Java Reader Tutorial with Examples
1. Reader
Reader is a class in java.io package, which is a base class representing a stream of characters obtained when reading a certain data source, such as a text file.

public abstract class Reader implements Readable, Closeable
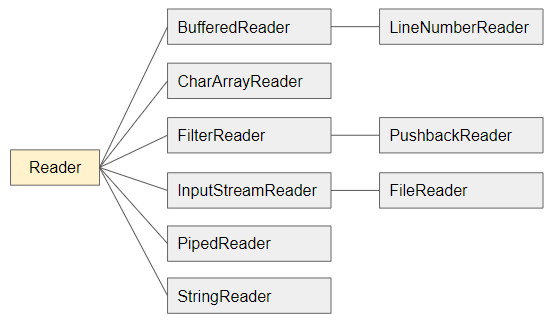
Basically, you cannot use Reader class directly because it is an abstract class. But in specific case you can use one of its subclasses.
Let's see a scenario of reading a text file with UTF-8 encoding:
utf-8-file.txt
JP日本-八洲
UTF-8 uses 1, 2, 3 or 4 bytes to store a character. The image below shows the bytes in the aforementioned file.
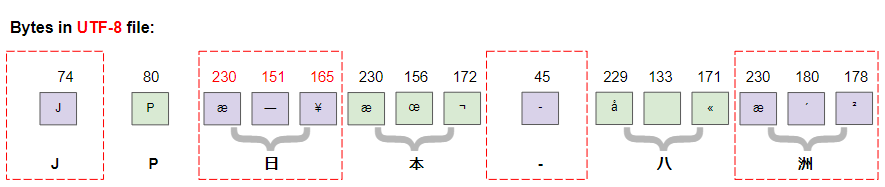
FileReader is a subclass of Reader commonly used to read text files and we will get a stream of characters. Somehow UTF-8 characters will be converted to Java characters.
Note: char type in Java is 2 bytes in size. Thus, the characters on FileReader are 2-byte characters.
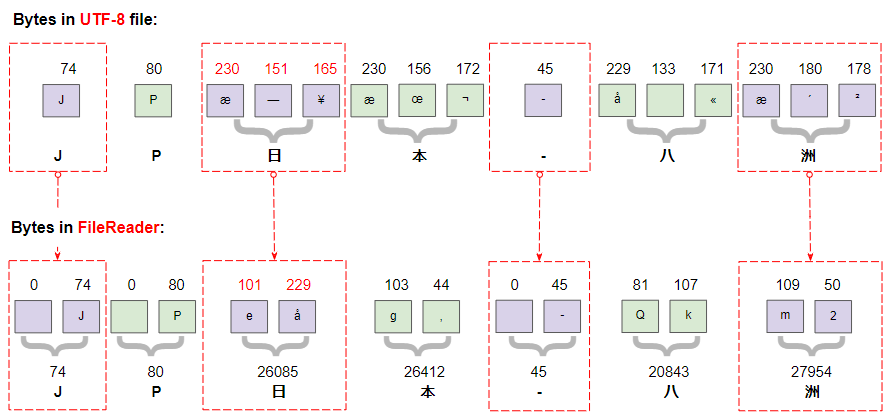
See also my explanation of how Java converts UTF-8 characters to Java characters:
Reader Methods
public static Reader nullReader()
public int read(java.nio.CharBuffer target) throws IOException
public int read() throws IOException
public int read(char cbuf[]) throws IOException
public int read(char cbuf[], int off, int len) throws IOException
public long skip(long n) throws IOException
public boolean ready() throws IOException
public boolean markSupported()
public void mark(int readAheadLimit) throws IOException
public void reset() throws IOException
public void close() throws IOException
public long transferTo(Writer out) throws IOException
2. read()
public int read() throws IOException
read() method is used to read a character, which returns the number of character that has just been read (An integer between 0 and 65535), or -1 if it has reached the end of the stream.
This method will block until the available character or an IO error occurs, or has reached the end of the stream.
Example:
Reader_read_ex1.java
package org.o7planning.reader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
public class Reader_read_ex1 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("JP日本-八洲");
int charCode;
while((charCode = reader.read()) != -1) {
System.out.println((char)charCode + " " + charCode);
}
reader.close();
}
}
Output:
J 74
P 80
日 26085
本 26412
- 45
八 20843
洲 27954
3. read(char[])
public int read(char[] cbuf) throws IOException
read(char[]) method reads characters and assigns elements of array and returns the number of characters just read. This method returns -1 if it has reached the end of the stream.
This method will block until the characters are available or an IO error occurs, or has reached the end of the stream.
Basically, using read(char[]) method will have better performance than read() method, since it reduces the number of times it needs to read from the stream.
Reader_read_ex1.java
package org.o7planning.reader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
public class Reader_read_ex2 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("123456789-987654321-12345");
// Create a temporary char array.
char[] temp = new char[10];
int charCount = -1;
while ((charCount = reader.read(temp)) != -1) {
String s = new String(temp, 0, charCount);
System.out.println(s);
}
reader.close();
}
}
Output:
123456789-
987654321-
12345
4. read(char[], int, int)
public int read(char[] cbuf, int offset, int len) throws IOException
read(char[],int,int) method reads characters and assigns elements of array from offset index to offset+len index, and returns the number of characters just read. This method returns -1 if it has reached the end of the stream.
This method will block until the characters are available or an IO error occurs, or reached the end of the stream.
5. read(java.nio.CharBuffer)
public int read(java.nio.CharBuffer target) throws IOException
read(CharBuffer) method is used to read characters into a CharBufffer object, and return the number of characters read from the stream or -1 if it has reached the end of the stream.
This method will block until the characters are available or an IO error occurs, or has reached the end of the stream.
Reader_read_ex3.java
package org.o7planning.reader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.nio.CharBuffer;
public class Reader_read_ex3 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("123456789-987654321-12345");
CharBuffer cb = CharBuffer.allocate(10);
// Read for the first time
reader.read(cb);
System.out.println(cb.flip().toString());
// Read for the second time
reader.read(cb);
System.out.println(cb.flip().toString());
// Read for the third time.
reader.read(cb);
System.out.println(cb.flip().toString());
reader.close();
}
}
Output:
123456789-
987654321-
12345
- Java CharBuffer
6. close()
public void close() throws IOException
Closes the stream and releases any system resources associated with it. Once the stream has been closed, further read(), ready(), mark(), reset(), or skip() invocations will throw an IOException. Closing a previously closed stream has no effect.
public interface Closeable extends AutoCloseable
Reader class implements a Closeable interface. If you write code according to the rules of AutoCloseable, system will automatically close the stream for you without having to call close() method directly.
Reader_close_ex1.java
package org.o7planning.reader.ex;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
public class Reader_close_ex1 {
// Or Windows path: C:/Somefolder/utf8-file-without-bom.txt
private static final String file_path = "/Volumes/Data/test/utf8-file-without-bom.txt";
public static void main(String[] args) throws IOException {
// (Reader class implements Closeable)
// (Closeable interface extends AutoCloseable)
// try block will automatically close stream for you.
try (Reader fileReader= new FileReader(file_path)) {
int code;
while((code = fileReader.read()) != -1) {
System.out.println((char)code);
}
} // end try
}
}
- Java Closeable
7. skip(long)
public long skip(long n) throws IOException
skip(long) method skips "n" characters.
This method will block until the characters are available or an IO error occurs, or has reached the end of the stream.
Reader_skip_ex1.java
package org.o7planning.reader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
public class Reader_skip_ex1 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("123456789-987654321-ABCDE");
int firstCharCode = reader.read();
int secondCharCode = reader.read();
System.out.println("First character: " + (char) firstCharCode);
System.out.println("Second character: " + (char) secondCharCode);
reader.skip(18); // Skips 18 characters.
int code;
while ((code = reader.read()) != -1) {
System.out.println((char) code);
}
reader.close();
}
}
Output:
First character: 1
Second character: 2
A
B
C
D
E
8. transferTo(Writer)
// Java 10+
public long transferTo(Writer out) throws IOException
transferTo(Writer) method is used to read all characters from the current Reader and write them to the specified Writer object, and return the number of characters transferred to Writer. Once completed, the current Reader object will be at the end of the stream. This method will not close the current Reader object or the Writer object.
Reader_transferTo_ex1.java
package org.o7planning.reader.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
import java.io.Writer;
public class Reader_transferTo_ex1 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("123456789-987654321-ABCDE");
// Or Windows path: C:/Somepath/out-file.txt
File file = new File("/Volumes/Data/test/out-file.txt");
// Create parent folder.
file.getParentFile().mkdirs();
Writer writer = new FileWriter(file);
reader.skip(10); // Skips 10 characters.
reader.transferTo(writer);
reader.close();
writer.close();
}
}
Output:
out-file.txt
987654321-ABCDE
9. markSupported()
public boolean markSupported()
The markSupported() method is used to check whether the current Reader object supports mark(int) operation. (See also mark(int) method)
Reader | markSupported()? |
StringReader | true |
CharArrayReader | true |
BufferedReader | true |
LineNumberReader | true |
FilterReader | true or false |
InputStreamReader | false |
FileReader | false |
PushbackReader | false |
10. mark(int)
public void mark(int readAheadLimit) throws IOException
mark(int) method allows you to mark the current position on the stream. You can continue to read the next character, and call reset() method to go back to the previously marked position, in which readAheadLimit is the maximum number of characters that can be read after marking without losing the marked position.
Note: Not all Reader supports mark(int) operation. To be sure you need to call markSupported() method to check if the current Reader object supports that operation.
Reader_mark_ex1.java
package org.o7planning.reader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StringReader;
public class Reader_mark_ex1 {
public static void main(String[] args) throws IOException {
// StringReader is a subclass of Reader.
Reader reader = new StringReader("123456789-987654321-ABCDE");
reader.skip(10); // Skips 10 characters.
System.out.println("StringReader markSupported? " + reader.markSupported()); // true
reader.mark(9);
int code1 = reader.read();
int code2 = reader.read();
System.out.println((char) code1); // '9'
System.out.println((char) code2); // '8'
reader.skip(5);
System.out.println("Reset");
reader.reset(); // Return to the marked position.
int code;
while((code = reader.read())!= -1) {
System.out.println((char)code);
}
reader.close();
}
}
Output:
StringReader markSupported? true
9
8
Reset
9
8
7
6
5
4
3
2
1
-
A
B
C
D
E
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More