ReactJS Refs Tutorial with Examples
1. React Refs
In ReactJS, refs are used as reference to an element for you. Basically, it is advisable to avoid using the refs in most cases. However, it is useful when you want to access the DOM nodes or elements created in the render() method.
Note: In this lesson, I will create Refs by the style of ReactJS, version 16.3, because it is easier for use.
React.createRef()
Use the React.createRef() method to create Refs, and then attach them to the elements (created in render() ) through the ref property.
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
render() {
return <div ref={this.myRef} />;
}
}
When a Ref is attached to an element create in the render() method, you may refer to the Node object of this element through the current property of the Ref.
const node = this.myRef.current;
Example:
refs-example.jsx
//
class SearchBox extends React.Component {
constructor(props) {
super(props);
this.state = {
searchText: "reactjs"
};
this.searchFieldRef = React.createRef();
}
clearAndFocus() {
this.setState({ searchText: "" });
// Focus to Input Field.
this.searchFieldRef.current.focus();
this.searchFieldRef.current.style.background = "#e8f8f5";
}
changeSearchText(event) {
var v = event.target.value;
this.setState((prevState, props) => {
return {
searchText: v
};
});
}
render() {
return (
<div className="search-box">
<input
value={this.state.searchText}
ref={this.searchFieldRef}
onChange={event => this.changeSearchText(event)}
/>
<button onClick={() => this.clearAndFocus()}>Clear And Focus</button>
<a href="">Reset</a>
</div>
);
}
}
// Render
ReactDOM.render(<SearchBox />, document.getElementById("searchbox1"));
refs-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Refs</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
<style>
.search-box {
border:1px solid #cbbfab;
padding: 5px;
margin: 5px;
}
</style>
</head>
<body>
<h3>React Refs:</h3>
<div id="searchbox1"></div>
<script src="refs-example.jsx" type="text/babel"></script>
</body>
</html>
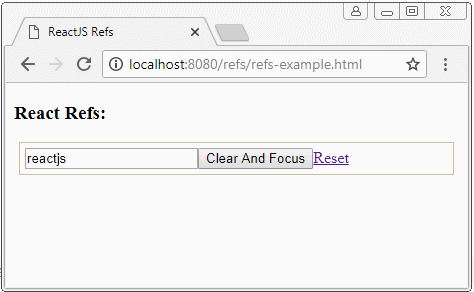
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
Show More