Create Java OSGi project with Maven and Tycho
1. Introduction
This document is based on:
- Eclipse 3.4 (LUNA)
- Tycho 0.20.0
We must first ensure that you have installed Tycho on Eclipse. If not installed you can see the instructions here:
2. Quick Create OSGi Project (SimpleOSGi)
First we create an OSGi project quickly accordance usual way.
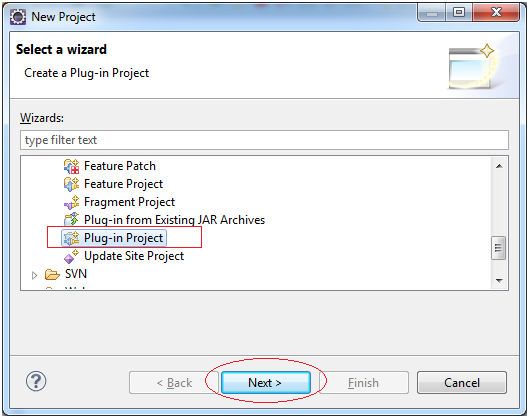
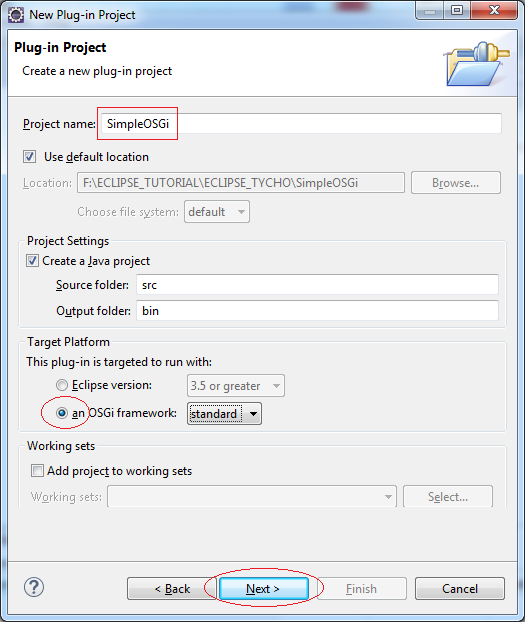
Enter:
- Version: 2.0.0.qualifier
- Activator: org.o7planning.tutorial.simpleosgi.Activator
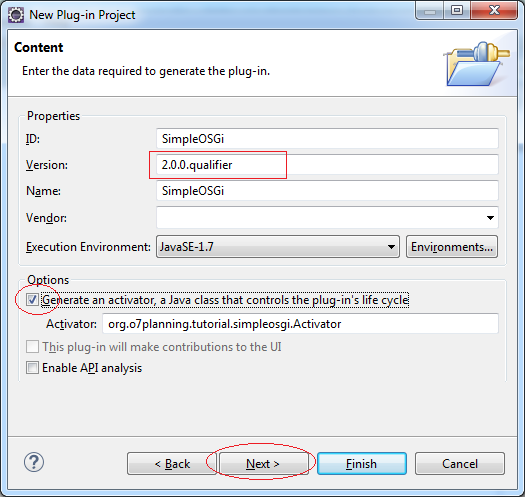
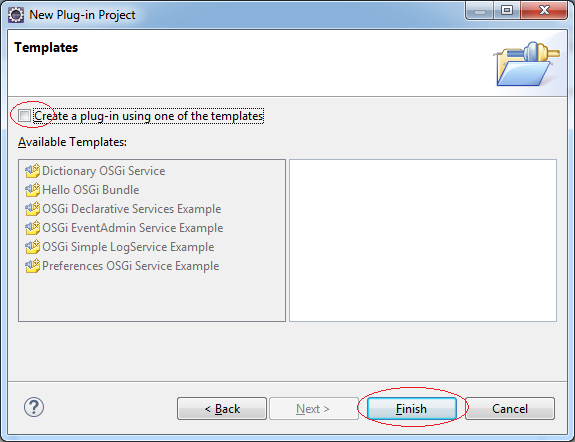
Add:
- org.eclipse.osgi
- org.eclipse.equinox.console
- org.apache.felix.gogo.command
- org.apache.felix.gogo.runtime
- org.apache.felix.gogo.shell
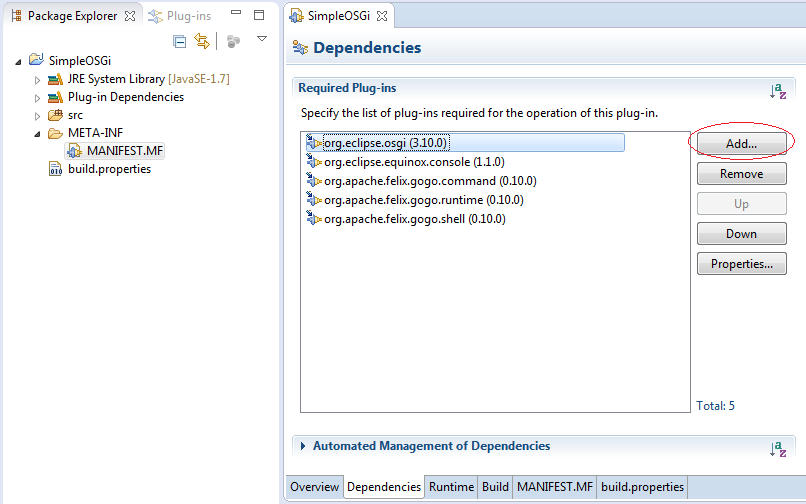
Activator.java
package org.o7planning.tutorial.simpleosgi;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("SimpleOSGi Started");
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("SimpleOSGi Stopped");
}
}
3. Run OSGi (SimpleOSGi)
We'll quickly configure and run this OSGi, to see that the status of the OSGi just created is good.
Right-click on the Project SimpleOSGi, select "Run As / Run Configuration .."
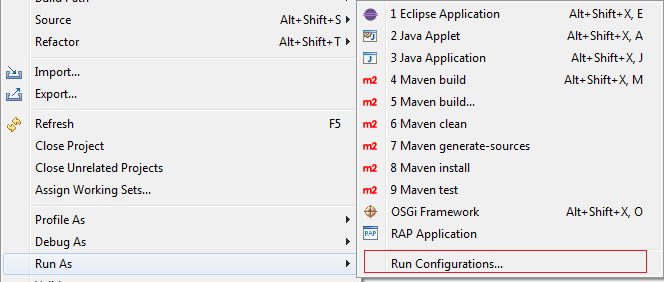
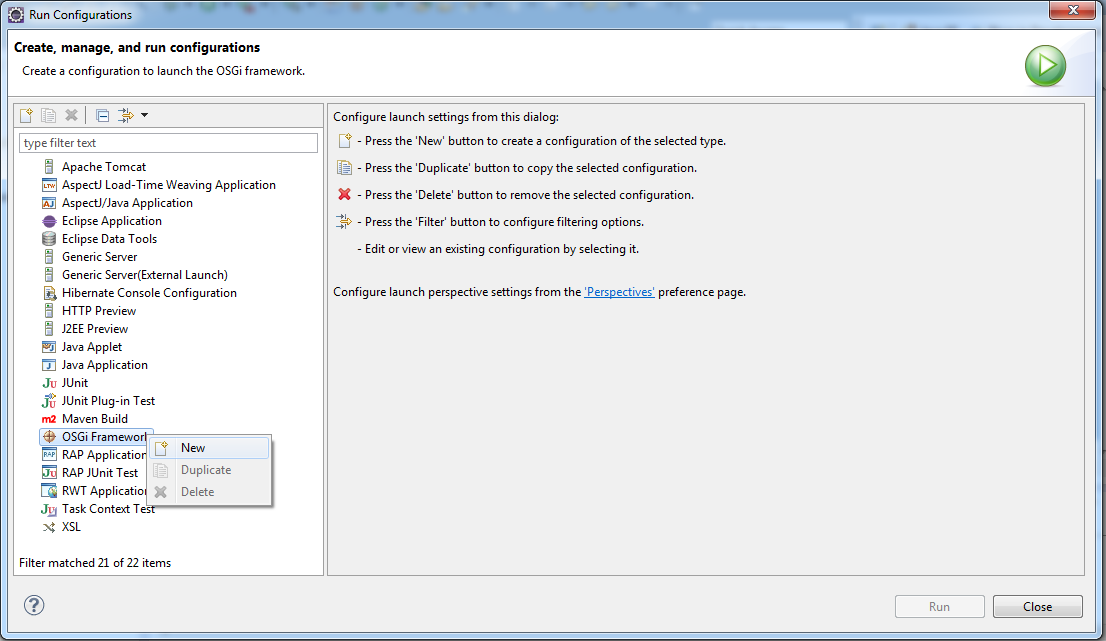
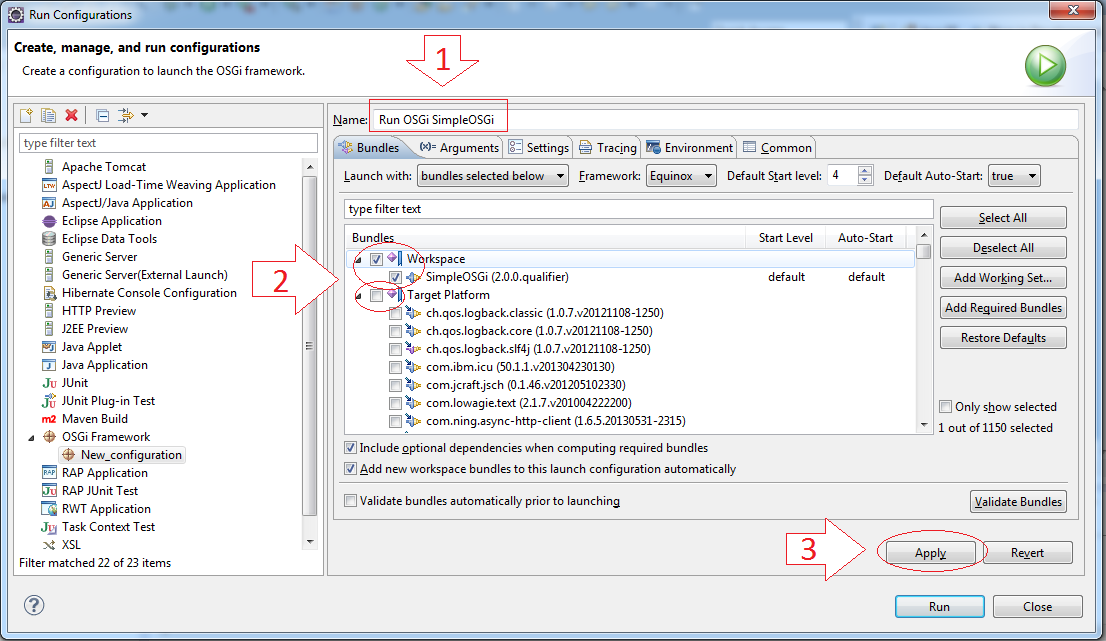
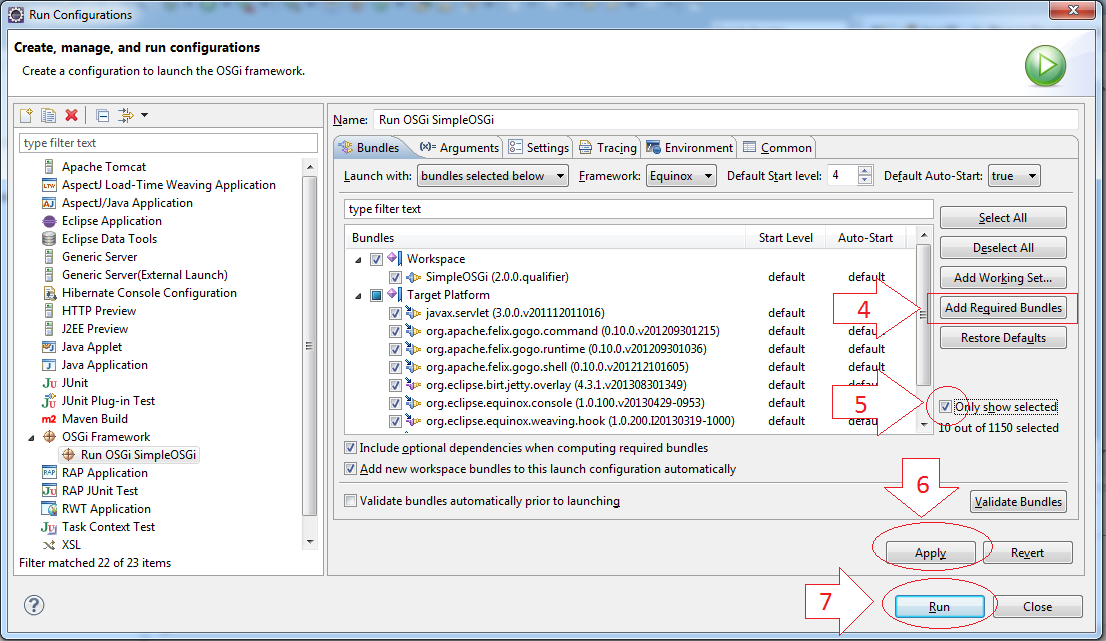
Running OSGi, and get successful results.
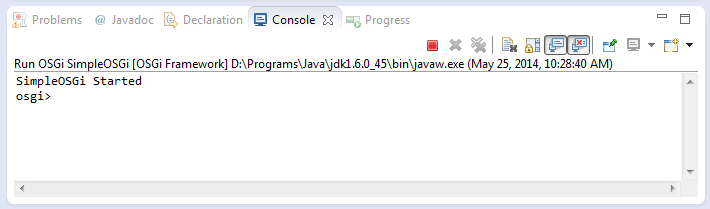
4. Quick Create Maven Project (SimpleMaven)
Next we will quick create Maven Project. Goals for the future, we will build 2 project (SimpleOSGi & SimpleMaven) with Maven & Tycho. And see that both project to be treated equally.
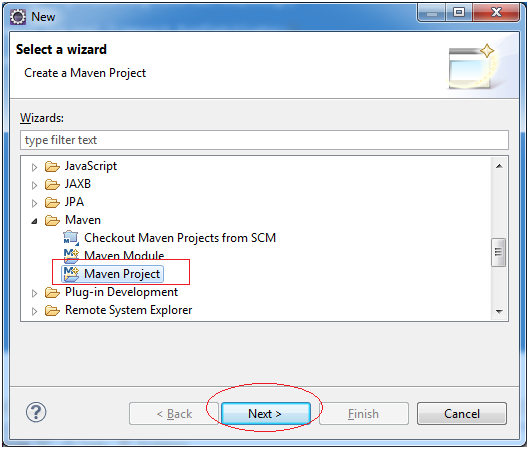
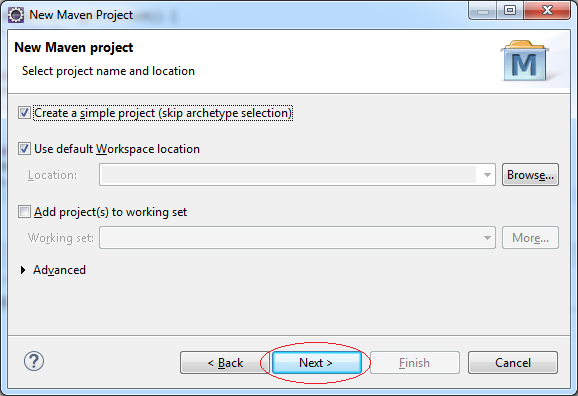
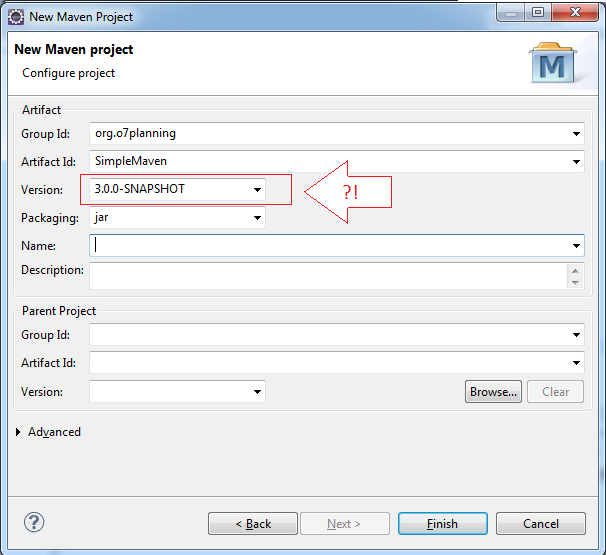
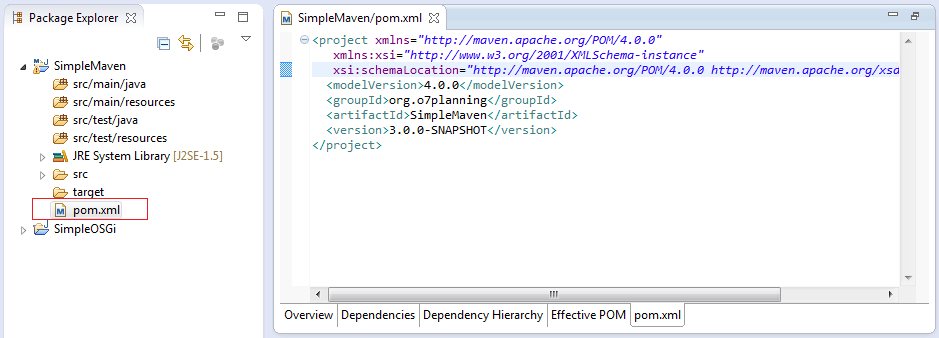
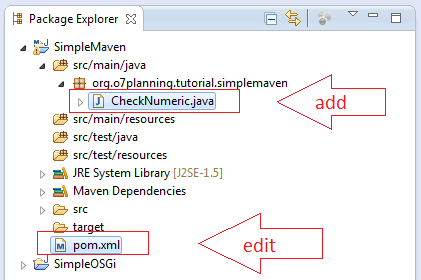
CheckNumeric.java
package org.o7planning.tutorial.simplemaven;
import org.apache.commons.lang3.StringUtils;
public class CheckNumeric {
public static void main(String[] args) {
String text1 = "0123a4";
String text2 = "01234";
boolean result1 = StringUtils.isNumeric(text1);
boolean result2 = StringUtils.isNumeric(text2);
System.out.println(text1 + " is a numeric? " + result1);
System.out.println(text1 + " is a numeric? " + result2);
}
}
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
</project>
5. Create MavenParent Project
The purpose of this project to configure Maven & Tycho to build two projects (SimpleOSGi & SimpleMaven).
Create a common Java Project
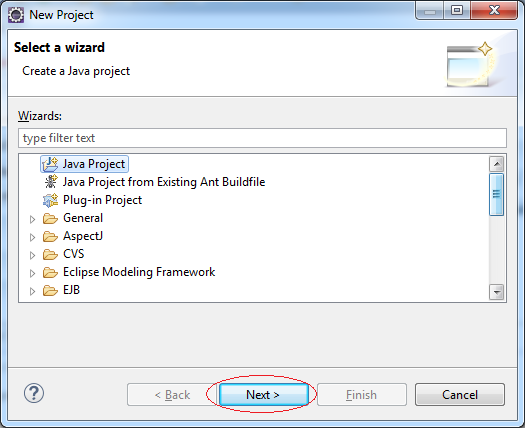
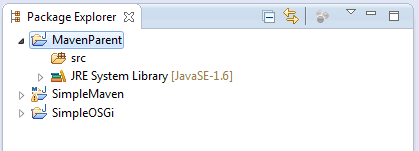
6. Configuring the OSGi project (SimpleOSGi)
First we convert SimpleOSGi into Maven Project. SimpleOSGi will be a Maven plugin and OSGi Project
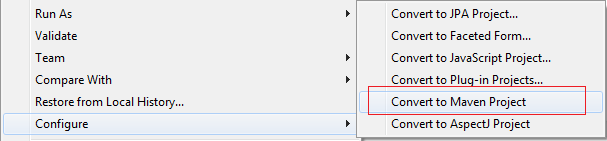
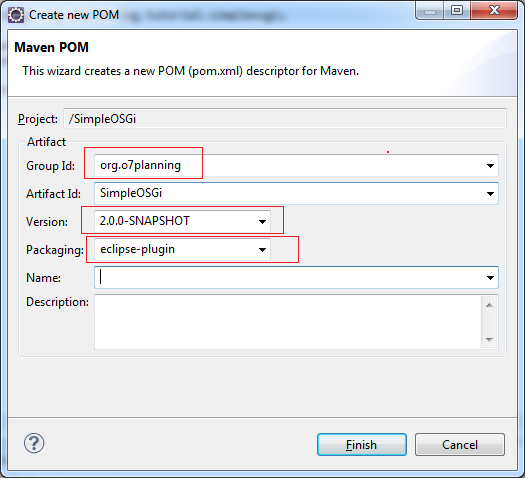
Project has been converted into a Maven project, and have error message, you do not need to worry about that.
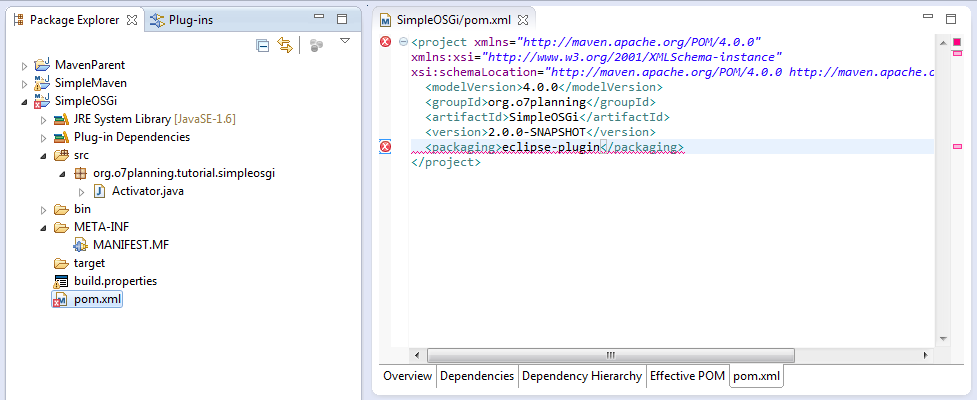
Note that if your OSGi Project configure version is AAA and suffix is qualifier, then on Maven you must also configure in the pom.xml file have version AAA and the suffix is SNAPSHOT. Like the illustration below.
Open the pom.xml file and reconfigure as follows:
SimpleOSGi/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleOSGi</artifactId>
<version>2.0.0-SNAPSHOT</version>
<packaging>eclipse-plugin</packaging>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
7. Configuring Maven Project (SimpleMaven)
Open pom.xml file on Project SimpleMaven and add the following code:
**
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
SimpleMaven/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMaven</artifactId>
<version>3.0.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
</dependencies>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
8. Configuring Maven Parent
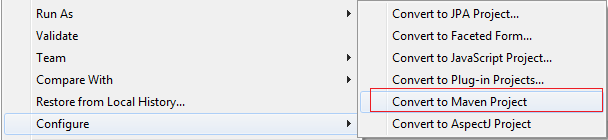
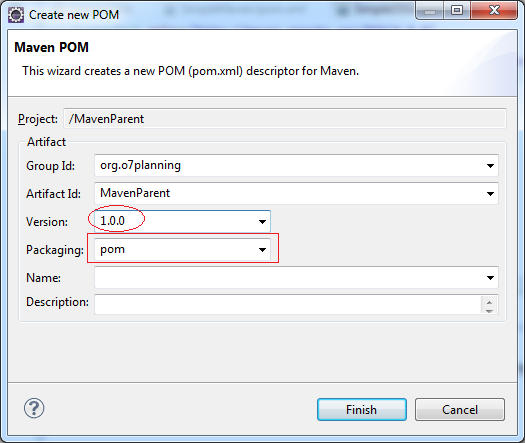
MavenParent/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0</version>
<packaging>pom</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<!-- 0.22.0 : Target location type: Directory is not supported -->
<tycho-version>0.22.0</tycho-version>
</properties>
<repositories>
<!-- Select the P2 repository to be used when resolving dependencies -->
<repository>
<id>eclipse-luna</id>
<layout>p2</layout>
<url>http://download.eclipse.org/releases/luna</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>tycho-snapshots</id>
<url>https://oss.sonatype.org/content/groups/public/</url>
</pluginRepository>
</pluginRepositories>
<build>
<plugins>
<plugin>
<!-- enable tycho build extension -->
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-maven-plugin</artifactId>
<version>${tycho-version}</version>
<extensions>true</extensions>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>tycho-versions-plugin</artifactId>
<version>${tycho-version}</version>
<configuration>
<dependency-resolution>
<optionalDependencies>ignore</optionalDependencies>
</dependency-resolution>
</configuration>
</plugin>
<!--
<plugin>
<groupId>org.eclipse.tycho</groupId>
<artifactId>target-platform-configuration</artifactId>
<version>${tycho-version}</version>
<configuration>
<target>
<artifact>
<groupId>org.o7planning</groupId>
<artifactId>TargetPlatform</artifactId>
<version>1.0.0</version>
</artifact>
</target>
</configuration>
</plugin>
-->
</plugins>
</build>
<modules>
<module>../SimpleMaven</module>
<module>../SimpleOSGi</module>
</modules>
</project>
Eclipse Technology
- How to get the open source Java libraries as OSGi(s)
- Install Tycho for Eclipse
- Java OSGi Tutorial for Beginners
- Create Java OSGi project with Maven and Tycho
- Install WindowBuilder for Eclipse
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Programming Java Desktop Application Using SWT
- Eclipse JFace Tutorial with Examples
- Install e4 Tools Developer Resources for Eclipse
- Package and Deploy Desktop Application SWT/RCP
- Install Eclipse RAP Target Platform
- Install EMF for Eclipse
- Install RAP e4 Tooling for Eclipse
- Create Eclipse RAP Widget from ClientScripting-based widget
- Install GEF for Eclipse
- Eclipse RAP Tutorial for Beginners - Workbench Application (OLD)
- Eclipse RCP 3 Tutorial for Beginners - Workbench Application
- Simple Eclipse RCP 3 Application - View and Editor integration
- Eclipse RCP 4 Tutorial for Beginners - e4 Workbench Application
- Install RAP Tools for Eclipse
- Eclipse RAP Tutorial for Beginners - Basic Application
- Eclipse RAP Tutorial for Beginners - e4 Workbench Application
- Package and deploy Eclipse RAP application
Show More
Maven Tutorials
- Install Maven for Eclipse
- Maven Tutorial for Beginners
- Maven Manage Dependencies
- Build a Multiple Module Project with Maven
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Install Tycho for Eclipse
- Create Java OSGi project with Maven and Tycho
- Create an Empty Maven Web App project in Eclipse
- OSGi and AspectJ integration
Show More