Java StringReader Tutorial with Examples
1. StringReader
StringReader class is used to read a String in style of a character input stream.
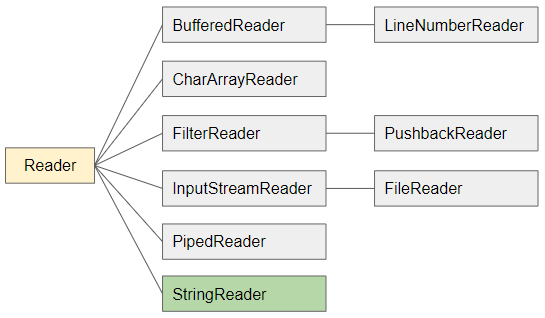
Basically, StringReader is a subclass of Reader. It has no other methods than methods inherited from its parent class. So you can read article about Reader to learn more about these methods.
StringReader constructor
public StringReader(String s)
2. Examples
Example: Read a text, listing the words that appear in text and the number of occurrences of each word.
- Java StreamTokenizer
StringReaderEx2.java
package org.o7planning.stringreader.ex;
import java.io.IOException;
import java.io.Reader;
import java.io.StreamTokenizer;
import java.io.StringReader;
import java.util.HashMap;
import java.util.Map;
public class StringReaderEx2 {
public static void main(String[] args) throws IOException {
String s = "Apple Apricots Apricots Blackberries Apple";
Reader reader = new StringReader(s);
StreamTokenizer tokenizer = new StreamTokenizer(reader); // StreamTokenizer(Reader)
// <String word, int count>
Map<String, Integer> wordsMap = new HashMap<>();
// Read and count the frequency for every word.
while (tokenizer.nextToken() != StreamTokenizer.TT_EOF) {
if (tokenizer.ttype == StreamTokenizer.TT_WORD) {
int count;
if(wordsMap.containsKey(tokenizer.sval)) {
count = wordsMap.get(tokenizer.sval).intValue();
count++;
}
else {
count = 1;
}
wordsMap.put(tokenizer.sval, count);
}
}
wordsMap.forEach((word, count) -> {
System.out.println(word + " : " + count);
});
}
}
Output:
Apple : 2
Apricots : 2
Blackberries : 1
Example: Read a text with multiple lines, print lines that end with "B" and not begin with "#" (Comment line).
StringReaderEx3.java
package org.o7planning.stringreader.ex;
import java.io.BufferedReader;
import java.io.Reader;
import java.io.StringReader;
public class StringReaderEx3 {
public static void main(String[] args) {
String students = //
"# Students:\n" //
+ "John P\n" //
+ "Sarah M\n" //
+ "# Sarah B\n" //
+ "Charles B\n" //
+ "Mary T\n" //
+ "Sophia B\n";
Reader reader = new StringReader(students);
BufferedReader br = new BufferedReader(reader);
br.lines() // return java.util.stream.Stream
.filter(s -> !s.trim().startsWith("#")) // Line not start with #
.filter(s -> s.endsWith("B")) // Line ends with B
.forEach(System.out::println);
}
}
Output:
Charles B
Sophia B
Java IO Tutorials
- Java CharArrayWriter Tutorial with Examples
- Java FilterReader Tutorial with Examples
- Java FilterWriter Tutorial with Examples
- Java PrintStream Tutorial with Examples
- Java BufferedReader Tutorial with Examples
- Java BufferedWriter Tutorial with Examples
- Java StringReader Tutorial with Examples
- Java StringWriter Tutorial with Examples
- Java PipedReader Tutorial with Examples
- Java LineNumberReader Tutorial with Examples
- Java PrintWriter Tutorial with Examples
- Java IO Binary Streams Tutorial with Examples
- Java IO Character Streams Tutorial with Examples
- Java BufferedOutputStream Tutorial with Examples
- Java ByteArrayOutputStream Tutorial with Examples
- Java DataOutputStream Tutorial with Examples
- Java PipedInputStream Tutorial with Examples
- Java OutputStream Tutorial with Examples
- Java ObjectOutputStream Tutorial with Examples
- Java PushbackInputStream Tutorial with Examples
- Java SequenceInputStream Tutorial with Examples
- Java BufferedInputStream Tutorial with Examples
- Java Reader Tutorial with Examples
- Java Writer Tutorial with Examples
- Java FileReader Tutorial with Examples
- Java FileWriter Tutorial with Examples
- Java CharArrayReader Tutorial with Examples
- Java ByteArrayInputStream Tutorial with Examples
- Java DataInputStream Tutorial with Examples
- Java ObjectInputStream Tutorial with Examples
- Java InputStreamReader Tutorial with Examples
- Java OutputStreamWriter Tutorial with Examples
- Java InputStream Tutorial with Examples
- Java FileInputStream Tutorial with Examples
Show More