Build a Multiple Module Project with Maven
1. Introduction
This document is based on:
Eclipse 4.6 (NEON)
You are viewing the advanced Maven. If you are a beginner Maven. You should look at the documentation for beginners Maven (Maven Hello world) at:
2. Model example
This is the model example in this document.
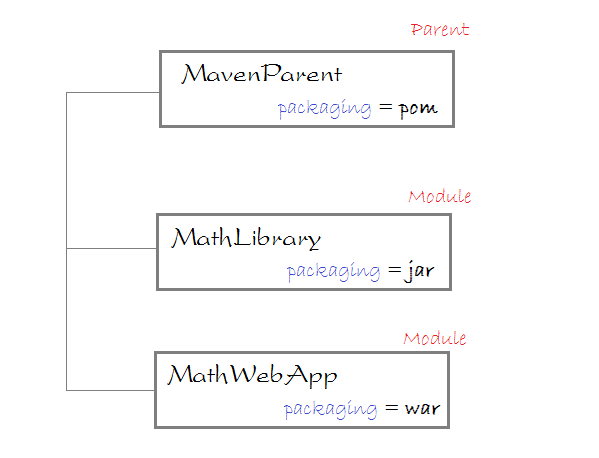
The objective of the guidelines is:
- How a module using other module in Maven
- Packing multiple Module using Maven (output: jar, war).
MathWebApp: is a WebApp project
MathLibrary: is a library Project, contains utility classes used by MathWebApp.
MavenParent: will pack two projects above, it is a parent module. MavenParent will:
MathLibrary: is a library Project, contains utility classes used by MathWebApp.
MavenParent: will pack two projects above, it is a parent module. MavenParent will:
- Packing MathLibary to a jar file
- Packing MathWebApp to a file war.
3. Create project MathLibrary
- File/New/Other...
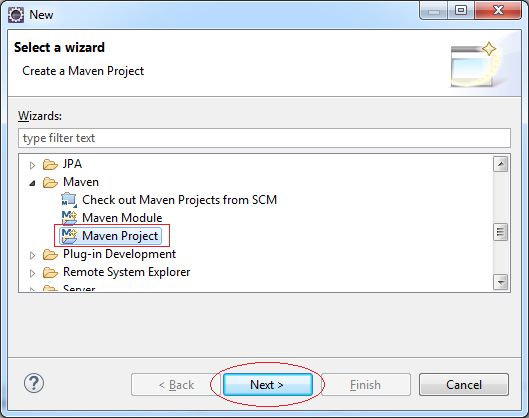
This is a simple Project, so we don't need to select a Maven archetype.
Select:
Select:
- Create a simple project (skip archetype selection)
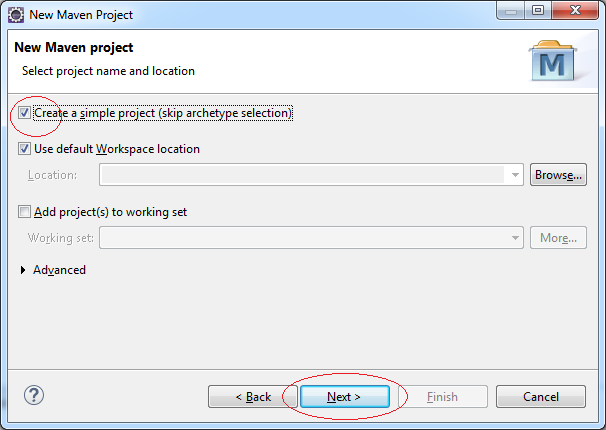
Enter:
- Group Id: org.o7planning
- Artifact Id: MathLibrary
- Packaging: jar
Skip parent module information.
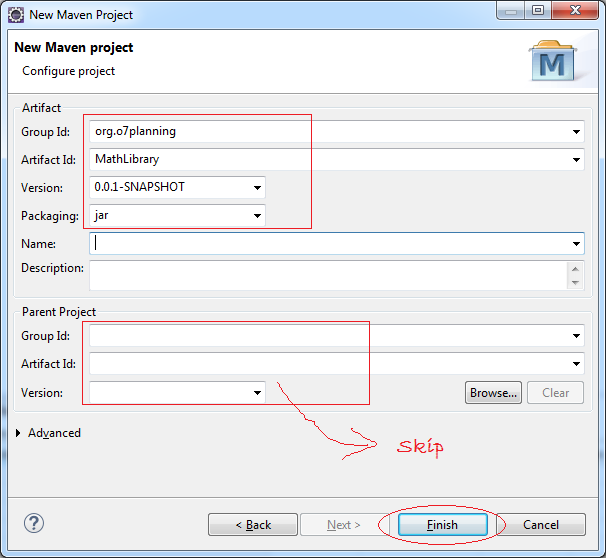
Project is created.
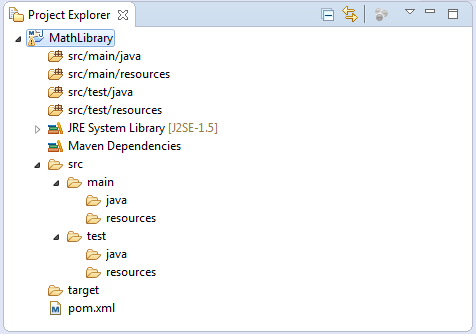
Create class MathUtils:
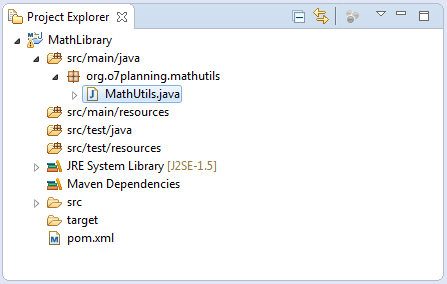
MathUtils.java
package org.o7planning.mathutils;
public class MathUtils {
public static int sum(int a, int b) {
return a + b;
}
}
4. Create project MathWebApp
- File/New/Other..
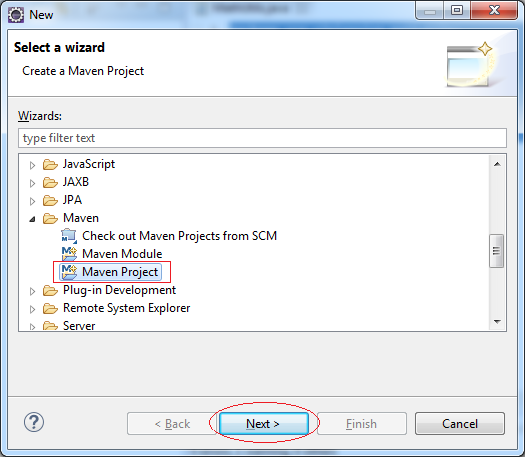
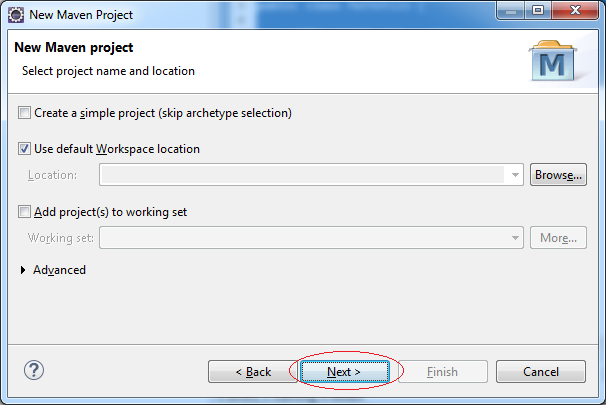
Select maven-archetype-webapp archetype. Eclipse will create a Project Maven that has the structure of a web application.
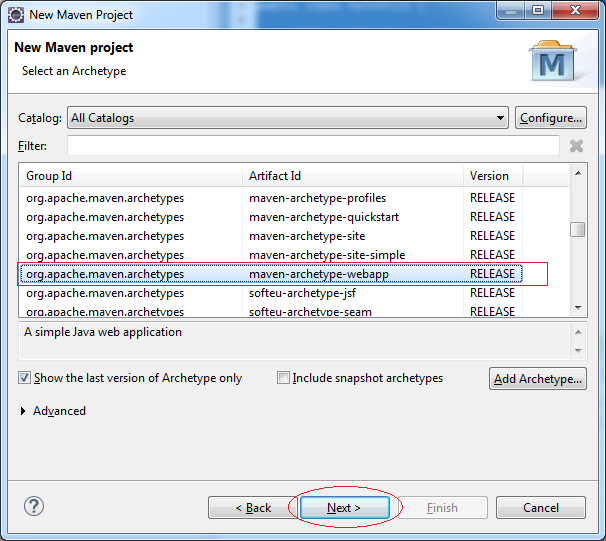
Enter:
- Group Id: org.o7planning
- Artifact Id: MathWebApp
- Version: 0.0.2-SNAPSHOT
- Package: org.o7planning.mathwebapp
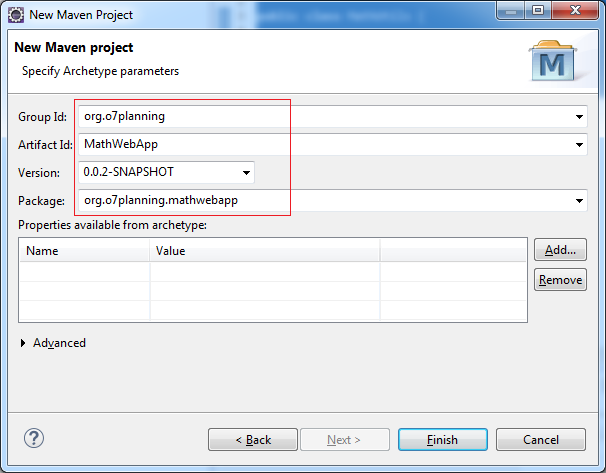
Here is a screenshot MathWebApp project has been created. You may see an error message somewhere on the Project, do not worry about it, cause you have not declared Servlet library.
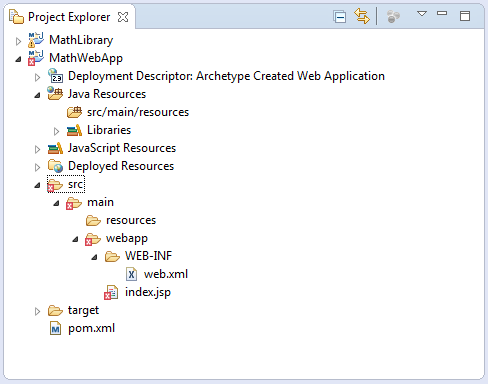
Eclipse create this project structure may be wrong. You need to check out.
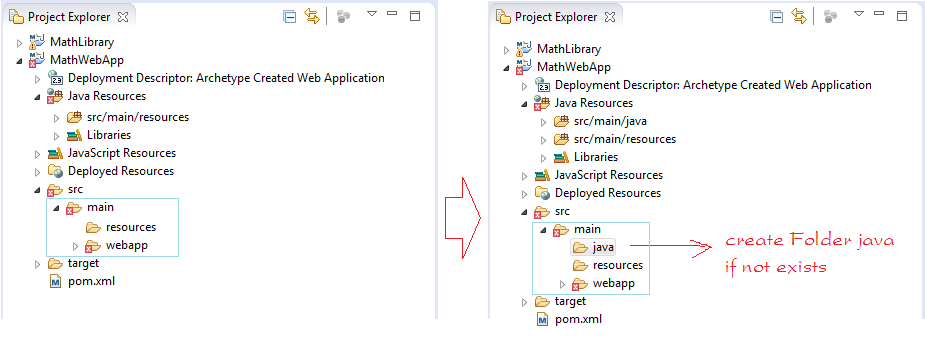
- Open file pom.xml
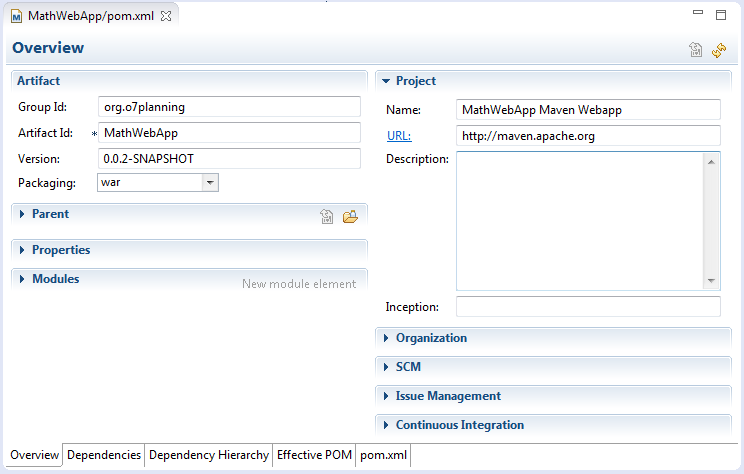
Add:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
As illustrated below:
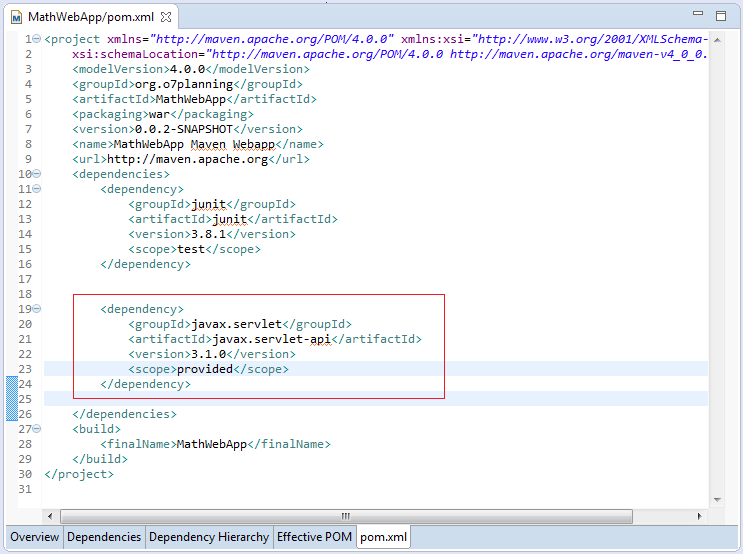
Now error has no longer exist:
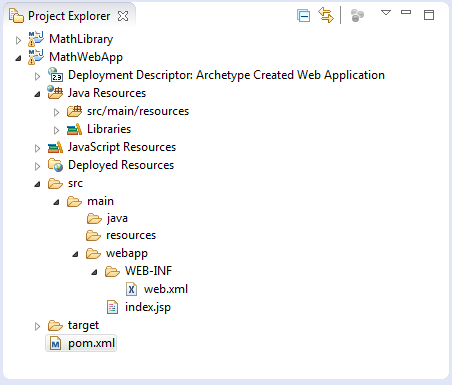
Continue configure Maven, MathWebApp use MathLibrary:
<dependency>
<groupId>org.o7planning</groupId>
<artifactId>MathLibrary</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
MathWebApp/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MathWebApp</artifactId>
<packaging>war</packaging>
<version>0.0.2-SNAPSHOT</version>
<name>MathWebApp Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.o7planning</groupId>
<artifactId>MathLibrary</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<finalName>MathWebApp</finalName>
</build>
</project>
Edit file index.jsp using MathUtils, class in project MathLibrary.
index.jsp
<html>
<body>
<h2>Hello World!</h2>
<%
int a = 100;
int b = 200;
int c = org.o7planning.mathutils.MathUtils.sum(a,b);
out.println("<h2>"+ c+"</h2>");
%>
</body>
</html>
5. Create project MavenParent
Create a Common Java Project.
- File/New/Other..
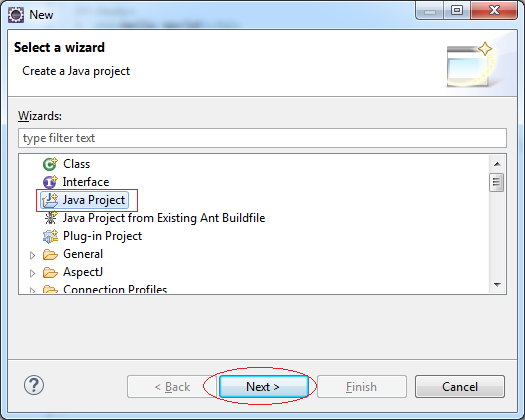
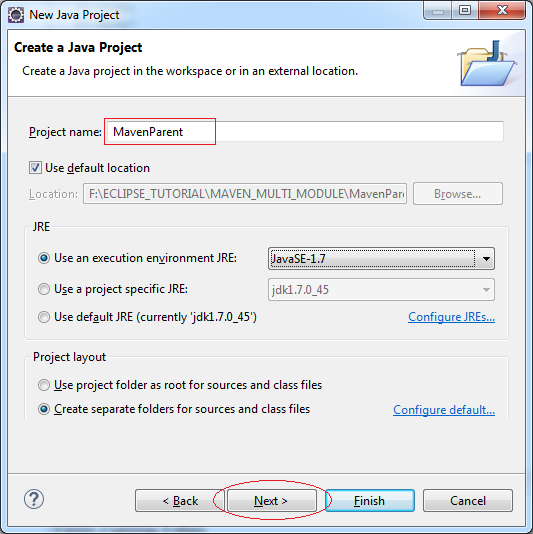
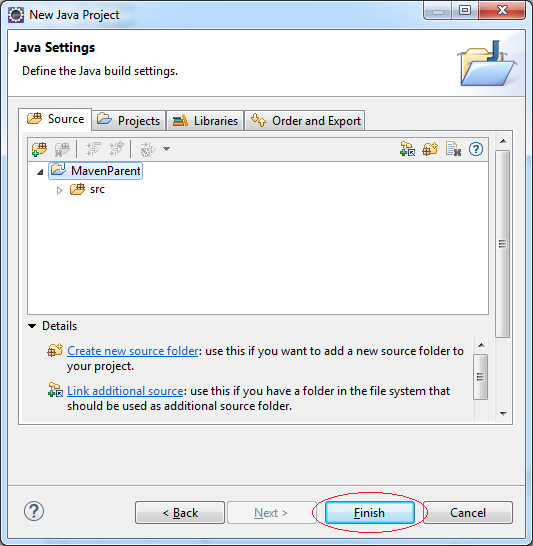
Right-click the newly created project MavenParent, and convert it to Maven Project.
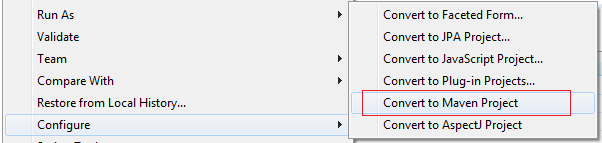
Enter:
- Group Id: org.o7planning
- Artifact Id: MavenParent
- Version: 1.0.0-SNAPSHOT
- Packaging: pom
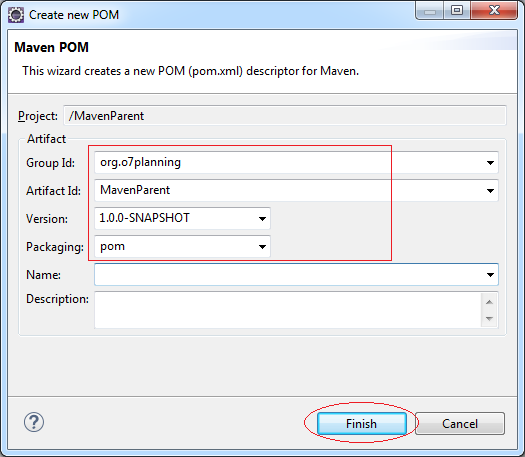
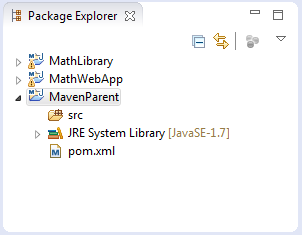
6. Configure Maven the relationship between the Projects
This image describes briefly the Maven relational configuration among Projects.
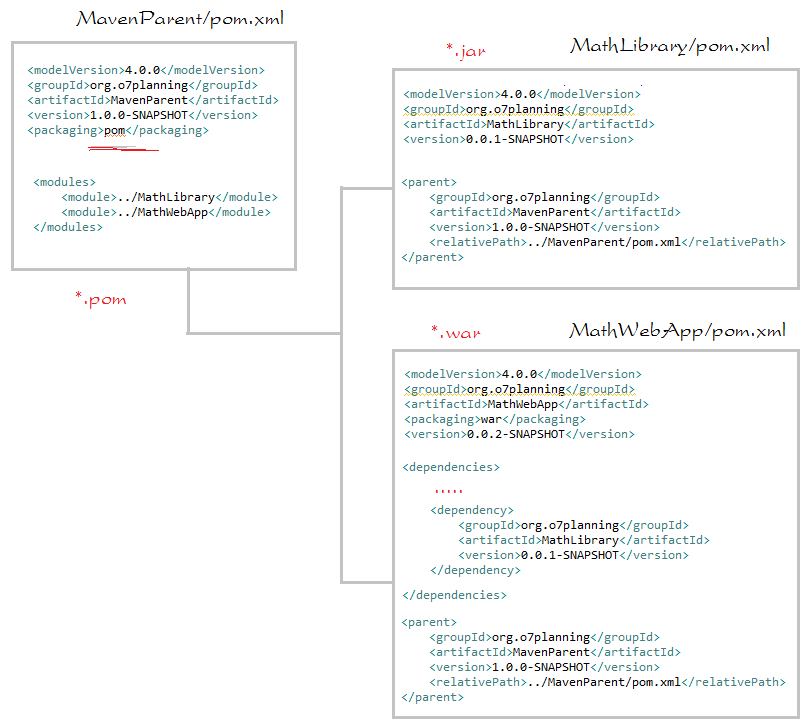
Open file pom.xml of 2 project MathLibrary & MathWebApp and add:
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0-SNAPSHOT</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
Open pom.xml of MavenParent add:
<modules>
<module>../MathLibrary</module>
<module>../MathWebApp</module>
</modules>
MathLibrary/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MathLibrary</artifactId>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0-SNAPSHOT</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
</project>
MathWebApp/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MathWebApp</artifactId>
<packaging>war</packaging>
<version>0.0.2-SNAPSHOT</version>
<name>MathWebApp Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.o7planning</groupId>
<artifactId>MathLibrary</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
<parent>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0-SNAPSHOT</version>
<relativePath>../MavenParent/pom.xml</relativePath>
</parent>
<build>
<finalName>MathWebApp</finalName>
</build>
</project>
MavenParent/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.o7planning</groupId>
<artifactId>MavenParent</artifactId>
<version>1.0.0-SNAPSHOT</version>
<packaging>pom</packaging>
<modules>
<module>../MathLibrary</module>
<module>../MathWebApp</module>
</modules>
</project>
Maven Tutorials
- Install Maven for Eclipse
- Maven Tutorial for Beginners
- Maven Manage Dependencies
- Build a Multiple Module Project with Maven
- Run Maven Java Web Application in Tomcat Maven Plugin
- Run Maven Java Web Application in Jetty Maven Plugin
- Install Tycho for Eclipse
- Create Java OSGi project with Maven and Tycho
- Create an Empty Maven Web App project in Eclipse
- OSGi and AspectJ integration
Show More