Undertanding ReactJS Router with example on the client side
1. What is React Router?
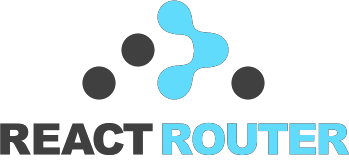
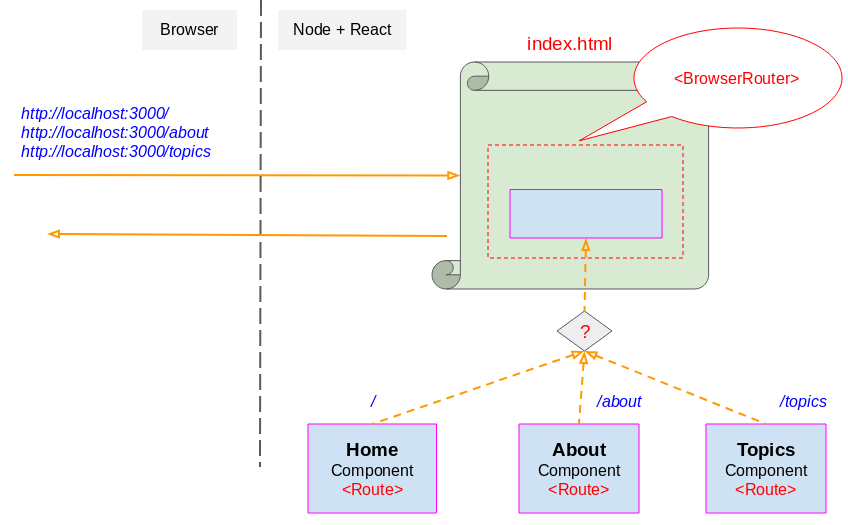
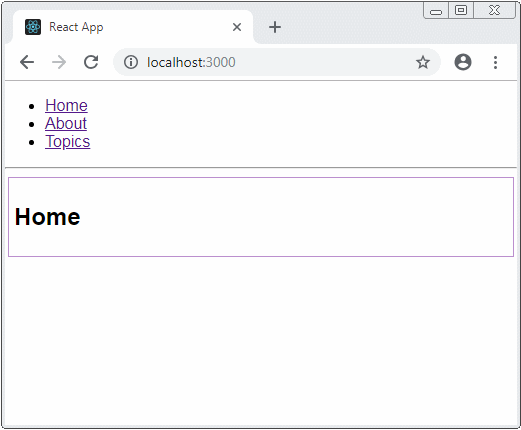
// <BrowserRouter>
http://example.com/about
// <HashRouter>
http://example.com/#/about
<BrowserRouter>
<Route exact path="/" component={Home}/>
<Route path="/about" component={About}/>
<Route path="/topics" component={Topics}/>
</BrowserRouter>
<HashRouter>
<Route exact path="/" component={Home}/>
<Route path="/about" component={About}/>
<Route path="/topics" component={Topics}/>
</HashRouter>
<BrowserRouter>
...
<Route path="/about" component={About}/>
...
</BrowserRouter>
http://example.com/about ==> OK Work!
http://example.com/about#somthing ==> OK Work!
http://example.com/about/something ==> OK Work!
http://example.com/about/a/b ==> OK Work!
-------------------
http://example.com/something/about ==> Not Work!
http://example.com/something/about#something ==> Not Work!
http://example.com/something/about/something ==> Not Work!
<HashRouter>
...
<Route path="/about" component={About}/>
...
</HashRouter>
http://example.com#/about ==> OK Work!
http://example.com#/about/somthing ==> OK Work!
----------------
http://example.com/something ==> Not Work!
http://example.com/something#/about ==> Not Work!
<BrowserRouter>
...
<Route exact path="/about" component={About}/>
...
</BrowserRouter>
http://example.com/about ==> OK Work!
http://example.com/about#somthing ==> OK Work!
-------------
http://example.com/about/something ==> Not Work!
http://example.com/about/a/b ==> Not Work!
http://example.com/something/about ==> Not Work!
http://example.com/something/about#something ==> Not Work!
http://example.com/something/about/something ==> Not Work!
<HashRouter>
...
<Route exact path="/about" component={About}/>
...
</HashRouter>
http://example.com#/about ==> OK Work!
----------------
http://example.com#/about/somthing ==> Not Work!
http://example.com/something ==> Not Work!
http://example.com/something#/about ==> Not Work!
If you are looking an example of React Router on the Server side (NodeJS), the following posts can be useful to you:
2. React Router on Client side
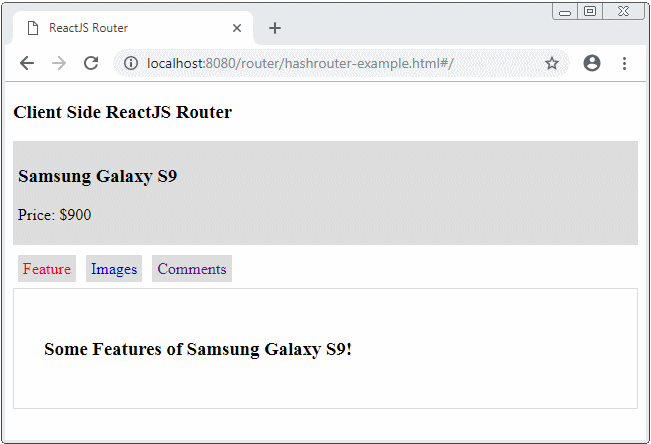
<!-- React & ReactDOM Libraries -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/16.4.2/umd/react.production.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.4.2/umd/react-dom.production.min.js"></script>
<!-- React Router -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-router-dom/4.3.1/react-router-dom.min.js"></script>
<!-- Babel -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/6.26.0/babel.min.js"></script>
<!-- CSS -->
<link rel = "stylesheet" type = "text/css" href = "router-example.css" />
If you want to find a new library version, please visit the following link:
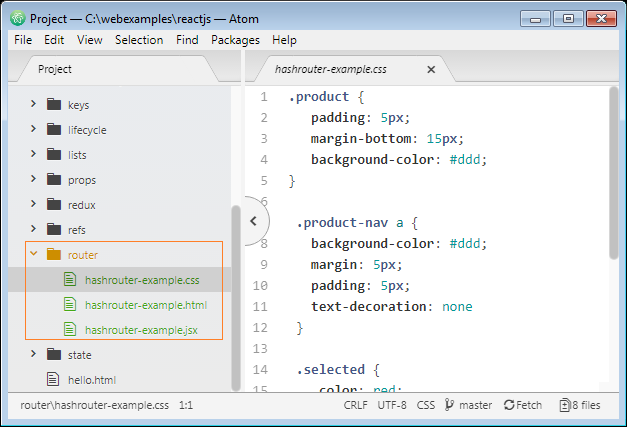
.product {
padding: 5px;
margin-bottom: 15px;
background-color: #ddd;
}
.product-nav a {
background-color: #ddd;
margin: 5px;
padding: 5px;
text-decoration: none
}
.selected {
color: red;
}
.route-place {
margin-top: 10px;
padding: 30px;
height: 100%;
border: 1px solid #ddd;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Router</title>
<!-- React & ReactDOM Libraries -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/16.4.2/umd/react.production.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.4.2/umd/react-dom.production.min.js"></script>
<!-- React Router -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-router-dom/4.3.1/react-router-dom.min.js"></script>
<!-- Babel -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/6.26.0/babel.min.js"></script>
<!-- CSS -->
<link rel = "stylesheet" type = "text/css" href = "hashrouter-example.css" />
</head>
<body>
<h3>Client Side ReactJS Router</h3>
<div id="app"></div>
<script src="hashrouter-example.jsx" type="text/babel"></script>
</body>
</html>
// import {BrowserRouter,NavLink} from 'react-router-dom';
// const { HashRouter, NavLink, Route } = ReactRouterDOM;
// Component
class ProductShortInfo extends React.Component {
render() {
return (
<div className="product">
<h3>Samsung Galaxy S9</h3>
<p>Price: $900</p>
</div>
);
}
}
// Component
class ProductFeature extends React.Component {
render() {
return <h3>Some Features of Samsung Galaxy S9!</h3>;
}
}
// Component
class ProductImages extends React.Component {
render() {
return <h3>Some Images of Samsung Galaxy S9</h3>;
}
}
// Component
class ProductComments extends React.Component {
render() {
return <h3>Some Customer Comments</h3>;
}
}
//
class Product extends React.Component {
render() {
return (
<ReactRouterDOM.HashRouter>
<div>
<ProductShortInfo />
<div className="product-nav">
<ReactRouterDOM.NavLink exact to="/" activeClassName="selected">
Feature
</ReactRouterDOM.NavLink>
<ReactRouterDOM.NavLink exact to="/images" activeClassName="selected">
Images
</ReactRouterDOM.NavLink>
<ReactRouterDOM.NavLink to="/comments" activeClassName="selected">
Comments
</ReactRouterDOM.NavLink>
</div>
<div className="route-place">
<ReactRouterDOM.Route exact path="/" component={ProductFeature} />
<ReactRouterDOM.Route exact path="/images" component={ProductImages} />
<ReactRouterDOM.Route path="/comments" component={ProductComments} />
</div>
</div>
</ReactRouterDOM.HashRouter>
);
}
}
//
class App extends React.Component {
render() {
return <Product />;
}
}
// Render
ReactDOM.render(<App />, document.querySelector("#app"));
Note: The components: <ReactRouterDOM.NavLink> & <ReactRouterDOM.Link> are very similar in usage. The <ReactRouterDOM.NavLink> is better because it supports the activeClassName attribute (useful in this example).
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS