Java OutputStream Tutorial with Examples
1. OutputStream
OutputStream is a class in java.io package, which is a base class representing a stream of bytes to write bytes to a target, such as file.
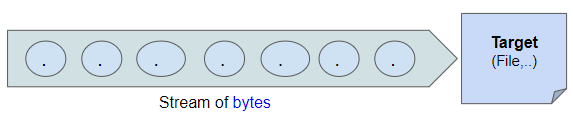
public abstract class OutputStream implements Closeable, Flushable
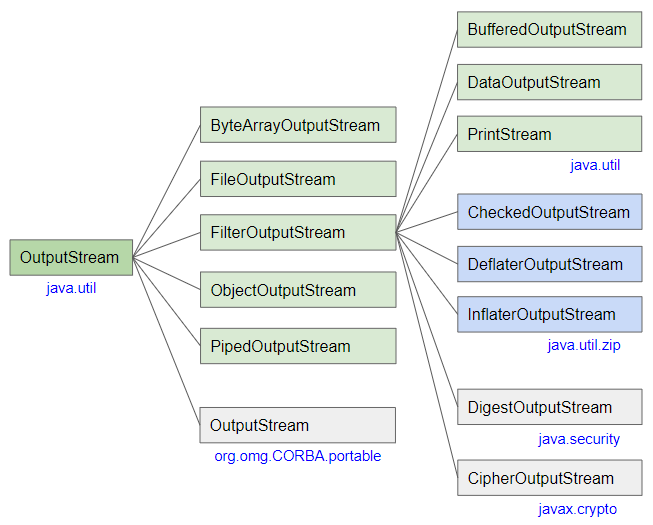
- ByteArrayOutputStream
- FileOutputStream
- FilterOutputStream
- ObjectOutputStream
- PipedOutputStream
- BufferedOutputStream
- DataOutputStream
- PrintStream
- CheckedOutputStream
- CipherOutputStream
- DeflaterOutputStream
- DigestOutputStream
- InflaterOutputStream
Basically, you cannot use OutputStream class directly because it is an abstract class. But in a particular case you can use one of its subclasses.
OutputStream methods
public static OutputStream nullOutputStream()
public abstract void write(int b) throws IOException
public void write(byte b[]) throws IOException
public void write(byte b[], int off, int len) throws IOException
public void flush() throws IOException
public void close() throws IOException
2. write(int)
public abstract void write(int b) throws IOException
Writes one byte to OutputStream. b parameter is the unsigned code of byte, which is an integer between 0 and 255. If value of b is out of the above range, it will be ignored.
OutputStream_write_ex1.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileOutputStream
OutputStream os = new FileOutputStream(file);
// 'J'
os.write((int)'J'); // 74
// 'P'
os.write('P'); // 80 (Automatically converted to int)
// '日' = 3 bytes UTF-8: [230, 151, 165]
os.write(230);
os.write(151);
os.write(165);
// '本' = 3 bytes UTF-8: [230, 156, 172]
os.write(230);
os.write(156);
os.write(172);
// '-'
os.write('-'); // 45 (Automatically converted to int)
// '八' = 3 bytes UTF-8: [229, 133, 171]
os.write(229);
os.write(133);
os.write(171);
// '洲' = 3 bytes UTF-8: [230, 180, 178]
os.write(230);
os.write(180);
os.write(178);
// Close
os.close();
}
}
Output:
out-file.txt
JP日本-八洲
UTF-8 files use 1, 2, 3 or 4 bytes to store a character. The image below shows the bytes on the newly created file.
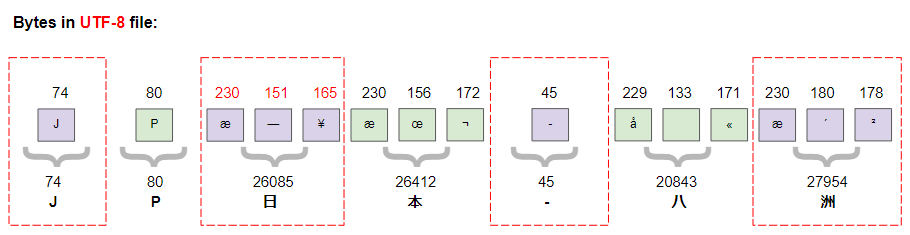
3. write(byte[])
public void write(byte[] b) throws IOException
Writes a byte array to OutputStream.
OutputStream_write_ex2.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex2 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileOutputStream
OutputStream writer = new FileOutputStream(file);
byte[] bytes = new byte[] { 'J', 'P', //
(byte)230, (byte)151, (byte)165, // '日' = 3 bytes UTF-8: [230, 151, 165]
(byte)230, (byte)156, (byte)172, // '本' = 3 bytes UTF-8: [230, 156, 172]
'-', //
(byte)229, (byte)133, (byte)171, // '八' = 3 bytes UTF-8: [229, 133, 171]
(byte)230, (byte)180, (byte)178, // '洲' = 3 bytes UTF-8: [230, 180, 178]
};
writer.write(bytes);
// Close
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
4. write(byte[], int, int)
public void write(byte b[], int offset, int len) throws IOException
Write part of a byte array to OutputStream. Write bytes from offset index to offset+len index.
OutputStream_write_ex3.java
package org.o7planning.outputstream.ex;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_write_ex3 {
public static void main(String[] args) throws IOException {
// ByteArrayOutputStream is a subclass of OutputStream.
OutputStream outputStream = new ByteArrayOutputStream();
byte[] bytes = new byte[] {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'};
outputStream.write(bytes, 2, 5); // '2', ...'6'
String s = outputStream.toString();
System.out.println(s);
outputStream.close();
}
}
Output:
23456
5. close()
public void close() throws IOException
The close() method is used to close this stream, the flush() method will be called first. Once the stream has been closed, further write() or flush() invocations will cause an IOException to be thrown. Closing a previously closed stream has no effect.
public interface Closeable extends AutoCloseable
Writer class implements Closeable interface. If you write code according to the rules of AutoCloseable, system will automatically close the stream for you without having to call close() method directly.
OutputStream_close_ex1.java
package org.o7planning.outputstream.ex;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class OutputStream_close_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
try (OutputStream writer = new FileOutputStream(file)) {
writer.write((int) '1');
writer.write('2');
writer.write((int) '3');
}
}
}
- Java Closeable
6. flush()
public void flush() throws IOException
The data that you write to OutputStream is sometimes temporarily stored on the buffer. flush() method is used to flush the data into the target.
You can read BufferedOutputStream article below to understand more about flush() method.
If the intended destination of this OutputStream is an abstraction provided by the underlying operating system, for example a file, then flushing the stream guarantees only that bytes previously written to the stream are passed to the operating system for writing; it does not guarantee that they are actually written to a physical device such as a disk drive.
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More