Java StringWriter Tutorial with Examples
1. StringWriter
StringWriter is a subclass of Writer that manages a StringBuffer object. The characters written to the StringWriter will be appended to the StringBuffer object it manages.
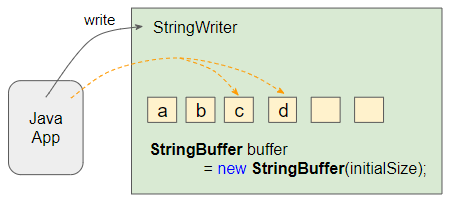
The StringWriter class does not use I/O or Network operations so you can use it without closing it.
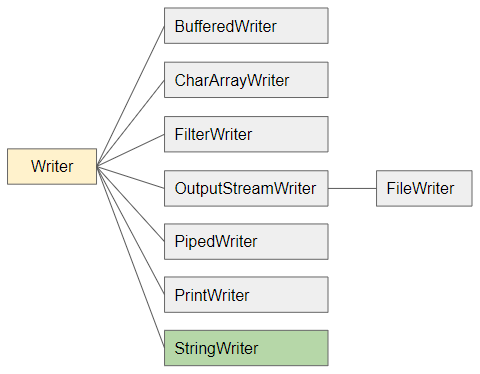
StringWriter constructor
StringWriter()
StringWriter(int initialSize)
Besides those methods inherited from its parent class, StringWriter also has a few more notable methods of its own, including:
String toString() | Returns the String of the buffer. |
StringBuffer getBuffer() | Returns the StringBuffer object that StringWriter is currently managing. |
StringWriter append(
char c) | Append a character to the buffer, the same as calling StringWriter.write(char) method, but this method returns the current StringWriter object, this allows calling another method of StringWriter instead of terminating with a semicolon (;) |
StringWriter append(
CharSequence csq) | Similar to the StringWriter.write(CharSequence) method, but returns the current StringWriter object. |
StringWriter append(
CharSequence csq, int start, int end) | Similar to the StringWriter.write(CharSequence,int,int) method, but returns the current StringWriter object. |
2. Examples
StringWriterEx1.java
package org.o7planning.stringwriter.ex;
import java.io.StringWriter;
public class StringWriterEx1 {
public static void main(String[] args) {
StringWriter sw = new StringWriter();
sw.write("Java Tutorial");
sw.append("\n").append("C# Tutorial").append("\n").append("Python Tutorial");
String s = sw.toString();
System.out.println(s);
}
}
Output:
Java Tutorial
C# Tutorial
Python Tutorial
Usually when an exception occurs, you often see "Stack Trace" information printed on the Console screen, how to get this content as a String?
StringWriterEx3.java
package org.o7planning.stringwriter.ex;
import java.io.PrintWriter;
import java.io.StringWriter;
public class StringWriterEx3 {
public static void main(String[] args) {
try {
int a = 100/0; // Exception occur here
} catch(Exception e) {
String s = getStackTrace(e);
System.err.println(s);
}
}
public static String getStackTrace(Throwable t) {
StringWriter writer = new StringWriter();
// Create PrintWriter via PrintWriter(Writer) constructor.
PrintWriter pw = new PrintWriter(writer);
// Call method: Throwable.printStackTrace(PrintWriter)
t.printStackTrace(pw);
pw.close();
String s = writer.getBuffer().toString();
return s;
}
}
Output:
java.lang.ArithmeticException: / by zero
at org.o7planning.stringwriter.ex.StringWriterEx5.main(StringWriterEx5.java:10)
GetStackTraceEx.java
package org.o7planning.printwriter.ex;
import java.io.PrintWriter;
import java.io.StringWriter;
public class GetStackTraceEx {
public static void main(String[] args) {
try {
int a = 100/0; // Exception occur here
} catch(Exception e) {
String s = getStackTrace(e);
System.err.println(s);
}
}
public static String getStackTrace(Throwable t) {
StringWriter stringWriter = new StringWriter();
// Create PrintWriter via PrintWriter(Writer) constructor.
PrintWriter pw = new PrintWriter(stringWriter);
// Call method: Throwable.printStackTrace(PrintWriter)
t.printStackTrace(pw);
pw.close();
String s = stringWriter.getBuffer().toString();
return s;
}
}
Output:
java.lang.ArithmeticException: / by zero
at org.o7planning.printwriter.ex.GetStackTraceEx.main(GetStackTraceEx.java:10)
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More