Java ArrayBlockingQueue Tutorial with Examples
1. ArrayBlockingQueue
ArrayBlockingQueue<E> is a class that implements the BlockingQueue<E> interface, so it has all the features of this interface. See BlockingQueue article for better understanding with basic examples.
public class ArrayBlockingQueue<E> extends AbstractQueue<E>
implements BlockingQueue<E>, java.io.Serializable
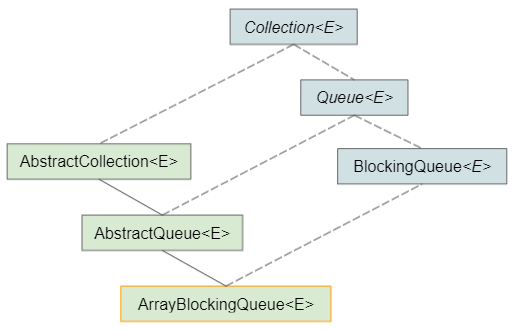
- Collection
- BlockingQueue
- Queue
ArrayBlockingQueue is a limited capacity queue, which contains a fixed-length internal array to store the elements. This queue sorts the elements according to the FIFO - First In First Out rule. The elements at the head of the queue are the elements that have been in the queue the longest, the elements at the tail of the queue are the newly added elements.
The following figure illustrates how ArrayBlockingQueue stores its elements on the internal array.
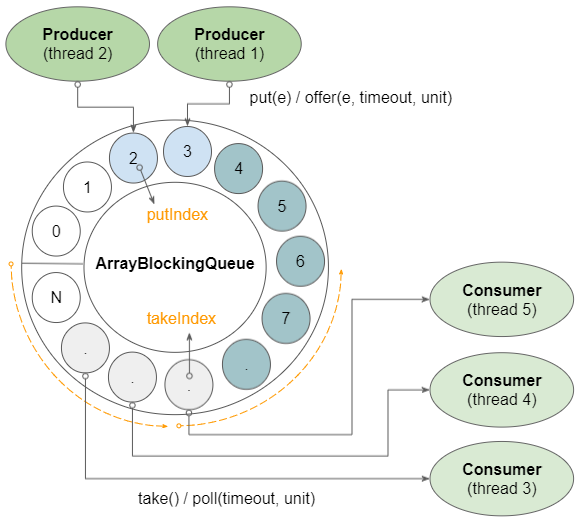
- Adding a new element to this queue corresponds to assigning a new value to the element of the array at index putIndex. Also, if putIndex is 0 it will be assigned to arrayLength-1, otherwise it will be assigned to putIndex-1.
- Taking an element out of this queue corresponds to returning the element of the array at index takeIndex. Also, if takeIndex is 0 it will be assigned to arrayLength-1, otherwise it will be assigned to takeIndex-1.
ArrayBlockingQueue supports fair policy as an option when creating objects. Once the fair policy is used, this queue will respect the order in which the Producer and Consumer threads are waiting. This avoids the starvation of a certain thread, such as a starving Consumer thread due to long waiting time without receiving any element from the queue.
ArrayBlockingQueue and its Iterator support all the optional methods defined in the Collectionand Iterator interfaces.
- Iterator
- Collection
2. Constructors
The constructors of the ArrayBlockingQueue class:
public ArrayBlockingQueue(int capacity)
public ArrayBlockingQueue(int capacity, boolean fair)
public ArrayBlockingQueue(int capacity, boolean fair, Collection<? extends E> c)
3. ArrayBlockingQueue(int)
public ArrayBlockingQueue(int capacity)
Creates an ArrayBlockingQueue object with the specified (fixed) capacity. Using this constructor is equivalent to using the ArrayBlockingQueue(capacity,false) constructor.
The "fair" policy is not applied to the ArrayBlockingQueue object created by this constructor, whose behavior is undefined.
4. ArrayBlockingQueue(int, boolean)
public ArrayBlockingQueue(int capacity, boolean fair)
Creates an ArrayBlockingQueue object with a specified (fixed) capacity, with a specified "fair" policy.
fair = true
A "fair" policy will be applied to this queue, which respects the order in which the Producer and Consumer threads are waiting according to FIFO rules, meaning that the thread that waits first will be processed first.
The "fair" policy helps to avoid starvation of a certain thread, for example, a Consumer waits for a long time but does not receive any elements from the queue.
fair = false
The "fair" policy is not applied, the order for the processing of threads is undefined
5. ArrayBlockingQueue(int, boolean, Collection)
public ArrayBlockingQueue(int capacity, boolean fair, Collection<? extends E> c)
Creates an ArrayBlockingQueue object with the specified (fixed) capacity, specified "fair" policy, and provided initial elements.
Example:
Collection<String> initialElements = List.of("A", "B", "C");
BlockingQueue<String> queue = new ArrayBlockingQueue<>(10, true, initialElements);
6. Methods
The methods that are inherited from the BockingQueue<E> interface:
void put(E e) throws InterruptedException;
boolean offer(E e, long timeout, TimeUnit unit) throws InterruptedException;
E take() throws InterruptedException;
E poll(long timeout, TimeUnit unit) throws InterruptedException;
int remainingCapacity();
int drainTo(Collection<? super E> c);
int drainTo(Collection<? super E> c, int maxElements);
See the BlockingQueue article to learn how to use the methods above.
Methods that are inherited from the Queue<E> interface:
boolean add(E e);
boolean offer(E e);
E remove();
E poll();
E element();
E peek();
The methods are inherited from the Collection<E> interface:
int size();
boolean isEmpty();
boolean contains(Object o);
Iterator<E> iterator();
Object[] toArray();
<T> T[] toArray(T[] a);
boolean add(E e);
boolean remove(Object o);
boolean containsAll(Collection<?> c);
boolean addAll(Collection<? extends E> c);
boolean removeAll(Collection<?> c);
boolean retainAll(Collection<?> c);
void clear();
boolean equals(Object o);
int hashCode();
default <T> T[] toArray(IntFunction<T[]> generator)
default boolean removeIf(Predicate<? super E> filter)
default Spliterator<E> spliterator()
default Stream<E> stream()
default Stream<E> parallelStream()
- Java Collection
- Java Iterator
Java Collections Framework Tutorials
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java PriorityBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Collections Framework Tutorial with Examples
Show More