Java ByteArrayOutputStream Tutorial with Examples
1. ByteArrayOutputStream
ByteArrayOutputStream is a subclass of OutputStream. True to its name, ByteArrayOutputStream is used to write bytes to a byte array that manages in the way of an OutputStream.
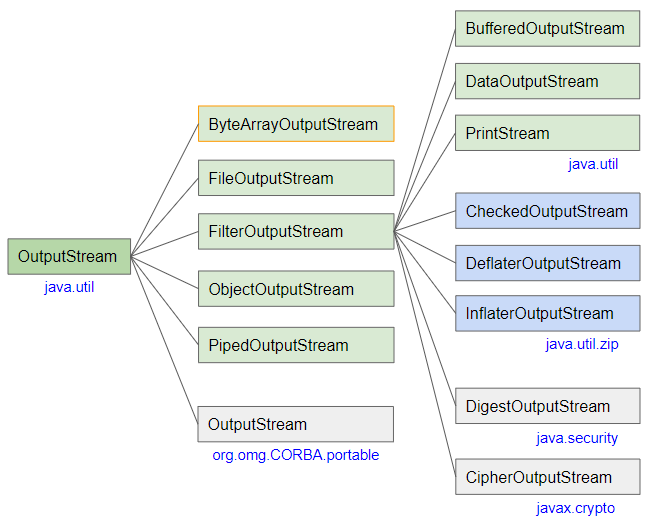
- OutputStream
- BufferedOutputStream
- ObjectOutputStream
- PrintStream
- FileOutputStream
- FilterOutputStream
- PipedOutputStream
- DataOutputStream
- InflaterOutputStream
- DigestOutputStream
- DeflaterOutputStream
- CipherOutputStream
- CheckedOutputStream
bytes written to ByteArrayOutputStream will be assigned to elements of the byte array that ByteArrayOutputStream manages.
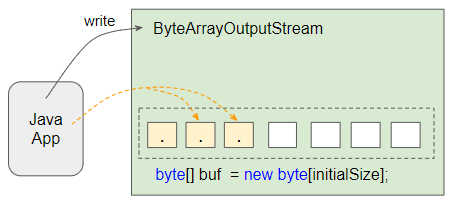
When the number of bytes written to ByteArrayOutputStream is greater than the length of array, ByteArrayOutputStream will create a new array with greater length and copy the bytes from the old array.
2. Constructors
Constructors
public ByteArrayOutputStream()
public ByteArrayOutputStream(int initialSize)
- ByteArrayOutputStream(int) constructor creates a ByteArrayOutputStream object with a byte array that has a specified initial size.
- ByteArrayOutputStream() constructor creates a ByteArrayOutputStream object with a byte array of that has default size (32).
3. Methods
All methods of ByteArrayOutputStream are inherited from its parent class - OutputStream.
void close()
void reset()
int size()
byte[] toByteArray()
String toString()
String toString(String charsetName)
String toString(Charset charset)
void write(byte[] b, int off, int len)
void write(int b)
void writeBytes(byte[] b)
void writeTo(OutputStream out)
See the OutputStream article for more examples of these methods.
4. Examples
ByteArrayOutputStreamEx1.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx1 {
public static void main(String[] args) throws IOException {
ByteArrayOutputStream baos = new ByteArrayOutputStream(1024);
byte[] bytes1 = new byte[] {'H', 'e'};
baos.write(bytes1);
baos.write(108); // 'l'
baos.write('l'); // code: 108
baos.write('o'); // code: 111
byte[] buffer = baos.toByteArray();
for(byte b: buffer) {
System.out.println(b + " --> " + (char)b);
}
}
}
Output:
72 --> H
101 --> e
108 --> l
108 --> l
111 --> o
For example, add 2 byte arrays to create a new array.
ByteArrayOutputStreamEx3.java
package org.o7planning.bytearrayoutputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class ByteArrayOutputStreamEx3 {
public static void main(String[] args) throws IOException {
byte[] arr1 = "Hello ".getBytes();
byte[] arr2 = new byte[] {'W', 'o', 'r', 'l', 'd', '!'};
byte[] result = add(arr1, arr2);
for(byte b: result) {
System.out.println(b + " " + (char)b);
}
}
public static byte[] add(byte[] arr1, byte[] arr2) {
if (arr1 == null) {
return arr2;
}
if (arr2 == null) {
return arr1;
}
ByteArrayOutputStream baos = new ByteArrayOutputStream();
try {
baos.write(arr1);
baos.write(arr2);
} catch (Exception e) {
// Never happened!
}
return baos.toByteArray();
}
}
Output:
72 H
101 e
108 l
108 l
111 o
32
87 W
111 o
114 r
108 l
100 d
33 !
Basically, most of ByteArrayOutputStream methods inherit from OutputStream, so you can find more examples of how to use these methods in the article below:
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More