-green bg-yellow'</div>
<br/>
<span class="txt-green bg-yellow">I am SPAN with class = 'txt-green bg-yellow'</span>
</body>
</html>
5. CSS ID Selector
CSS ID Selector helps you select elements based on the value of the ID attribute. The value of the ID attribute must exactly match the value given by Selector. Note: The CSS ID Selector is "Case insensitive".
id-selector-example.css
#demo {
color: blue;
font-size: 30px;
}
id-selector-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8"/>
<title>ID Selector</title>
<link rel="stylesheet" type="text/css" href="id-selector-example.css" />
</head>
<body>
<h1 id = "demo">Demo</h1>
<span>I am span element</span>
<p>I am P element</p>
</body>
</html>
CSS Tutorials
- Units in CSS
- Basic CSS Selectors Tutorial with Examples
- CSS Attribute Selector Tutorial with Examples
- CSS combinator Selectors Tutorial with Examples
- CSS Backgrounds Tutorial with Examples
- CSS Opacity Tutorial with Examples
- CSS Padding Tutorial with Examples
- CSS Margins Tutorial with Examples
- CSS Borders Tutorial with Examples
- CSS Outline Tutorial with Examples
- CSS box-sizing Tutorial with Examples
- CSS max-width and min-width Tutorial with Examples
- The keywords min-content, max-content, fit-content, stretch in CSS
- CSS Links Tutorial with Examples
- CSS Fonts Tutorial with Examples
- Understanding Generic Font Family Names in CSS
- CSS @font-face Tutorial with Examples
- CSS Align Tutorial with Examples
- CSS Cursors Tutorial with Examples
- CSS Overflow Tutorial with Examples
- CSS Lists Tutorial with Examples
- CSS Tables Tutorial with Examples
- CSS visibility Tutorial with Examples
- CSS Display Tutorial with Examples
- CSS Grid Layout Tutorial with Examples
- CSS Float and Clear Tutorial with Examples
- CSS Position Tutorial with Examples
- CSS line-height Tutorial with Examples
- CSS text-align Tutorial with Examples
- CSS text-decoration Tutorial with Examples
Show More