Java SWT FillLayout Tutorial with Examples
1. FillLayout
FillLayout is the simplest layout class. It lays out controls in a single row or column, forcing them to be the same size.
Horizontal FillLayout:
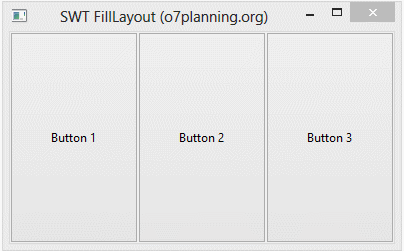
Vertical FillLayout:
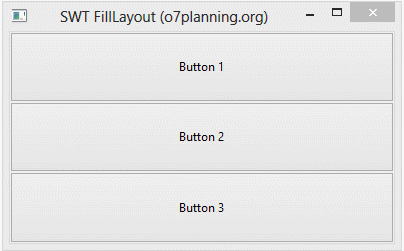
// Create a Horizontal FillLayout.
FillLayout fillLayout= new FillLayout(SWT.HORIZONTAL);
// Set Layout for component
component.setLayout(fillLayout);
// Create a Vertical FillLayout.
FillLayout fillLayout= new FillLayout(SWT.VERTICAL);
// Set Layout for component
component.setLayout(fillLayout);
margin & spacing
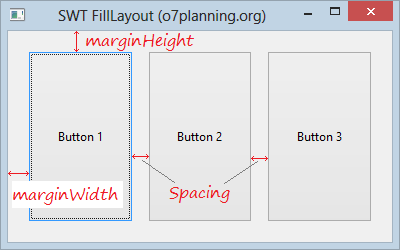
FillLayout fillLayout= new FillLayout(SWT.HORIZONTAL);
fillLayout.marginHeight= 20;
fillLayout.marginWidth= 20;
fillLayout.spacing=15;
2. FillLayout Example
Horizontal FillLayout example:
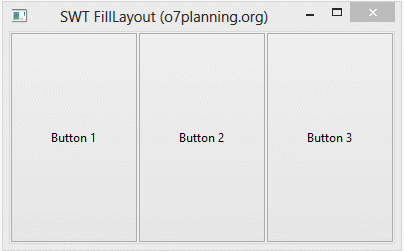
HorizontalFillLayoutDemo.java
package org.o7planning.swt.filllayout;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class HorizontalFillLayoutDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT FillLayout (o7planning.org)");
// Create a Horizontal FillLayout.
FillLayout fillLayout= new FillLayout(SWT.HORIZONTAL);
shell.setLayout(fillLayout);
Button button1= new Button(shell, SWT.NONE);
button1.setText("Button 1");
Button button2= new Button(shell, SWT.NONE);
button2.setText("Button 2");
Button button3= new Button(shell, SWT.NONE);
button3.setText("Button 3");
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Horizontal FillLayout with margin & spacing:
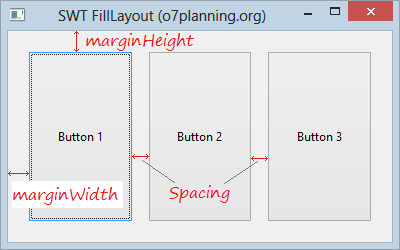
HorizontalFillLayoutDemo2.java
package org.o7planning.swt.filllayout;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class HorizontalFillLayoutDemo2 {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT FillLayout (o7planning.org)");
FillLayout fillLayout= new FillLayout(SWT.HORIZONTAL);
fillLayout.marginHeight= 20;
fillLayout.marginWidth= 20;
fillLayout.spacing=15;
shell.setLayout(fillLayout);
Button button1= new Button(shell, SWT.NONE);
button1.setText("Button 1");
Button button2= new Button(shell, SWT.NONE);
button2.setText("Button 2");
Button button3= new Button(shell, SWT.NONE);
button3.setText("Button 3");
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More