Java BufferedInputStream Tutorial with Examples
1. BufferedInputStream
BufferedInputStream is a subclass of InputStream, which is used to simplify reading text from binary input streams, and improve program performance.
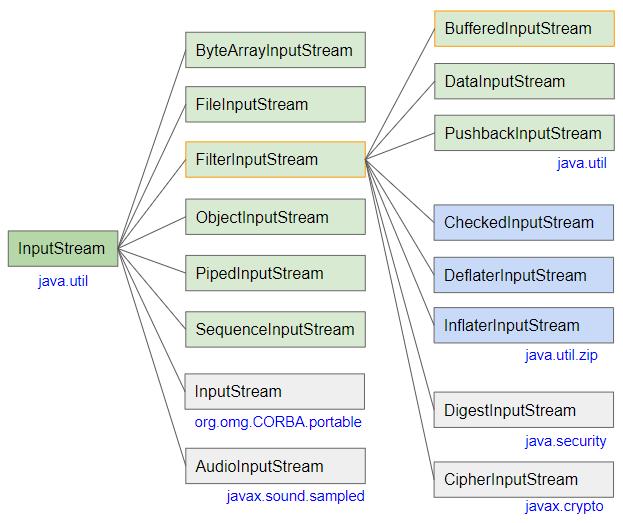
- InputStream
- FileInputStream
- ByteArrayInputStream
- PushbackInputStream
- PipedInputStream
- SequenceInputStream
- DataInputStream
- FilterInputStream
- FilterInputStream
- ObjectInputStream
- AudioInputStream
- CheckedInputStream
- DigestInputStream
- DeflaterInputStream
- CipherInputStream
BufferedInputStream operation principle looks like the following illustration:
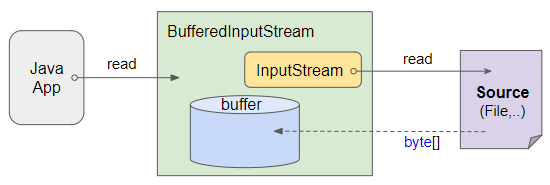
BufferedInputStream wraps inside it a InputStream object, which automatically reads data from the origin (such as file) and stores it in BufferedInputStream's buffer.
BufferedInputStream overrides methods that inherit from its parent class, such as read(), read(byte[]), ... to ensure that they will manipulate data from the buffer rather than from the origin (eg file).
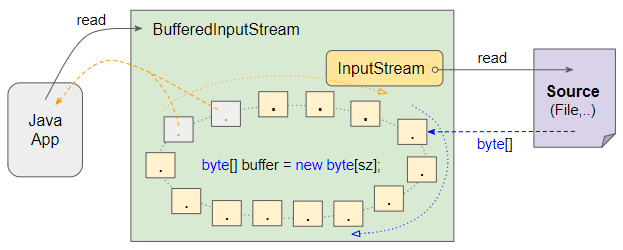
The so-called "buffer" mentioned above is actually just a byte array. InputStream object (of BufferedInputStream) will automatically read bytes from the origin to store in this array.
BufferedInputStream reads bytes from buffer array and frees the read positions. Freed positions will be used to store the newly read bytes from the origin.
BufferedInputStream constructors
BufferedInputStream(InputStream in, int size)
BufferedInputStream(InputStream in)
- BufferedInputStream(InputStream,int) constructor creates a BufferedInputStream object with specified buffer array.
- BufferedInputStream(InputStream) constructor creates a BufferedInputStream object with buffer array of default size (size = 8192).
2. mark() & reset()
An interesting aspect of BufferedInputStream is that it supports mark() and reset(), two methods inherited from InputStream. Not all InputStream subclasses support these two methods. You can basically call markSupported() method to check if the above 2 methods are supported.
See more detailed explanation of mark() and reset() methods in the article about InputStream:
3. Examples
To create a BufferedInputStream object, we need to create an InputStream object to read data from the origin (eg from File). Because InputStream is an abstract class, you need to create it from one of its subclasses.
Example: Create a BufferedInputStream with buffer array size of 16384, whichmeans this buffer is 16384 bytes (16 KB) in size.
// Create InputStream to read a file.
InputStream is = new FileInputStream("/Volumes/Data/test/test.txt");
// Create a BufferedInputStream with buffer array size of 16384 (16 KB).
BufferedReader br = new BufferedReader(is, 16384);
Create a BufferedInputStream with default buffer array size (8192), which is equivalent to 8192 bytes (8 KB).
// Create Reader to read a file.
Reader reader = new FileReader("/Volumes/Data/test/test.txt");
// Create a BufferedReader with default buffer array size of 8192 (16384 bytes = 16 KB).
BufferedReader br = new BufferedReader(reader);
Example: Using BufferedInputStream to read a file.
test.txt
This is the Latin text
BufferedInputStreamEx1.java
package org.o7planning.bufferedinputstream.ex;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class BufferedInputStreamEx1 {
// C:/test/test.txt
private static String file_path = "/Volumes/Data/test/test.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
InputStream is = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(is);
int code;
while((code = bis.read())!= -1) {
System.out.println(code +" " + (char)code);
}
bis.close();
}
}
Output:
84 T
104 h
105 i
115 s
32
105 i
115 s
32
116 t
104 h
101 e
32
76 L
97 a
116 t
105 i
110 n
32
116 t
101 e
120 x
116 t
10
Example: Using BufferedInputStream to read an URL, to help enhance program performance.
BufferedInputStreamEx2.java
package org.o7planning.bufferedinputstream.ex;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
public class BufferedInputStreamEx2 {
private static String urlString = "https://s3.o7planning.com/images/smile-32.png";
public static void main(String[] args) throws IOException {
URL url = new URL(urlString);
InputStream is = url.openConnection().getInputStream();
BufferedInputStream bis = new BufferedInputStream(is);
int code;
while ((code = bis.read()) != -1) {
System.out.print((char) code);
}
bis.close();
}
}
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More