Java Writer Tutorial with Examples
1. Writer
Writer is a class in java.io package, which is a base class representing a stream of characters to write characters to a target, such as a text file.
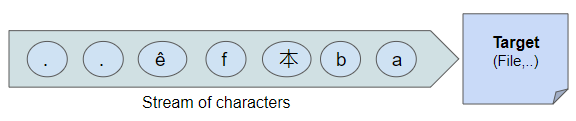
public abstract class Writer implements Appendable, Closeable, Flushable
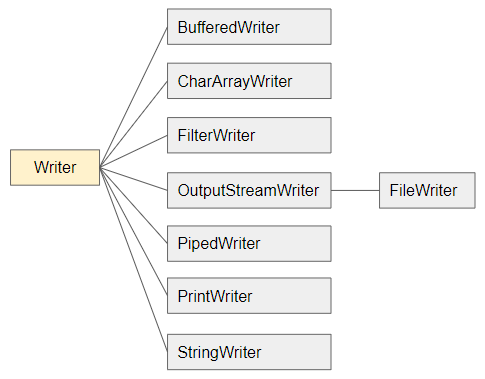
Basically, you cannot use Writer class directly because it is an abstract class. But in a particular case you can use one of its subclasses.
Consider a scenario of writing the following characters to a file with UTF-8 encoding:
JP日本-八洲
Java uses 2 bytes to store a character, and here is an illustration of bytes of the aforementioned text:
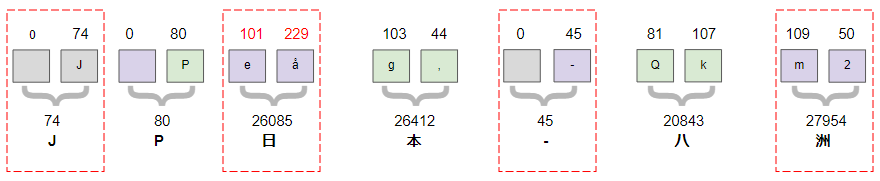
FileWriter is a subclass of Writer commonly used to write characters to a text file. Each character on Writer is 2 bytes, but when you write them to a UTF-8 text file, each character can be stored by 1, 2, 3 or 4 bytes.
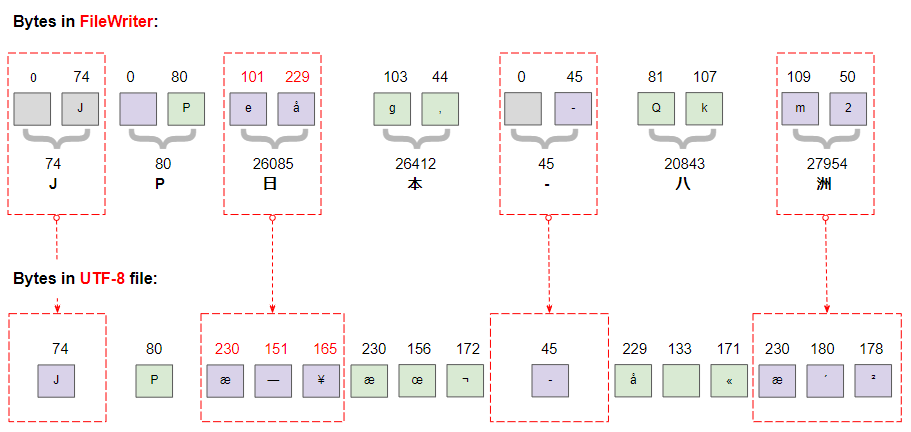
See also my explanation of how Java converts Java characters to UTF-8 characters:
Writer methods
public static Writer nullWriter()
public void write(int c) throws IOException
public void write(char cbuf[]) throws IOException
public void write(char cbuf[], int off, int len) throws IOException
public void write(String str) throws IOException
public void write(String str, int off, int len) throws IOException
public Writer append(CharSequence csq) throws IOException
public Writer append(CharSequence csq, int start, int end) throws IOException
public Writer append(char c) throws IOException
public void flush() throws IOException
public void close() throws IOException
2. write(int)
public void write(int chr) throws IOException
Write a character to Writer. chr parameter is the character's code, which is an integer between 0 and 65535. If the value of chr is out of the range above it will be ignored.
Writer_write_ex1.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_write_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileWriter constructor in Java 11.
Writer writer = new FileWriter(file, StandardCharsets.UTF_8);
writer.write((int)'J'); // 74
writer.write((int)'P'); // 80
writer.write((int)'日'); // 26085
writer.write(26412); // 本
writer.write((int)'-'); // 45
writer.write(20843); // 八
writer.write((int)'洲'); // 27954
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
3. write(char[])
public void write(char[] cbuf) throws IOException
Write a character array to Writer.
Writer_write_ex2.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_write_ex2 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
// FileWriter constructor in Java 11.
Writer writer = new FileWriter(file, StandardCharsets.UTF_8);
writer.write((int)'J'); // 74
char[] cbuf = new char[] {'P', '日', '本', '-', '八', '洲'};
writer.write(cbuf);
writer.close();
}
}
Output:
out-file.txt
JP日本-八洲
4. write(char[], int, int)
public void write(char[] cbuf, int off, int len) throws IOException
Write part of a character array to Writer. Write characters from index offset to offset+len.
Writer_write_ex3.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
public class Writer_write_ex3 {
public static void main(String[] args) throws IOException {
// StringWriter is a subclass of Writer.
StringWriter stringWriter = new StringWriter();
char[] cbuf = new char[] {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'};
stringWriter.write(cbuf, 2, 5); // '2', ...'6'
String s = stringWriter.toString();
System.out.println(s);
stringWriter.close();
}
}
Output:
23456
5. close()
public void close() throws IOException
The close() method is used to close this stream, the flush() method will be called first. Once the stream has been closed, further write() or flush() invocations will cause an IOException to be thrown. Closing a previously closed stream has no effect.
public interface Closeable extends AutoCloseable
Writer class implements Closeable interface. If you write code according to the rules of AutoCloseable, system will automatically close the stream for you without having to call close() method directly.
Writer_close_ex1.java
package org.o7planning.writer.ex;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.nio.charset.StandardCharsets;
public class Writer_close_ex1 {
// Windows path: C:/Somedirectory/out-file.txt
private static final String file_path = "/Volumes/Data/test/out-file.txt";
public static void main(String[] args) throws IOException {
File file = new File(file_path);
// Create parent folder:
file.getParentFile().mkdirs();
try (Writer writer = new FileWriter(file, StandardCharsets.UTF_8)) {
writer.write((int) 'J'); // 74
writer.write((int) 'P'); // 80
writer.write((int) '日'); // 26085
writer.write(26412); // 本
writer.write((int) '-'); // 45
writer.write(20843); // 八
writer.write((int) '洲'); // 27954
}
}
}
- Java Closeable
6. write(String)
public void write(String str) throws IOException
Write a String to Writer.
Example:
writer.write("Some String");
7. write(String, int, int)
public void write(String str, int offset, int len) throws IOException
Write part of a String to Writer. Write characters from index offset to offset+len to Writer.
Example:
writer.write("0123456789", 2, 5); // 23456
8. append(CharSequence)
public Writer append(CharSequence csq) throws IOException
Append CharSequence characters to Writer. This method returns the current Writer object, which allows you to call another Writer method instead of ending with a semicolon (;).
Writer_append_ex1.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
public class Writer_append_ex1 {
public static void main(String[] args) throws IOException {
// StringWriter is a subclass of Writer.
Writer writer = new StringWriter();
writer.append("01234").append("5678").write("9");
System.out.println(writer.toString()); // 0123456789
writer.close();
}
}
9. append(CharSequence, int , int)
public Writer append(CharSequence csq, int start, int end) throws IOException
Append a CharSequence part to Writer. This method returns the current Writer object.
10. append(char)
public Writer append(char chr) throws IOException
Append a character to Writer. This method returns the current Writer object, which allows you to call another Writer method instead of ending with a semicolon ( ; ).
Writer_append_ex2.java
package org.o7planning.writer.ex;
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
public class Writer_append_ex2 {
public static void main(String[] args) throws IOException {
Writer writer = new StringWriter();
writer.append('J').append('P').append('日').append('本');
System.out.println(writer.toString()); // JP日本
writer.close();
}
}
11. flush()
public void flush() throws IOException
The data that you write to Writer is sometimes stored temporarily in a buffer. flush() method is used to flush the entire data on the buffer into the target.
You can read the BufferedWriter article below to understand more about the flush() method.
If the intended destination of this Writer is an abstraction provided by the underlying operating system, for example a file, then flushing the stream guarantees only that characters previously written to the stream are passed to the operating system for writing; it does not guarantee that they are actually written to a physical device such as a disk drive.
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More