Java Deque Tutorial with Examples
1. Deque
Deque stand for "Double Ended Queue". Like people lining up at the supermarket, only the first and the last person in the queue are served. Deque offers a bit more flexibility than a regular queue.
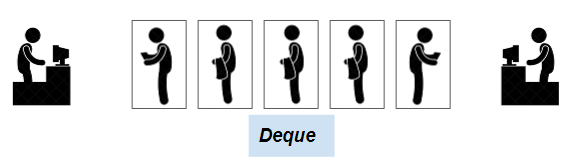
public interface Deque<E> extends Queue<E>
Deque is a subinterface of Queue, providing methods to insert an element in the beginning or end, and methods to access or remove its first or last element.
Deque also includes methods that allow it to act as a stack (See more explanation in the article).
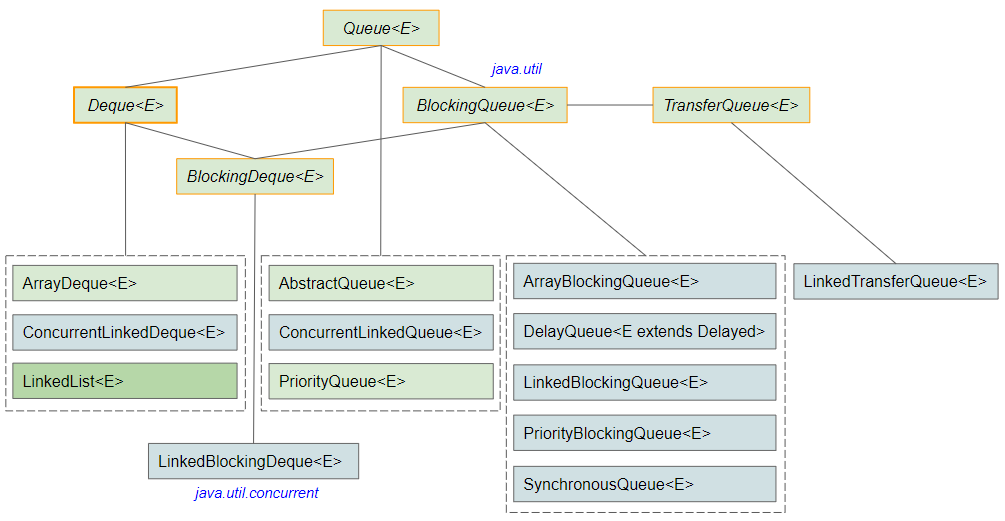
- Queue
- BlockingDeque
- BlockingQueue
- TransferQueue
- ArrayDeque
- ConcurrentLinkedDeque
- ConcurrentLinkedQueue
- LinkedList
- PriorityQueue
- ArrayBlockingQueue
- DelayQueue
- LinkedBlockingQueue
- LinkedBlockingDeque
- PriorityBlockingQueue
- SynchronousQueue
- LinkedTransferQueue
2. Deque methods
Methods of Deque
void addFirst(E e);
void addLast(E e);
boolean offerFirst(E e);
boolean offerLast(E e);
E removeFirst();
E removeLast();
E pollFirst();
E pollLast();
E getFirst();
E getLast();
E peekFirst();
E peekLast();
void push(E e);
E pop();
boolean removeFirstOccurrence(Object o);
boolean removeLastOccurrence(Object o);
Iterator<E> descendingIterator();
// Methods inherited from Queue:
boolean add(E e);
boolean offer(E e);
E remove();
E poll();
E element();
E peek();
// Methods inherited from Collection:
boolean addAll(Collection<? extends E> c);
boolean remove(Object o);
boolean contains(Object o);
int size();
Iterator<E> iterator();
...
List of 12 characteristic methods of Deque:
Insert | addFirst(E) | offerFirst(E) | addLast(E) | offerLast(E) |
Remove | removeFirst() | pollFirst() | removeLast() | pollLast() |
Examine | getFirst() | peekFirst() | getLast() | peekLast() |
boolean addFirst(E) / boolean offerFirst(E)
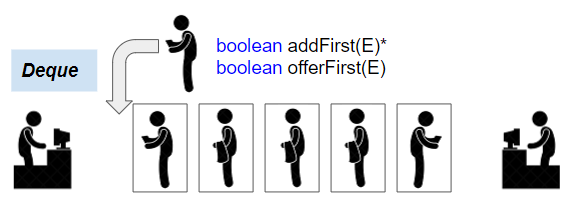
boolean addFirst(E) | Inserts an element in front of Deque. This method will throw an exception if Deque's capacity is full. The method returns true if the insertion was successfull. |
boolean offerFirst(E) | Inserts an element in front of Deque. If Deque capacity is full or the insert fails, the method will return false, otherwise true. |
Note: Depending on the Deque type, it can limit (or not limit) the number of elements.
boolean addLast(E) / boolean offerLast(E)
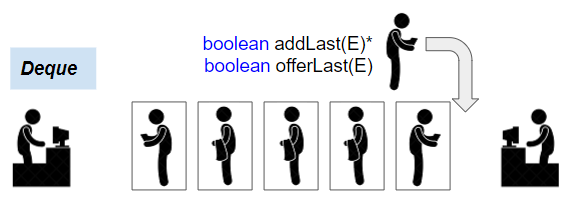
boolean addLast(E) | Inserts an element at the end of Deque. This method will throw an exception if Deque's capacity is full. The method returns true if insertion was successful. |
boolean offerLast(E) | Inserts an element at the end of Deque. If Deque capacity is full or the insert fails, the method will return false, otherwise true. |
E removeFirst() / E pollFirst()
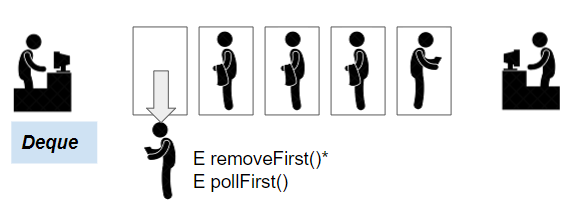
E removeFirst() | Returns the first element of Deque and removes it from Deque. This method will throw an exception if Deque has no elements. |
E pollFirst() | Returns the first element of Deque and removes it from Deque. This method will return null if Deque has no elements. |
E removeLast() / E pollLast()
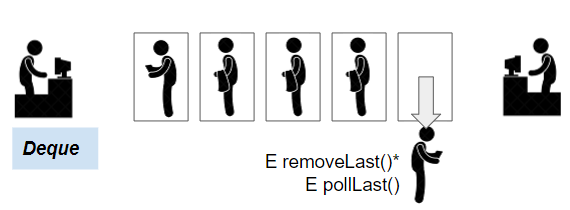
E removeLast() | Returns the last element of Deque and removes it from Deque. This method will throw an exception if Deque has no elements. |
E pollLast() | Returns the last element of Deque and removes it from Deque. This method will return null if Deque has no elements. |
E getFirst() / E peekFirst()
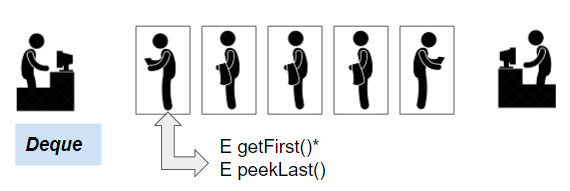
E getFirst() | Returns the first element of Deque but does not remove it from Deque. This method throws an exception if Deque has no elements. |
E peekFirst() | Returns the first element of Deque but does not remove it from Deque. This method returns null if Deque has no elements. |
E getLast() / E peekLast()
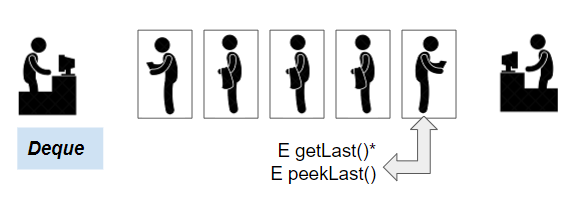
E getLast() | Returns the last element of Deque but does not remove it from Deque. This method throws an exception if Deque has no elements. |
E peekLast() | Returns the last element of Deque but does not remove it from Deque. This method returns null if Deque has no elements. |
Methods inherited from Queue:
Inherited methods from Queue and corresponding methods in Deque:
booleanadd(E) | booleanaddLast(E) | (*) |
booleanoffer(E) | booleanofferLast(E) | (*) |
E remove() | E removeFirst() | |
E poll() | E pollFirst() | |
E element() | E getFirst() | |
E peek() | E peekFirst() |
(*) - In most Queue types, add(E) and offer(E) methods insert an element in the end. But this is not true for PriorityQueue, because PriorityQueue specifies the position of the inserted element based on its priority.
Stack?
Deque can act as a stack, as it provides methods to work under the LIFO (Last In First Out) mechanism.
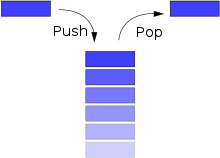
boolean push(e) | boolean addFirst(e) |
E pop() | E removeFirst() |
E peek() | E getFirst() |
3. Examples
Deque is an interface, so to create a Deque object, you must use one of its subclasses, such as ArrayDeque, ConcurrentLinkedDeque, LinkedList, LinkedBlockingDeque.
Deque<String> deque = new ArrayDeque<>();
Deque<String> deque = new LinkedList<>();
Customer class will participate in the examples.
Customer.java
package com.o7planning.deque.ex;
public class Customer {
private Integer cusId;
private String cusName;
public Customer(Integer cusId, String cusName) {
super();
this.cusId = cusId;
this.cusName = cusName;
}
public Integer getCusId() {
return cusId;
}
public String getCusName() {
return cusName;
}
}
DequeEx1.java
package com.o7planning.deque.ex;
import java.util.ArrayDeque;
import java.util.Deque;
public class DequeEx1 {
public static void main(String[] args) {
// Create a Deque with maximum capacity of 10 elements.
Deque<Customer> deque = new ArrayDeque<Customer>(10);
deque.addFirst(new Customer(1, "Tom"));
deque.addFirst(new Customer(2, "Jerry"));
deque.addLast(new Customer(3, "Donald"));
Customer current = null;
// Retrieve first element and remove it from Deque.
while((current = deque.pollFirst())!= null) {
System.out.println("Serving customer: " + current.getCusName());
}
}
}
Output:
Serving customer: Jerry
Serving customer: Tom
Serving customer: Donald
Deque can be used as a stack. See an example simulating cards:
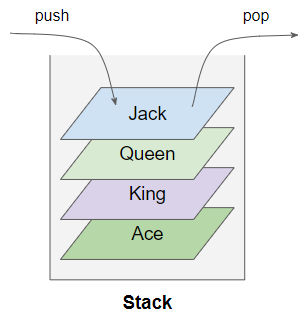
DequeStackEx1.java
package com.o7planning.deque.ex;
import java.util.Deque;
import java.util.LinkedList;
public class DequeStackEx1 {
public static void main(String[] args) {
// Create a Deque to use as a Stack.
Deque<String> stackOfCards = new LinkedList<>();
// Pushing new items to the Stack
stackOfCards.push("Jack");
stackOfCards.push("Queen");
stackOfCards.push("King");
stackOfCards.push("Ace");
System.out.println("Stack => " + stackOfCards);
System.out.println();
// Popping items from the Stack
String cardAtTop = stackOfCards.pop(); // Throws NoSuchElementException if the stack is empty
System.out.println("Stack.pop() => " + cardAtTop);
System.out.println("Current Stack => " + stackOfCards);
System.out.println();
// Get the item at the top of the stack without removing it
cardAtTop = stackOfCards.peek();
System.out.println("Stack.peek() => " + cardAtTop);
System.out.println("Current Stack => " + stackOfCards);
}
}
Output:
Stack => [Ace, King, Queen, Jack]
Stack.pop() => Ace
Current Stack => [King, Queen, Jack]
Stack.peek() => King
Current Stack => [King, Queen, Jack]
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More