Java SWT Toggle Button Tutorial with Examples
1. SWT Toggle Button
In SWT, the toggle is an object of Button with SWT.TOGGLE style.
Toggle button have two options "selected" and "not selected". By default, Toggle buttons are independent, and they don't depend on other Toggle buttons.
// Create a radio button
Button radio = new Button(parent, SWT.TOGGLE);
Toggles running on the different OSs are different on interface. Below is the Toggle button image when Toggle runs on Windows XP and Windows 8:
Toggle Button (Windows XP):
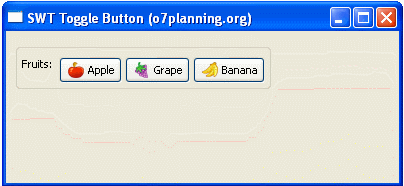
Toggle Button (Windows 8)
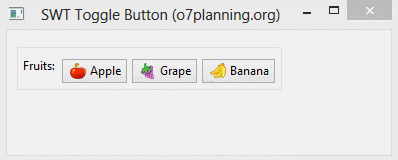
In fact, for Windows 8 you have difficulty in distinguishing between Toggle Button with "selected" state and Toggle Button with "not selected" state. The solution to solve this problem is to use the different icons for the "selected" state and "not selected".
// Create a Toggle Button
Button appleButton = new Button(parent, SWT.TOGGLE);
appleButton.setText("Apple");
You can also set the icon for toggle button by using setImage method.
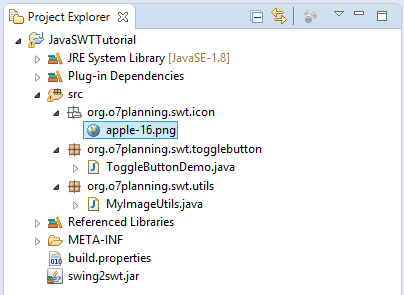
InputStream input
= RadioButtonDemo.class.getResourceAsStream("/org/o7planning/swt/icon/apple-16.png");
Image image = new Image(null, input);
toggleButton.setImage(image);
2. ToggleButton Example
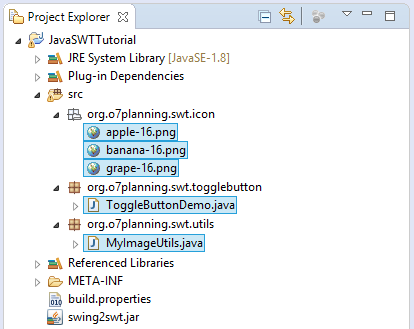
In SWT, Toggle Buttons are independent of each other by default. It differs from the manner of the Radio Button, when radio buttons are put on a same Composite or Group, if you select a radio button, all radio buttons will be deselected.
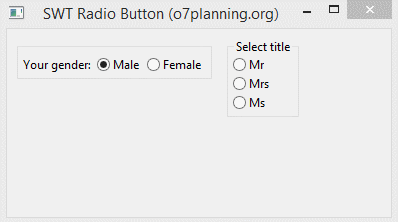
ToggleButtonDemo.java
package org.o7planning.swt.togglebutton;
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.o7planning.swt.utils.MyImageUtils;
public class ToggleButtonDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Toggle Button (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
Group fruitGroup = new Group(shell, SWT.NONE);
fruitGroup.setLayout(new RowLayout(SWT.HORIZONTAL));
Label label = new Label(fruitGroup, SWT.NONE);
label.setText("Fruits: ");
// Apple
Button appleButton = new Button(fruitGroup, SWT.TOGGLE);
appleButton.setText("Apple");
Image appleIcon = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/apple-16.png");
appleButton.setImage(appleIcon);
// Grape
Button grapeButton = new Button(fruitGroup, SWT.TOGGLE);
grapeButton.setText("Grape");
Image grapeIcon = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/grape-16.png");
grapeButton.setImage(grapeIcon);
// Banana
Button bananaButton = new Button(fruitGroup, SWT.TOGGLE);
bananaButton.setText("Banana");
Image bananaIcon = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/banana-16.png");
bananaButton.setImage(bananaIcon);
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Running examples (See on Windows XP & Windows 8)
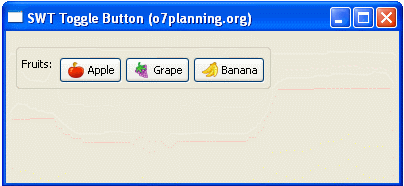
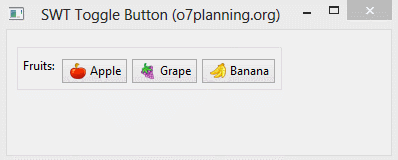
3. Processing Events for Toggle Button
The example below process event when the user selects Toggle buttons.
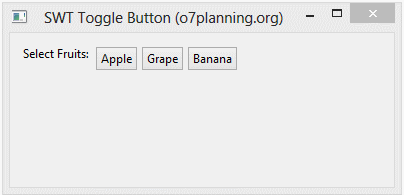
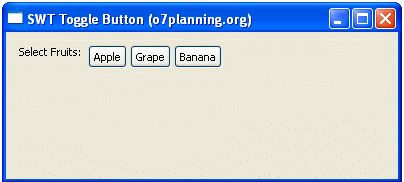
RadioButtonEventDemo.java
package org.o7planning.swt.togglebutton;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.events.SelectionListener;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
public class ToggleButtonEventDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Toggle Button (o7planning.org)");
RowLayout rowLayout = new RowLayout(SWT.VERTICAL);
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
// Composite
Composite composite = new Composite(shell, SWT.NONE);
composite.setLayout(new RowLayout(SWT.HORIZONTAL));
Label label = new Label(composite, SWT.NONE);
label.setText("Select Fruits: ");
// Apple
Button toggleApple = new Button(composite, SWT.TOGGLE);
toggleApple.setText("Apple");
// Grape
Button toggleGrape = new Button(composite, SWT.TOGGLE);
toggleGrape.setText("Grape");
// Banana
Button toggleBanana = new Button(composite, SWT.TOGGLE);
toggleBanana.setText("Banana");
Label labelAnswer = new Label(shell, SWT.NONE);
labelAnswer.setForeground(display.getSystemColor(SWT.COLOR_BLUE));
SelectionListener selectionListener = new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
Button source = (Button) e.getSource();
if (source.getSelection()) {
labelAnswer.setText("You select " + source.getText());
labelAnswer.pack();
} else {
labelAnswer.setText("You deselect " + source.getText());
labelAnswer.pack();
}
}
};
toggleApple.addSelectionListener(selectionListener);
toggleGrape.addSelectionListener(selectionListener);
toggleBanana.addSelectionListener(selectionListener);
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
4. Use different icons for different states
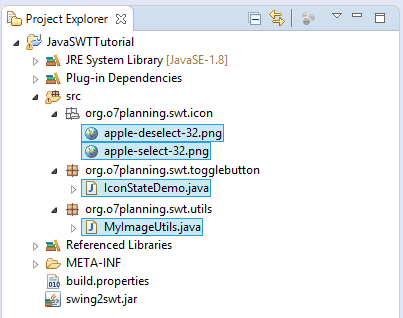
As mentioned above, for Window 8, the user sometimes has difficulty in distinguish between the state "selected" and "not selected" of Toggle Button. The solution is to use different icons for different states.
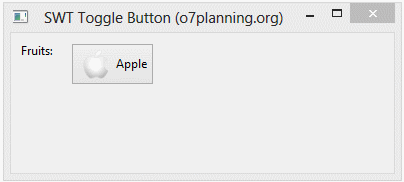
IconStateDemo.java
package org.o7planning.swt.togglebutton;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.layout.RowLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.o7planning.swt.utils.MyImageUtils;
public class IconStateDemo {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("SWT Toggle Button (o7planning.org)");
RowLayout rowLayout = new RowLayout();
rowLayout.marginLeft = 10;
rowLayout.marginTop = 10;
rowLayout.spacing = 15;
shell.setLayout(rowLayout);
Label label = new Label(shell, SWT.NONE);
label.setText("Fruits: ");
Image iconSelect = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/apple-select-32.png");
Image iconDeselect = MyImageUtils.getImage(display, "/org/o7planning/swt/icon/apple-deselect-32.png");
// Apple
Button appleButton = new Button(shell, SWT.TOGGLE);
appleButton.setText("Apple");
appleButton.setImage(iconDeselect);
appleButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
Button source = (Button) e.getSource();
if (source.getSelection()) {
appleButton.setImage(iconSelect);
} else {
appleButton.setImage(iconDeselect);
}
}
});
shell.setSize(400, 250);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
Java SWT Tutorials
- Java SWT FillLayout Tutorial with Examples
- Java SWT RowLayout Tutorial with Examples
- Java SWT SashForm Tutorial with Examples
- Java SWT Label Tutorial with Examples
- Java SWT Button Tutorial with Examples
- Java SWT Toggle Button Tutorial with Examples
- Java SWT Radio Button Tutorial with Examples
- Java SWT Text Tutorial with Examples
- Java SWT Password Field Tutorial with Examples
- Java SWT Link Tutorial with Examples
- Programming Java Desktop Application Using SWT
- Java SWT Combo Tutorial with Examples
- Java SWT Spinner Tutorial with Examples
- Java SWT Slider Tutorial with Examples
- Java SWT Scale Tutorial with Examples
- Java SWT ProgressBar Tutorial with Examples
- Java SWT TabFolder and CTabFolder Tutorial with Examples
- Java SWT List Tutorial with Examples
Show More