Java Iterator Tutorial with Examples
1. Iterator
Iterator is one of the ways to traverse elements of a Collection. Here are the characteristics of the Iterator:
- Iterator does not guarantee iteration order of elements.
- Iterator can allow to remove elements from collection during iteration, which depends on the Collection type.
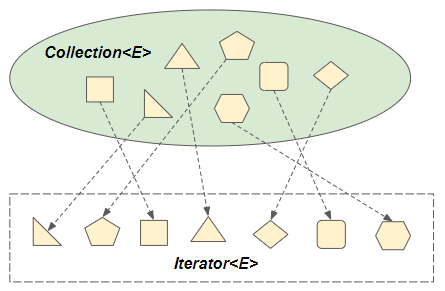
Hierarchy of Iterator sub-interfaces:
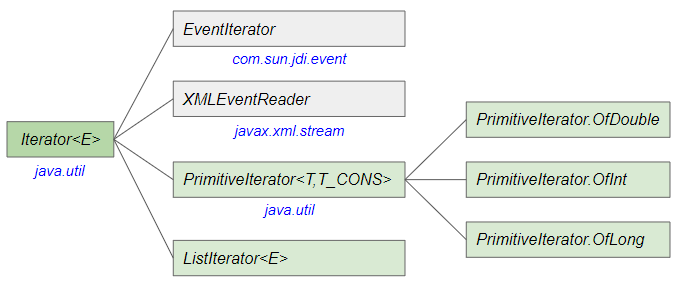
The reason why you can traverse elements of a Collection by Iterator is because Collection extends from Iterable interface.
Collection Interface
// Definition of the Collection interface:
public interface Collection<E> extends Iterable<E>
// Definition of the Iterable interface:
public interface Iterable<T> {
Iterator<T> iterator();
default void forEach(Consumer<? super T> action) {
Objects.requireNonNull(action);
for (T t : this) {
action.accept(t);
}
}
default Spliterator<T> spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), 0);
}
}
Iterator Methods
boolean hasNext()
E next();
// Optional operation.
default void remove()
default void forEachRemaining(Consumer<? super E> action)
2. Examples
With a Collection object, you can create an Iterator by Collection.iterator() method, then iterate over elements of Iterator using next() method.
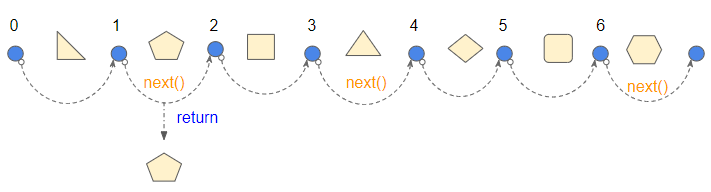
Example:
IteratorEx1.java
package org.o7planning.iterator.ex;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class IteratorEx1 {
public static void main(String[] args) {
// List is a subinterface of Collection.
List<String> flowers = new ArrayList<String>();
flowers.add("Tulip");
flowers.add("Daffodil");
flowers.add("Poppy");
flowers.add("Sunflower");
flowers.add("Bluebell");
Iterator<String> iterator = flowers.iterator();
while(iterator.hasNext()) {
String flower = iterator.next();
System.out.println(flower);
}
}
}
Output:
Tulip
Daffodil
Poppy
Sunflower
Bluebell
3. remove()
While traversing elements of a Collection using an Iterator, you can remove the current element from Collection. Iterator.remove() method allows you to do that. However, not all Iterator(s) support this operation, it depends on the Collection type. If unsupported, UnsupportedOperationException will be thrown.
// Optional Operation
public default void remove()
Example: An ArrayList<Integer> contains numbers. We will iterate over its elements and remove the current one if it is even.
Iterator_remove_ex1.java
package org.o7planning.iterator.ex;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class Iterator_remove_ex1 {
public static void main(String[] args) {
// List is a subinterface of Collection.
List<Integer> years = new ArrayList<Integer>();
years.add(1998);
years.add(1995);
years.add(2000);
years.add(2006);
years.add(2021);
Iterator<Integer> iterator = years.iterator();
while(iterator.hasNext()) {
Integer current = iterator.next();
if(current % 2 ==0) {
iterator.remove(); // Remove current element.
}
}
// After remove all even numbers:
for(Integer year: years) {
System.out.println(year);
}
}
}
Output:
1995
2021
Example of a Collection whose Iterator does not support Iterator.remove() operation:
Iterator_remove_ex2.java
package org.o7planning.iterator.ex;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
public class Iterator_remove_ex2 {
public static void main(String[] args) {
// Fixed-size List.
// Its Iterator does not support remove() operation.
List<Integer> years = Arrays.asList(1998, 1995, 2000, 2006, 2021);
Iterator<Integer> iterator = years.iterator();
while(iterator.hasNext()) {
Integer current = iterator.next();
if(current % 2 ==0) {
iterator.remove(); // UnsupportedOperationException!!
}
}
// After remove all even numbers:
for(Integer year: years) {
System.out.println(year);
}
}
}
Output:
Exception in thread "main" java.lang.UnsupportedOperationException: remove
at java.base/java.util.Iterator.remove(Iterator.java:102)
at org.o7planning.iterator.ex.Iterator_remove_ex2.main(Iterator_remove_ex2.java:20)
4. forEachRemaining(Consumer)
Performs the given action for each remaining element until all elements have been processed or the action throws an exception.
public default void forEachRemaining(Consumer<? super E> action)
Example:
Iterator_forEachRemaining.java
package org.o7planning.iterator.ex;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
public class Iterator_forEachRemaining {
public static void main(String[] args) {
// Set is a subinterface of Collection.
Set<String> flowers = new HashSet<String>();
flowers.add("Tulip");
flowers.add("Daffodil");
flowers.add("Poppy");
flowers.add("Sunflower");
flowers.add("Bluebell");
// Note: Iterator doesn't guarantee iteration order
Iterator<String> iterator = flowers.iterator();
String flower1 = iterator.next();
String flower2 = iterator.next();
System.out.println("Flower 1: " + flower1);
System.out.println("Flower 2: " + flower2);
System.out.println();
iterator.forEachRemaining(flower -> System.out.println(flower));
}
}
Output:
Flower 1: Poppy
Flower 2: Tulip
Daffodil
Sunflower
Bluebell
- Java Consumer
- Java HashSet
5. ListIterator
ListIterator is a sub interface of Iterator. It is one of the ways to traverse the elements of a List. Unlike Iterator, ListIterator supports traversing elements in both forward and backward directions. ListIterator also supports removing, updating or inserting an element during iteration.
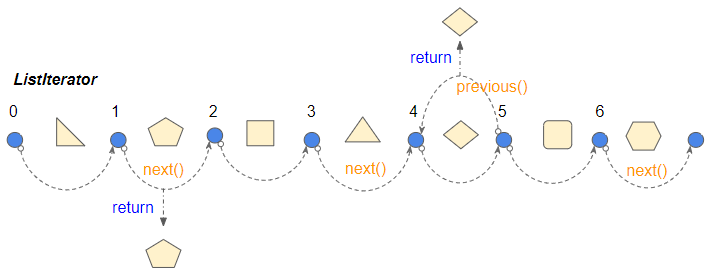
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples
Show More