Quickstart with ReactJS - Hello ReactJS
1. Approaches to ReactJS
First of all, We confirm that React is a Javascript library, which is used to run on the Client side. But it can also be run on the Server if the Server understands Javascript, for example, NodeJS Server.
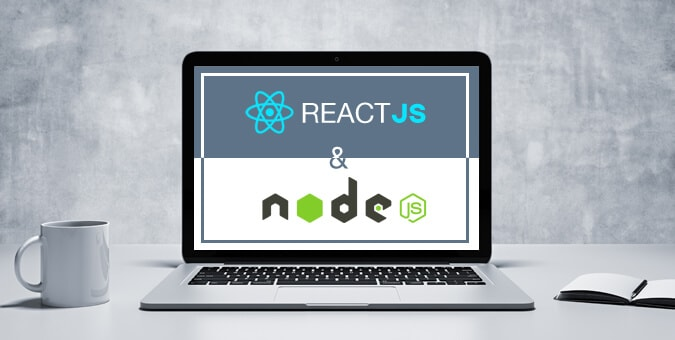
So, basically you have 2 ways to approach the React.
- Install a NodeJS Server environment, then you can program Fullstack applications, which means you can program the functions at the Server, and the functions at the Client need to know only Javascript language.
- Need to study only React to program the applications at the Client side using the Javascript, and at the Server side, it can be one of the languages such as Java, DotNet, PHP,...
If you are a beginner of React, you should learn pure React without having to learn NodeJS. You need only React libraries and can practice lessons with the browser.
After you have be proficient with the React on the Client side, you can learn about how to install the NodeJS Server environment, and how to create a React application running on NodeJS. Of course if you are not a fan of NodeJS you can skip this hint.
2. HTTP Server?
When studying the ReactJS, you need a HTTP Server. Then, your HTML file can run on the browser by the link http:// instead of file:///. The reason you need a HTTP Server is that sometimes the browser prevents a local Javascript file from working. It requires that the Javascript file has to come from http (Or https).
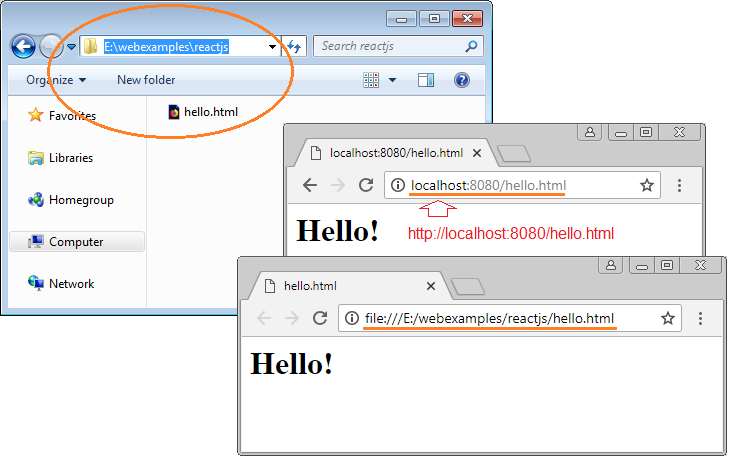
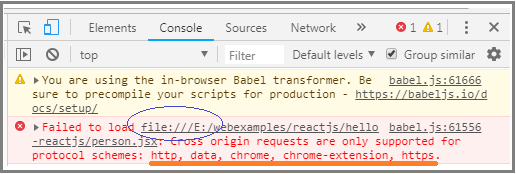
You have many ways to obtain a HTTP Server. If you are familiar with Java you can use Tomcat. if you are familiar with PHP you can use NGINX...
NodeJS HTTP Server?You probably do not need to know about NodeJS, but in only 5 minutes you can create a simple NodeJS HTTP Server. Of course, if you already have an HTTP Server, you can skip this hint.
3. Download ReactJS & Babel
React is a Javascript library. You can use the direct sources of Internet without downloading. In the posts on the o7planning, I will use ReactJS library directly on Internet.
Click on the following link. It helps you to check the released versions of React:
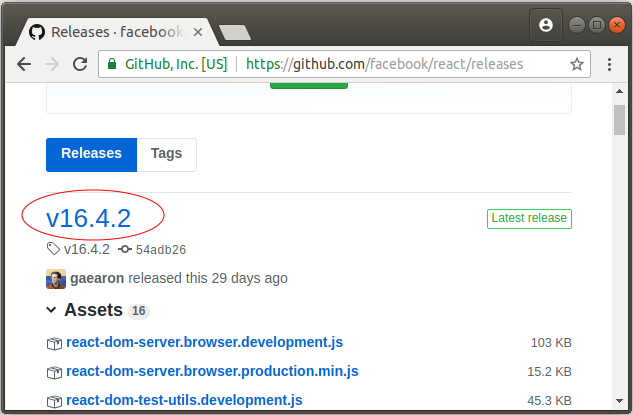
The React library includes 2 parts such as React & ReactDOM. You can use them directly through 2 following CDN Links. Note: Replace *** with a specific version that you see above.
<script crossorigin src="https://unpkg.com/react@***/umd/react.production.min.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@***/umd/react-dom.production.min.js"></script>
Replace *** with a specific version:
ReactJS 16.4.2
<script crossorigin src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
The latest version in August 2018 is 16.4.2. If you want to use the latest version out of the 16.x versions, you can use CDN Link like below:
ReactJS 16.x (Newest).
<script crossorigin src="https://unpkg.com/react@16/umd/react.production.min.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16/umd/react-dom.production.min.js"></script>
Babel
Babel is a Javascript library. It helps to convert JSX codes into Javascript. I will explain more about the Babel while practising it with examples.
Babel
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
You can seek a newer Babel version by the following link:
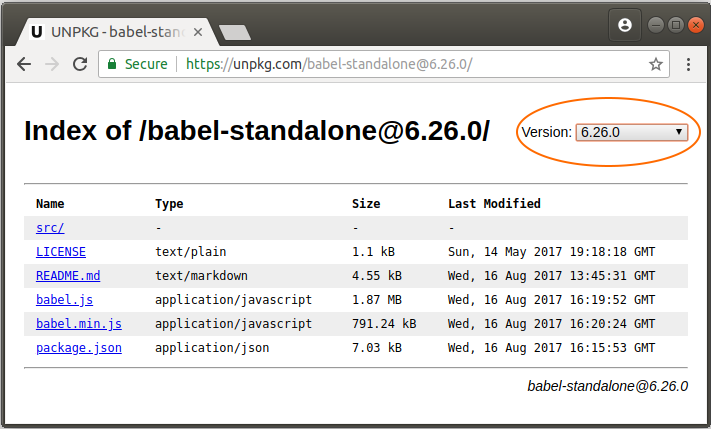
- Babel la gi?
4. Start with ReactJS
The most important feature of React is allowing you to create your own Component, and use it in your project.
OK, the objectives of this lesson:
- Create a Rect Component with JSX (Javascript Syntax Extension) and use this Component in HTML.
- Explain the difference between Javascript & JSX, Explain about Babel.
- Create a React Component with Javascript (not using JSX).
Create hello-reactjs directory and 2 person.jsx & people.html files inside it. You can use any editor to compose it, for example, Atom,...
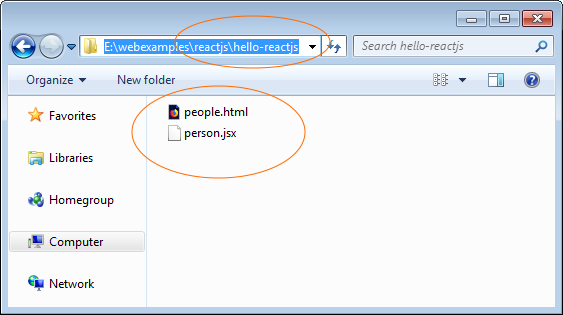
people.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello ReactJS</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.person-info {
border: 1px solid blue;
margin: 5px;
padding: 5px;
width: 250px;
}
</style>
</head>
<body>
<h1>People</h1>
<div id="person1"></div>
<div id="person2"></div>
<script src="person.jsx" type="text/babel"></script>
</body>
</html>
person.jsx
// Create a ES6 class component
class Person extends React.Component {
// Use the render function to return JSX component
render() {
return (
<div className="person-info">
<h3>Person {this.props.personNo}:</h3>
<ul>
<li>First Name: {this.props.firstName}</li>
<li>Last Name: {this.props.lastName}</li>
</ul>
</div>
);
}
}
const element1 = document.getElementById('person1')
const element2 = document.getElementById('person2')
// Use the ReactDOM.render to show your component on the browser
ReactDOM.render(
<Person personNo='1' firstName='Bill' lastName='Gates' />, element1
)
// Use the ReactDOM.render to show your component on the browser
ReactDOM.render(
<Person personNo='2' firstName='Donald' lastName='Trump' />, element2
)
Note: In JSX, you want to say about "class" attribute, you have to use the word "className", because "class" is a key word in the Javascript. This helps to avoid conflict.<div className="person-info">
Start HTTP Server so that we can run HTML files through http:// (See the above instructions of the post).
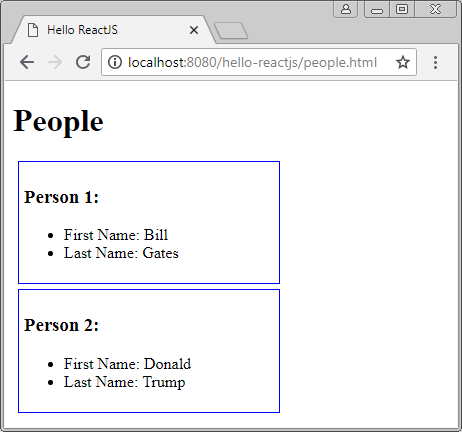
OK, In the above step, you have created and run an example with ReactJS successfully. Now, I will explain about the code of the example.
Component: Person (?)
The idea is that you want to define your <Person> element and it has three attributes such as personNo, firstName, lastName.
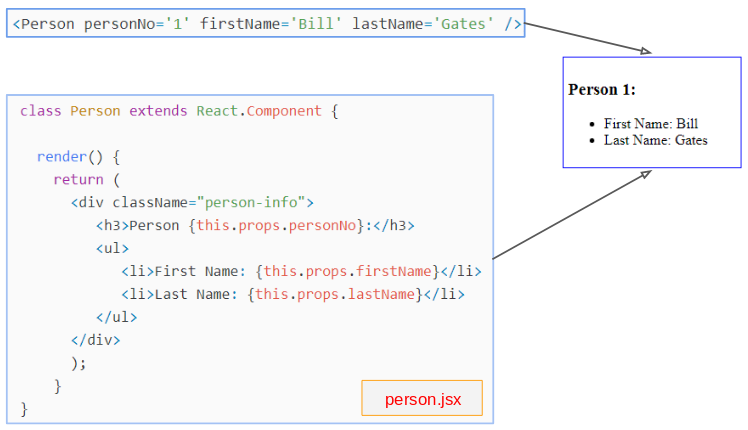
You define a Person class in the person.jsx file. Please note that the syntax used is JSX (Javascript Syntax Extension), which is the mixture between Javascript and HTML. Your browser can only understand Javascript. It doesn't understand JSX. But, BABEL helps convert the JSX code into Javasript.
If it is imperative to write a Component with Javascript, your code will be very long and much more difficult to see:
Component (Javascript)
// Create React Component with Javascript (Noooooooo JSX)!!
class Person extends React.Component {
render( ) {
var h3Element = React.createElement("h3", null, 'Person ' + this.props.personNo);
var ulElement = React.createElement("ul", null, [
React.createElement("li", null, "First Name: " + this.props.firstName),
React.createElement("li", null, "Last Name: " + this.props.lastName)
]);
var e = React.createElement("div", {
class: 'person-info'
}, [
h3Element, ulElement
]);
return e;
}
}
Embed the JSX file into HTML, you need to use <script type = "text / babel">.
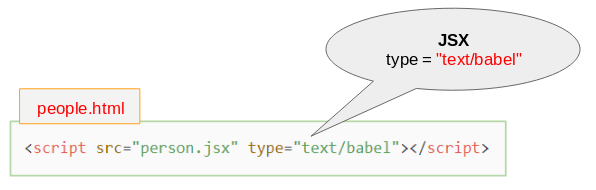
After creating a Component, which is like that you have just created a new tag.
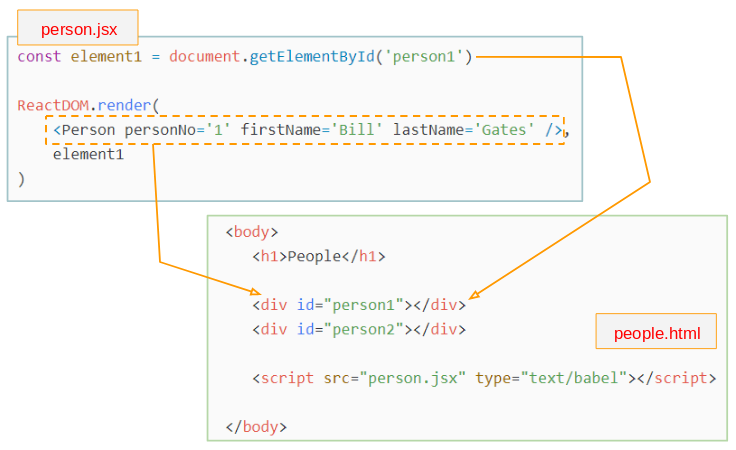
5. React with Javascript (no JSX)
What happens if you want ReactJS but say no with JSX?
- The answer is that you have to use Javascript to create a HTML code instead of writing the HTML code directly such as the syntax of JSX. This makes your code longer and more difficult to see.
OK, below is an example using the ReactJS with Javascript (say no with JSX).
person2.js
class Person extends React.Component {
render() {
var h3Element = React.createElement("h3", null, 'Person ' + this.props.personNo);
var ulElement = React.createElement("ul", null, [
React.createElement("li", null, "First Name: " + this.props.firstName),
React.createElement("li", null, "Last Name: " + this.props.lastName)
]
);
var e = React.createElement("div", {class: 'person-info'}, [
h3Element, ulElement
]);
return e;
}
}
const element1 = document.getElementById('person1')
const element2 = document.getElementById('person2')
// Use the ReactDOM.render to show your component on the browser
ReactDOM.render(
React.createElement(Person, {personNo: 1, firstName:'Bill', lastName: 'Gates'}, null),
element1
)
ReactDOM.render(
React.createElement(Person, {personNo: 2, firstName:'Donald', lastName: 'Trump'}, null),
element2
)
people2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello ReactJS</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<style>
.person-info {
border: 1px solid blue;
margin: 5px;
padding: 5px;
width: 250px;
}
</style>
</head>
<body>
<h1>People</h1>
<div id="person1"></div>
<div id="person2"></div>
<script src="person2.js" type="text/javascript"></script>
</body>
</html>
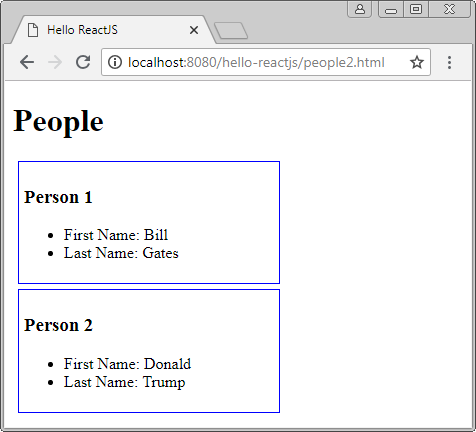
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
Show More