Java Collections Framework Tutorial with Examples
1. Introduction
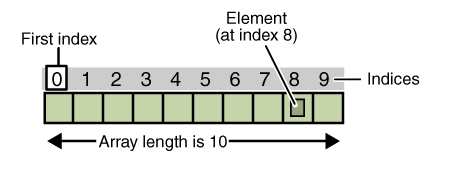
2. First example
package org.o7planning.tutorial.javacollection.helloworld;
import java.util.LinkedList;
public class HelloLinkedList {
public static void main(String[] args) {
// Create LinkedList object.
LinkedList<String> list = new LinkedList<String>();
// Add elements to the linked list.
list.add("F");
list.add("B");
list.add("D");
list.add("E");
list.add("C");
// Add the specified element to the end of this list.
list.addLast("Z");
// Add the specified element to the beginning of this list.
list.addFirst("A");
// Inserts the specified element at position with index 1.
list.add(1, "A2");
// Print out, all elements
System.out.println("Original contents of list: " + list);
// Remove a element from the linked list
list.remove("F");
// Remove the element at index 2.
list.remove(2);
// Print out the list, after removing two elements.
System.out.println("Contents of list after deletion: " + list);
// Remove first and last elements.
list.removeFirst();
list.removeLast();
// Print out collection after removing.
System.out.println("List after deleting first and last: " + list);
// Get element at index 2.
Object val = list.get(2);
// Set element at index 2.
list.set(2, (String) val + " Changed");
System.out.println("List after change: " + list);
}
}
Original contents of list: [A, A2, F, B, D, E, C, Z]
Contents of list after deletion: [A, A2, D, E, C, Z]
List after deleting first and last: [A2, D, E, C]
List after change: [A2, D, E Changed, C]
package org.o7planning.tutorial.javacollection.helloworld;
import java.util.HashMap;
public class HelloHashMap {
public static void main(String[] args) {
// Create a HashMap objects, store pairs of employee-code and salary.
// String key: Employee-code
// Float value: Salary
HashMap<String, Float> salaryMap = new HashMap<String, Float>();
salaryMap.put("E01", 1000f);
salaryMap.put("E02", 12000f);
salaryMap.put("E03", 12300f);
salaryMap.put("E04", 1000f);
salaryMap.put("E05", 300.5f);
// Get the salary of employee 'E02'
Float salary= salaryMap.get("E01");
System.out.println("Salary of employee E01 = "+ salary);
// Update the salary for employee 'E05'
salaryMap.put("E05", 400f);
System.out.println("Salary of employee E05 = "+ salaryMap.get("E05"));
}
}
Salary of employee E01 = 1000.0
Salary of employee E05 = 400.0
3. The limitations of using arrays - A suggestion to solve the problem.
- Array is very basic and familiar.
- storing the reference type, primitive type
- int[] myArray=new int[]{1,4,3};
- Object[] myArrayObj =new Object[]{"Object",new Integer(100)};
- Arrays have fixed sizes and dimensions.
- This makes it difficult to expend an array
- Elements are arranged and referenced sequentially in memory.
- This makes it difficult to remove an element from the array.
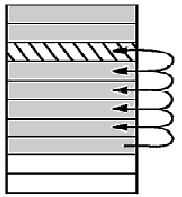
Obviously, array is not a good way in many situations of application.
Let's see features of LinkedList:
- Elements in this list can be discontinuously isolated in the memory.
- It is a two-way link between elements.
- Each element in the list makes a reference to the opposing element in front of it and the element right behind it.
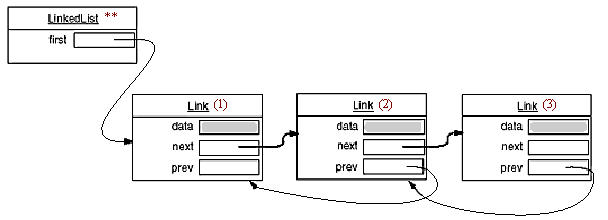
Like a group of people in queue, each person needs to remember two people standing right in front of and behind his/her.
Remove an element from LinkedList is the same as eliminating a person who are standing in a queue. Two people adjacent to this person have to reupdate the information of people in front of and behind them.
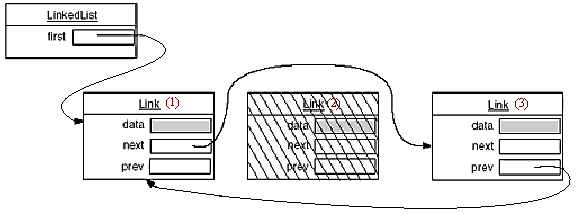
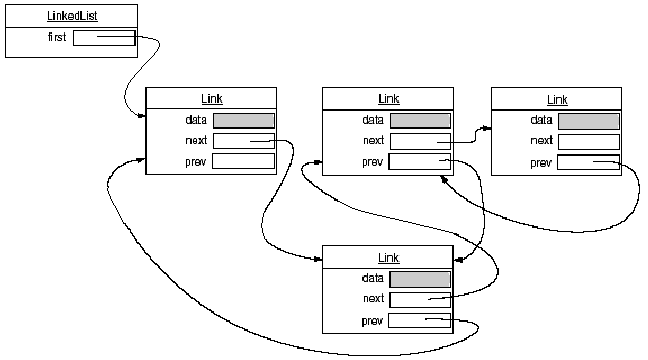
Note: LinkedList is one of solutions to array's weaknesses. ArrayList is a way of managing data collection. It can deal with array's weaknesses, but the way it manages data is different from LinkedList's.
4. Oveview of Java Collections Framework
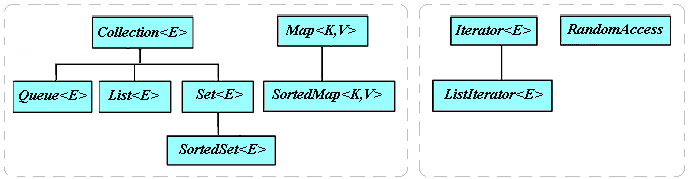
public class HashMap<K,V> extends AbstractMap<K,V>
implements Map<K,V>, Cloneable, Serializable
public class Vector<E> extends AbstractList<E>
implements List<E>, RandomAccess, Cloneable, Serializable
- Collection group stores objects.
- There are three sub-branch in Collection group: Queue, List, and Set.
- Elements may be either similar or not, depending on their branch. (More details are discussed later).
- Map group stores pairs of key and value
- The key in the Map are not allowed the same.
- If we know the key, we can extract the value equivalent to its key in Map.
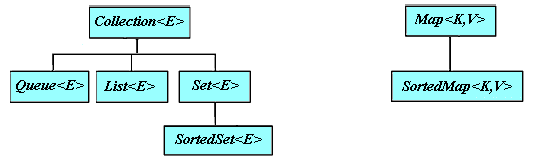
Collection<String> c=new ArrayList<String>();
// Add element to collection.
c.add("One");
Map<Integer,String> m=new LinkedHashMap<Integer,String>();
Integer key=new Integer(123);
String value="One two three";
// Add a pair of 'key/value' to Map m.
// If 'key' already exists, 'value' will be replaced by new value.
m.put(key,value);
// Print out the value corresponding to the key
System.out.println(m.get(new Integer(123));
- java.util.Iterator
- Is a iterator to retrieve data, visiting in turn from this element to another element.
- java.util.RandomAccess
- Method of random access, for example to position elements and retrieve that element in the set
- For instance, java.util.Vector implements this interface, can retrieve random element vector.get (int index).
- The Collection group can also access in turn by calling method iterator() to retrieve the Iterator object.
- java.util.Collection extending from java.lang.Iterable interface, so it inherited public Iterator<E> iterator() method, the iterator over elements of Collection.
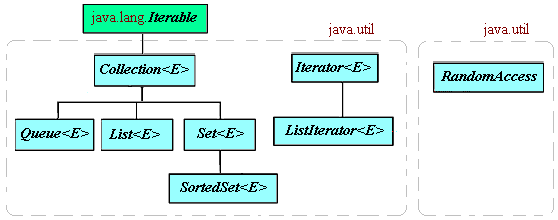
public class Vector<E> extends AbstractList<E>
implements List<E>, RandomAccess, Cloneable, Serializable
Note: For classes in the List group, you can also retrieve the ListIterator object, this iterator allows you backward or forward the cursor position on the list instead of may only forward as of the Iterator.
5. Collection Group
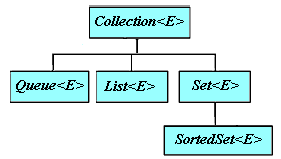
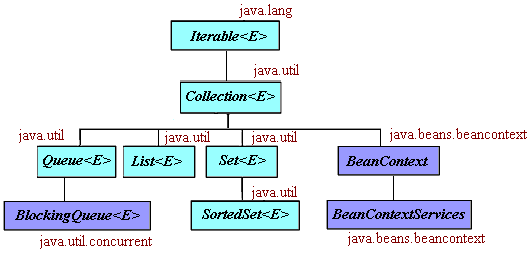
public interface Collection<E> extends java.lang.Iterable<E> {
//
// Add element to collection
// return true if this collection changed as a result of the call
//
boolean add(E o);
//
// Adds all of the elements in the specified collection to this collection.
// return true if this collection changed as a result of the call
//
boolean addAll(Collection<? extends E> c);
// Removes all of the elements from this collection (optional operation).
// The collection will be empty after this method returns.
void clear();
// Returns true if this collection contains the specified element.
boolean contains(Object o);
// Returns true if this collection contains all of the elements
// in the specified collection.
boolean containsAll(Collection<?> c);
// Compares the specified object with this collection for equality
boolean equals(Object o);
int hashCode();
// Returns true if this collection contains no elements.
boolean isEmpty();
//
// Removes a single instance of the specified element from this
// collection, if it is present (optional operation).
//
boolean remove(Object o);
// Removes all of this collection's elements that are also contained in the
// specified collection (optional operation)
boolean removeAll(Collection<?> c);
//
// Retains only the elements in this collection that are contained in the
// specified collection (optional operation)
//
boolean retainAll(Collection<?> c);
// Returns the number of elements in this collection
int size();
// Returns an array containing all of the elements in this collection
Object[] toArray();
<T> T[] toArray(T[] a);
// Returns an iterator over the elements in this collection.
Iterator<E> iterator();
}
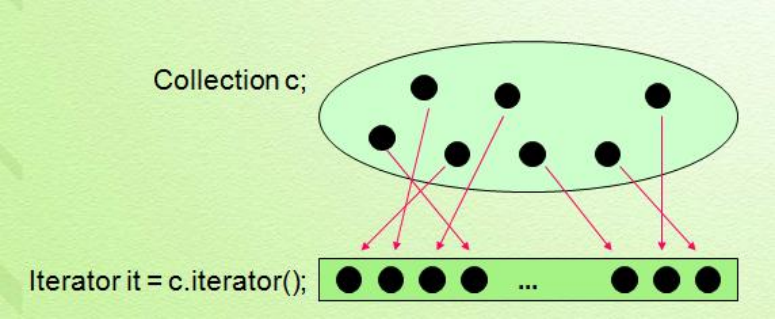
package org.o7planning.tutorial.javacollection.collection;
import java.util.Collection;
import java.util.Iterator;
import java.util.Vector;
public class CollectionAndIterator {
public static void main(String[] args) {
// Create empty collection.
// A Collection containing only String.
Collection<String> coll = new Vector<String>();
coll.add("Collection");
coll.add("Queue");
coll.add("List");
coll.add("Map");
// Print out the number of elements in this collection.
System.out.println("Size:" + coll.size());
// Returns an iterator over the elements in this collection.
// This Iterator containing only String.
Iterator<String> ite = coll.iterator();
// Check if Iteractor has next element or not?
while (ite.hasNext()) {
// Get the element at the position of the cursor.
// Then move the cursor one step further.
String s = ite.next();
System.out.println("Element:" + s);
}
}
}
Size:4
Element:Collection
Element:Queue
Element:List
Element:Map
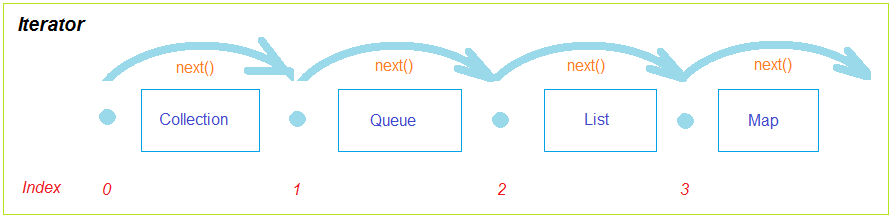
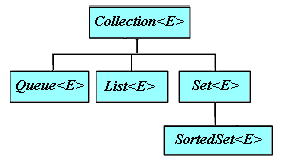
java.util.Queue | java.util.List | java.util.Set |
Allow contain duplicate elements | Allow contain duplicate elements | Do not allow duplicate elements |
Not allowed contain null elements | Allow contains one or more null elements | Depending on the class implements Set interface, supported contain null element or not. If supported, It contains only at most one null element. |
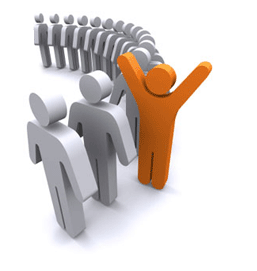
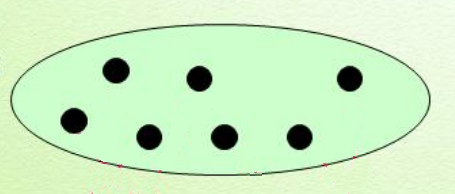
- Allow duplicate elements
- Allow zero or many null elements to exist.
- A list is an ordered list of objects
// Returns a list iterator over the elements in this list
public ListIterator<E> listIterator()
// Returns a ListIterator over the elements in this list
// (in proper sequence),
// starting at the specified position in the list..
public ListIterator<E> listIterator(int index)
package org.o7planning.tutorial.javacollection.list;
import java.util.ArrayList;
import java.util.List;
import java.util.ListIterator;
public class ListAndListIterator {
public static void main(String[] args) {
// Create List object (Containing only String).
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
list.add("Three");
list.add("Four");
ListIterator<String> listIterator = list.listIterator();
// Currently the cursor at the first position of the Iterator.
// (Index 0).
// Get the first element in the interator, the cursor forward one step.
String first = listIterator.next();
System.out.println("first:" + first);// -->"One"
// Current cursor at index 1
// Get next element.
String second = listIterator.next();
System.out.println("second:" + second);// -->"Two"
// Check if the cursor can jump back 1 step or not.
if (listIterator.hasPrevious()) {
// Go back one step.
String value = listIterator.previous();
System.out.println("value:" + value);// -->"Two"
}
System.out.println(" ----- ");
while (listIterator.hasNext()) {
String value = listIterator.next();
System.out.println("value:" + value);
}
}
}
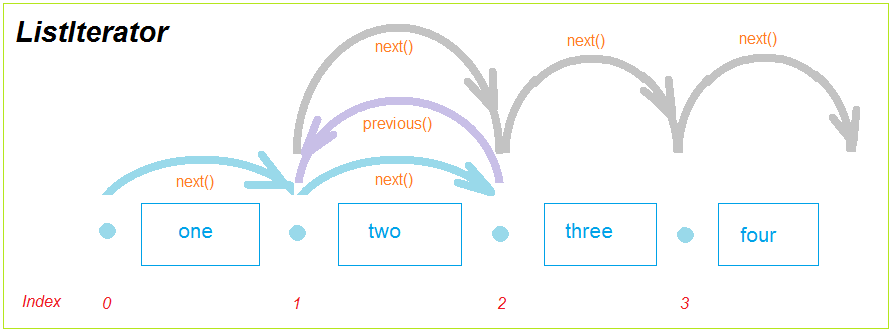
first:One
second:Two
value:Two
-----
value:Two
value:Three
value:Four
- Describe a set that does not allow duplicate elements
- Allow the existence of one null element, if any.
package org.o7planning.tutorial.javacollection.set;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
public class HashSetExample {
public static void main(String[] args) {
// Create a Set object with initial capacity 10.
// Automatically increase capacity 80% if the number of elements to
// overcome the current capacity.
// HashSet (LIFO - Last in first out)
// (element to be added later will stand first).
Set<String> set = new HashSet<String>(10, (float) 0.8);
set.add("One");
set.add("Two");
// When Duplication occurs.
// With HashSet: It will add new element, and remove the old element.
set.add("One");
set.add("Three");
Iterator<String> it = set.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
}
}
One
Two
Three
- Queue allows elements to duplicate .
- Do not allow null elements.
- java.util.LinkedList
- java.util.PriorityQueue
PriorityQueue stores its elements internally according to their natural order (if they implement Comparable), or according to a Comparator passed to the PriorityQueue.
Throws exception | Returns special value | |
Insert | add(e) | offer(e) |
Remove | remove() | poll() |
Examine | element() | peek() |
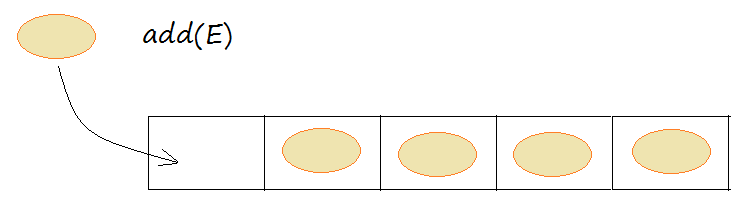
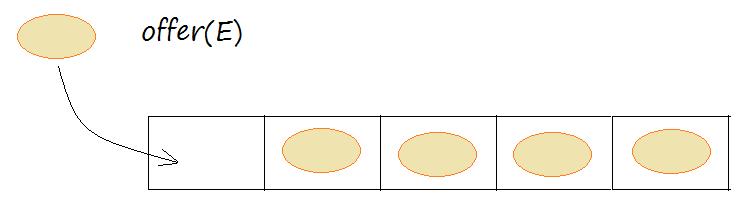
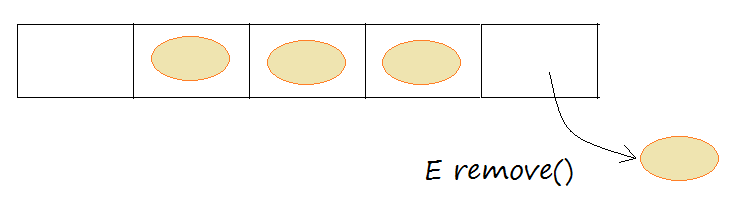
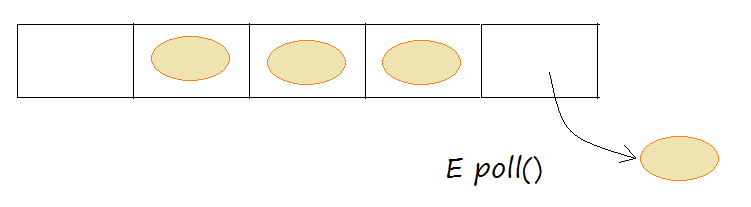
peek
only in that it throws an exception if this queue is empty.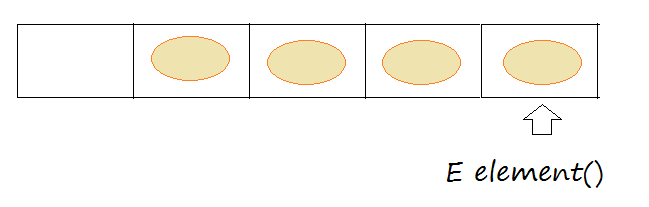
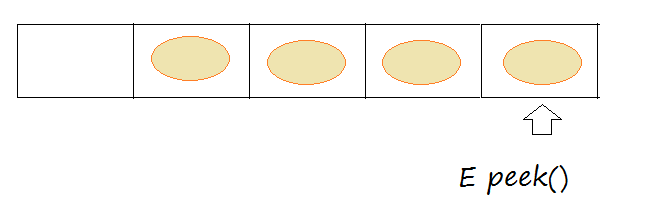
null
if this queue is empty.
package org.o7planning.tutorial.javacollection.queue;
import java.util.LinkedList;
import java.util.Queue;
public class QueueDemo {
public static void main(String[] args) {
Queue<String> names = new LinkedList<String>();
// offer(E): Insert element to queue.
// With LinkedList, element will inserted at the end of queue.
// Return true if success.
// Return false if queue full.
names.offer("E");
names.offer("A");
names.offer("M");
// add(E): Insert element to queue
// With LinkedList, element will inserted at the end of queue.
// Return true if success.
// Throw exception if queue full.
names.add("G");
names.add("B");
while (true) {
// Retrieves and removes the head of this queue,
// or returns null if this queue is empty.
String name = names.poll();
if (name == null) {
break;
}
System.out.println("Name=" + name);
}
}
}
Name=E
Name=A
Name=M
Name=G
Name=B
package org.o7planning.tutorial.javacollection.queue;
import java.util.PriorityQueue;
import java.util.Queue;
public class PriorityQueueDemo {
public static void main(String[] args) {
// With PriorityQueue queue, the elements will be arranged on the natural order.
Queue<String> names = new PriorityQueue<String>();
// offer(E): Insert element to queue.
// Return true if success
// Return false if queue is full.
names.offer("E");
names.offer("A");
names.offer("M");
// add(E): Insert element to queue.
// Return true if success
// Throw exception if queue is full.
names.add("G");
names.add("B");
while (true) {
// Retrieves and removes the head of this queue,
// or returns null if this queue is empty.
String name = names.poll();
if (name == null) {
break;
}
System.out.println("Name=" + name);
}
}
}
Name=A
Name=B
Name=E
Name=G
Name=M
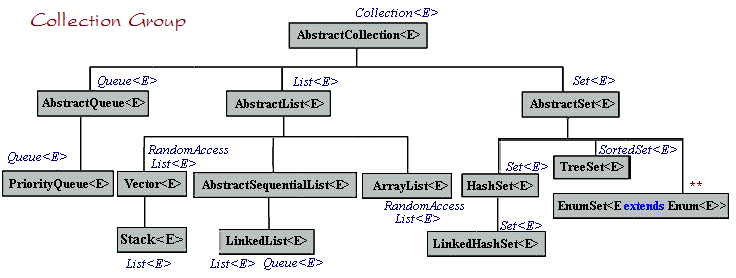
Implementations | |||||||
Hash Table | Resizable Array | Balanced Tree | Linked List | Hash Table + Linked List | |||
Interfaces | Set | HashSet | TreeSet | LinkedHashSet | |||
Interfaces | List | ArrayList |
package org.o7planning.tutorial.javacollection.list;
import java.util.ArrayList;
public class ArrayListDemo {
public static void main(String[] args) {
// Create an ArrayList object that contains the element of type Integer.
ArrayList<Integer> list = new ArrayList<Integer>(10);
// Add elements.
list.add(123);
list.add(245);
list.add(new Integer(345));
// ArrayList allow add null elements.
// (Feature of List)
list.add(null);
// Print out the number of elements in this list.
System.out.println("Size:" + list.size());// =4
// Random access to elements of index 1.
Integer i = list.get(1);
System.out.println("Element index 1 =" + i);// =245
Integer newInt = 1000;
// Replaces the element at the index 1.
// This method return old element.
Integer old = list.set(1, newInt);
//
System.out.println("Old value:" + old);// =245 .
System.out.println("New value:" + list.get(1));// =1000 .
}
}
Size:4
Element index 1 =245
Old value:245
New value:1000
Methods of Vector are synchronized, so it works well in Multiple Thread applications.
Methods of Vector are synchronized, so it works well in Multiple Thread applications.
// Legacy method from 1.0, get element at index position
// Like get(index)
public E elementAt(int index)
// Method inherited from the List interface, get element at position index.
public E get(int index)
// Replaces the element at the specified position in this list with the specified element
// Return old element.
// setElementAt(int,E) like set(int,E)
public void setElementAt(int index, E element);
// Replaces the element at the specified position in this list with the specified element
// Return old element.
public E set(int index, E element)
package org.o7planning.tutorial.javacollection.list;
import java.util.Vector;
public class VectorDemo {
public static void main(String[] args) {
// Create Vector object with capacity 10 (element)
// Automatically increase capacity 5,
// if the number of elements to overcome the current capacity.
Vector<Integer> v = new Vector<Integer>(10, 5);
v.add(123);
v.add(245);
v.add(new Integer(345));
v.add(null);
// Print out the number of elements in this Vector. (Not capacity).
System.out.println("Size:" + v.size());// =4
// Get element at index 1
// (Same as get(int) method)
Integer i = v.elementAt(1);
System.out.println("v.elementAt(1)=" + i);// 245
v.setElementAt(1000, 1);
//
System.out.println("New value:" + v.get(1));// =1000 .
}
}
Size:4
v.elementAt(1)=245
New value:1000
Therefore, the elements of the SortedSet must be compared with each other, and they must be the object of java.lang.Comparable (Can be comparable). If you add an element which is not the object of Comparable to the SortedSet, you'll get an exception.
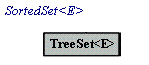
Players can be compared with each other according to the principle:
- Who has more gold medals will rank higher.
- If two of the same number of gold medals, who has more silver medal will rank higher.
- If two people have the same number of gold and silver medals, who has more bronze medals than the other will rank higher.
- Others considered as same rank.
package org.o7planning.tutorial.javacollection.sortedset;
public class Player implements Comparable<Player> {
private String name;
private int goldMedal;
private int silverMedal;
private int bronzeMedal;
public Player(String name, int goldMedal, int silverMedal, int bronzeMedal) {
this.name = name;
this.goldMedal = goldMedal;
this.silverMedal = silverMedal;
this.bronzeMedal = bronzeMedal;
}
// Compare this player with other player
// If return value < 0 means this player < other
// If return value > 0 means this player > other
// If return value = 0 means this player = other
@Override
public int compareTo(Player other) {
// Compare the number of gold medals.
int value = this.goldMedal - other.goldMedal;
if (value != 0) {
return value;
}
// Compare the number of silver medals.
value = this.silverMedal - other.silverMedal;
if (value != 0) {
return value;
}
// Compare the number of bronze medals.
value = this.bronzeMedal - other.bronzeMedal;
return value;
}
@Override
public String toString() {
return "[" + this.name + ", Gold: " + this.goldMedal //
+ ", Silver: " + this.silverMedal + ", Bronze: " //
+ this.bronzeMedal + "]";
}
}
package org.o7planning.tutorial.javacollection.sortedset;
import java.util.SortedSet;
import java.util.TreeSet;
public class SortedSetDemo {
public static void main(String[] args) {
// Create a SortedSet object via the subclass TreeSet.
SortedSet<Player> players = new TreeSet<Player>();
Player tom = new Player("Tom", 1, 3, 5);
Player jerry = new Player("Jerry", 3, 1, 3);
Player donald = new Player("Donal", 2, 10, 0);
// Add element to set
// They will automatically be sorted (Ascending).
players.add(tom);
players.add(jerry);
players.add(donald);
// Print out elements
for (Player player : players) {
System.out.println("Player: " + player);
}
}
}
Player: [Tom, Gold: 1, Silver: 3, Bronze: 5]
Player: [Donal, Gold: 2, Silver: 10, Bronze: 0]
Player: [Jerry, Gold: 3, Silver: 1, Bronze: 3]
6. Map group
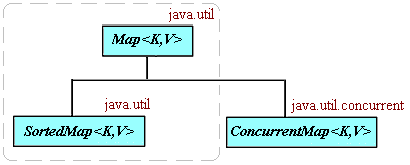
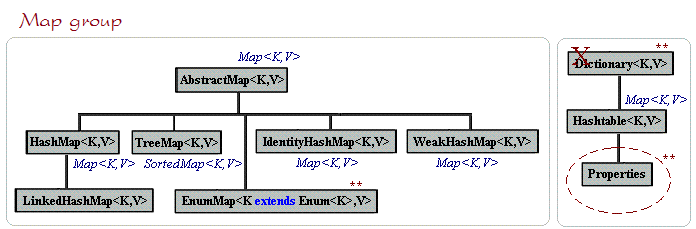
SN | Methods with Description |
1 | void clear( ) Removes all key/value pairs from the invoking map.(optional operation). |
2 | boolean containsKey(Object k) Returns true if the invoking map contains k as a key. Otherwise, returns false. |
3 | boolean containsValue(Object v) Returns true if the map contains v as a value. Otherwise, returns false |
4 | Set<Map.Entry<K,V>> entrySet( ) Returns a Set that contains the entries in the map. The set contains objects of type Map.Entry. This method provides a set-view of the invoking map. |
5 | boolean equals(Object obj) Returns true if obj is a Map and contains the same entries. Otherwise, returns false. |
6 | Object get(K k) Returns the value associated with the key k. |
7 | int hashCode( ) Returns the hash code for the invoking map. |
8 | boolean isEmpty( ) Returns true if the invoking map is empty. Otherwise, returns false. |
9 | Set<K> keySet( ) Returns a Set that contains the keys in the invoking map. This method provides a set-view of the keys in the invoking map. |
10 | Object put(K k, V v) Puts an entry in the invoking map, overwriting any previous value associated with the key. The key and value are k and v, respectively. Returns null if the key did not already exist. Otherwise, the previous value linked to the key is returned.(optional operation). |
11 | void putAll(Map<? extends K,? extends V> m) Puts all the entries from m into this map.(optional operation). |
12 | Object remove(Object k) Removes the entry whose key equals k. (optional operation). |
13 | int size( ) Returns the number of key/value pairs in the map. |
14 | Collection values( ) Returns a collection containing the values in the map. This method provides a collection-view of the values in the map. |
import java.util.Collection;
import java.util.Map;
import java.util.Set;
public class MyMap<K,V> implements Map<K,V>{
.....
// If you call this method, an exception will be thrown unconditionally.
@Override
public void clear() {
throw new java.lang.UnsupportedOperationException();
}
}
package org.o7planning.tutorial.javacollection.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class MapDemo {
public static void main(String[] args) {
Map<String, String> map = new HashMap<String, String>();
map.put("01000005", "Tom");
map.put("01000002", "Jerry");
map.put("01000003", "Tom");
map.put("01000004", "Donald");
// Get a set view of the keys contained in this map.
// This collection is not sorted.
Set<String> phones = map.keySet();
for (String phone : phones) {
System.out.println("Phone: " + phone + " : " + map.get(phone));
}
}
}
Phone: 01000004 : Donald
Phone: 01000003 : Tom
Phone: 01000005 : Tom
Phone: 01000002 : Jerry
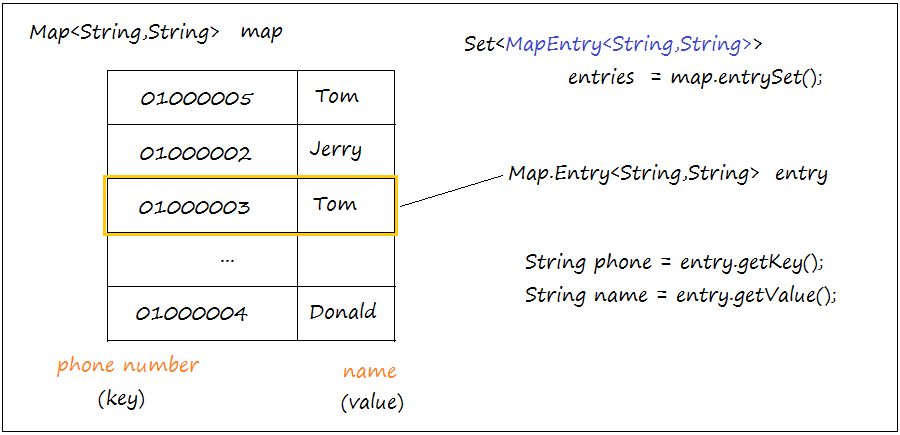
package org.o7planning.tutorial.javacollection.map;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.Map.Entry;
public class MapEntryDemo {
public static void main(String[] args) {
Map<String, String> map = new HashMap<String, String>();
map.put("01000005", "Tom");
map.put("01000002", "Jerry");
map.put("01000003", "Tom");
map.put("01000004", "Donald");
// Get set of entries
// This entry may not sort by key.
Set<Entry<String, String>> entries = map.entrySet();
for (Entry<String, String> entry : entries) {
System.out.println("Phone: " + entry.getKey() + " : " + entry.getValue());
}
}
}
Phone: 01000004 : Donald
Phone: 01000003 : Tom
Phone: 01000005 : Tom
Phone: 01000002 : Jerry
SN | Methods with Description |
1 | Comparator comparator( ) Returns the invoking sorted map's comparator. If the natural ordering is used for the invoking map, null is returned. |
2 | Object firstKey( ) Returns the first key in the invoking map. |
3 | SortedMap headMap(Object end) Returns a sorted map for those map entries with keys that are less than end. |
4 | Object lastKey( ) Returns the last key in the invoking map. |
5 | SortedMap subMap(Object start, Object end) Returns a map containing those entries with keys that are greater than or equal to start and less than end |
6 | SortedMap tailMap(Object start) Returns a map containing those entries with keys that are greater than or equal to start. |
package org.o7planning.tutorial.javacollection.sortedmap;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
public class SortedMapDemo {
public static void main(String[] args) {
Map<String, String> map = new TreeMap<String, String>();
map.put("01000005", "Tom");
map.put("01000002", "Jerry");
map.put("01000003", "Tom");
map.put("01000004", "Donald");
// This set has been sorted in ascending.
Set<String> keys = map.keySet();
for (String key : keys) {
System.out.println("Phone: " + key);
}
System.out.println("-----");
// This set has been sorted in ascending.
Set<Map.Entry<String, String>> entries = map.entrySet();
for (Map.Entry<String, String> entry : entries) {
System.out.println("Phone: " + entry.getKey());
}
}
}
Phone: 01000002
Phone: 01000003
Phone: 01000004
Phone: 01000005
-----
Phone: 01000002
Phone: 01000003
Phone: 01000004
Phone: 01000005
Java Collections Framework Tutorials
- Java PriorityBlockingQueue Tutorial with Examples
- Java Collections Framework Tutorial with Examples
- Java SortedSet Tutorial with Examples
- Java List Tutorial with Examples
- Java Iterator Tutorial with Examples
- Java NavigableSet Tutorial with Examples
- Java ListIterator Tutorial with Examples
- Java ArrayList Tutorial with Examples
- Java CopyOnWriteArrayList Tutorial with Examples
- Java LinkedList Tutorial with Examples
- Java Set Tutorial with Examples
- Java TreeSet Tutorial with Examples
- Java CopyOnWriteArraySet Tutorial with Examples
- Java Queue Tutorial with Examples
- Java Deque Tutorial with Examples
- Java IdentityHashMap Tutorial with Examples
- Java WeakHashMap Tutorial with Examples
- Java Map Tutorial with Examples
- Java SortedMap Tutorial with Examples
- Java NavigableMap Tutorial with Examples
- Java HashMap Tutorial with Examples
- Java TreeMap Tutorial with Examples
- Java PriorityQueue Tutorial with Examples
- Java BlockingQueue Tutorial with Examples
- Java ArrayBlockingQueue Tutorial with Examples
- Java TransferQueue Tutorial with Examples