Bootstrap Border Utilities Tutorial with Examples
1. Border Utility
Border Utility means part of Bootstrap. It provides classes to help users to set up border for elements.
Lớp | Description |
.border | Set up border for 4 sides of element. |
.border-left | Set up border for the left side of element. |
.border-right | Set up border for the right side of element. |
.border-top | Set up border for the top side of element. |
.border-bottom | Set up border for the bottom side of element. |
.border-left-0 | Set up border for all sides, except for left side. |
.border-right-0 | Set up border for all sides, except for right side. |
.border-top-0 | Set up border for all sides, except for top side. |
.border-bottom-0 | Set up border for all sides, except for bottom side. |
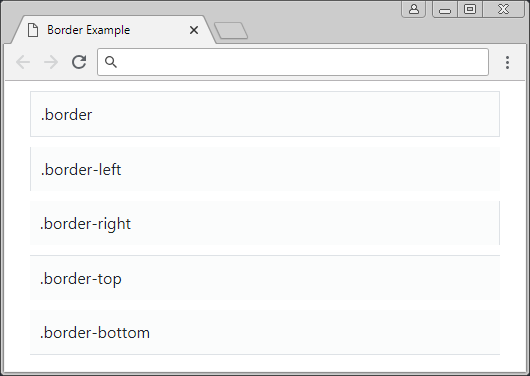
border-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Border Example</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css">
<style>
.container div {
margin: 10px;
padding :10px;
background: #fbfcfc;
}
</style>
</head>
<body>
<div class="container">
<div class="border ">
.border
</div>
<div class="border-left ">
.border-left
</div>
<div class="border-right ">
.border-right
</div>
<div class="border-top ">
.border-top
</div>
<div class="border-bottom ">
.border-bottom
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"></script>
</body>
</html>
2. Border color
There are a few classes to set up color for border built-in by the Bootstrap. You can use it in a suitable context:
- .border-primary
- .border-secondary
- .border-success
- .border-danger
- .border-warning
- .border-info
- .border-light
- .border-dark
- .border-muted
- .border-white
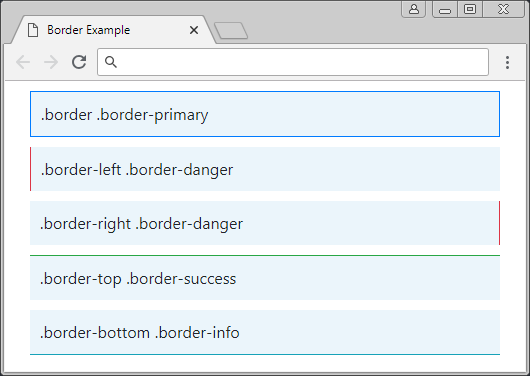
border-color-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Border Example</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css">
<style>
.container div {
margin: 10px;
padding :10px;
background: #ebf5fb;
}
</style>
</head>
<body>
<div class="container">
<div class="border border-primary">
.border .border-primary
</div>
<div class="border-left border-danger">
.border-left .border-danger
</div>
<div class="border-right border-danger">
.border-right .border-danger
</div>
<div class="border-top border-success">
.border-top .border-success
</div>
<div class="border-bottom border-info">
.border-bottom .border-info
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"></script>
</body>
</html>
3. Border width
Bootstrap doesn't define any class to set up the width of border. But, you can self-definite such classes, for example, .border-* (* = 1, 2, 3, ...).
.border-5 {
border-width:5px !important;
}
.border-2 {
border-width:2px !important;
}
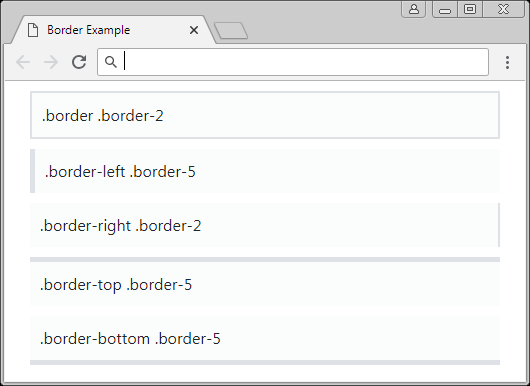
border-width-example.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Border Example</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css">
<style>
.container div {
margin: 10px;
padding :10px;
background: #fbfcfc;
}
.border-5 {
border-width:5px !important;
}
.border-2 {
border-width:2px !important;
}
</style>
</head>
<body>
<div class="container">
<div class="border border-2">
.border .border-2
</div>
<div class="border-left border-5">
.border-left .border-5
</div>
<div class="border-right border-2">
.border-right .border-2
</div>
<div class="border-top border-5">
.border-top .border-5
</div>
<div class="border-bottom border-5">
.border-bottom .border-5
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"></script>
</body>
</html>
4. Border-Radius
Some additional classes help you to round element angles.
- .rounded
- .rounded-left
- .rounded-right
- .rounded-top
- .rounded-bottom
- .rounded-0
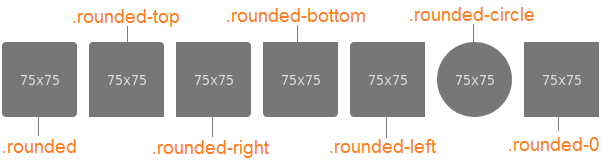
round-example
<div class="container">
<div class="border rounded">
.border .rounded
</div>
<div class="border rounded-left">
.border-left .rounded-left
</div>
<div class="border rounded-right">
.border .rounded-right
</div>
<div class="border rounded-top">
.border .rounded-top
</div>
<div class="border rounded-bottom">
.border .rounded-bottom
</div>
<div class="border rounded-circle">
.border-bottom .rounded-circle
</div>
<div class="border rounded-0">
.border-bottom .rounded-0
</div>
</div>
Bootstrap Tutorials
- Bootstrap Jumbotron Tutorial with Examples
- Bootstrap Dropdowns Tutorial with Examples
- Bootstrap Alerts Tutorial with Examples
- Bootstrap Buttons Tutorial with Examples
- Bootstrap Button Group Tutorial with Examples
- Bootstrap Popovers (Tooltips) Tutorial with Examples
- Bootstrap Spinners Tutorial with Examples
- Introduction to Bootstrap
- Bootstrap Grid System Tutorial with Examples
- Bootstrap Cards Tutorial with Examples
- Bootstrap Containers Tutorial with Examples
- Bootstrap Nav Tab/Pill Tutorial with Examples
- Bootstrap NavBars Tutorial with Examples
- Bootstrap Tables Tutorial with Examples
- Bootstrap Modal Tutorial with Examples
- Bootstrap Forms Tutorial with Examples
- Bootstrap Pagination Tutorial with Examples
- Bootstrap Badges Tutorial with Examples
- Bootstrap Input Group Tutorial with Examples
- Bootstrap List Groups Tutorial with Examples
- Bootstrap ProgressBars Tutorial with Examples
- Bootstrap Collapse and Accordion Tutorial with Examples
- Bootstrap Scrollspy Tutorial with Examples
- Bootstrap Breadcrumb Tutorial with Examples
- Bootstrap Carousel Tutorial with Examples
- Bootstrap Spacing Utilities Tutorial with Examples
- Bootstrap Border Utilities Tutorial with Examples
- Bootstrap Color Utilities Tutorial with Examples
- Bootstrap Text Utilities Tutorial with Examples
- Bootstrap Sizing Utilities Tutorial with Examples
- Bootstrap Position Utilities Tutorial with Examples
- Bootstrap Flex Utilities Tutorial with Examples
- Bootstrap Display Utilities Tutorial with Examples
- Bootstrap Visibility Utilities Tutorial with Examples
- Bootstrap Embed Utilities Tutorial with Examples
Show More