Java BufferedReader Tutorial with Examples
1. BufferedReader
BufferedReader is a subclass of Reader, which is used to simplify reading text from character input streams, and imrove program performance.
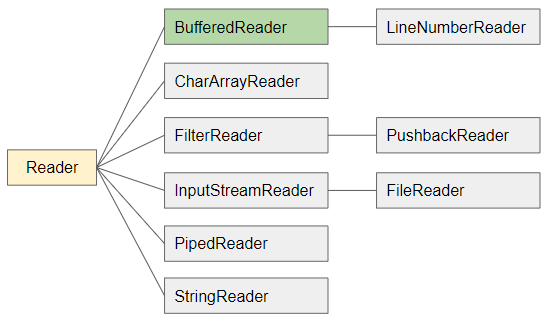
BufferedReader operation principle looks like the following illustration:
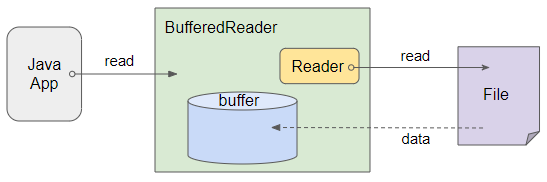
BufferedReader wraps inside it a Reader object, which automatically reads data from the origin (such as file) and stores it in BufferedReader's buffer.
BufferedReader overriedes methods that inherit from its parent class, such as read(), read(char ),... to ensure that they will work with data from buffer rather than from the origin (eg file).
BufferedReader also provides a readLine() method to read a line of text from buffer.
BufferedReader also provides a readLine() method to read a line of text from buffer.
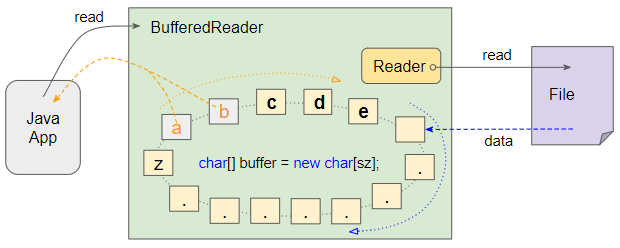
The so-called "buffer" mentioned above is actually just a character array. Reader object (of BufferedReader) automatically reads characters from the origin to store in this array.
BufferedReader reads characters from buffer array and frees those positions that are read. Freed positions will be used to store newly read characters from the origin.
BufferedReader constructors
public BufferedReader(Reader in)
public BufferedReader(Reader in, int sz)
- BufferedReader(Read,int) constructor creates a BufferedReader object with the specified buffer array.
- BufferedReader(Reader) constructor creates a BufferedReader object with the buffer array of default size (sz = 8192).
2. BufferedReader vs Scanner
In some cases, you might consider to use Scanner instead of BufferedReader. Below is a comparison between two classes:
- BufferedReader is synchronized (thread-safe) while Scanner is not.
- Scannercan parse primitive types and strings using regular expressions.
- BufferedReaderallows to specify the the size of the buffer while Scanner has a fixed buffer size (1024).
- BufferedReader has a larger default buffer size.
- Scanner hides IOException, while BufferedReader forces us to handle it.
- BufferedReader is usually faster than Scanner because it only reads the data without parsing it.
- Java Scanner
3. Examples
To create a BufferedReader object, we need to create a Reader object to read data from the origin (eg from File). Because Reader is an abstract class, you need to create it from one of its subclasses.
Example: Create a BufferedReader with buffer array size of 16384. Note: Java's char data type is 2 bytes. That means this buffer is 32786 bytes (32 KB) in size.
// Create Reader to read a file.
Reader reader = new FileReader("/Volumes/Data/test/test.txt");
// Create a BufferedReader with buffer array size of 16384 (32786 bytes = 32 KB).
BufferedReader br = new BufferedReader(reader, 16384);
Creates a BufferedReader with default buffer array size (8192), which is equivalent to 16384 bytes (16 KB).
// Create Reader to read a file.
Reader reader = new FileReader("/Volumes/Data/test/test.txt");
// Create a BufferedReader with default buffer array size of 8192 (16384 bytes = 16 KB).
BufferedReader br = new BufferedReader(reader);
Instead of creating a BufferedReader from its constructor, you can create a BufferedReader in the following way:
BufferedReader br =
Files.newBufferedReader(Paths.get("/Volumes/Data/test/test.txt"));
Example: Using readLine() method of BufferedReader to read each line of text.
test.txt
Java Tutorials:
Java Reader Tutorial
Java Writer Tutorial
BufferedReaderEx1.java
package org.o7planning.bufferedreader.ex;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
public class BufferedReaderEx1 {
public static void main(String[] args) throws IOException {
// Create Reader to read a file.
Reader reader = new FileReader("/Volumes/Data/test/test.txt");
// Create a BufferedReader with buffer array size of 16384 (32786 bytes = 32 KB).
BufferedReader br = new BufferedReader(reader, 16384);
String line = null;
while((line = br.readLine())!= null) {
System.out.println(line);
}
br.close();
}
}
Output:
Java Tutorials:
Java Reader Tutorial
Java Writer Tutorial
Example: Using BufferedReader to read a URL:
BufferedReaderEx2.java
package org.o7planning.bufferedreader.ex;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.URL;
public class BufferedReaderEx2 {
public static void main(String[] args) throws IOException {
URL oracleURL = new URL("https://www.oracle.com/");
InputStream inputStream = oracleURL.openStream();
// Create a Reader from its subclass InputStreamReader.
Reader reader = new InputStreamReader(inputStream);
BufferedReader br = new BufferedReader(reader);
String inputLine = null;
while ((inputLine = br.readLine()) != null) {
System.out.println(inputLine);
}
br.close();
}
}
4. lines()
Returns a Stream, its elements are the lines of text read from BufferedReader. This Stream is lazy, reading only happens when the user really needs the data.
public Stream<String> lines()
students.txt
# Students:
John P
Sarah M
# Sarah B
Charles B
Mary T
Sophia B
Example: Read a text file containing a list of names of the students, remove comment lines beginning with '#', and print out lines ending with "B".
BufferedReader_lines_ex1.java
package org.o7planning.bufferedreader.ex;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import java.util.stream.Stream;
public class BufferedReader_lines_ex1 {
public static void main(String[] args) throws IOException {
Reader reader = new FileReader("/Volumes/Data/test/students.txt");
BufferedReader br = new BufferedReader(reader, 16384);
Stream<String> stream = br.lines();
stream //
.filter(line -> {
return !line.trim().startsWith("#") && line.endsWith("B");
})
.forEach(System.out::println);
br.close();
}
}
Output:
Charles B
Sophia B
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More