Java SWT Label Tutorial with Examples
1. SWT Label
SWT Label is an UI Component that can displays text or image but can not display both simultaneously. To display both of them simultaneously, you can use CLabel, a subclass of the Label.
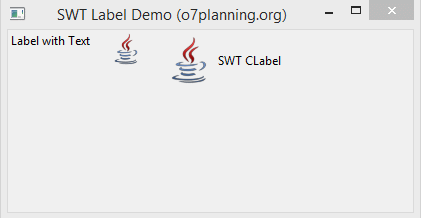
2. Label example
This is a simple example of Label displaying text. Label class sometimes is used to create Separators horizontally or vertically.
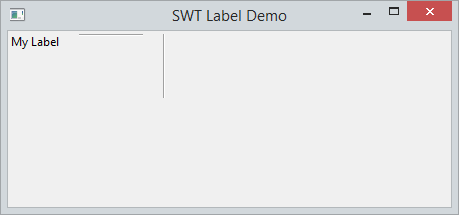
LabelDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class LabelDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
// Create a Label with Text
Label label1 = new Label(shell, SWT.NONE);
label1.setText("My Label");
// Create a Label is Horizontal Separator
Label hSeparator = new Label(shell, SWT.SEPARATOR | SWT.HORIZONTAL);
// Create a Label is Vertical Separator
Label vSeparator = new Label(shell, SWT.SEPARATOR | SWT.VERTICAL);
shell.setText("SWT Label Demo");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
3. Label with Icon
Note that the Label class can display Icon or Text, if you want to display both text & Icon, you should use CLabel class.
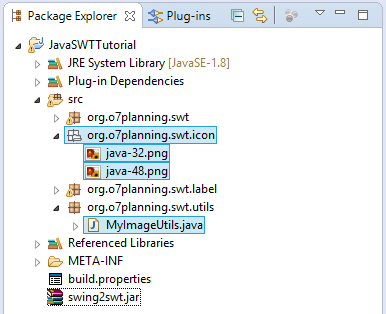
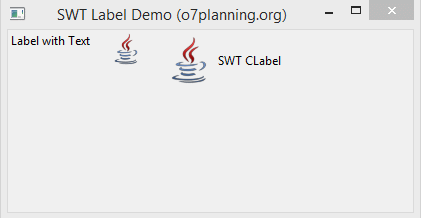
MyImageUtils.java
package org.o7planning.swt.utils;
import java.io.IOException;
import java.io.InputStream;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.widgets.Display;
public class MyImageUtils {
// resourcePath: "/org/o7planning/swt/icon/java-32.png"
public static Image getImage(Display display, String resourcePath) {
InputStream input = null;
try {
// /org/o7planning/swt/icon/java-32.png
input = MyImageUtils.class.getResourceAsStream(resourcePath);
Image image = new Image(display, input);
return image;
} finally {
closeQuietly(input);
}
}
private static void closeQuietly(InputStream is) {
try {
if (is != null) {
is.close();
}
} catch (IOException e) {
}
}
}
View full example:
LabelIconDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.CLabel;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.o7planning.swt.utils.MyImageUtils;
public class LabelIconDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
Image image = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/java-48.png");
Image image2 = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/java-32.png");
// Create a Label with Text
Label label1 = new Label(shell, SWT.NONE);
label1.setText("Label with Text");
// Create a Label with Icon
Label label2 = new Label(shell, SWT.NONE);
label2.setImage(image2);
// Create a CLabel with Text & Icon
CLabel cLabel = new CLabel(shell, SWT.NONE);
cLabel.setImage(image);
cLabel.setText("SWT CLabel");
shell.setText("SWT Label Demo (o7planning.org)");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. Label with Font and Color
See more SWT Font & Color:
- swt font and color
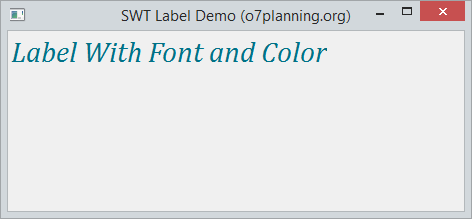
Font
You can set font (Include Font name and size) for the Label through setFont method.
// Tạo một đối tượng Font mới
Font font = new Font(display, "Cambria", 22, SWT.ITALIC);
label.setFont(font);
Color
Using setForeground method to set the text color for the Label.
Color color = new Color(display, 0, 114, 135);
// Set fore color
label.setForeground(color);
View full example:
LabelFullDemo.java
package org.o7planning.swt.label;
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class LabelFontColorDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
RowLayout layout = new RowLayout();
layout.spacing = 20;
shell.setLayout(layout);
// ------ Label -----
Label label = new Label(shell, SWT.NONE);
label.setText("Label With Font and Color");
label.setFont(new Font(display, "Cambria", 22, SWT.ITALIC));
Color color = new Color(display, 0, 114, 135);
// Set fore color
label.setForeground(color);
shell.setText("SWT Label Demo (o7planning.org)");
shell.setSize(450, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More