Java PrintWriter Tutorial with Examples
1. PrintWriter
PrintWriter is a subclass of Writer, which is used to print formatted data into an OutputStream or another Writer it manages.
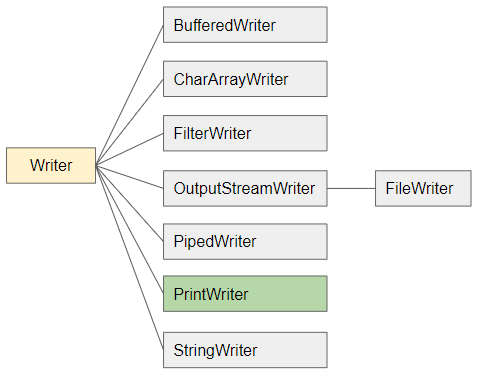
Characteristics of PrintWriter:
All methods of PrintWriter do not throw I/O exceptions, to check whether the exception has occurred, you can call checkError() method.
Optionally, PrintWriter is capable of auto-flush, meaning that the flush() method will be called immediately after calling println(..) or when printing a text that includes the '\n' character.
2. PrintWriter Constructors
The constructors to create the PrintWriter object without automatic flushing:
public PrintWriter(File file)
public PrintWriter(File file, String csn)
public PrintWriter(File file, Charset charset)
public PrintWriter(OutputStream out)
public PrintWriter(Writer out)
public PrintWriter(String fileName)
public PrintWriter(String fileName, String csn)
public PrintWriter(String fileName, Charset charset)
Constructors to create a PrintWriter object with the automatic flush option:
public PrintWriter(OutputStream out, boolean autoFlush)
public PrintWriter(OutputStream out, boolean autoFlush, Charset charset)
public PrintWriter(Writer out, boolean autoFlush)
There are quite a few constructors to initialize a PrintWriter object. Let's see what happens when creating a PrintWriter in some specific situations:
PrintWriter(Writer)
Creates a PrintWriter object to print formatted data into another Writer.
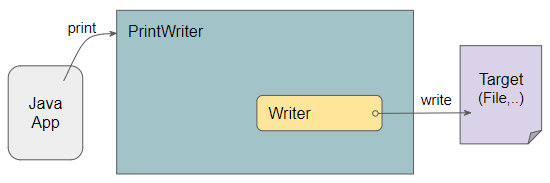
PrintWriter(OutputStream):
Create a PrintWriter object to print formatted data into an OutputStream.
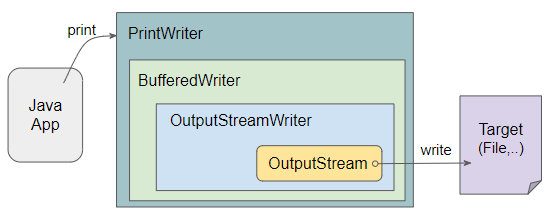
PrintWriter(File file) / PrintWriter(String fileName)
Creates a PrintWriter object to print formatted data to a file.
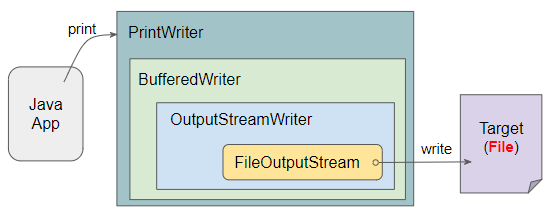
If BufferedWriter participates in structure of PrintWriter, data will be written temporarily to buffer (of BufferedWriter), Which will be pushed down to targert when buffer is full (See illustration above). You can proactively push data into target by calling PrintWriter.flush() method.
If PrintWriter is created with autoFlush feature enabled, data is pushed into target when PrinWriter.Println(..) or PrintWriter.format(..) method is called.
3. PrintWriter Methods
PrintWriter methods:
Methods of PrintWriter
public PrintWriter append(char c)
public PrintWriter append(CharSequence csq)
public PrintWriter append(CharSequence csq, int start, int end)
public PrintWriter format(String format, Object... args)
public PrintWriter format(Locale l, String format, Object... args)
public PrintWriter printf(String format, Object... args)
public PrintWriter printf(Locale l, String format, Object... args)
public boolean checkError()
protected void clearError()
protected void setError()
public void print(boolean b)
public void print(char c)
public void print(char[] s)
public void print(double d)
public void print(float f)
public void print(int i)
public void print(long l)
public void print(String s)
public void print(Object obj)
public void println()
public void println(boolean x)
public void println(char x)
public void println(char[] x)
public void println(double x)
public void println(float x)
public void println(int x)
public void println(long x)
public void println(String x)
public void println(Object x)
Other methods override the methods of the parent class and do not throw an exception:
public void write(char[] buf)
public void write(char[] buf, int off, int len)
public void write(int c)
public void write(String s)
public void write(String s, int off, int len)
public void close()
public void flush()
4. Examples
Get the stack trace from an Exception.
GetStackTraceEx.java
package org.o7planning.printwriter.ex;
import java.io.PrintWriter;
import java.io.StringWriter;
public class GetStackTraceEx {
public static void main(String[] args) {
try {
int a = 100/0; // Exception occur here
} catch(Exception e) {
String s = getStackTrace(e);
System.err.println(s);
}
}
public static String getStackTrace(Throwable t) {
StringWriter stringWriter = new StringWriter();
// Create PrintWriter via PrintWriter(Writer) constructor.
PrintWriter pw = new PrintWriter(stringWriter);
// Call method: Throwable.printStackTrace(PrintWriter)
t.printStackTrace(pw);
pw.close();
String s = stringWriter.getBuffer().toString();
return s;
}
}
Output:
java.lang.ArithmeticException: / by zero
at org.o7planning.printwriter.ex.GetStackTraceEx.main(GetStackTraceEx.java:10)
PrintWriterEx1.java
package org.o7planning.printwriter.ex;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Locale;
public class PrintWriterEx1 {
// Windows: C:/SomeFolder/pw-out-test.txt
private static final String filePath = "/Volumes/Data/test/pw-out-test.txt";
public static void main(String[] args) throws IOException {
// Create a PrintWriter to write a file.
PrintWriter printWriter = new PrintWriter(filePath);
LocalDateTime now = LocalDateTime.now();
String empName = "Tran";
LocalDate hireDate = LocalDate.of(2000, 4, 23);
int salary = 10000;
printWriter.printf("# File generated on %1$tA, %1$tB %1$tY %tH:%tM:%tS %n", now, now, now);
printWriter.println(); // New line
printWriter.printf("Employee Name: %s%n", empName);
printWriter.printf("Hire date: %1$td.%1$tm.%1$tY %n", hireDate);
printWriter.printf(Locale.US, "Salary: $%,d %n", salary);
printWriter.close();
}
}
Output:
pw-out-test.txt
# File generated on Thursday, February 2021 01:31:22
Employee Name: Tran
Hire date: 23.04.2000
Salary: $10,000
PrintStream.printf(..) and PrintWriter.printf(..) methods are the same in usage, you can refer to its usage in article below:
5. checkError()
The checkError() method returns the error state of this PrintWriter, and the flush() method is also called if the PrintWriter has not been closed.
public boolean checkError()
Note: All PrintWriter methods do not throw an IOException, but once an IOException occurs within the methods its state is considered error.
The error state of this PrintWriter is only cleared if clearError() is called, but this is a protected method. You can write a class that extends PrintWriter and override this method if you want to use it (See example below).
MyPrintWriter.java
package org.o7planning.printwriter.ex;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class MyPrintWriter extends PrintWriter {
public MyPrintWriter(File file) throws FileNotFoundException {
super(file);
}
@Override
public void clearError() {
super.clearError(); // Call protected method.
}
}
Example: Use the checkError() method to check the error state of the PrintWriter:
PrintWriter_checkError_ex1.java
package org.o7planning.printwriter.ex;
import java.io.File;
public class PrintWriter_checkError_ex1 {
// Windows: C:/SomeFolder/logFile.txt
private static final String logFilePath = "/Volumes/Data/test/logFile.txt";
public static void main(String[] args) throws Exception {
File logFile = new File(logFilePath);
MyPrintWriter mps = new MyPrintWriter(logFile);
int errorCount = 0;
while (true) {
// Write log..
mps.println("Some Log..");
Thread.sleep(1000);
// Check if IOException happened.
if (mps.checkError()) {
errorCount++;
mps.clearError();
if (errorCount > 10) {
sendAlertEmail();
break;
}
}
}
mps.close();
}
private static void sendAlertEmail() {
System.out.println("There is a problem in the Log system.");
}
}
6. print(..) *
The print(..) method is used to print a primitive value into this PrintWriter.
public void print(boolean b)
public void print(char c)
public void print(char[] s)
public void print(double d)
public void print(float f)
public void print(int i)
public void print(long l)
public void print(String s)
Example:
PrintWriter_print_primitive_ex1.java
Writer swriter = new StringWriter();
PrintWriter ps = new PrintWriter(swriter);
ps.print(true);
ps.println();
ps.print(new char[] { 'a', 'b', 'c' });
ps.println();
ps.print("Text");
System.out.println(swriter.toString());
Output:
true
abc
Text
7. print(Object)
Convert an object to a String using the String.valueOf(Object) method, and print the result to this PrintWriter.
public void print(Object obj) {
this.write(String.valueOf(obj));
}
Example:
PrintWriter_print_object_ex1.java
Writer writer = new StringWriter();
PrintWriter ps = new PrintWriter(writer);
Object obj1 = null;
ps.print(obj1);
ps.println();
Object obj2 = new Socket();
ps.print(obj2);
ps.println();
Object obj3 = Arrays.asList("One", "Two", "Three");
ps.print(obj3);
String content = writer.toString();
System.out.println(content);
Output:
null
Socket[unconnected]
[One, Two, Three]
8. println()
Print the newline character to this PrintWriter. The flush() method is also called if the PrintWriter has auto flush mode.
public void println() {
print('\n');
if(autoFlush) this.flush();
}
9. println(..) *
The println(..) method is used to print a primitive value and a newline character into this PrintWriter. The flush() method is also called if the PrintWriter has auto flush mode.
public void println(boolean x)
public void println(char x)
public void println(char[] x)
public void println(double x)
public void println(float x)
public void println(int x)
public void println(long x)
public void println(String x)
Example:
PrintWriter_println_primitive_ex1.java
OutputStream baos = new ByteArrayOutputStream();
PrintWriter ps = new PrintWriter(baos);
ps.println(true);
ps.println(new char[] { 'a', 'b', 'c' });
ps.println("Text");
ps.flush();
String content = baos.toString();
System.out.println(content);
Output:
true
abc
Text
10. println(Object)
Convert an object to a String using the String.valueOf(Object) method and then print the result and newline character into this PrintWriter. The flush() method is also called if the PrintWriter has auto flush mode. This method works like calling print(Object) and then println().
public void println(Object x) {
this.write(String.valueOf(x));
this.write("\n");
if(this.autoFlush) this.flush();
}
Example:
PrintWriter_println_object_ex1.java
Writer swriter = new StringWriter();
PrintWriter ps = new PrintWriter(swriter);
Object obj1 = null;
ps.println(obj1);
Object obj2 = new Socket();
ps.println(obj2);
Object obj3 = Arrays.asList("One", "Two", "Three");
ps.println(obj3);
String content = swriter.toString();
System.out.println(content);
Output:
null
Socket[unconnected]
[One, Two, Three]
11. printf(..) *
A convenience method to write a formatted string to this PrintWriter using the specified format string and arguments.
public PrintWriter printf(String format, Object... args)
public PrintWriter printf(Locale l, String format, Object... args)
This method works similar to the code below:
String result = String.format(format, args);
printStream.print(result);
// Or:
String result = String.format(locale, format, args);
printStream.print(result);
See also:
12. format(..) *
This method works like the printf(..) method.
public PrintWriter format(String format, Object... args)
public PrintWriter format(Locale l, String format, Object... args)
It works like the code below:
String result = String.format(format, args);
printStream.print(result);
// Or:
String result = String.format(locale, format, args);
printStream.print(result);
See also:
13. append(..) *
The append(..) methods work like the print(..) methods, the only difference is that it returns this PrintWriter. So you can continue calling next method instead of ending with a semicolon ( ; ).
public PrintWriter append(char c)
public PrintWriter append(CharSequence csq)
public PrintWriter append(CharSequence csq, int start, int end)
Example:
PrintWriter_append_ex1.java
ByteArrayOutputStream baos = new ByteArrayOutputStream();
PrintWriter ps = new PrintWriter(baos);
ps.append("This").append(" is").append(' ').append('a').append(" Text");
ps.flush();
String text = baos.toString();
System.out.println(text);// This is a Text
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More