Java SWT List Tutorial with Examples
1. SWT List
SWT List is UI component which displays the item list as strings, and allow the user to select one or more items.
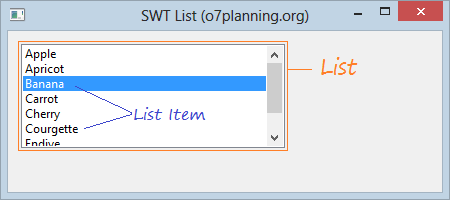
Note: SWT List is a common UI component of a SWT application but the SWT List has some disadvantages below:SWT List only contains items (List Items) with the String type.List-Items can not display icons.To overcome the above disadvantages you can use SWT Table instead of SWT List.
The styles can be applied to SWT List:
- SWT.BORDER
- SWT.MULTI: Allow you to select one or more items.
- SWT.SINGLE: Allow you select the only item.
- SWT.V_SCROLL: Display vertical scroll bar.
- SWT.H_SCROLL: Display horizontal scroll bar.
2. SWT List Example
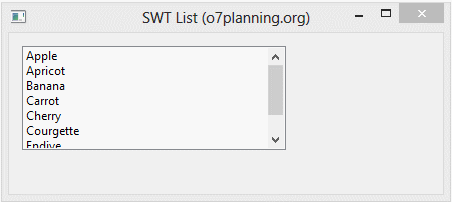
ListDemo.java
package org.o7planning.swt.list;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.List;
import org.eclipse.swt.widgets.Shell;
public class ListDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT List (o7planning.org)");
shell.setSize(450, 200);
RowLayout layout = new RowLayout(SWT.VERTICAL);
layout.spacing = 10;
layout.marginHeight = 10;
layout.marginWidth = 10;
shell.setLayout(layout);
// Create a List
// (Allows selecte multiple lines and display vertical scroll bar.).
final List list = new List(shell, SWT.BORDER | SWT.MULTI | SWT.V_SCROLL);
list.setLayoutData(new RowData(240, 100));
list.add("Apple");
list.add("Apricot");
list.add("Banana");
list.add("Carrot");
list.add("Cherry");
list.add("Courgette");
list.add("Endive");
list.add("Grape");
Label label = new Label(shell, SWT.NONE);
label.setLayoutData(new RowData(240, SWT.DEFAULT));
list.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent event) {
int[] selections = list.getSelectionIndices();
String outText = "";
for (int i = 0; i < selections.length; i++) {
outText += selections[i] + " ";
}
label.setText("You selected: " + outText);
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More