Java OSGi Tutorial for Beginners
2. Create OSGi "MathConsumer"
Create Project MathConsumer
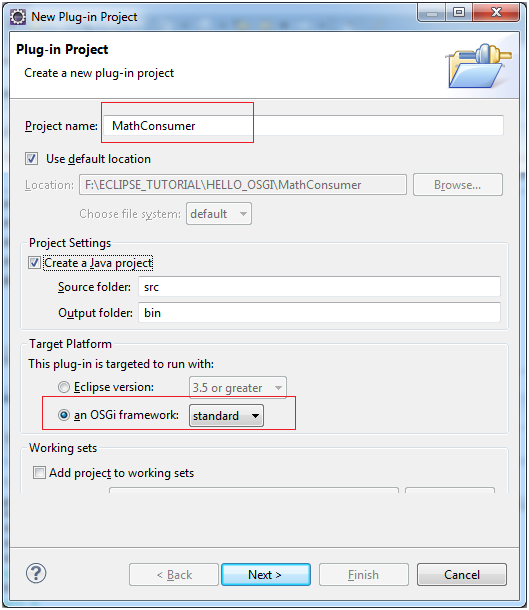
- org.o7planning.tutorial.helloosgi.mathconsumer.Activator
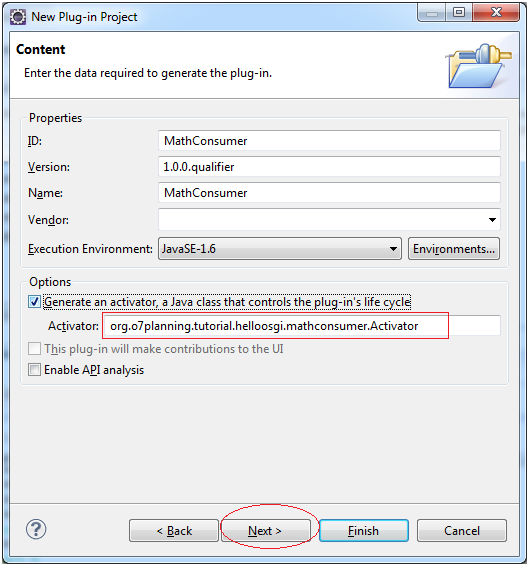
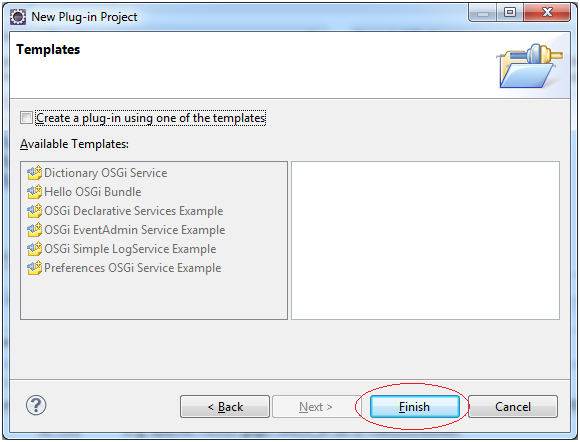
This is image after Project was created .
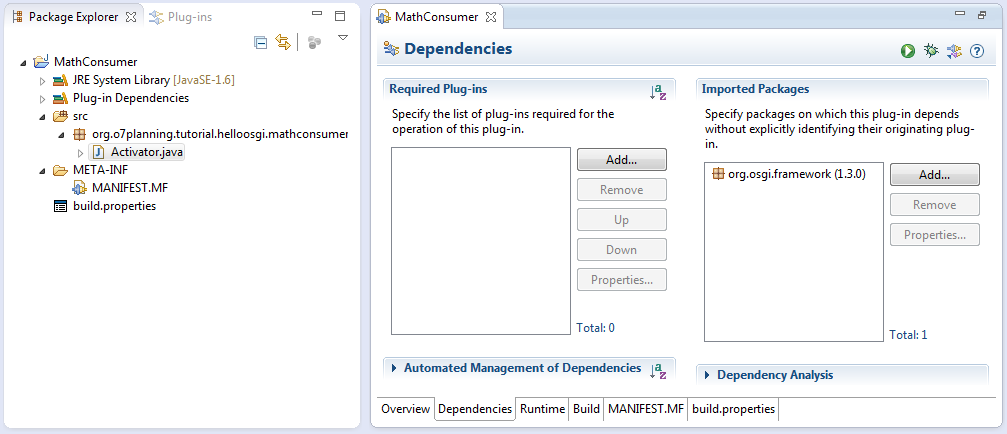
Open class Activator to edit the code:
Activator.java
package org.o7planning.tutorial.helloosgi.mathconsumer;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("MathConsumer Starting...");
System.out.println("MathConsumer Started");
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("MathConsumer Stopped");
}
}
Configure the dependency bundles for MathConsumer
MathConsumer is an OSGi also known as a Bundle. Now we will declare MathConsumer using some other Bundle, purpose to be able to run MathConsumer later.
- org.eclipse.osgi
- org.eclipse.equinox.console
- org.apache.felix.gogo.command
- org.apache.felix.gogo.runtime
- org.apache.felix.gogo.shell
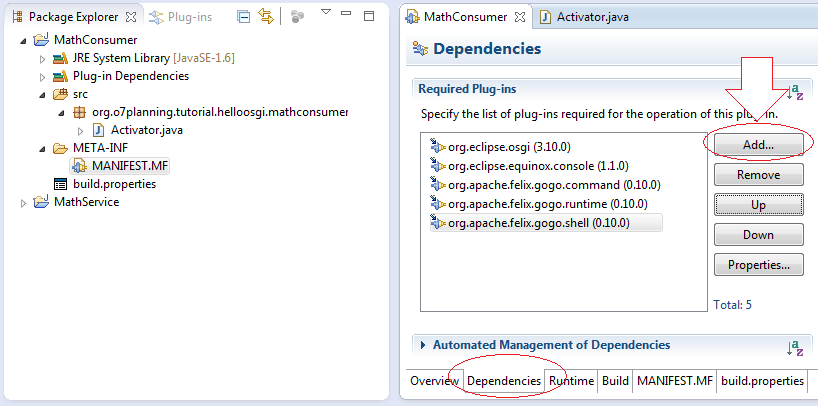
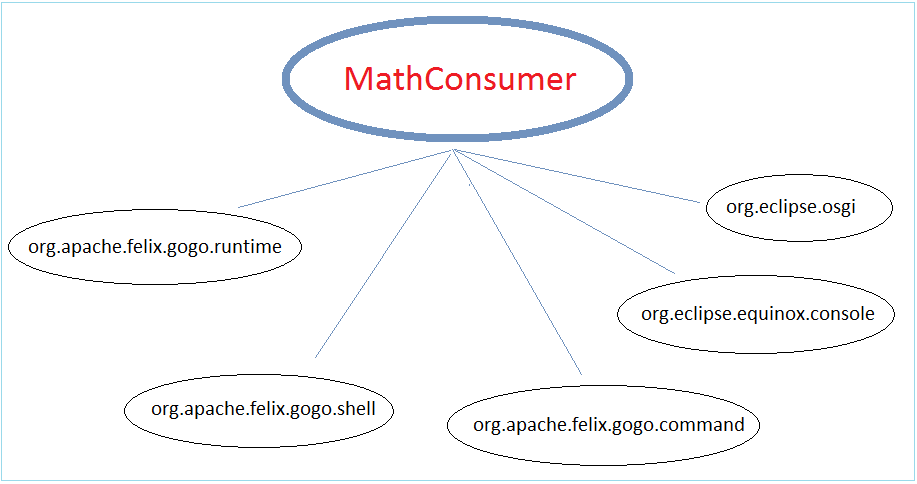
Configuring Eclipse to run MathConsumer
Here we will configure to run directly MathConsumer in Eclipse
Right-click on Project MathService and select "Run As / Run Configuration .."
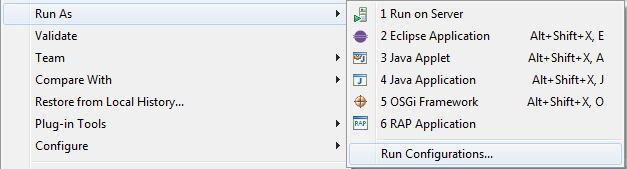
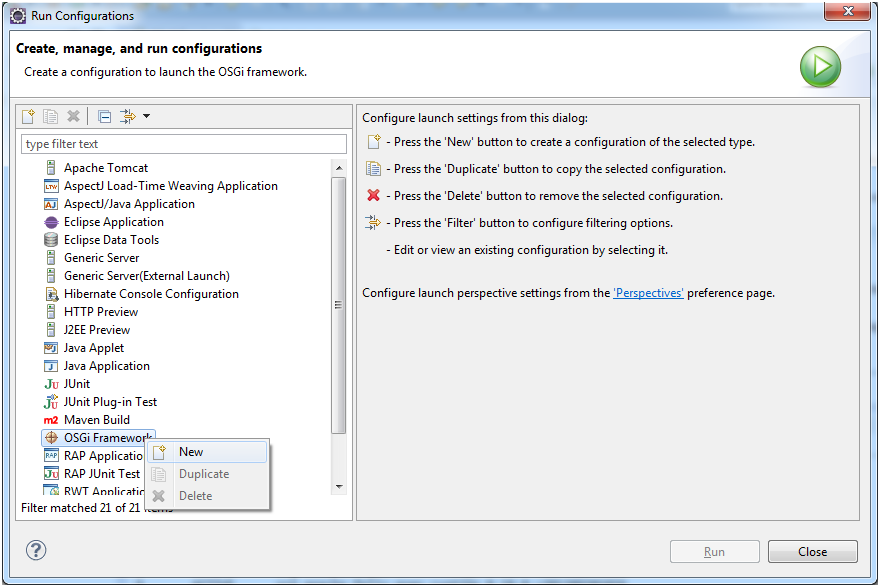
Enter name:
- Run OSGi MathConsumer
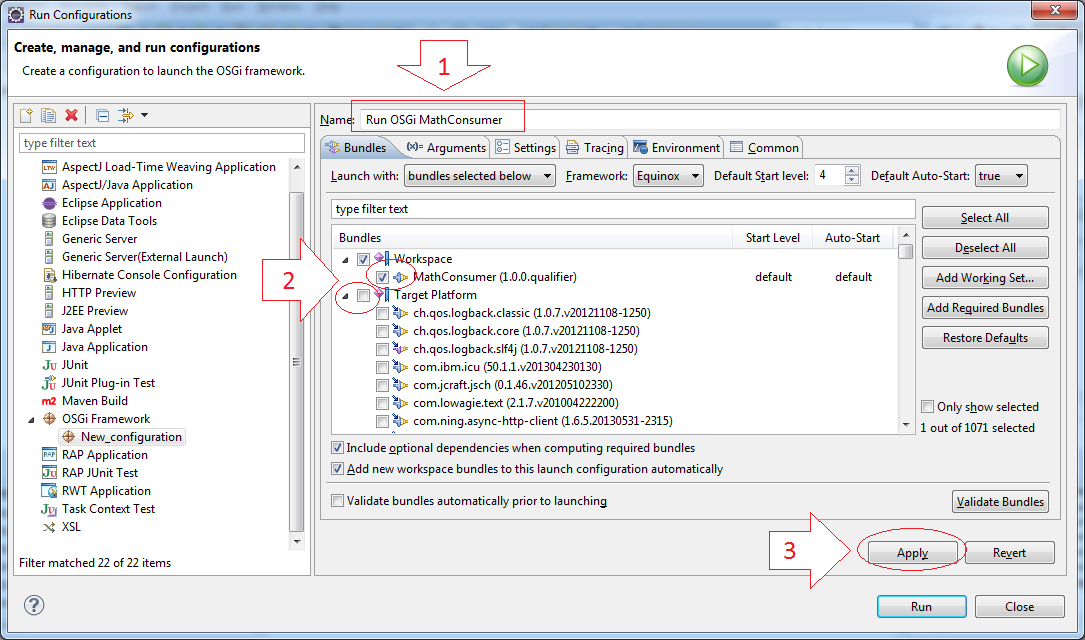
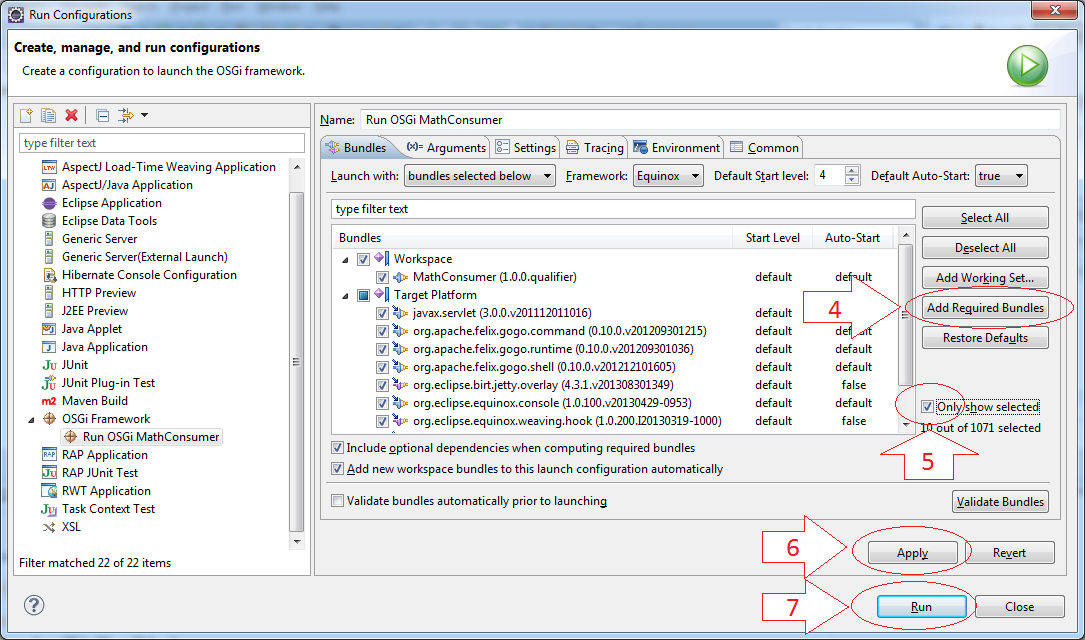
Running MathConsumer
This is the result of running OSGi MathConsumer
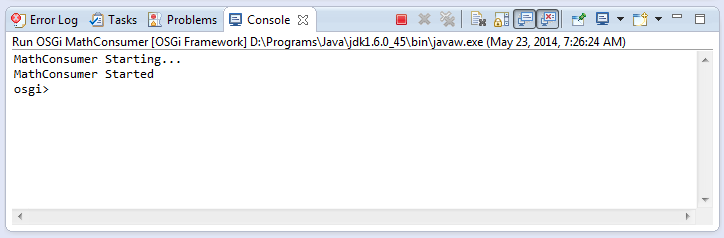
Using the OSGi command ss to see what is running, and their status.
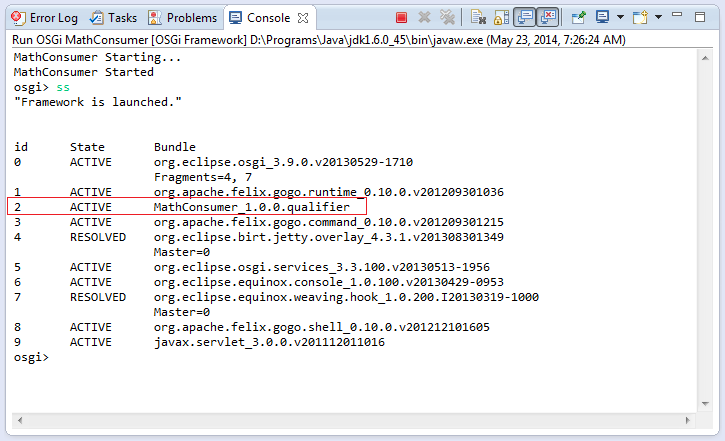
As we see in illustrations, the ID of OSGi MathConsumer is 2, use the command "stop" to stop this Bundle.
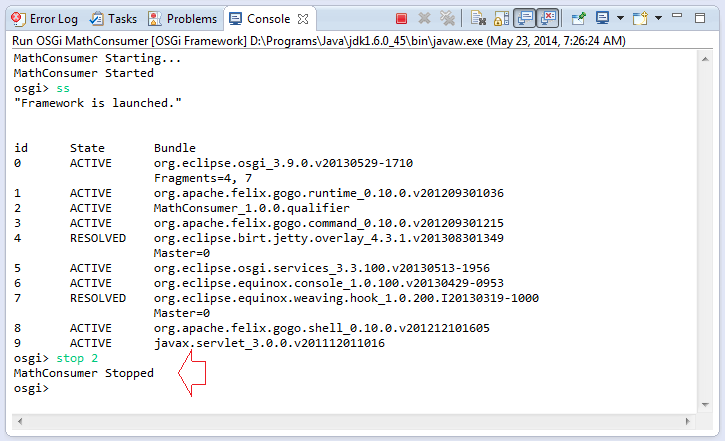
And use the "start" to rerun this OSGi.
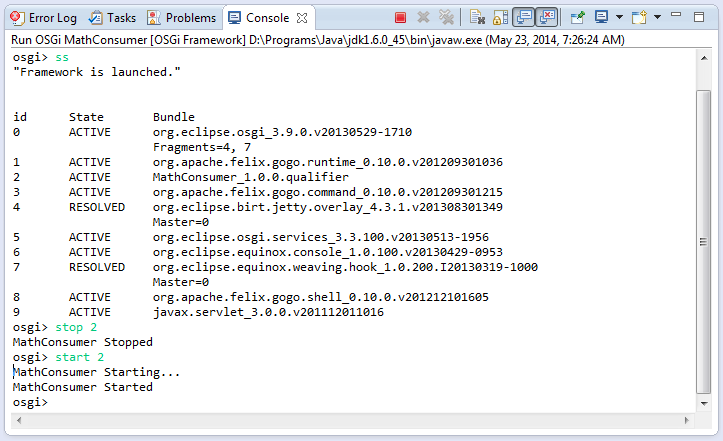
3. Create OSGi "MathService"
Create Project "MathService"
In Eclipse select:
- File/New/Other
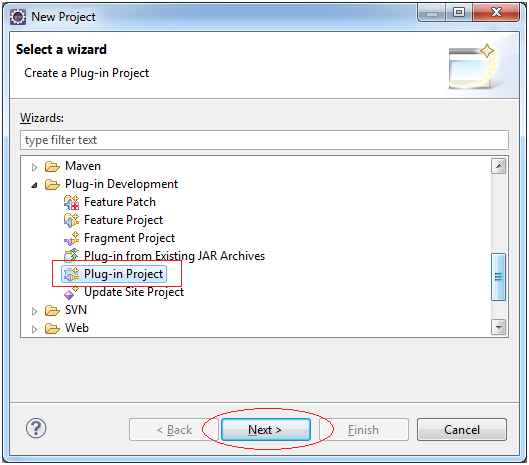
Select the type of OSGi is Standard
- MathService
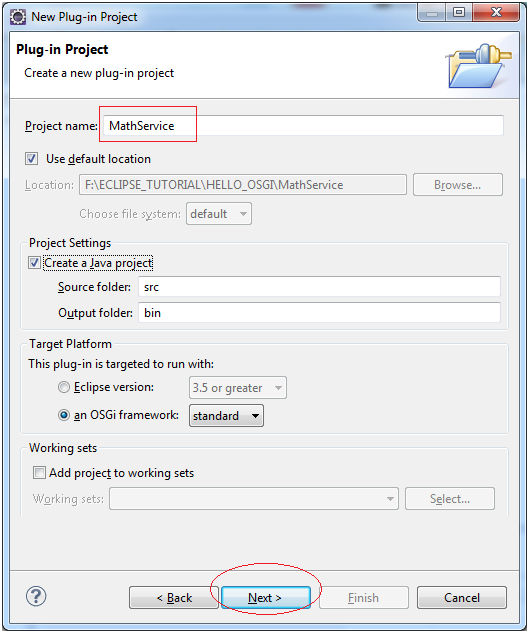
- org.o7planning.tutorial.helloosgi.Activator
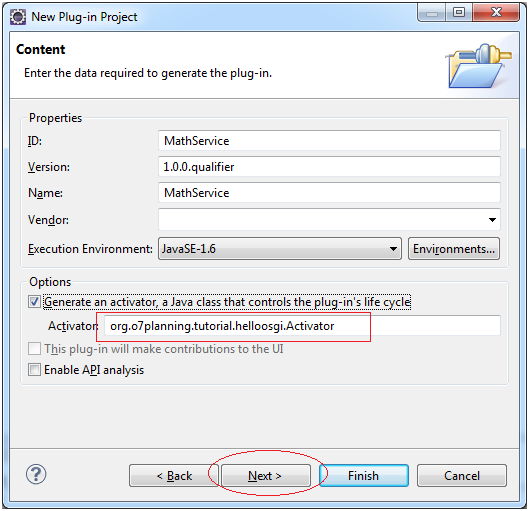
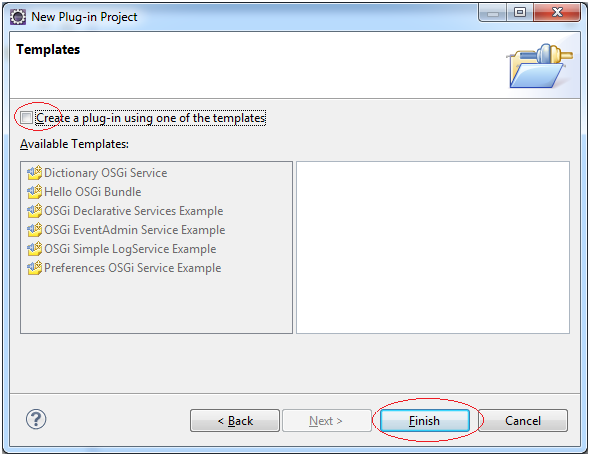
This is project image has been created:
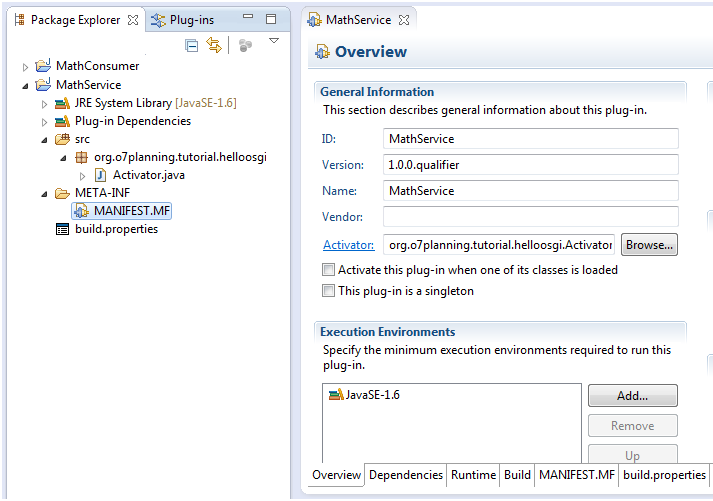
Code Project MathService ®ister MathService serivce
We will add some class to get the final image of the Project as follows:
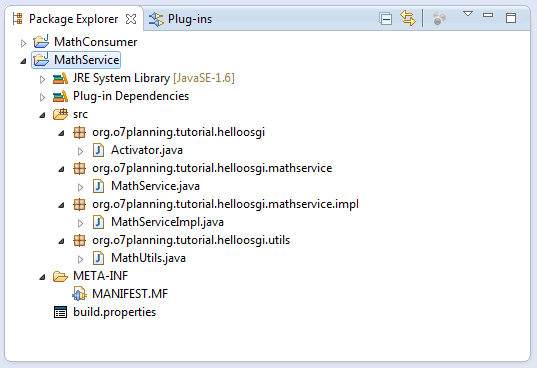
MathService.java
package org.o7planning.tutorial.helloosgi.mathservice;
public interface MathService {
public int sum(int a, int b);
}
MathServiceImpl.java
package org.o7planning.tutorial.helloosgi.mathservice.impl;
import org.o7planning.tutorial.helloosgi.mathservice.MathService;
public class MathServiceImpl implements MathService {
@Override
public int sum(int a, int b) {
return a+ b;
}
}
MathUtils.java
package org.o7planning.tutorial.helloosgi.utils;
public class MathUtils {
public static int minus(int a, int b) {
return a- b;
}
}
And here is an overview picture of class relations have created.
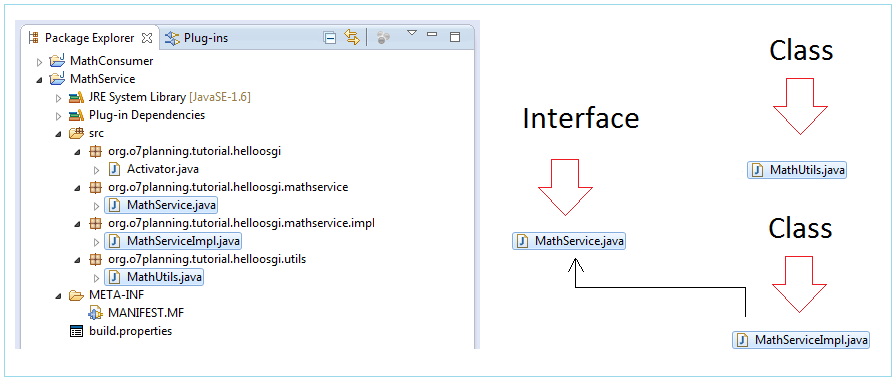
Register MathService service for others can use. This is done in the Activator of OSGi MathService
Activator.java
package org.o7planning.tutorial.helloosgi;
import org.o7planning.tutorial.helloosgi.mathservice.MathService;
import org.o7planning.tutorial.helloosgi.mathservice.impl.MathServiceImpl;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("Registry Service MathService...");
this.registryMathService();
System.out.println("OSGi MathService Started");
}
private void registryMathService() {
MathService service = new MathServiceImpl();
context.registerService(MathService.class, service, null);
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("OSGi MathService Stopped!");
}
}
Configure OSGi MathService and Explains
We configure to export 2 packages org.o7planning.tutorial.helloosgi.utils and org.o7planning.tutorial.helloosgi.mathservice. OSGi is like a closed box, the other OSGi can only use the class/interface is inside the package is exported to the outside.
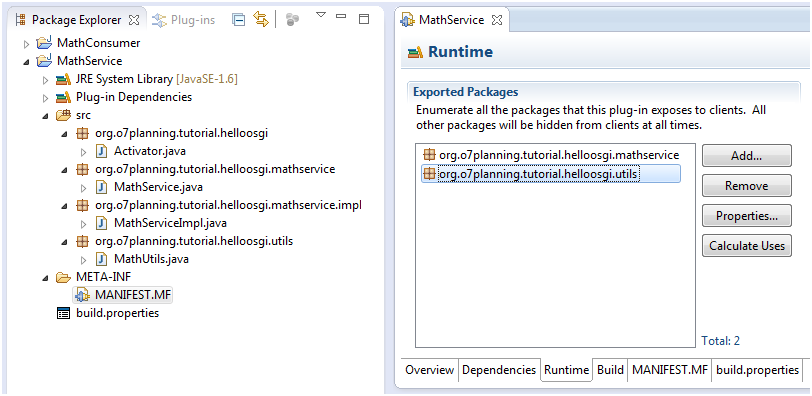
The following figure illustrates OSGi MathService export 2 package:
- org.o7planning.tutorial.helloosgi.mathservice
- org.o7planning.tutorial.helloosgi.utils
OSGi MathConsumer can only use the class/interface located in the export package of the MathService.
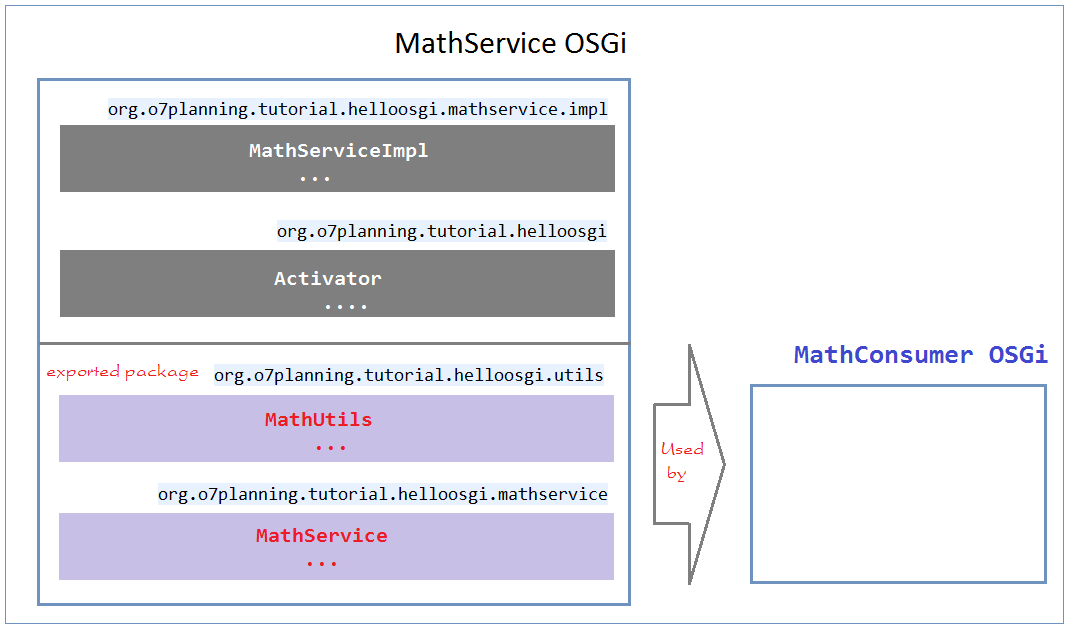
4. Configure MathConsumer use OSGi MathService
Next we will configure to MathConsumer can use OSGi MathService.
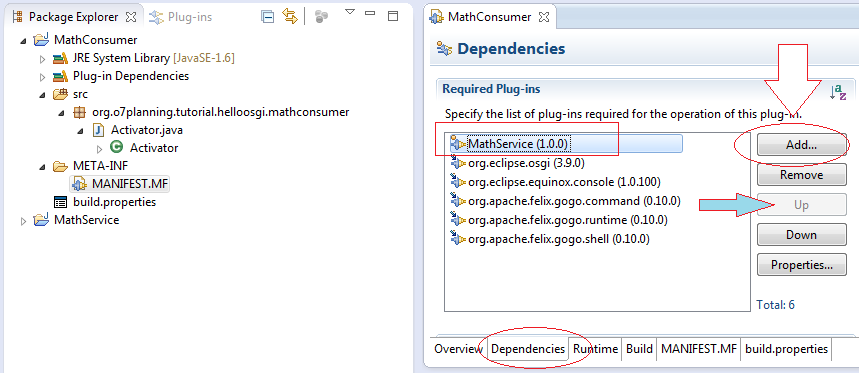
Edit class Activator:
Activator.java
package org.o7planning.tutorial.helloosgi.mathconsumer;
import org.o7planning.tutorial.helloosgi.mathservice.MathService;
import org.o7planning.tutorial.helloosgi.utils.MathUtils;
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceReference;
public class Activator implements BundleActivator {
private static BundleContext context;
static BundleContext getContext() {
return context;
}
public void start(BundleContext bundleContext) throws Exception {
Activator.context = bundleContext;
System.out.println("MathConsumer Starting...");
System.out.println("5-3 = " + MathUtils.minus(5, 3));
//
ServiceReference<?> serviceReference = context
.getServiceReference(MathService.class);
MathService service = (MathService) context
.getService(serviceReference);
System.out.println("5+3 = " + service.sum(5, 3));
System.out.println("MathConsumer Started");
}
public void stop(BundleContext bundleContext) throws Exception {
Activator.context = null;
System.out.println("MathConsumer Stopped");
}
}
Reconfigured to run OSGi MathConsumer
Right-click on the Project MathConsumer select "Run As / Run Configuration .."
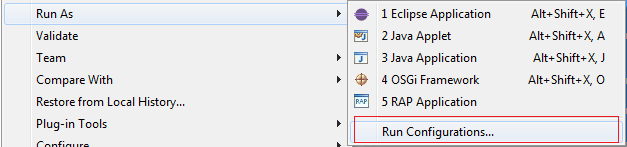
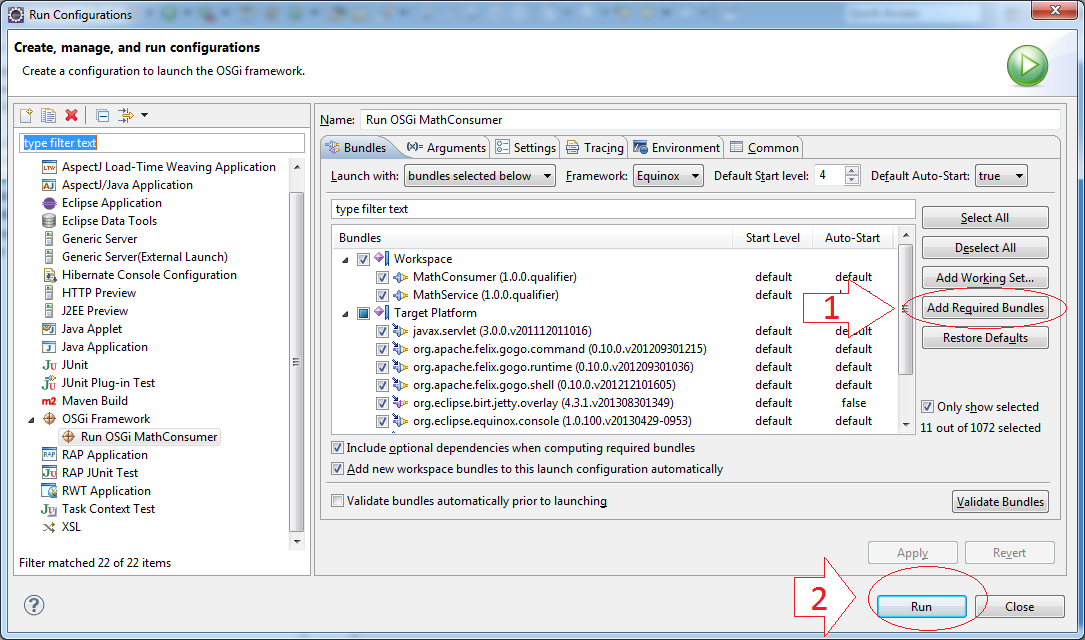
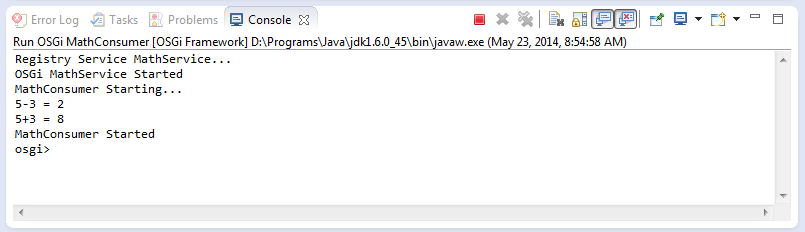
Eclipse Technology
- How to get the open source Java libraries as OSGi(s)
- Install Tycho for Eclipse
- Java OSGi Tutorial for Beginners
- Create Java OSGi project with Maven and Tycho
- Install WindowBuilder for Eclipse
- Which Platform Should You Choose for Developing Java Desktop Applications?
- Programming Java Desktop Application Using SWT
- Eclipse JFace Tutorial with Examples
- Install e4 Tools Developer Resources for Eclipse
- Package and Deploy Desktop Application SWT/RCP
- Install Eclipse RAP Target Platform
- Install EMF for Eclipse
- Install RAP e4 Tooling for Eclipse
- Create Eclipse RAP Widget from ClientScripting-based widget
- Install GEF for Eclipse
- Eclipse RAP Tutorial for Beginners - Workbench Application (OLD)
- Eclipse RCP 3 Tutorial for Beginners - Workbench Application
- Simple Eclipse RCP 3 Application - View and Editor integration
- Eclipse RCP 4 Tutorial for Beginners - e4 Workbench Application
- Install RAP Tools for Eclipse
- Eclipse RAP Tutorial for Beginners - Basic Application
- Eclipse RAP Tutorial for Beginners - e4 Workbench Application
- Package and deploy Eclipse RAP application
Show More