Java ListIterator Tutorial with Examples
1. ListIterator
ListIterator is a sub interface of Iterator. It is one of the ways to traverse the elements of a List. Unlike Iterator, ListIterator supports traversing elements in both forward and backward directions. ListIterator also supports removing, updating or inserting an element during iteration.
public interface ListIterator<E> extends Iterator<E>
Here's a comparison between Iterator and ListIterator:
Iterator | ListIterator |
Used to iterate over the elements of a Collection. | Used to iterate over the elements of a List. |
Supports traversing elements in forward direction. | Supports traversing elements in forward and backward directions. |
Can support removing an element during iteration (Depends on Collection type) | Can support removing, updating or inserting an element during iteration (Depends on List type). |
The iteration order of elements is not guaranteed. | The iteration order of elements is guaranteed. |
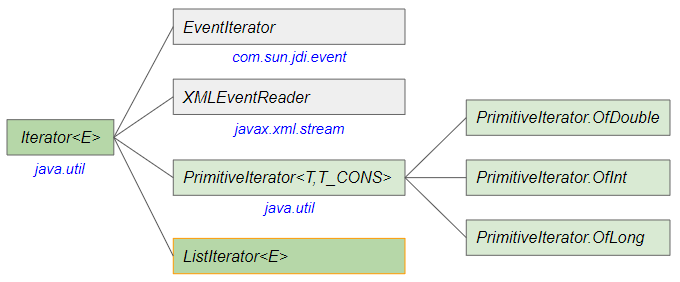
ListIterator methods
boolean hasPrevious()
E previous()
int nextIndex()
int previousIndex()
void set(E e) // Optional operation.
void add(E e) // Optional operation.
// Methods inherited from Iterator:
boolean hasNext()
E next();
default void remove() // Optional operation.
default void forEachRemaining(Consumer<? super E> action)
2. Example
Example: Using ListIterator to traverse the elements of a List:
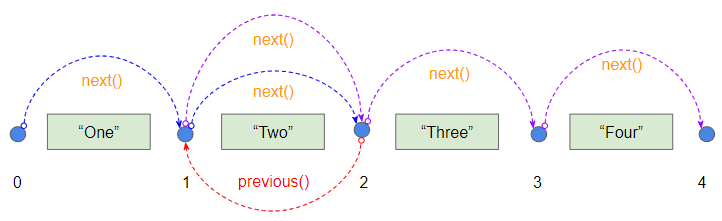
ListIteratorEx1.java
package org.o7planning.listiterator.ex;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListIteratorEx1 {
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
// Create ListIterator.
ListIterator<String> listIterator = list.listIterator();
String first = listIterator.next();
System.out.println("First:" + first);// -->"One"
String second = listIterator.next();
System.out.println("Second:" + second);// -->"Two"
if (listIterator.hasPrevious()) {
// Take a step back
String value = listIterator.previous();
System.out.println("Value:" + value);// -->"Two"
}
System.out.println(" ----- ");
while (listIterator.hasNext()) {
String value = listIterator.next();
System.out.println("value:" + value);
}
}
}
Output:
First:One
Second:Two
Value:Two
-----
value:Two
value:Three
value:Four
3. remove()
While traversing elements of a Collection using an Iterator, you can remove the current element from Collection. Iterator.remove() method allows you to do that. However, not all Iterator(s) support this operation, it depends on the Collection type. If unsupported, UnsupportedOperationException will be thrown.
Note: remove() method is inherited from Iterator.
// Optional operation
// Inherited from Iterator interface
public void remove()
Example: A List contains integers, remove the elements from the list to ensure that the list only includes incrementing integers.
ListIterator_remove_ex1.java
package org.o7planning.listiterator.ex;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListIterator_remove_ex1 {
public static void main(String[] args) {
List<Integer> list= new ArrayList<Integer>();
list.add(11);
list.add(55);
list.add(22);
list.add(77);
list.add(33);
list.add(99);
list.add(11);
ListIterator<Integer> listIter = list.listIterator();
int temp = listIter.next();
while(listIter.hasNext()) {
int current = listIter.next();
if(current < temp) {
listIter.remove();
} else {
temp = current;
}
}
for(Integer value: list) {
System.out.println(value);
}
}
}
Output:
11
55
77
99
4. set(E e)
While traversing elements of a List using an ListIterator, you can update the current element. ListIterator.set(E) method allows you to do that. However, not all ListIterator(s) support this operation, it depends on the List type. If unsupported, UnsupportedOperationException will be thrown.
public void set(E e)
Example: A List contains integers, if an element of list is negative integer, it needs to be replaced by zero.
ListIterator_set_ex1.java
package org.o7planning.listiterator.ex;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListIterator_set_ex1 {
public static void main(String[] args) {
List<Integer> list= new ArrayList<Integer>();
list.add(-111);
list.add(555);
list.add(-222);
list.add(777);
list.add(-333);
list.add(999);
list.add(111);
ListIterator<Integer> listIter = list.listIterator();
while(listIter.hasNext()) {
int current = listIter.next();
if(current < 0) {
listIter.set(0);
}
}
for(Integer value: list) {
System.out.println(value);
}
}
}
Output:
0
555
0
777
0
999
111
An example of a List that doesn't support ListIterator.set operation:
ListIterator_set_ex2.java
package org.o7planning.listiterator.ex;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.ListIterator;
public class ListIterator_set_ex2 {
public static void main(String[] args) {
List<Integer> aList = Arrays.asList(-111, 555,-222,777,-333,999,111);
// UnmodifiableList
// This list does not support "set" operation!
List<Integer> unmodifiableList = Collections.unmodifiableList(aList);
ListIterator<Integer> listIter = unmodifiableList.listIterator();
int current = listIter.next();
listIter.set(1000); // throw UnsupportedOperationException
}
}
Output:
Exception in thread "main" java.lang.UnsupportedOperationException
at java.base/java.util.Collections$UnmodifiableList$1.set(Collections.java:1353)
at org.o7planning.listiterator.ex.ListIterator_set_ex2.main(ListIterator_set_ex2.java:21)
5. add(E e)
While traversing elements of a List using an ListIterator, you can insert an element into List. ListIterator.add(E) method allows you to do that. However, not all ListIterator(s) support this operation, it depends on the List type. If unsupported, UnsupportedOperationException will be thrown.
public void add(E e);
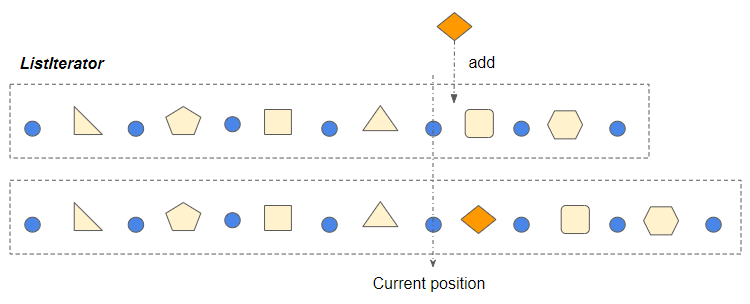
Example:
ListIterator_add_ex1.java
package org.o7planning.listiterator.ex;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListIterator_add_ex1 {
public static void main(String[] args) {
List<String> list= new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
list.add("Five");
ListIterator<String> listIter = list.listIterator();
listIter.next(); // "One"
listIter.next(); // "Two"
listIter.next(); // "Three"
listIter.previous(); // "Three"
listIter.add("X1");
for(String value: list) {
System.out.println(value);
}
}
}
Output:
One
Two
X1
Three
Four
Five
An example of a List that doesn't support ListIterator.add operation:
ListIterator_add_ex2.java
package org.o7planning.listiterator.ex;
import java.util.Arrays;
import java.util.List;
import java.util.ListIterator;
public class ListIterator_add_ex2 {
public static void main(String[] args) {
// Fixed-size List
// It does not support add or set operation.
List<String> list= Arrays.asList("One","Two","Three","Four","Five");
ListIterator<String> listIter = list.listIterator();
listIter.next(); // "One"
listIter.next(); // "Two"
listIter.next(); // "Three"
listIter.previous(); // "Three"
listIter.add("X1"); // throw UnsupportedOperationException
}
}
Output:
Exception in thread "main" java.lang.UnsupportedOperationException
at java.base/java.util.AbstractList.add(AbstractList.java:153)
at java.base/java.util.AbstractList$ListItr.add(AbstractList.java:451)
at org.o7planning.listiterator.ex.ListIterator_add_ex2.main(ListIterator_add_ex2.java:19)
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More