ReactJS Component API Tutorial with Examples
1. ReactJS Component API
ReactJS goes through a development process. Initially, the components are written based on the old Javascript syntax. Until 0.14, ReactJS version, it was transfered to use the Javascript according to the ES6 standard. Many old Component APIs are deprecated or removed to conform to the new standard. In this lesson, I just introduce the useful Component APIs and is suitable for Javascript ES6.
- setState()
- forceUpdate
- ReactDOM.findDOMNode()
2. setState( )
The setState() method is used to update the status of Component, and it says with the React that "let's re-render the Component on interface based on changes of the status.
Usage of the setState() method is detailed in the following post by me:
3. forceUpdate( )
Sometimes, you want to update the component manually. This can be achieved by using the forceUpdate () method. This is a method of the React.Component class, therefore, the subclasses of React.Component will inherit this method.
forceUpdate-example.jsx
class Random extends React.Component {
constructor(props) {
super(props);
}
newRandomValue(event) {
this.forceUpdate();
}
render() {
return (
<div>
<button onClick={event => this.newRandomValue(event)}>Random</button>
<div>Random Value: {Math.random()}</div>
</div>
);
}
}
// Render
ReactDOM.render(<Random />, document.getElementById("random1"));
forceUpdate-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS forceUpdate()</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.search-box {
border:1px solid #cbbfab;
padding: 5px;
}
</style>
</head>
<body>
<h1>forceUpdate() example:</h1>
<div id="random1"></div>
<script src="forceUpdate-example.jsx" type="text/babel"></script>
</body>
</html>
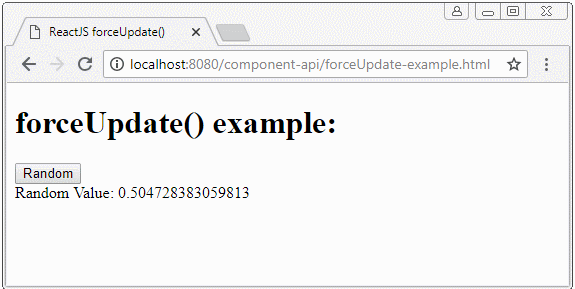
4. ReactDOM.findDOMNode( )
As you know, a Component is a class, when the Component is rendered on the interface, you will obtain a DOM model. Thus, the Component and the DOM are two different concepts. How do you do to be able to access to the Nodes of the DOM from the Component (class)?
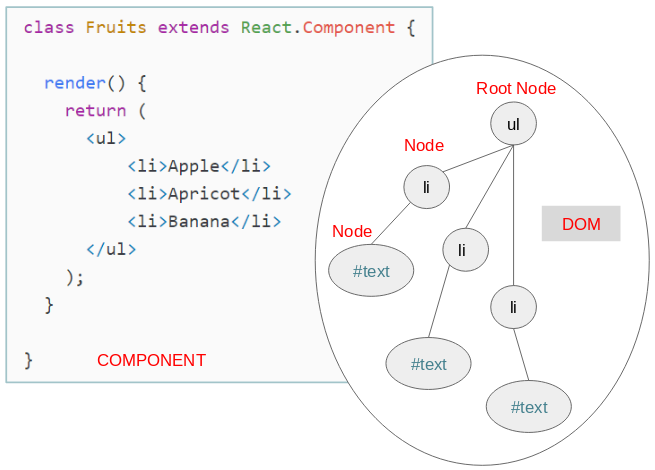
ReactDOM provides you with the ReactDOM.findDOMNode(param) method to seek a Node object corresponding to the parameters of the method.
ReactDOM.findDOMNode(this)
Inside the Component (class), if you callthe ReactDOM.findDOMNode(this) method, it will return you the Root Node of DOM model.
findDOMNode-example.jsx
class Fruits extends React.Component {
doFind() {
// Find root Node of this Component
var node = ReactDOM.findDOMNode(this);
node.style.border = "1px solid red";
}
render() {
return (
<ul>
<li>Apple</li>
<li>Apricot</li>
<li>Banana</li>
<li>
<button onClick={() => this.doFind()}>Find Root Node</button>
</li>
</ul>
);
}
}
// Render
ReactDOM.render(<Fruits />, document.getElementById("fruits1"));
findDOMNode-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS findDOMNode()</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
#fruits1 {
border:1px solid blue;
padding: 5px;
margin-top: 20px;
}
</style>
</head>
<body>
<h3>Example: findDOMNode(this)</h3>
<a href="">Reset</a>
<div id="fruits1"></div>
<script src="findDOMNode-example.jsx" type="text/babel"></script>
</body>
</html>
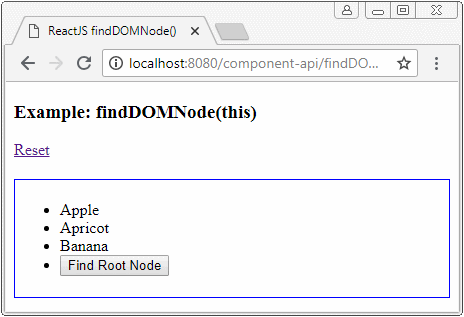
findDOMNode(ref)
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
Show More