Java ArrayList Tutorial with Examples
1. ArrayList
ArrayList is a class that implements List interface and supports all the features of List including optional ones. Basically an ArrayList manages an array to store its elements, which can be replaced by another array of larger length if the number of ArrayList's elements increases.
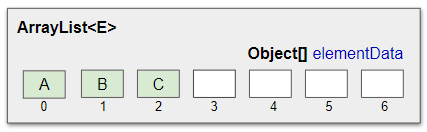
public class ArrayList<E> extends AbstractList<E>
implements List<E>, RandomAccess, Cloneable, java.io.Serializable
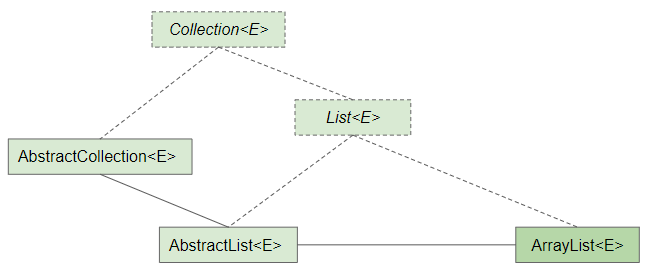
- Collection
- List
- LinkedList
- CopyOnWriteArrayList
Here are the characteristics of ArrayList:
- ArrayList allows to contain duplicate and null elements.
- ArrayList maintains insertion order of the elements.
- ArrayList is not synchronized, so it needs to be synchronized to be used in a Multithreading environment.
- ArrayList allows random access to elements based on the index.
- Operation in ArrayList is a bit slower than LinkedList (See more explanation in operation principle of ArrayList).
ArrayList is quite similar to Vector, except it is asynchronous. In a Multithreading environment, it should be wrapped using Collections.synchronizedList method.
ArrayList<String> arrayList = new ArrayList<String>();
List<String> syncList = Collections.synchronizedList(arrayList);
CopyOnWriteArrayList is a thread-safe variant of ArrayList that you might consider to use:
ArrayList constructors:
ArrayList() | Create an empty ArrayList object with an internal array with an initial capacity of 10 elements. |
ArrayList(Collection<? extends E> c) | Create an ArrayList object containing all elements of the specified Collection, the order of elements is determined by Iterator of Collection. |
ArrayList(int initialCapacity) | Create an empty ArrayList object with an internal array of the specified initial capacity. |
2. How does ArrayList store elements?
ArrayList manages an array of objects. All actions of adding, inserting or removing an element from the ArrayList will produce action of assigning (or updating) value to the other elements of the array, which can affect many elements of the array.
arrayList.add(X)
When you add an element to ArrayList, it will be assigned to an element of array at the index arrayList.size().
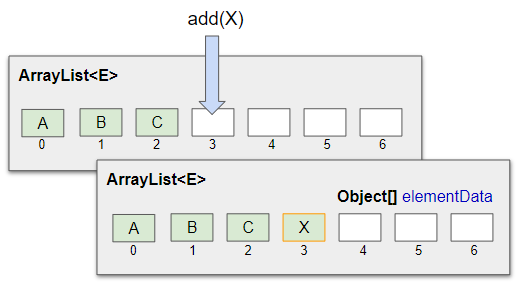
arrayList.add(idx,X)
When you insert an element into ArrayList, many elements on the array will have to update their values.
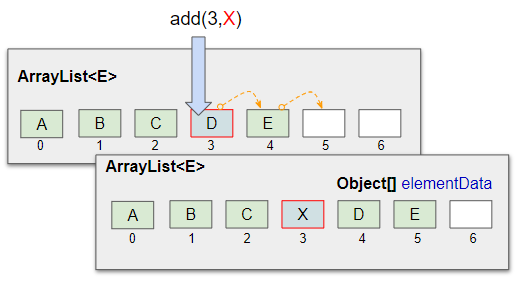
arrayList.remove(idx)
Removing an element at index idx from ArrayList also causes many elements of the array to update with new values.
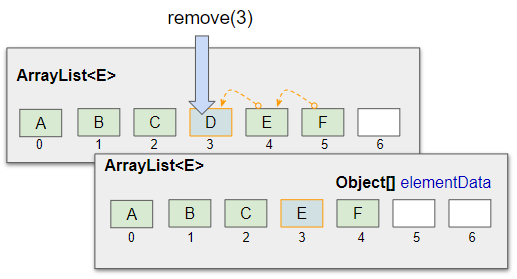
ArrayList replaces the array it manages with a new array of a greater length if the number of elements added is greater than the length of the current array.
3. Examples
For example, using ArrayList in a Multithreading environment:
ArrayList_sync.java
package org.o7planning.arraylist.ex;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ArrayList_sync {
public static void main(String[] args) throws InterruptedException {
new ArrayList_sync();
}
public ArrayList_sync() throws InterruptedException {
ArrayList<String> arrayList = new ArrayList<String>();
List<String> syncList = Collections.synchronizedList(arrayList);
ThreadA threadA = new ThreadA(syncList);
ThreadB threadB = new ThreadB(syncList);
threadA.start();
threadB.start();
threadA.join();
threadB.join();
for(String s: syncList) {
System.out.println(s);
}
}
class ThreadA extends Thread {
private List<String> list;
public ThreadA(List<String> list) {
this.list = list;
}
@Override
public void run() {
for(int i= 0; i< 1000; i++) {
this.list.add("A "+ i);
}
}
}
class ThreadB extends Thread {
private List<String> list;
public ThreadB(List<String> list) {
this.list = list;
}
@Override
public void run() {
for(int i= 0; i< 1000; i++) {
this.list.add("B "+ i);
}
}
}
}
Output:
...
A 938
B 898
A 939
B 899
A 940
B 900
A 941
B 901
A 942
...
ArrayList supports all the features specified in List interface, including optional features. You can find the best examples of ArrayList in the article below:
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More