Java CopyOnWriteArraySet Tutorial with Examples
1. CopyOnWriteArraySet
CopyOnWriteArraySet is a class that implements Set interface, which is commonly used in a multithreading environment, and element traversal operations are more commonly used than mutable operations like add, remove, clear.
public class CopyOnWriteArraySet<E> extends AbstractSet<E> implements java.io.Serializable
CopyOnWriteArraySet manages a CopyOnWriteArrayList object within it. All add, remove, clear operations will be performed on CopyOnWriteArrayList and ensure that it does not contain duplicate elements.
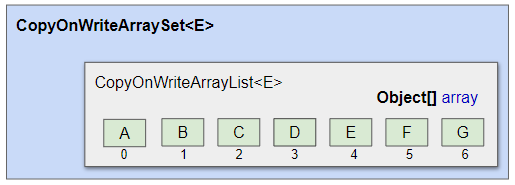
CopyOnWriteArraySet is a thread-safe Set. It will be easier to understand if you spend some time reading the article about CopyOnWriteArrayList below:
Every time you perform a mutable operation like add, remove, clear,it will create a new array to store elements. So the cost to use CopyOnWriteArraySet is very expensive, you pay more for resource and performance. However CopyOnWriteArraySet comes in handy when you can not or do not want to synchronize while traversing the elements of the Set.
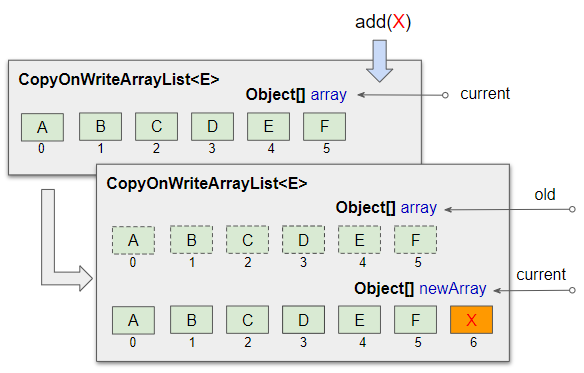
When you create an Iterator (or Stream) object from CopyOnWriteArraySet, it traverses the elements of the current array (when Iterator or Stream was created). The elements of this array will remain unchanged for the lifetime of Iterator (or Stream). This is possible because any add, remove, clear, .. operations on CopyOnWriteArraySet will create another array that is a copy of the current array.
Iterator object obtained from CopyOnWriteArraySet does not support Iterator.remove operation, if used intentionally you will get UnsupportedOperationException.
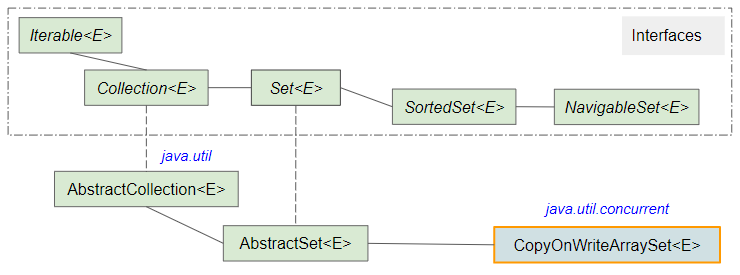
2. Examples
Example: Iterate over the elements of CopyOnWriteArraySet using Iterator, which will help you iterate over the elements of the current array (At the time the Iterator is being created).
CopyOnWriteArraySetEx1.java
package org.o7planning.copyonwritearrayset.ex;
import java.util.Iterator;
import java.util.Set;
import java.util.concurrent.CopyOnWriteArraySet;
public class CopyOnWriteArraySetEx1 {
public static void main(String[] args) {
// Create a CopyOnWriteArraySet object:
Set<String> set = new CopyOnWriteArraySet<String>();
set.add("A");
set.add("B");
set.add("C");
Iterator<String> iterator1 = set.iterator();
set.add("X1");
set.add("X2");
Iterator<String> iterator2 = set.iterator();
System.out.println("--- Iterator 1: -----");
while(iterator1.hasNext()) {
System.out.println(iterator1.next());
}
System.out.println("--- Iterator 2: -----");
while(iterator2.hasNext()) {
System.out.println(iterator2.next());
}
}
}
Output:
--- Iterator 1: -----
A
B
C
--- Iterator 2: -----
A
B
C
X1
X2
Note: CopyOnWriteArraySet's Iterator does not support Iterator.remove operation.
Stream:
Traverse the elements of CopyOnWriteArraySet through Stream also has the same result as Iterator.
CopyOnWriteArraySetEx2.java
package org.o7planning.copyonwritearrayset.ex;
import java.util.Set;
import java.util.concurrent.CopyOnWriteArraySet;
import java.util.stream.Stream;
public class CopyOnWriteArraySetEx2 {
public static void main(String[] args) {
// Create a CopyOnWriteArraySet object:
Set<String> set = new CopyOnWriteArraySet<String>();
set.add("A");
set.add("B");
set.add("C");
Stream<String> stream1 = set.stream();
set.add("X1");
set.add("X2");
Stream<String> stream2 = set.stream();
System.out.println("--- Stream 1: -----");
stream1.forEach(System.out::println);
System.out.println("--- Stream 2: -----");
stream2.forEach(System.out::println);
}
}
Output:
--- Stream 1: -----
A
B
C
--- Stream 2: -----
A
B
C
X1
X2
Besides those special characteristics mentioned above, CopyOnWriteArraySet is also a regular Set, you can find other examples of Set in the article below:
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More