Java SWT ProgressBar Tutorial with Examples
1. SWT ProgressBar
ProgressBar is UI control that visualize progress of any operations in your SWT applications.
The figure below illustrates 3 ProgressBars, 2 first ProgressBars describe the progress of work with a definite volume. The last one describes the progress of work with indefinite volume.
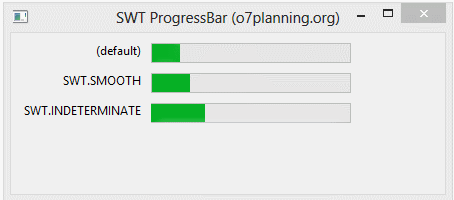
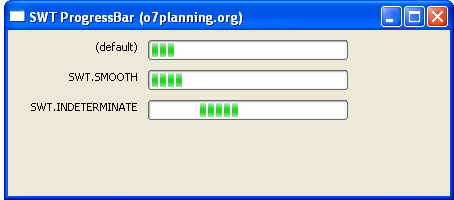
Vertical ProgressBar:
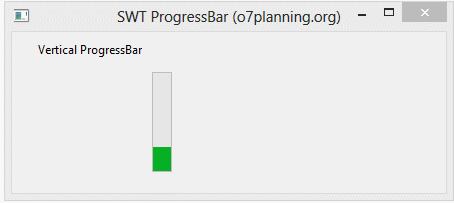
The styles can be applied to ProgressBar:
- SMOOTH
- HORIZONTAL
- VERTICAL
- INDETERMINATE
// Horizontal ProgressBar
ProgressBar progressBar1 = new ProgressBar(shell, SWT.NULL);
ProgressBar progressBar2 = new ProgressBar(shell, SWT.SMOOTH);
ProgressBar progressBar3 = new ProgressBar(shell, SWT.INDETERMINATE);
// Vertical ProgressBar
ProgressBar progressBar4 = new ProgressBar(shell, SWT.VERTICAL | SWT.SMOOTH);
2. SWT ProgressBar Example
For example. Create ProgressBars with different styles:
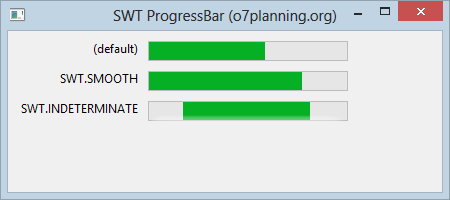
ProgressDemo.java
package org.o7planning.swt.progressbar;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.ProgressBar;
import org.eclipse.swt.widgets.Shell;
public class ProgressBarDemo {
public ProgressBarDemo() {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT ProgressBar (o7planning.org)");
shell.setSize(450, 200);
ProgressBar progressBar1 = new ProgressBar(shell, SWT.NULL);
ProgressBar progressBar2 = new ProgressBar(shell, SWT.SMOOTH);
ProgressBar progressBar3 = new ProgressBar(shell, SWT.INDETERMINATE);
progressBar1.setMinimum(30);
progressBar1.setMaximum(250);
progressBar1.setSelection(160);
progressBar2.setMinimum(30);
progressBar2.setMaximum(250);
progressBar2.setSelection(200);
progressBar1.setBounds(140, 10, 200, 20);
progressBar2.setBounds(140, 40, 200, 20);
progressBar3.setBounds(140, 70, 200, 20);
Label label1 = new Label(shell, SWT.NULL);
label1.setText("(default)");
Label label2 = new Label(shell, SWT.NULL);
label2.setText("SWT.SMOOTH");
Label label3 = new Label(shell, SWT.NULL);
label3.setText("SWT.INDETERMINATE");
label1.setAlignment(SWT.RIGHT);
label2.setAlignment(SWT.RIGHT);
label3.setAlignment(SWT.RIGHT);
label1.setBounds(10, 10, 120, 20);
label2.setBounds(10, 40, 120, 20);
label3.setBounds(10, 70, 120, 20);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
public static void main(String[] args) {
new ProgressBarDemo();
}
}
3. ProgressBar and Thread
You can create a Thread object to perform certain task, such as copying files list. Copying requires take some time, you need to use ProgressBar to display the percentage of work already done.
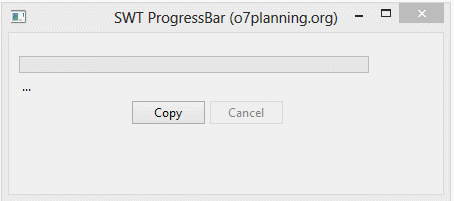
ProgressBarCopyDemo.java
package org.o7planning.swt.progressbar;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.ProgressBar;
import org.eclipse.swt.widgets.Shell;
public class ProgressBarCopyDemo {
private CopyThread copyThread = null;
public ProgressBarCopyDemo() {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT ProgressBar (o7planning.org)");
shell.setSize(450, 200);
shell.setLayout(null);
ProgressBar progressBar = new ProgressBar(shell, SWT.NONE);
progressBar.setBounds(10, 23, 350, 17);
Label labelInfo = new Label(shell, SWT.NONE);
labelInfo.setBounds(10, 46, 350, 15);
labelInfo.setText(" ...");
// Button Copy
Button buttonCopy = new Button(shell, SWT.NONE);
buttonCopy.setBounds(122, 67, 75, 25);
buttonCopy.setText("Copy");
// Button Cancel
Button buttonCancel = new Button(shell, SWT.NONE);
buttonCancel.setBounds(200, 67, 75, 25);
buttonCancel.setText("Cancel");
buttonCancel.setEnabled(false);
buttonCopy.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
copyThread = new CopyThread(display, progressBar, labelInfo, buttonCopy, buttonCancel);
copyThread.start();
}
});
buttonCancel.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
if (copyThread != null) {
copyThread.cancel();
}
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
public static void main(String[] args) {
new ProgressBarCopyDemo();
}
}
CopyThread.java
package org.o7planning.swt.progressbar;
import java.io.File;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.ProgressBar;
public class CopyThread extends Thread {
private Display display;
private ProgressBar progressBar;
private Button buttonCopy;
private Button buttonCancel;
private Label labelInfo;
private boolean cancel;
public CopyThread(Display display, ProgressBar progressBar, //
Label labelInfo, Button buttonCopy, Button buttonCancel) {
this.display = display;
this.progressBar = progressBar;
this.buttonCopy = buttonCopy;
this.buttonCancel = buttonCancel;
this.labelInfo = labelInfo;
}
@Override
public void run() {
if (display.isDisposed()) {
return;
}
this.updateGUIWhenStart();
// Copy All file In C:/Windows
File dir = new File("C:/Windows");
File[] files = dir.listFiles();
int count = files.length;
int i = 0;
for (File file : files) {
if (cancel) {
break;
}
i++;
if (file.isFile()) {
this.copy(file);
} else {
continue;
}
this.updateGUIInProgress(file, i, count);
}
this.updateGUIWhenFinish();
}
private void copy(File file) {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
}
}
private void updateGUIWhenStart() {
display.asyncExec(new Runnable() {
@Override
public void run() {
buttonCopy.setEnabled(false);
buttonCancel.setEnabled(true);
}
});
}
private void updateGUIWhenFinish() {
display.asyncExec(new Runnable() {
@Override
public void run() {
buttonCopy.setEnabled(true);
buttonCancel.setEnabled(false);
progressBar.setSelection(0);
progressBar.setMaximum(1);
if (cancel) {
labelInfo.setText("Cancelled!");
} else {
labelInfo.setText("Finished!");
}
}
});
}
private void updateGUIInProgress(File file, int value, int count) {
display.asyncExec(new Runnable() {
@Override
public void run() {
labelInfo.setText("Copying file: " + file.getAbsolutePath());
progressBar.setMaximum(count);
progressBar.setSelection(value);
}
});
}
public void cancel() {
this.cancel = true;
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More