Java LinkedList Tutorial with Examples
1. LinkedList
LinkedList is one of the most special classes in the Java Collection Framework. Here are its characteristics:
- LinkedList can be used as a List, Deque (Double ended Queue) or a stack.
- LinkedList is non synchronized.
- LinkedList allows duplicate elements.
- LinkedList does not allow null elements (Characteristic of a Queue).
- LinkedList maintains elements insertion order.
public class LinkedList<E>
extends AbstractSequentialList<E>
implements List<E>, Deque<E>, Cloneable, java.io.Serializable
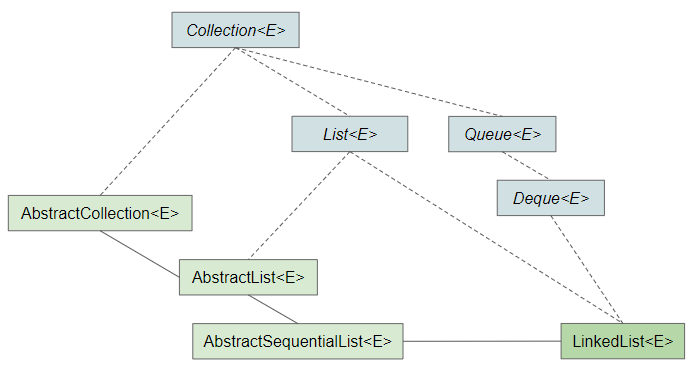
LinkedList constructors
LinkedList()
LinkedList(Collection<? extends E> c)
LinkedList methods
void add(int index, E element)
boolean add(E e)
boolean addAll(int index, Collection<? extends E> c)
boolean addAll(Collection<? extends E> c)
void addFirst(E e)
void addLast(E e)
void clear()
Object clone()
boolean contains(Object o)
Iterator<E> descendingIterator()
E element()
E get(int index)
E getFirst()
E getLast()
int indexOf(Object o)
int lastIndexOf(Object o)
ListIterator<E> listIterator(int index)
boolean offer(E e)
boolean offerFirst(E e)
boolean offerLast(E e)
E peek()
E peekFirst()
E peekLast()
E poll()
E pollFirst()
E pollLast()
E pop()
void push(E e)
E remove()
E remove(int index)
boolean remove(Object o)
E removeFirst()
boolean removeFirstOccurrence(Object o)
E removeLast()
boolean removeLastOccurrence(Object o)
E set(int index, E element)
int size()
Spliterator<E> spliterator()
Object[] toArray()
<T> T[] toArray(T[] a)
2. How does LinkedList store elements?
LinkedList manages elements specifically, overcoming the disadvantages of arrays. In this section we will analyze how LinkedList manages its elements.
Each LinkedList element is wrapped by a Node object. Each Node object has a reference to the Node preceding it and following it. Therefore, we can move from the first to the last LinkedList element.
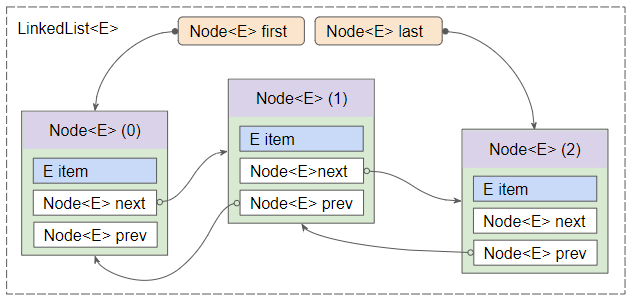
Removing the element from LinkedList is equivalent to removing the corresponding Node. To do that, preceding and following Node(s) simply update their references:
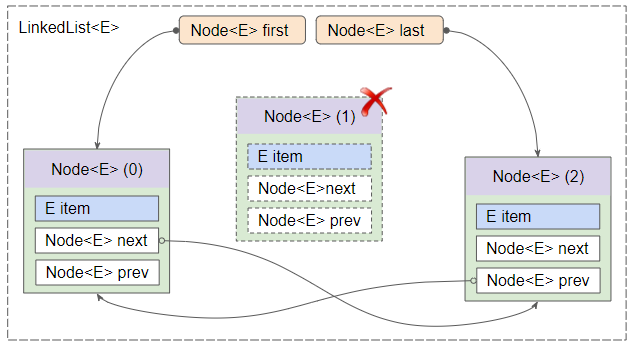
Inserting an element into LinkedList is also done in a simple way as shown below:
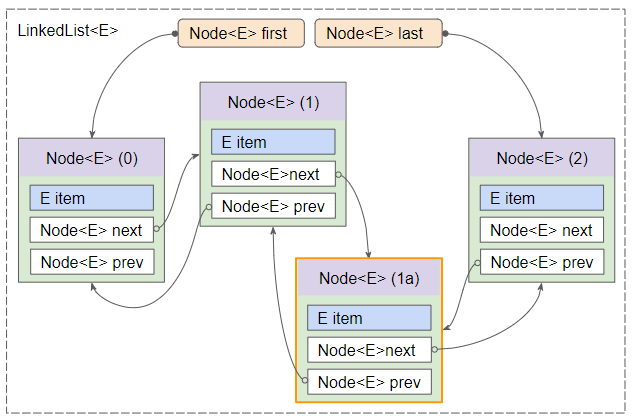
3. Examples (as List)
LinkedList can be used as a List. It supports all the methods specified in List interface, including optional methods. Therefore, you can access elements of LinkedList through Iterator, ListIterator or Stream. You can find the best examples of List in the article below:
4. Example (as Deque)
LinkedList can also be used as a Deque (Double ended Queue), or used as a stack. The best examples of Deque and stack are covered in the article below:
Deque (Double ended Queue) is like a regular queue, but you can access its first and last element.
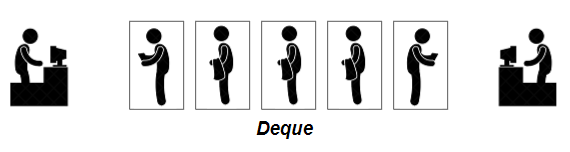
Stack works by applying LIFO principle (Last In First Out) (The last inserted element will be retrieved first).
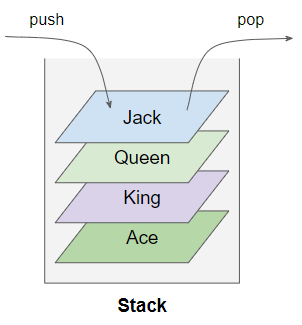
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More