Java SWT Radio Button Tutorial with Examples
1. SWT Radio Button
In SWT, the radio is an object of Button with SWT.RADIO style.
The same as a toggle button, radio button has the two states selected and deselected. When radio buttons are combined into a Group or Composite, at a time only one button is selected. Unlike toggle button, users can only deselect a radio button by selecting a different radio button in the same Group (or Composite).
// Create a radio button
Button radio = new Button(parent, SWT.RADIO);
ToggleButton:
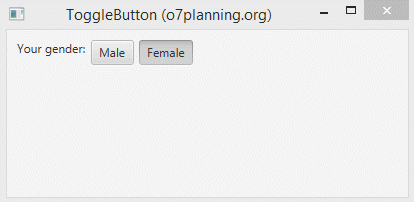
RadioButton:
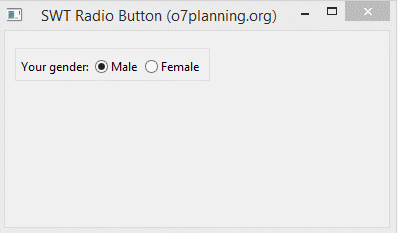
// Create a group to contain 2 radio (Male & Female)
Group genderGroup = new Group(shell, SWT.NONE);
genderGroup.setLayout(new RowLayout(SWT.HORIZONTAL));
Button buttonMale = new Button(genderGroup, SWT.RADIO);
buttonMale.setText("Male");
Button buttonFemale = new Button(genderGroup, SWT.RADIO);
buttonFemale.setText("Female");
You can also set the icon for radio button by using setImage method.
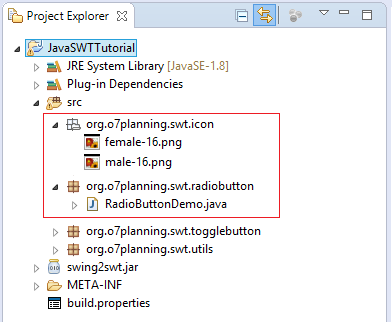
InputStream input
= RadioButtonDemo.class.getResourceAsStream("/org/o7planning/swt/icon/male-16.png");
Image image = new Image(null, input);
radioButton.setImage(image);
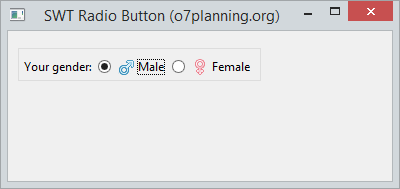
2. RadioButton Example
Radio buttons are put in the same Group or Composite, only one button is selected at a time. When you select a different radio button in the same Group (or Composite), the current radio will be deselected. So you should put radios with the same theme in the same Group (or Compsite).
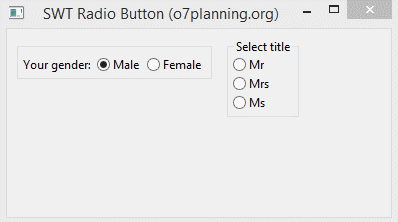
RadioButtonDemo.java
package org.o7planning.swt.radiobutton;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class RadioButtonDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Radio Button (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Create a group to contain 2 radio (Male & Female)
// Tạo một nhóm để chứa 2 radio (Male & Female).
Group genderGroup = new Group(shell, SWT.NONE);
genderGroup.setLayout(new RowLayout(SWT.HORIZONTAL));
Label label = new Label(genderGroup, SWT.NONE);
label.setText("Your gender: ");
Button buttonMale = new Button(genderGroup, SWT.RADIO);
buttonMale.setText("Male");
Button buttonFemale = new Button(genderGroup, SWT.RADIO);
buttonFemale.setText("Female");
// Group
Group titleGroup = new Group(shell, SWT.NONE);
titleGroup.setLayout(new RowLayout(SWT.VERTICAL));
titleGroup.setText("Select title");
Button buttonMr = new Button(titleGroup, SWT.RADIO);
buttonMr.setText("Mr");
Button buttonMrs = new Button(titleGroup, SWT.RADIO);
buttonMrs.setText("Mrs");
Button buttonMs = new Button(titleGroup, SWT.RADIO);
buttonMs.setText("Ms");
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. Processing Events for RadioButton
The example below handles the event when the user selects the radio button.
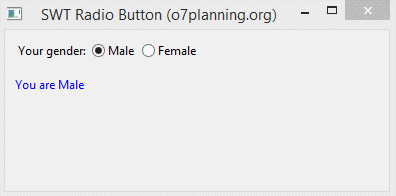
RadioButtonEventDemo.java
package org.o7planning.swt.radiobutton;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class RadioButtonCancelChangeDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Radio Button (o7planning.org)");
RowLayout rowLayout = new RowLayout(SWT.VERTICAL);
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Group
Composite genderGroup = new Composite (shell, SWT.NONE);
genderGroup.setLayout(new RowLayout(SWT.HORIZONTAL));
Label label = new Label(genderGroup, SWT.NONE);
label.setText("Select Titles: ");
// Radio - mrs
Button buttonMale = new Button(genderGroup, SWT.RADIO);
buttonMale.setText("Male");
// Radio - mss
Button buttonFemale = new Button(genderGroup, SWT.RADIO);
buttonFemale.setText("Female");
Label labelAnswer = new Label(shell, SWT.NONE);
labelAnswer.setForeground(display.getSystemColor(SWT.COLOR_BLUE));
buttonMale.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
Button source= (Button) e.getSource();
if(source.getSelection()) {
labelAnswer.setText("You are "+ source.getText());
labelAnswer.pack();
}
}
});
buttonFemale.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
Button source= (Button) e.getSource();
if(source.getSelection()) {
labelAnswer.setText("You are "+ source.getText());
labelAnswer.pack();
}
}
});
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More