Undertanding ReactJS Router with a basic example (NodeJS)
1. What is React Router?
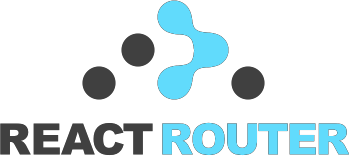
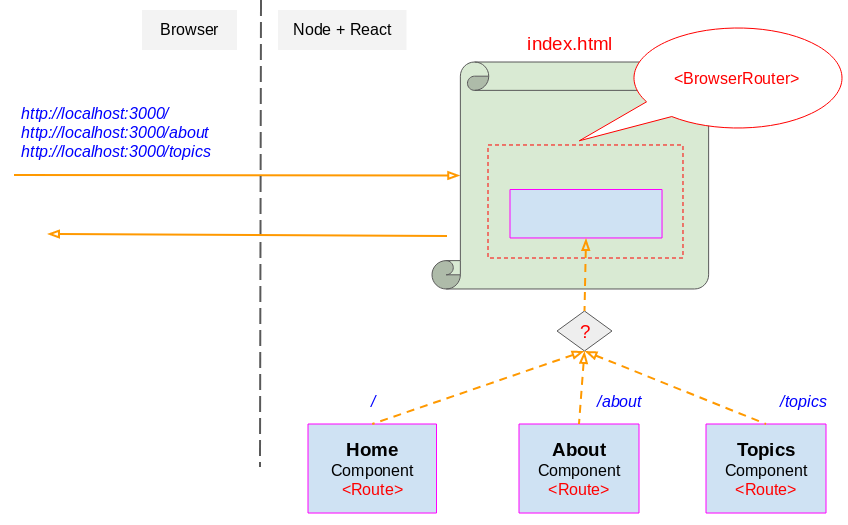
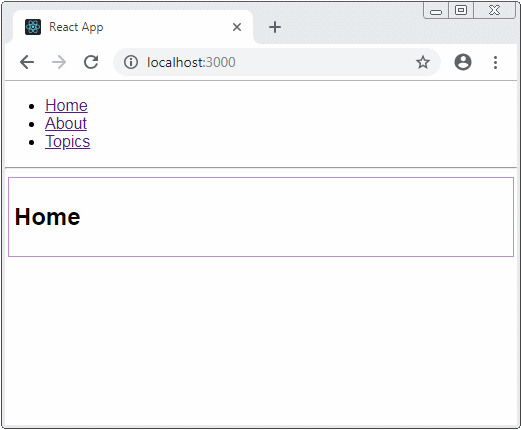
// <BrowserRouter>
http://example.com/about
// <HashRouter>
http://example.com/#/about
<BrowserRouter>
<Route exact path="/" component={Home}/>
<Route path="/about" component={About}/>
<Route path="/topics" component={Topics}/>
</BrowserRouter>
<HashRouter>
<Route exact path="/" component={Home}/>
<Route path="/about" component={About}/>
<Route path="/topics" component={Topics}/>
</HashRouter>
<BrowserRouter>
...
<Route path="/about" component={About}/>
...
</BrowserRouter>
http://example.com/about ==> OK Work!
http://example.com/about#somthing ==> OK Work!
http://example.com/about/something ==> OK Work!
http://example.com/about/a/b ==> OK Work!
-------------------
http://example.com/something/about ==> Not Work!
http://example.com/something/about#something ==> Not Work!
http://example.com/something/about/something ==> Not Work!
<HashRouter>
...
<Route path="/about" component={About}/>
...
</HashRouter>
http://example.com#/about ==> OK Work!
http://example.com#/about/somthing ==> OK Work!
----------------
http://example.com/something ==> Not Work!
http://example.com/something#/about ==> Not Work!
<BrowserRouter>
...
<Route exact path="/about" component={About}/>
...
</BrowserRouter>
http://example.com/about ==> OK Work!
http://example.com/about#somthing ==> OK Work!
-------------
http://example.com/about/something ==> Not Work!
http://example.com/about/a/b ==> Not Work!
http://example.com/something/about ==> Not Work!
http://example.com/something/about#something ==> Not Work!
http://example.com/something/about/something ==> Not Work!
<HashRouter>
...
<Route exact path="/about" component={About}/>
...
</HashRouter>
http://example.com#/about ==> OK Work!
----------------
http://example.com#/about/somthing ==> Not Work!
http://example.com/something ==> Not Work!
http://example.com/something#/about ==> Not Work!
2. Create a project and install library
# Install tool:
npm install -g create-react-app
# Create project named 'react-router-basic-app':
create-react-app react-router-basic-app
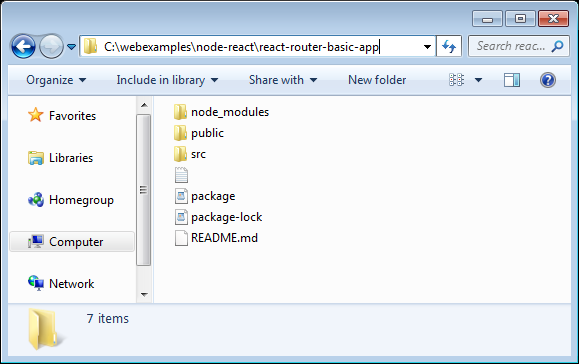
# CD to your project
cd react-router-basic-app
# Install react-router-dom library:
npm install --save react-router-dom
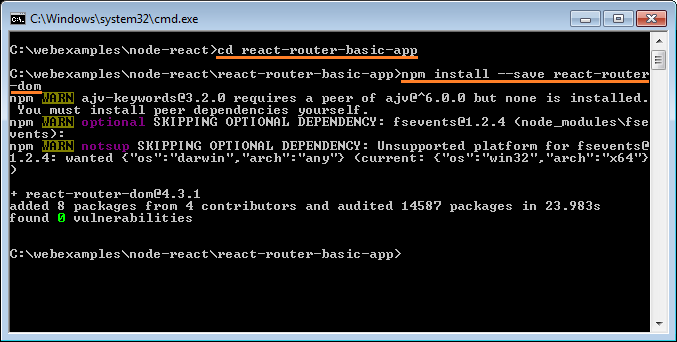
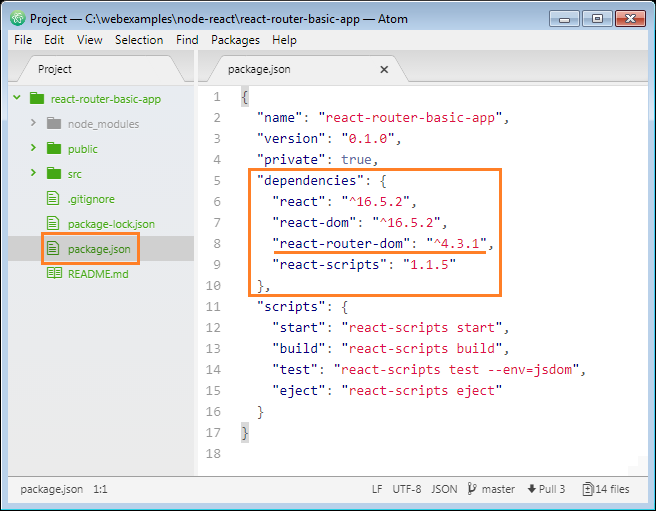
# Start App
npm start
3. Write code
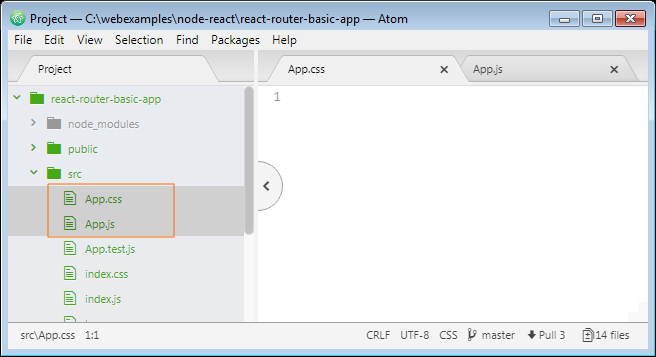
.main-route-place {
border: 1px solid #bb8fce;
margin:3px;
padding: 5px;
}
.secondary-route-place {
border: 1px solid #a2d9ce;
margin: 5px;
padding: 5px;
}
import React from "react";
import { BrowserRouter, Route, Link } from "react-router-dom";
import './App.css';
class App extends React.Component {
render() {
return (
<BrowserRouter>
<div>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/topics">Topics</Link>
</li>
</ul>
<hr />
<div className="main-route-place">
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/topics" component={Topics} />
</div>
</div>
</BrowserRouter>
);
}
}
class Home extends React.Component {
render() {
return (
<div>
<h2>Home</h2>
</div>
);
}
}
class About extends React.Component {
render() {
return (
<div>
<h2>About</h2>
</div>
);
}
}
class Topics extends React.Component {
render( ) {
return (
<div>
<h2>Topics</h2>
<ul>
<li>
<Link to={`${this.props.match.url}/rendering`}>
Rendering with React
</Link>
</li>
<li>
<Link to={`${this.props.match.url}/components`}>Components</Link>
</li>
<li>
<Link to={`${this.props.match.url}/props-v-state`}>
Props v. State
</Link>
</li>
</ul>
<div className="secondary-route-place">
<Route
path={`${this.props.match.url}/:topicId`}
component={Topic} />
<Route
exact
path={this.props.match.url}
render={() =>
<h3>
Please select a topic.
</h3>
}
/>
</div>
</div>
);
}
}
class Topic extends React.Component {
render() {
return (
<div>
<h3>
{this.props.match.params.topicId}
</h3>
</div>
);
}
}
export default App;
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
ReactDOM.render(<App />, document.getElementById('root'));
registerServiceWorker();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<title>React App</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
</body>
</html>
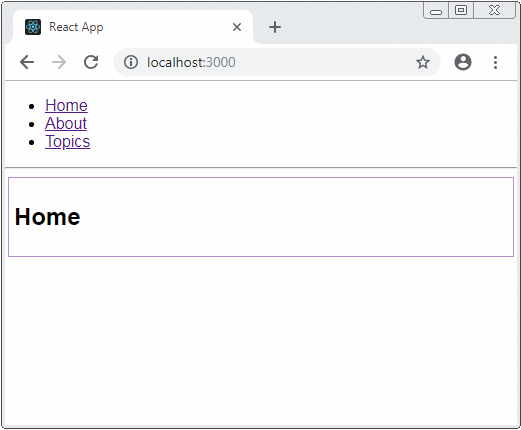
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS