- Map
- Examples
- Null Key/Value
- Map Methods
- of(..), ofEntries(..), copyOf(..)
- put(K key, V value)
- putIfAbsent(K key, V value)
- putAll(Map)
- get(Object key)
- getOrDefault(Object key, V defaultValue)
- containsKey(Object key)
- containsValue(Object value)
- remove(Object key)
- remove(Object key, Object value)
- clear()
- forEach(BiConsumer)
- keySet()
- entrySet()
- values()
- size()
- replace(K key, V value)
- replace(K key, V oldValue, V newValue)
- replaceAll(BiFunction)
- merge(K key, V value, BiFunction)
- compute(K key, BiFunction)
- computeIfAbsent(K key, Function)
- computeIfPresent(K key, BiFunction)
Java Map Tutorial with Examples
1. Map
In Java, Map is an interface that represents an object that contains mappings between keys and values. Each key corresponds to a value. One of the typical examples can be mentioned here is a phone book, the phone number is the key and the person's name is the value. Each key will correspond to only one value.
Hierarchy of interfaces of the Map group:
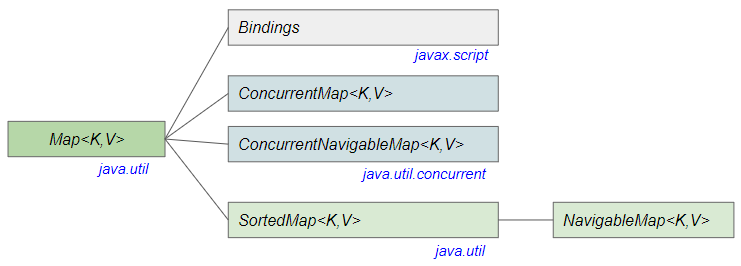
- SortedMap
- NavigableMap
- ConcurrentMap
- ConcurrentNavigableMap
Hierarchy of classes in Map group:
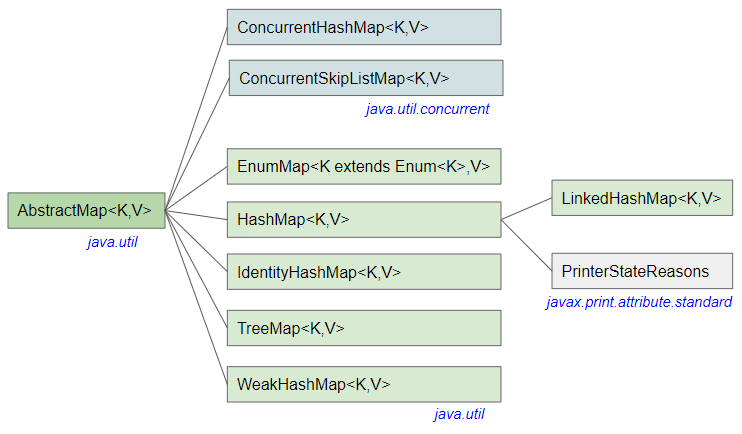
Relationship between interfaces and classes in the Map group:
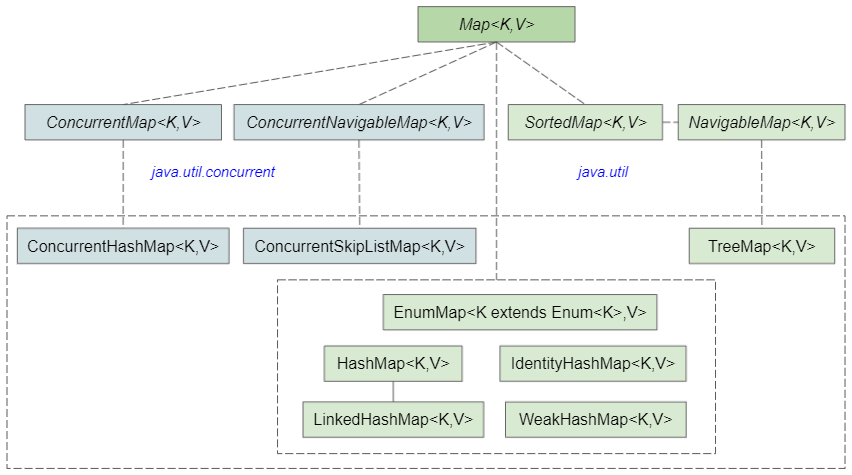
- ConcurrentMap
- ConcurrentNavigableMap
- SortedMap
- NavigableMap
- ConcurrentHashMap
- ConcurrentSkipListMap
- TreeMap
- EnumMap
- HashMap
- LinkedHashMap
- IdentityHashMap
- WeakHashMap
2. Examples
Since Map is an interface, you can only create it from one of the subclasses. In this example we will create a Map object simulating a phone book.
MapEx1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MapEx1 {
public static void main(String[] args) {
Map<String, String> phonebook = new HashMap<String, String>();
phonebook.put("01000005", "Tom");
phonebook.put("01000002", "Jerry");
phonebook.put("01000003", "Tom");
phonebook.put("01000004", "Donald");
Set<String> phoneNumbers = phonebook.keySet();
for (String phoneNumber : phoneNumbers) {
String name = phonebook.get(phoneNumber);
System.out.println("Phone Number: " + phoneNumber + " ==> Name: " + name);
}
}
}
Output:
Phone Number: 01000004 ==> Name: Donald
Phone Number: 01000003 ==> Name: Tom
Phone Number: 01000005 ==> Name: Tom
Phone Number: 01000002 ==> Name: Jerry
Continue with the above example, you can access Map data through a collection of Map.Entry(s):
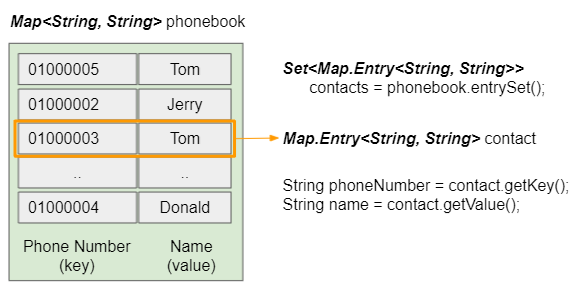
MapEx2.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MapEx2 {
public static void main(String[] args) {
Map<String, String> phonebook = new HashMap<String, String>();
phonebook.put("01000005", "Tom");
phonebook.put("01000002", "Jerry");
phonebook.put("01000003", "Tom");
phonebook.put("01000004", "Donald");
Set<Map.Entry<String,String>> contacts = phonebook.entrySet();
for (Map.Entry<String,String> contact: contacts) {
String phoneNumber = contact.getKey();
String name = contact.getValue();
System.out.println("Phone Number: " + phoneNumber + " ==> Name: " + name);
}
}
}
Output:
Phone Number: 01000004 ==> Name: Donald
Phone Number: 01000003 ==> Name: Tom
Phone Number: 01000005 ==> Name: Tom
Phone Number: 01000002 ==> Name: Jerry
3. Null Key/Value
Map may allow null key, this depend on the class that implements it, such as the HashMap class:
MapEx3.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class MapEx3 {
public static void main(String[] args) {
// String employeeNumber, int salary
Map<String, Integer> mapSalary = new HashMap<String, Integer>();
mapSalary.put("E001", 1200);
mapSalary.put("E002", 2000);
mapSalary.put(null, 1000); // Base salary
int value = mapSalary.get(null);
System.out.println(value); // 1000
}
}
Output:
1000
Map can allow a mapping with null value. This depends on the class implementing the Map interface.
MapEx3.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class MapEx3 {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<String, Integer>();
map.put("AA", null);
Integer value = map.get("AA");
System.out.println("AA ==> " + value);
boolean test = map.containsKey("AA");
System.out.println("Contains AA? " + test); // true
test = map.containsKey("BB");
System.out.println("Contains BB? " + test); // false
}
}
Output:
AA ==> null
Contains AA? true
Contains BB? false
4. Map Methods
Methods of the Map interface:
void clear()
boolean containsKey(Object key)
boolean containsValue(Object value)
boolean isEmpty()
Set<Map.Entry<K,V>> entrySet()
boolean equals(Object o)
V get(Object key)
Set<K> keySet()
Collection<V> values()
V remove(Object key)
V put(K key, V value)
void putAll(Map<? extends K,? extends V> m)
int size()
int hashCode()
static <K,V> Map<K,V> of()
static <K,V> Map<K,V> of(K k1, V v1)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8, K k9, V v9)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8, K k9, V v9, K k10, V v10)
static <K,V> Map<K,V> ofEntries(Map.Entry<? extends K,? extends V>... entries)
static <K,V> Map<K,V> copyOf(Map<? extends K,? extends V> map)
static <K,V> Map.Entry<K,V> entry(K k, V v)
default V putIfAbsent(K key, V value)
default boolean remove(Object key, Object value)
default V replace(K key, V value)
default boolean replace(K key, V oldValue, V newValue)
default void replaceAll(BiFunction<? super K,? super V,? extends V> function)
default V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction)
default V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction)
default V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction)
default V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction)
default void forEach(BiConsumer<? super K,? super V> action)
default V getOrDefault(Object key, V defaultValue)
Map interface has a few optional methods that may not be supported in the class that implements this interface. If you use them you will get UnsupportedOperationException.
void clear()
V put(K key, V value)
void putAll(Map<? extends K,? extends V> m)
V remove(Object key)
5. of(..), ofEntries(..), copyOf(..)
Map.of(..) static method creates an unmodifiable Map object from key and value pairs.
static <K,V> Map<K,V> of()
static <K,V> Map<K,V> of(K k1, V v1)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8, K k9, V v9)
static <K,V> Map<K,V> of(K k1, V v1, K k2, V v2, K k3, V v3, K k4, V v4, K k5, V v5, K k6, V v6, K k7, V v7, K k8, V v8, K k9, V v9, K k10, V v10)
Map.ofEntry(..) static method creates an unmodifiable Map object from the given Map.Entry objects.
static <K,V> Map<K,V> ofEntries(Map.Entry<? extends K,? extends V>... entries)
Map.copyOf(..) static method creates an unmodifiable Map object from a given Map object.
static <K,V> Map<K,V> copyOf(Map<? extends K,? extends V> map)
The Map.of, Map.ofEntries, and Map.copyOf static factory methods provide a convenient way to create unmodifiable Map(s). The Map instances created by these methods have the following characteristics:
- They are unmodifiable. Keys and values cannot be added, removed, or updated. Calling any mutator method on the Map will always cause UnsupportedOperationException to be thrown. However, if the contained keys or values are themselves mutable, this may cause the Map to behave inconsistently or its contents to appear to change.
- They disallow null keys and values. Attempts to create them with null keys or values result in NullPointerException.
- They are serializable if all keys and values are serializable.
- Do not allow duplicate keys in input parameters. Otherwise IllegalArgumentException will be thrown.
- The iteration order of mappings is unspecified and is subject to change.
Map.of Example:
Map_of_ex1.java
package org.o7planning.map.ex;
import java.util.Map;
public class Map_of_ex1 {
public static void main(String[] args) {
// Create an unmodifiable Map:
Map<String, Integer> empMap = Map.of("E01", 1000, "E02", 2000, "E03", 1200);
empMap.forEach((empNumber, salary) -> {
System.out.println("Emp Number: " + empNumber + ", Salary: " + salary);
});
}
}
Output:
Emp Number: E03, Salary: 1200
Emp Number: E02, Salary: 2000
Emp Number: E01, Salary: 1000
Map.ofEntries Example:
Map_ofEntry_ex1.java
package org.o7planning.map.ex;
import java.util.Map;
public class Map_ofEntry_ex1 {
public static void main(String[] args) {
Map.Entry<String, Integer> entry1 = Map.entry("E01", 1000);
Map.Entry<String, Integer> entry2 = Map.entry("E02", 2000);
Map.Entry<String, Integer> entry3 = Map.entry("E03", 1200);
Map<String, Integer> unmodifiableMap = Map.ofEntries(entry1, entry2, entry3);
unmodifiableMap.forEach((empNumber, salary) -> {
System.out.println("Emp Number: " + empNumber + ", Salary: " + salary);
});
}
}
Output:
Emp Number: E02, Salary: 2000
Emp Number: E03, Salary: 1200
Emp Number: E01, Salary: 1000
Map.copyOf Example:
Map_copyOf_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_copyOf_ex1 {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("E01", 1000);
map.put("E02", 2000);
map.put("E03", 1200);
// Unmodifiable Map
Map<String, Integer> unmodifiableMap = Map.copyOf(map);
unmodifiableMap.forEach((empNumber, salary) -> {
System.out.println("Emp Number: " + empNumber + ", Salary: " + salary);
});
}
}
Example: Map object created by Map.of, Map.ofEntries and Map.copyOf static methods is unmodifiable. It does not support any operations to modify its data. For example put, clear, ...
Map_of_ex2.java
package org.o7planning.map.ex;
import java.util.Map;
public class Map_of_ex2 {
public static void main(String[] args) {
// Create an unmodifiable Map:
Map<String, Integer> empMap = Map.of("E01", 1000, "E02", 2000, "E03", 1200);
// Update new value.
empMap.put("E02", 5000); // ==> throw UnsupportedOperationException
empMap.put("E05", 3000); // ==> throw UnsupportedOperationException
empMap.remove("E01"); // ==> throw UnsupportedOperationException
empMap.clear(); // ==> throw UnsupportedOperationException
}
}
6. put(K key, V value)
public V put(K key, V value) // Optional Operation
Add a mapping (key, value) to Map if key does not exist and return null. Otherwise, the new value will be replaced for the old value corresponding to key, and return the old value.
7. putIfAbsent(K key, V value)
default V putIfAbsent(K key, V value) {
V v = get(key);
if (v == null) {
v = put(key, value);
}
return v;
}
This method is similar to put(key,value) method but it only works if the key does not exist in the Map or it is mapping to a null value. Method returns null or old value.
8. putAll(Map)
public void putAll(Map<? extends K,? extends V> m)
Copy all mappings from the specified Map object to the current Map object.
9. get(Object key)
public V get(Object key)
Returns the value of the mapping corresponding to key, or null if no such mapping exists.
10. getOrDefault(Object key, V defaultValue)
public default V getOrDefault(Object key, V defaultValue) // Java 8
Returns the value of the mapping corresponding to the key, or defaultValue if no such mapping exists.
Example:
Map_getOrDefault_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_getOrDefault_ex1 {
public static void main(String[] args) {
Map<String, String> appConfigs = new HashMap<String, String>();
appConfigs.put("INPUT_DIRECTORY", "C:/App/input");
appConfigs.put("IMAGE_DIRECTORY", "C:/App/image");
String outputDir = appConfigs.getOrDefault("OUTPUT_DIRECTORY", "C:/App/output");
System.out.println("App Output Directory: " + outputDir);
}
}
Output:
App Output Directory: C:/App/output
11. containsKey(Object key)
public boolean containsKey(Object key)
Returns true if this Map contains a mapping for the specified key.
12. containsValue(Object value)
public boolean containsValue(Object value)
Returns true if this Map has at least one mapping to the specified value.
Example: A Map contains mappings between brand name and country, check whether or not a brand is from a certain country.
Map_containsValue_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_containsValue_ex1 {
public static void main(String[] args) {
// String brand ==> String country
Map<String, String> brandMap = new HashMap<String, String>();
brandMap.put("SamSung", "Korea");
brandMap.put("LG", "Korea");
brandMap.put("Alibaba", "China");
brandMap.put("Toyota", "Japan");
brandMap.put("Hyundai", "Korea");
brandMap.put("Vinfast", "Vietnam"); // value = "Vietnam"!
brandMap.put("Honda", "Japan");
brandMap.put("Huwei", "China");
boolean contain = brandMap.containsValue("Vietnam");
System.out.println("Does the Map contain 'Vietnam' value? " + contain);
contain = brandMap.containsValue("Thailand");
System.out.println("Does the Map contain 'Thailand' value? " + contain);
}
}
Output:
Does the Map contain 'Vietnam' value? true
Does the Map contain 'Thailand' value? false
13. remove(Object key)
public V remove(Object key) // Optional Operation
Remove the mapping corresponding to the key from the Map. Returns value of the mapping, or null if the mapping does not exist.
14. remove(Object key, Object value)
public default boolean remove(Object key, Object value) {
if (this.containsKey(key) && Objects.equals(this.get(key), value)) {
this.remove(key);
return true;
} else {
return false;
}
}
Removes the mapping (key,value) from the Map, and returns true if it actually exists, otherwise returns false.
15. clear()
public void clear() // Optional operation.
Removes all of the mappings from this Map. The Map will be empty after the method is called.
16. forEach(BiConsumer)
public default void forEach(BiConsumer<? super K,? super V> action)
Performs a specified action on each pair (key,value) of the Map until all pairs are handled or an exception occurs.
Example: A Map object contains mappings between country name and population.
Map_forEach_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_forEach_ex1 {
public static void main(String[] args) {
// Data in 2021.
// String country ==> Integer population (Million)
Map<String, Integer> populationMap = new HashMap<String, Integer>();
populationMap.put("Vietnam", 98);
populationMap.put("Phillipine", 109);
populationMap.put("United States", 330);
populationMap.put("Indonesia", 273);
populationMap.put("Russia", 145);
// forEach(BiConsumer):
populationMap.forEach((country, population) -> System.out.println(country + " --> " + population));
}
}
Output:
Vietnam --> 98
United States --> 330
Phillipine --> 109
Indonesia --> 273
Russia --> 145
17. keySet()
public Set<K> keySet()
Returns a view as a Set containing all Map keys. The Set object and the current Map object are related. Changes on the Map will affect the Set and vice versa.
- Adding or removing a mapping from Map will add or remove an element from Set.
- Methods that remove elements from Set such as Set.iterator().remove, Set.remove, Set.removeAll, Set.retainAll, Set.clear .. will remove the corresponding mappings from Map.
- This Set object does not support the Set.add, Set.addAll operations.
Example: A Map object contains mappings between country name and population.
Map_keySet_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class Map_keySet_ex1 {
public static void main(String[] args) {
// Data in 2021.
// String country ==> Integer population (Million)
Map<String, Integer> populationMap = new HashMap<String, Integer>();
populationMap.put("Vietnam", 98);
populationMap.put("Phillipine", 109);
populationMap.put("United States", 330);
populationMap.put("Indonesia", 273);
populationMap.put("Russia", 145);
Set<String> countrySet = populationMap.keySet();
for(String country: countrySet) {
System.out.println(country);
}
}
}
Output:
Vietnam
United States
Phillipine
Indonesia
Russia
Map_keySet_ex2.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class Map_keySet_ex2 {
public static void main(String[] args) {
// Data in 2021.
// String country ==> Integer population (Million)
Map<String, Integer> populationMap = new HashMap<String, Integer>();
populationMap.put("Vietnam", 98);
populationMap.put("Phillipine", 109);
populationMap.put("United States", 330);
populationMap.put("Indonesia", 273);
populationMap.put("Russia", 145);
Set<String> countrySet = populationMap.keySet();
System.out.println("--- Countries: ---");
for(String country: countrySet) {
System.out.println(country);
}
//
countrySet.remove("Phillipine");
countrySet.remove("Indonesia");
System.out.println();
System.out.println("--- Map (After removal): ---");
populationMap.forEach((country, population) -> {
System.out.println(country +" --> " + population);
});
}
}
Output:
--- Countries: ---
Vietnam
United States
Phillipine
Indonesia
Russia
--- Map (After removal): ---
Vietnam --> 98
United States --> 330
Russia --> 145
18. entrySet()
public Set<Map.Entry<K,V>> entrySet()
Returns a view as a Set containing all Map.Entry elements of the Map. The Set object and the current Map object are related. Changes on the Map will affect the Set and vice versa.
- Adding or removing a mapping from Map will add or remove an element from Set.
- Methods that remove elements from Set such as Set.iterator().remove, Set.remove, Set.removeAll, Set.retainAll, Set.clear .. will remove the corresponding mappings from Map.
- This Set object does not support the Set.add, Set.addAll operations.
Example: A Map object contains mappings between country name and population.
Map_entrySet_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class Map_entrySet_ex1 {
public static void main(String[] args) {
// Data in 2021.
// String country ==> Integer population (Million)
Map<String, Integer> populationMap = new HashMap<String, Integer>();
populationMap.put("Vietnam", 98);
populationMap.put("Phillipine", 109);
populationMap.put("United States", 330);
populationMap.put("Indonesia", 273);
populationMap.put("Russia", 145);
Set<Map.Entry<String,Integer>> set = populationMap.entrySet();
for(Map.Entry<String,Integer> entry: set) {
System.out.println(entry.getKey() +" --> " + entry.getValue());
}
}
}
Output:
Vietnam --> 98
United States --> 330
Phillipine --> 109
Indonesia --> 273
Russia --> 145
Continue with the above example, we remove countries with population greater than 200 (Million) from the Map object.
Map_entrySet_ex2.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
public class Map_entrySet_ex2 {
public static void main(String[] args) {
// Data in 2021.
// String country ==> Integer population (Million)
Map<String, Integer> populationMap = new HashMap<String, Integer>();
populationMap.put("Vietnam", 98);
populationMap.put("Phillipine", 109);
populationMap.put("United States", 330);
populationMap.put("Indonesia", 273);
populationMap.put("Russia", 145);
Set<Map.Entry<String,Integer>> set = populationMap.entrySet();
Iterator<Map.Entry<String,Integer>> iterator = set.iterator();
// Remove the Countries with population greater than 200 Million.
while(iterator.hasNext()) {
Map.Entry<String,Integer> entry = iterator.next();
if(entry.getValue() > 200) {
iterator.remove();
}
}
// After removal.
// forEach(Consumer)
set.forEach(entry -> {
System.out.println(entry.getKey() +" --> " + entry.getValue());
});
}
}
Output:
Vietnam --> 98
Phillipine --> 109
Russia --> 145
19. values()
public Collection<V> values()
Returns a view as Collection containing all Map values. Collection object and current Map object are related to each other. Changes on Map will affect Collection and vice versa.
- Adding or removing a map from Map will add or remove an element from Collection.
- Modify the value of a mapping will affect Collection.
- Methods that remove elements from Collection such as Collection.iterator().remove, Collection.remove, Collection.removeAll, Collection.retainAll, Collection.clear .. will remove the corresponding mappings from the Map.
- This Collection object does not support Collection.add, Collection.addAll operations.
Example: A Map object contains mappings between brand name and country. Print out the values of the Map object.
Map_values_ex1.java
package org.o7planning.map.ex;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
public class Map_values_ex1 {
public static void main(String[] args) {
// String brand ==> String country
Map<String, String> brandMap = new HashMap<String, String>();
brandMap.put("SamSung", "Korea");
brandMap.put("LG", "Korea");
brandMap.put("Alibaba", "China");
brandMap.put("Toyota", "Japan");
brandMap.put("Hyundai", "Korea");
brandMap.put("Vinfast", "Vietnam");
brandMap.put("Honda", "Japan");
brandMap.put("Huwei", "China");
Collection<String> countries = brandMap.values();
for(String country: countries) {
System.out.println(country);
}
}
}
Output:
China
Japan
Vietnam
Korea
China
Korea
Korea
Japan
Continue with the above example, remove those brands from China from the Map object:
Map_values_ex2.java
package org.o7planning.map.ex;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class Map_values_ex2 {
public static void main(String[] args) {
// String brand ==> String country
Map<String, String> brandMap = new HashMap<String, String>();
brandMap.put("SamSung", "Korea");
brandMap.put("LG", "Korea");
brandMap.put("Alibaba", "China");
brandMap.put("Toyota", "Japan");
brandMap.put("Hyundai", "Korea");
brandMap.put("Vinfast", "Vietnam");
brandMap.put("Honda", "Japan");
brandMap.put("Huwei", "China");
Collection<String> countries = brandMap.values();
Iterator<String> countryIte = countries.iterator();
String country;
while(countryIte.hasNext()) {
country = countryIte.next();
if("China".equals(country)) {
countryIte.remove();
}
}
// After remove
for(String brand: brandMap.keySet()) {
System.out.println(brand +" : " + brandMap.get(brand));
}
}
}
Output:
Toyota : Japan
Vinfast : Vietnam
SamSung : Korea
LG : Korea
Hyundai : Korea
Honda : Japan
20. size()
public int size()
Returns the number of mappings in this Map. If the Map contains more than Integer.MAX_VALUE elements, returns Integer.MAX_VALUE.
21. replace(K key, V value)
public default V replace(K key, V value)
If there is a mapping with key key in Map, it will be replaced by the mapping (key,value) and return the old value. Otherwise no action is taken and null is returned.
Example:
Map_replace_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_replace_ex1 {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<String, Integer>();
map.put("A", 1200);
map.put("B", null);
map.put("C", 1500);
// key does not exists, no replace, return null
Integer oldValue1 = map.replace("A1", 3000);
// key exists!, replace!, return null (old value).
Integer oldValue2 = map.replace("B", 5000);
// key exists!, replace!, return 1500 (old value).
Integer oldValue3 = map.replace("C", 2000);
System.out.println("oldValue1: " + oldValue1); // null
System.out.println("oldValue2: " + oldValue2); // null (old value).
System.out.println("oldValue3: " + oldValue3); // 1500 (old value).
System.out.println();
for (String key : map.keySet()) {
System.out.println(key + " ==> " + map.get(key));
}
}
}
Output:
oldValue1: null
oldValue2: null
oldValue3: 1500
A ==> 1200
B ==> 5000
C ==> 2000
22. replace(K key, V oldValue, V newValue)
public default boolean replace(K key, V oldValue, V newValue)
If mapping (key, oldValue) actually exists in Map, it will be replaced by mapping (key,newValue) and return true. Otherwise no action is taken and return false.
23. replaceAll(BiFunction)
public default void replaceAll(BiFunction<? super K,? super V,? extends V> function)
Use function given by the parameter to replace value for all mapping in the Map. Calling the above method would be equivalent to executing the following code:
for (Map.Entry<K, V> entry : map.entrySet()) {
entry.setValue(function.apply(entry.getKey(), entry.getValue()));
}
Example: A Map object contains mappings between Employee Number and salary. Use the replaceAll method to increase salary by 50% per employee.
Map_replaceAll_ex1.java
package org.o7planning.map.ex;
import java.util.HashMap;
import java.util.Map;
public class Map_replaceAll_ex1 {
public static void main(String[] args) {
// Create Map (Employee Number --> Salary)
Map<String, Float> empMap = new HashMap<>();
empMap.put("E01", 1000f);
empMap.put("E02", 2000f);
empMap.put("E03", 1200f);
// Salary update for all employees, 50% increase.
empMap.replaceAll((empNumber, salary) -> {
return salary + salary * 0.5f;
});
// Print out:
empMap.forEach((empNumber, salary) -> {
System.out.println(empNumber + " --> " + salary);
});
}
}
Output:
E02 --> 3000.0
E01 --> 1500.0
E03 --> 1800.0
24. merge(K key, V value, BiFunction)
default V merge(K key, V value,
BiFunction<? super V, ? super V, ? extends V> remappingFunction) {
Objects.requireNonNull(remappingFunction);
Objects.requireNonNull(value);
V oldValue = get(key);
V newValue = (oldValue == null) ? value :
remappingFunction.apply(oldValue, value);
if (newValue == null) {
remove(key);
} else {
put(key, newValue);
}
return newValue;
}
25. compute(K key, BiFunction)
default V compute(K key,
BiFunction<? super K, ? super V, ? extends V> remappingFunction) {
Objects.requireNonNull(remappingFunction);
V oldValue = get(key);
V newValue = remappingFunction.apply(key, oldValue);
if (newValue == null) {
// delete mapping
if (oldValue != null || containsKey(key)) {
// something to remove
remove(key);
return null;
} else {
// nothing to do. Leave things as they were.
return null;
}
} else {
// add or replace old mapping
put(key, newValue);
return newValue;
}
}
26. computeIfAbsent(K key, Function)
default V computeIfAbsent(K key,
Function<? super K, ? extends V> mappingFunction) {
Objects.requireNonNull(mappingFunction);
V v;
if ((v = get(key)) == null) {
V newValue;
if ((newValue = mappingFunction.apply(key)) != null) {
put(key, newValue);
return newValue;
}
}
return v;
}
27. computeIfPresent(K key, BiFunction)
default V computeIfPresent(K key,
BiFunction<? super K, ? super V, ? extends V> remappingFunction) {
Objects.requireNonNull(remappingFunction);
V oldValue;
if ((oldValue = get(key)) != null) {
V newValue = remappingFunction.apply(key, oldValue);
if (newValue != null) {
put(key, newValue);
return newValue;
} else {
remove(key);
return null;
}
} else {
return null;
}
}
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More