Java SequenceInputStream Tutorial with Examples
1. SequenceInputStream
SequenceInputStream allows you to concatenate two or multiple InputStreams together. It reads from the first byte to the last byte of the first InputStream, then does the same with the next InputStream until the last InputStream.
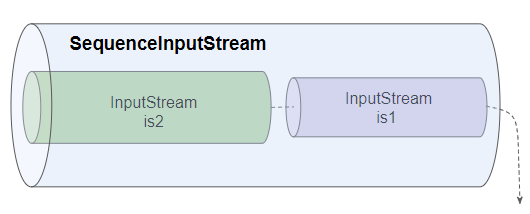
SequenceInputStream is a subclass of InputStream:
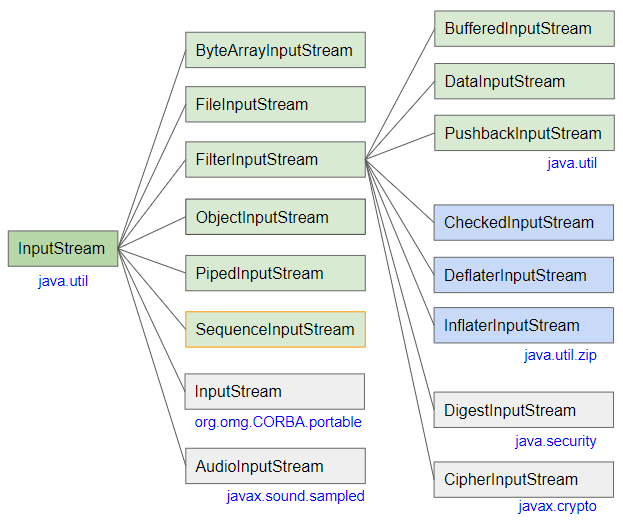
- InputStream
- ObjectInputStream
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- PipedInputStream
- AudioInputStream
- BufferedInputStream
- DataInputStream
- PushbackInputStream
- CheckedInputStream
- InflaterInputStream
- DigestInputStream
- DeflaterInputStream
- CipherInputStream
SequenceInputStream Methods
final void nextStream() throws IOException
// Methods inherited from InputStream
public int available() throws IOException
public int read() throws IOException
public int read(byte b[], int off, int len) throws IOException
public int read(byte[] b) throws IOException
public byte[] readAllBytes() throws IOException
public byte[] readNBytes(int len) throws IOException
public int readNBytes(byte[] b, int off, int len) throws IOException
public long skip(long n) throws IOException
public boolean markSupported()
public synchronized void mark(int readlimit)
public synchronized void reset() throws IOException
public void close() throws IOException
public long transferTo(OutputStream out) throws IOException
Most of the methods of SequenceInputStream are inherited from InputStream, so you can learn how to use these methods in the article about InputStream.
SequenceInputStream Constructors
public SequenceInputStream(InputStream s1, InputStream s2)
public SequenceInputStream(Enumeration<? extends InputStream> e)
2. Examples
Suppose we have two UTF-8 encoded text files, each file contains a list of flower names.
flowers-1.txt
# Flower names (1)
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
flowers-2.txt
# Flower names (2)
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
We will read the above 2 files using SequenceInputStream, InputStreamReader and BufferedReader.
SequenceInputStreamEx1.java
package org.o7planning.sequenceinputstream.ex;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.SequenceInputStream;
public class SequenceInputStreamEx1 {
// Windows: C:/Data/test/flowers-1.txt
private static String file_path1 = "/Volumes/Data/test/flowers-1.txt";
private static String file_path2 = "/Volumes/Data/test/flowers-2.txt";
public static void main(String[] args) throws IOException {
InputStream is1 = new FileInputStream(file_path1);
InputStream is2 = new FileInputStream(file_path2);
// Create SequenceInputStream:
InputStream is = new SequenceInputStream(is1, is2);
InputStreamReader isr = new InputStreamReader(is, "UTF-8");
BufferedReader br = new BufferedReader(isr);
String line;
while((line = br.readLine())!= null) {
System.out.println(line);
}
br.close();
}
}
Output:
# Flower names (1)
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
# Flower names (2)
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
Improve the example above, just print out the line that is not empty and not a comment line (Start with "#").
SequenceInputStreamEx2.java
package org.o7planning.sequenceinputstream.ex;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.SequenceInputStream;
public class SequenceInputStreamEx2 {
// Windows: C:/Data/test/flowers-1.txt
private static String file_path1 = "/Volumes/Data/test/flowers-1.txt";
private static String file_path2 = "/Volumes/Data/test/flowers-2.txt";
public static void main(String[] args) throws IOException {
InputStream is1 = new FileInputStream(file_path1);
InputStream is2 = new FileInputStream(file_path2);
// Create SequenceInputStream:
InputStream is = new SequenceInputStream(is1, is2);
InputStreamReader isr = new InputStreamReader(is, "UTF-8");
BufferedReader br = new BufferedReader(isr);
br.lines() // Stream
.filter(line -> !line.isBlank()) // Not blank
.filter(line -> !line.startsWith("#")) // Not start with "#"
.forEach(System.out::println);
br.close();
}
}
Output:
Tulip
Daffodil
Poppy
Sunflower
Bluebell
Rose
Snowdrop
Cherry blossom
Orchid
Iris
Peony
Chrysanthemum
Geranium
Lily
Lotus
Water lily
Dandelion
Hyacinth
Daisy
Crocus
Java IO Tutorials
- Java InputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
Show More