Maven Manage Dependencies
1. How are the libraries interdependent in Maven
Maven is a excellent tool which helps you explore the world of open source in Java. But sometimes, you do not understand enough about Maven to control it. And so there may be some unexplained error occurred.
In this document, I would guide you how to avoid duplication library in Maven.
To make things easier you should see the contents of following pom.xml file:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMavenWebApp</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SimpleMavenWebApp Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- JSTL Library -->
<!-- http://mvnrepository.com/artifact/org.glassfish.web/javax.servlet.jsp.jstl -->
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>javax.servlet.jsp.jstl</artifactId>
<version>1.2.4</version>
</dependency>
</dependencies>
<build>
<finalName>SimpleMavenWebApp</finalName>
</build>
</project>
pom.xml file above you declared using javax.servlet.jsp.jstl library, this library depends on jstl-api. And jstl-api depends on two libraries servlet-api and jsp-api. The dependence is like the illustration below:
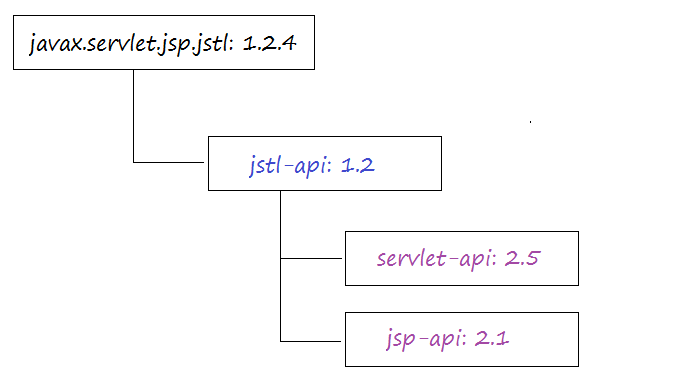
You can see this dependency tree on Eclipse
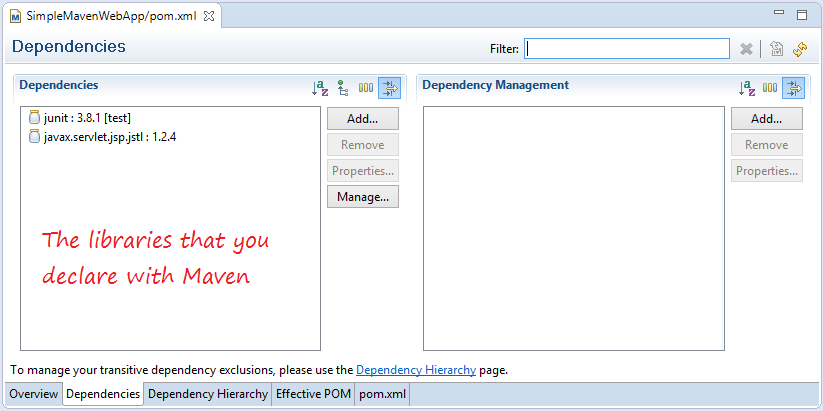
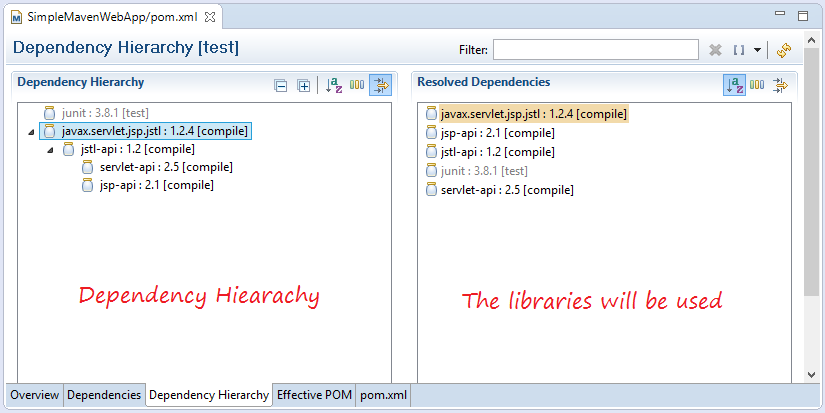
The library that will be used is the library you declared to Maven and dependent libraries.
2. Exclude duplicate library
When declaring library in Maven, library duplication may be happened. For example, you declare use of two library A and B with Maven. A depends on C and D, and B depends on C and E.That means the C library duplicate. You can see the illustration below.
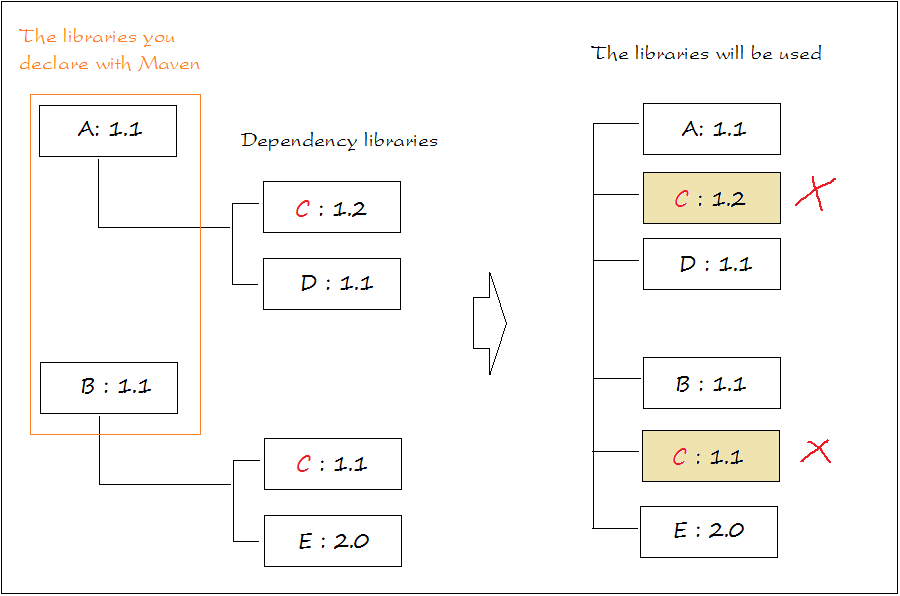
The illustration above shows that there are two versions of C library will be used, which may cause errors in runtime of the application. You need to configure to remove one of two versions of the library C. Normally, you would remove the older version.
Using <exclusions> to remove the dependent libraries that you do not want to use.
Using <exclusions> to remove the dependent libraries that you do not want to use.
<dependencies>
<dependency>
<groupId>aa</groupId>
<artifactId>A</artifactId>
<version>1.1</version>
</dependency>
<dependency>
<groupId>bb</groupId>
<artifactId>B</artifactId>
<version>1.1</version>
<exclusions>
<exclusion>
<artifactId>C</artifactId>
<groupId>cc</groupId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
Eclipse tools support you in detecting and eliminating duplication. Take an example:
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>SimpleMavenWebApp</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>SimpleMavenWebApp Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Servlet API - Servlet Library -->
<!-- http://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- JSTL Library -->
<!-- http://mvnrepository.com/artifact/org.glassfish.web/javax.servlet.jsp.jstl -->
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>javax.servlet.jsp.jstl</artifactId>
<version>1.2.4</version>
</dependency>
</dependencies>
<build>
<finalName>SimpleMavenWebApp</finalName>
</build>
</project>
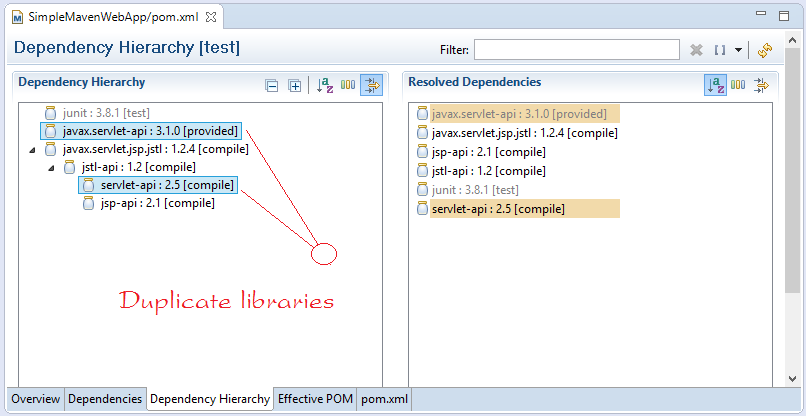
Right-click the dependent library that needs to exclude, and choose Exclude Maven Artifact ...
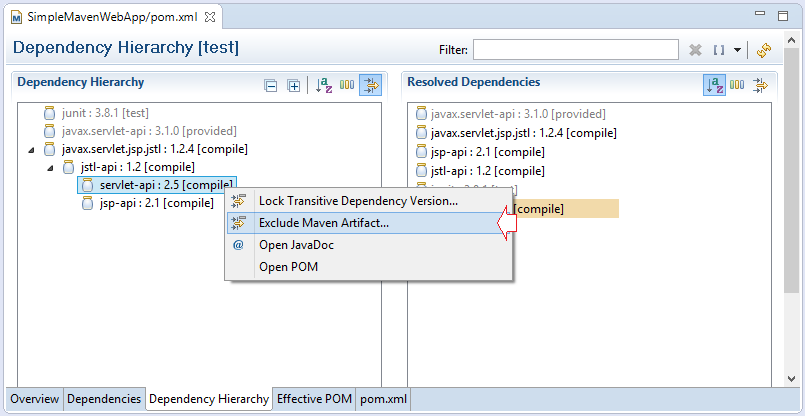
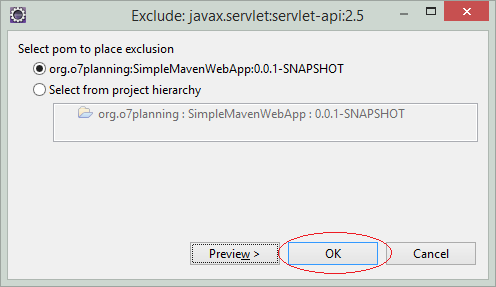
Eclipse will automatically add the <exclusions> code in pom.xml:
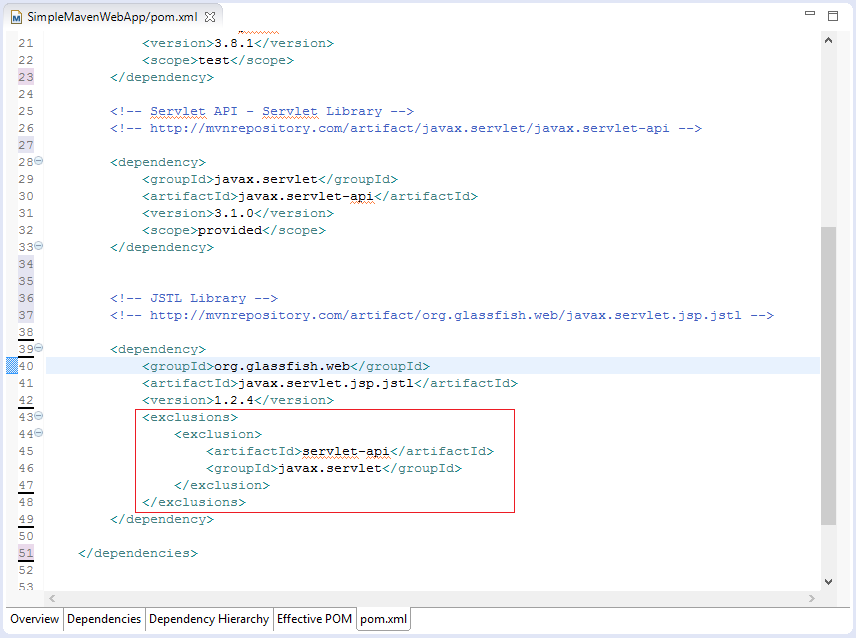
Here, there is no duplication library in your pom.xml file
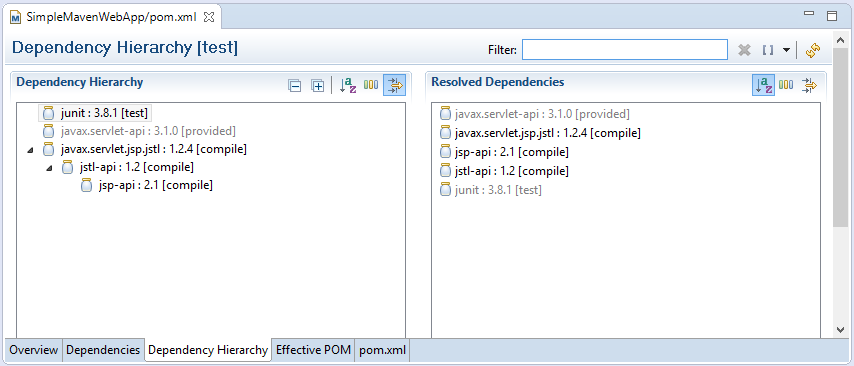
Maven Tutorials
- Install Maven for Eclipse
- Maven Tutorial for Beginners
- Maven Manage Dependencies
- Build a Multiple Module Project with Maven
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Install Tycho for Eclipse
- Create Java OSGi project with Maven and Tycho
- Create an Empty Maven Web App project in Eclipse
- OSGi and AspectJ integration
Show More