Java SWT Spinner Tutorial with Examples
1. SWT Spinner
In SWT, Spinner allows user to choose the value of a set of numeric values. Unfortunately SWT Spinner does not allow you to choose the value Object.
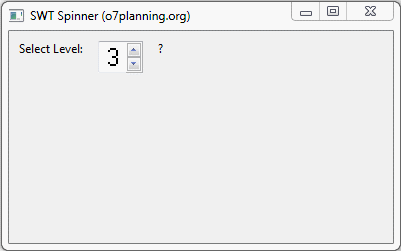
2. Spinner Example
The simple example below illustrates a Spinner with numerical values.
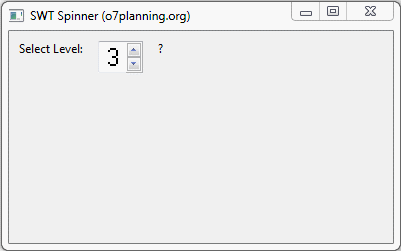
SpinnerDemo.java
package org.o7planning.swt.spinner;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.layout.RowData;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Spinner;
public class SpinnerDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Spinner (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Label
Label label = new Label(shell, SWT.NONE);
label.setText("Select Level:");
// Spinner
final Spinner spinner = new Spinner(shell, SWT.BORDER);
spinner.setMinimum(1);
spinner.setMaximum(5);
spinner.setSelection(3);
Font font = new Font(display, "Courier", 20, SWT.NORMAL);
spinner.setFont(font);
// Label
Label labelMsg = new Label(shell, SWT.NONE);
labelMsg.setText("?");
labelMsg.setLayoutData(new RowData(150, SWT.DEFAULT));
spinner.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
labelMsg.setText("You select: " + spinner.getSelection());
}
});
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More