Java FilterWriter Tutorial with Examples
1. FilterWriter
FilterWriter is an abstract subclass of the Writer class. It is the base class for creating subclasses used to selectively write the required characters.
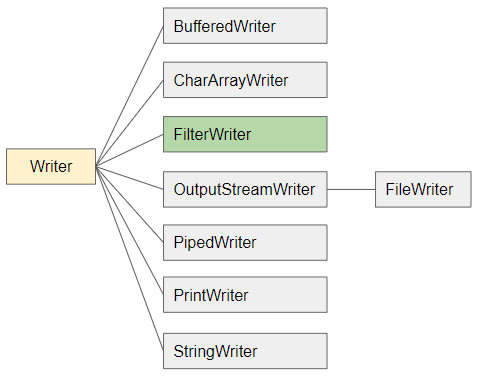
FilterWriter does not directly write characters to the target (e.g. file), instead it manages another Writer that is responsible for writing the data to the target. FilterWriter filters or processes the characters written to it, then writes the results to the Writer it manages.
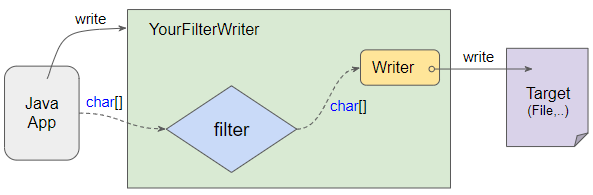
Take a look at the source code of the FilterWriter class: All the methods that it inherits from the superclass are overridden to act as a proxy of the Writer object it manages:
FilterWriter
public abstract class FilterWriter extends Writer {
protected Writer out;
protected FilterWriter(Writer out) {
super(out);
this.out = out;
}
public void write(int c) throws IOException {
out.write(c);
}
public void write(char cbuf[], int off, int len) throws IOException {
out.write(cbuf, off, len);
}
public void write(String str, int off, int len) throws IOException {
out.write(str, off, len);
}
public void flush() throws IOException {
out.flush();
}
public void close() throws IOException {
out.close();
}
}
FilterWriter constructors
protected FilterWriter(Writer out)
2. Examples
ROT13 is a simple letter substitution cipher, replacing each letter with another letter that is 13 position after it on the alphabet. ROT13 is a simple case of the Caesar cipher.
Below is a table of letters and their corresponding substitutions as the result of using the ROT13 algorithm.
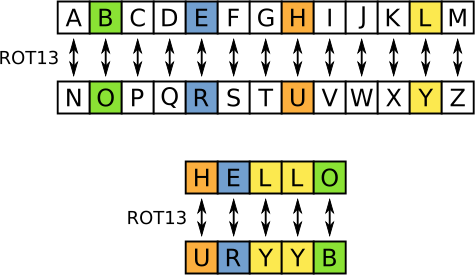
The standard Latin alphabet consists of 26 letters (2x13). Basically, ROT13 algorithm substitutes one letter for another, and uses this same algorithm to reverse the result. For example, algorithm ROT13 turns the letter "A" to "N", and uses ROT13 algorithm for "N" to get "A" as the substitute.
ROT13 is often considered a prime example of a weak encryption.
The following ROT13 class provides a static rotate(int) method that accepts a letter as input and returns a substitute letter as output using ROT13 algorithm:
ROT13.java
package org.o7planning.filterwriter.ex;
public class ROT13 {
/**
* <pre>
* a <==> n
* b <==> o
* c <==> p
* d <==> q
* e <==> r
* ...
* y <==> l
* z <==> m
* </pre>
*/
public static int rotate(int inChar) {
int outChar;
if (inChar >= (int) 'a' && inChar <= (int) 'z') {
outChar = (((inChar - 'a') + 13) % 26) + 'a';
} else if (inChar >= (int) 'A' && inChar <= (int) 'Z') {
outChar = (((inChar - 'A') + 13) % 26) + 'A';
} else {
outChar = inChar;
}
return outChar;
}
// Test
public static void main(String[] args) {
for (char ch = 'a'; ch <= 'z'; ch++) {
char m = (char) rotate(ch);
System.out.println(ch + " ==> " + m);
}
System.out.println(" --- ");
for (char ch = 'A'; ch <= 'Z'; ch++) {
char m = (char) rotate(ch);
System.out.println(ch + " ==> " + m);
}
}
}
Write ROT13Writer class extended from FilterWriter class. Characters written in ROT13Writer will be substituted using ROT13 algorithm:
ROT13Writer.java
package org.o7planning.filterwriter.ex;
import java.io.FilterWriter;
import java.io.IOException;
import java.io.Writer;
public class ROT13Writer extends FilterWriter {
public ROT13Writer(Writer out) {
super(out);
}
@Override
public void write(int outChar) throws IOException {
super.write(ROT13.rotate(outChar));
}
@Override
public void write(char[] cbuf, int offset, int length) throws IOException {
char[] tempbuf = new char[length];
for (int i = 0; i < length; i++) {
tempbuf[i] = (char) ROT13.rotate(cbuf[offset + i]);
}
super.write(tempbuf, 0, length);
}
@Override
public void write(String str, int off, int len) throws IOException {
char[] cbuf = str.toCharArray();
this.write(cbuf, off, len);
}
}
An example for using ROT13Writer:
ROT13WriterTest1.java
package org.o7planning.filterwriter.ex;
import java.io.FilterWriter;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
public class ROT13WriterTest1 {
public static void main(String[] args) throws IOException {
Writer targetWriter = new StringWriter();
FilterWriter filterWriter = new ROT13Writer(targetWriter);
String inputString = "Hello";
filterWriter.write(inputString);
filterWriter.close();
String outputString = targetWriter.toString();
System.out.println(inputString + " ==> " + outputString);
}
}
Output:
Hello ==> Uryyb
An example of using ROT13Writer to write data to the file:
ROT13WriterTest2.java
package org.o7planning.filterwriter.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.FilterWriter;
import java.io.IOException;
import java.io.Writer;
public class ROT13WriterTest2 {
public static void main(String[] args) throws IOException {
// Windows: C:/somedir/rot13-out-file.txt
File outFile = new File("/Volumes/Data/test/rot13-out-file.txt");
outFile.getParentFile().mkdirs();
Writer targetWriter = new FileWriter(outFile);
FilterWriter filterWriter = new ROT13Writer(targetWriter);
String inputString = "Uryyb";
filterWriter.write(inputString);
filterWriter.close();
}
}
Output:
rot13-out-file.txt
Hello
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More