Java Queue Tutorial with Examples
1. Queue
Queue is a subinterface of Collection, so it has all the features of Collection. It has methods for you to access or remove the first element, and methods to insert an element into the queue. If you want to access a certain element, you must remove all elements that precede it from the queue.
Queue is a Collection that allows duplicate elements but not null elements.
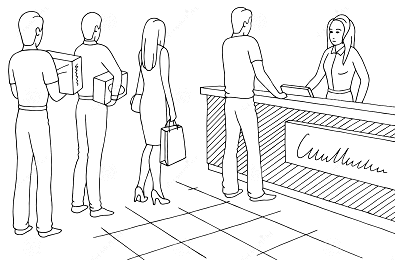
A common example is a queue at a bar. At a time only the first person on the queue is served, the newcomer will be inserted somewhere in the queue. It may not be the last position, depending on the type of queue and the priority of newcomer.
public interface Queue<E> extends Collection<E>
Hierarchy of interfaces in Java Collection Framework:
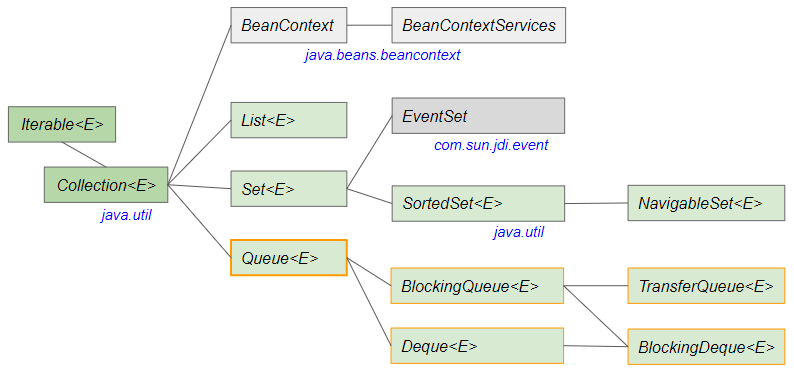
- Collection
- Deque
- BlockingQueue
- TransferQueue
- BlockingDeque
- Set
- List
- NavigableSet
- SortedSet
Hierarchy of classes in Queue group:
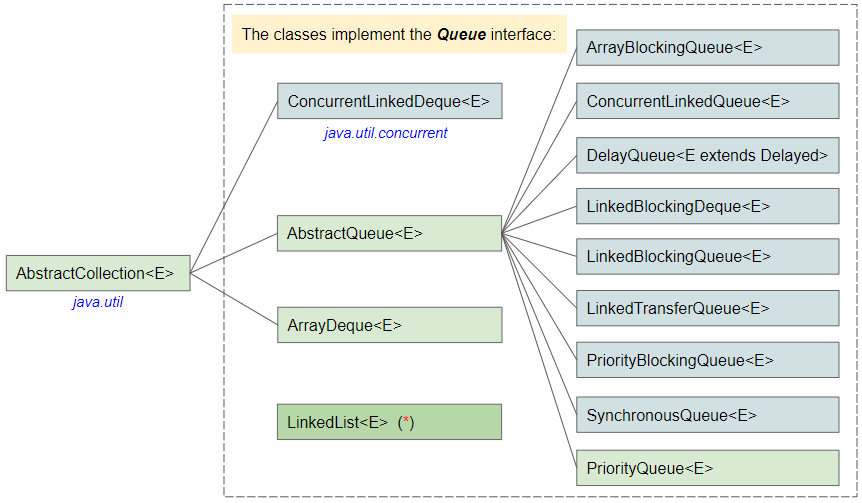
Relationship between interfaces and classes in Queue group:
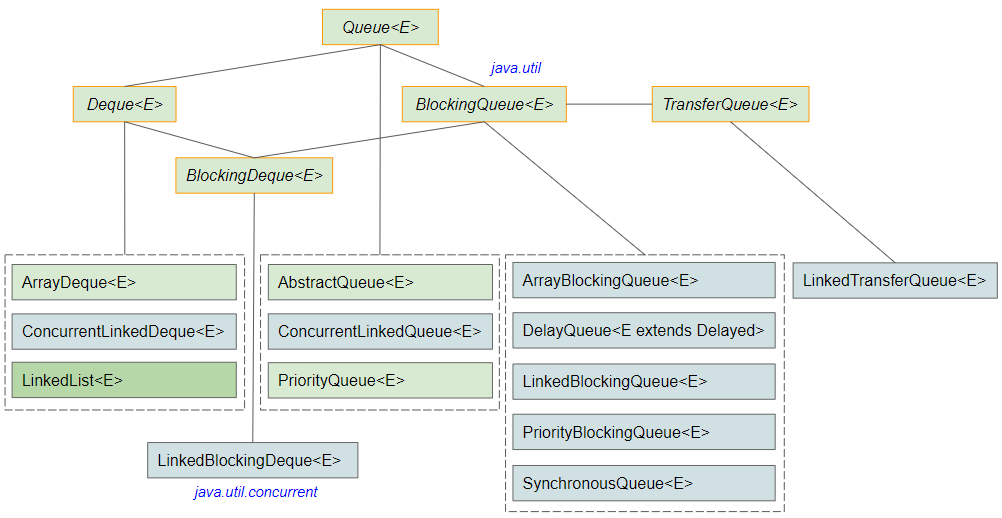
- LinkedList
- ArrayDeque
- PriorityQueue
- LinkedBlockingDeque
- ConcurrentLinkedQueue
- LinkedTransferQueue
- ArrayBlockingQueue
- ConcurrentLinkedDeque
- DelayQueue
- LinkedBlockingQueue
- PriorityBlockingQueue
- SynchronousQueue
LinkedList is a special class, which belongs to both List group and Queue group:
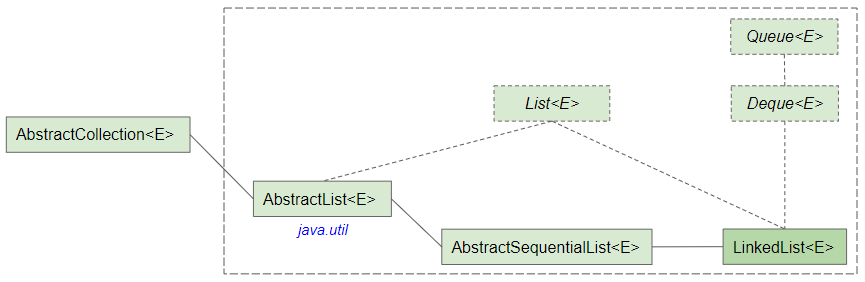
2. Queue methods
In addition to the methods inherited from Collection, Queue also has its own methods:
boolean add(E e);
boolean offer(E e);
E remove();
E poll();
E element();
E peek();
Throws exception | Returns special value | |
Insert | boolean add(E) | boolean offer(E) |
Remove | E remove() | E poll() |
Examine | E element() | E peek() |
boolean add(E)* / boolean offer(E)
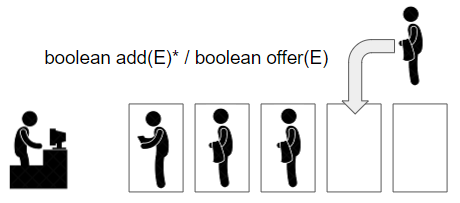
boolean add(E) | Inserts an element into Queue. If there is no more space to insert, this method will throw an exception. The method returns true if the insertion is successful. |
boolean offer(E) | Inserts an element into Queue. If Queue has no more spaces or fails, the method returns false. |
When an element is inserted into Queue, its position is determined by Queue type and the priority of the element. You cannot specify its position.
Depending on the type of Queue you are using, it can limit the number of elements, or increase the size automatically.
E remove()* / E poll()
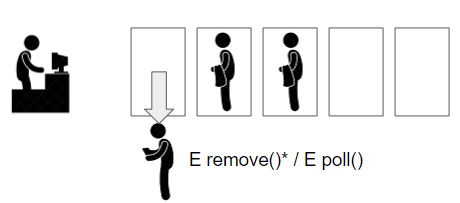
E remove() | Returns the first element of Queue and removes it from Queue. This method throws an exception if Queue has no elements. |
E poll() | Returns the first element of Queue and removes it from Queue. This method returns null if Queue has no elements. |
E element() * / E peek()
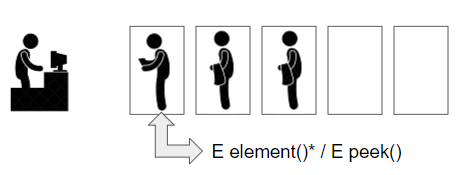
E element() | Returns the first element of Queue but does not remove it from Queue. This method throws an exception if Queue has no elements. |
E peek() | Returns the first element of Queue but does not remove it from Queue. This method returns null if Queue has no elements. |
3. Examples
LinkedList(Queue)
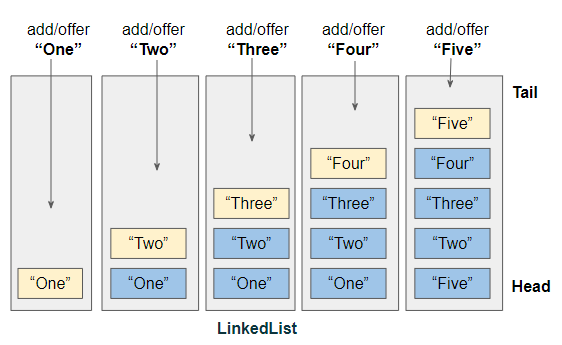
LinkedList represents a traditional queue. add/offer methods add an element to the tail of this queue.
LinkedListEx1.java
package org.o7planning.queue.ex;
import java.util.LinkedList;
import java.util.Queue;
public class LinkedListEx1 {
public static void main(String[] args) {
// Create Queue
Queue<String> queue = new LinkedList<String>();
queue.offer("One");
queue.offer("Two");
queue.offer("Three");
queue.offer("Four");
queue.offer("Five");
String current;
while((current = queue.poll())!= null) {
System.out.println(current);
}
}
}
Output:
One
Two
Three
Four
Five
PriorityQueue
PriorityQueue is a Queue that can automatically sort the elements by their priority order or by a given Comparator. This means that an element inserted into PriorityQueue may not be in the last position.
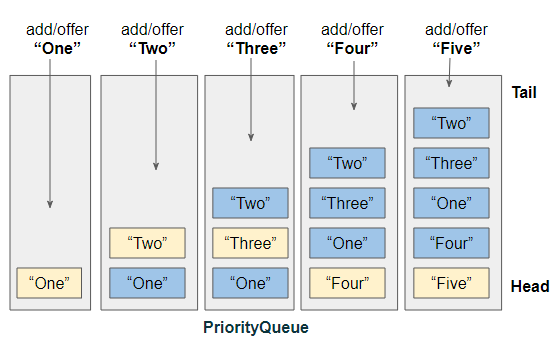
For example, a PriorityQueue<String> sorts the elements alphabetically.
PriorityQueueEx1.java
package org.o7planning.queue.ex;
import java.util.PriorityQueue;
import java.util.Queue;
public class PriorityQueueEx1 {
public static void main(String[] args) {
// Create Queue
Queue<String> queue = new PriorityQueue<String>();
queue.offer("One");
queue.offer("Two");
queue.offer("Three");
queue.offer("Four");
queue.offer("Five");
String current;
while((current = queue.poll())!= null) {
System.out.println(current);
}
}
}
Output:
Five
Four
One
Three
Two
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More