ReactJS Forms Tutorial with Examples
In HTML, the elements of Form such as <input>, <textarea>, <select> themselves manage their statuses. Their status may be changed by the impaction of user.
Basically, the data of Form will be directly sent to Server when the user clicks on Submit. But, if you want to control the behavior and data of the form by React. You need to create a two-way relationship between the values of the Form elements and the status of the React.
1. Form Input
Below is a simple example with the <input> element. The value of this element is assigned from this.state.fullName (A managed state in Component). When people change the value of <input>, this value needs to be updated for this.state.fullName via the setState() method.
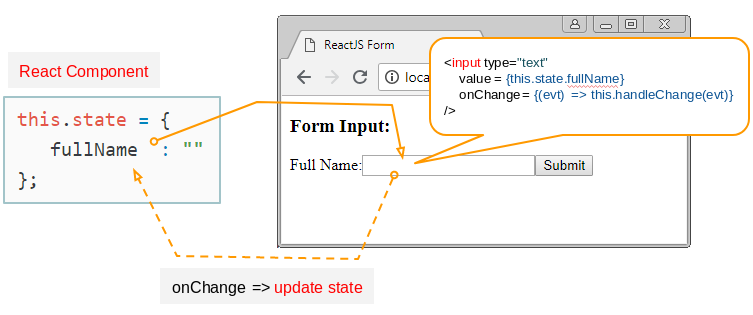
form-input-example.jsx
class SimpleForm extends React.Component {
constructor(props) {
super(props);
this.state = {
fullName: ""
};
}
handleSubmitForm(event) {
alert("Full Name: " + this.state.fullName);
event.preventDefault();
}
handleChange(event) {
var value = event.target.value;
this.setState({
fullName: value
});
}
render() {
return (
<form onSubmit={event => this.handleSubmitForm(event)}>
<label>
Full Name:
<input
type="text"
value={this.state.fullName}
onChange={event => this.handleChange(event)}
/>
</label>
<input type="submit" value="Submit" />
<p>{this.state.fullName}</p>
</form>
);
}
}
// Render
ReactDOM.render(<SimpleForm />, document.getElementById("form1"));
form-input-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Form</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
</head>
<body>
<h3>Form Input:</h3>
<div id="form1"></div>
<script src="form-input-example.jsx" type="text/babel"></script>
</body>
</html>
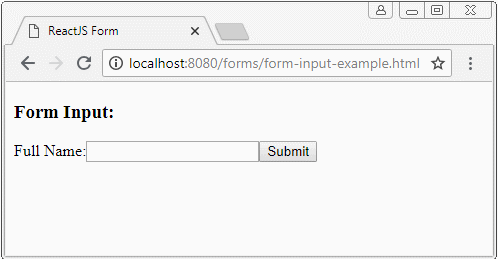
2. Form textarea
In HTML, different from the <input> element, the <textarea> element allows users to enter contents of longer and more complex text. The text content of <textarea> is its child-element (This child-element is a #text Node).
<textarea>
Hello there, this is some text in a text area
</textarea>
In React, the <textarea> text content will be set up through the value property instead of the #text Node. Thus, in the React , the use way of <textarea> hasn't have anthing different from the <input>.
form-textarea-example.jsx
class EssayForm extends React.Component {
constructor(props) {
super(props);
this.state = {
content: ""
};
}
handleSubmitForm(event) {
alert("Textarea Content: " + this.state.content);
event.preventDefault();
}
handleChange(event) {
var value = event.target.value;
this.setState({
content: value
});
}
render() {
return (
<form onSubmit={event => this.handleSubmitForm(event)}>
<label>Content</label>
<br />
<textarea cols="45" rows="5"
value={this.state.content}
onChange={event => this.handleChange(event)} />
<br />
<input type="submit" value="Submit" />
<p>{this.state.content}</p>
</form>
);
}
}
// Render
ReactDOM.render(<EssayForm />, document.getElementById("form1"));
form-textarea-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Form textarea</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
</head>
<body>
<h3>Form textarea:</h3>
<div id="form1"></div>
<script src="form-textarea-example.jsx" type="text/babel"></script>
</body>
</html>
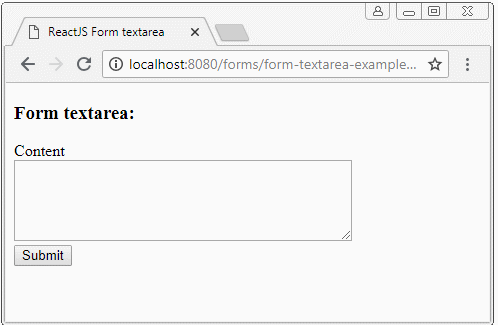
3. Form select/option
form-select-example.jsx
class FlavorForm extends React.Component {
constructor(props) {
super(props);
this.state = {
favoriteFlavor: "coconut"
};
}
handleSubmitForm(event) {
alert("Favorite Flavor: " + this.state.favoriteFlavor);
event.preventDefault();
}
handleChange(event) {
var value = event.target.value;
this.setState({
favoriteFlavor: value
});
}
render() {
return (
<form onSubmit={event => this.handleSubmitForm(event)}>
<p>Pick your favorite flavor:</p>
<select
value={this.state.favoriteFlavor}
onChange={event => this.handleChange(event)}>
<option value="grapefruit">Grapefruit</option>
<option value="lime">Lime</option>
<option value="coconut">Coconut</option>
<option value="mango">Mango</option>
</select>
<input type="submit" value="Submit" />
</form>
);
}
}
// Render
ReactDOM.render(<FlavorForm />, document.getElementById("form1"));
form-select-example.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>ReactJS Form select/option</title>
<script src="https://unpkg.com/react@16.4.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@16.4.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/babel-standalone@6.26.0/babel.min.js"></script>
</head>
<body>
<h3>Form select/option:</h3>
<div id="form1"></div>
<script src="form-select-example.jsx" type="text/babel"></script>
</body>
</html>
ReactJS Tutorials
- ReactJS props and state Tutorial with Examples
- ReactJS Events Tutorial with Examples
- ReactJS Component API Tutorial with Examples
- ReactJs component Lifecycle methods
- ReactJS Refs Tutorial with Examples
- ReactJS Lists and Keys Tutorial with Examples
- ReactJS Forms Tutorial with Examples
- Undertanding ReactJS Router with example on the client side
- Introduction to Redux
- Simple example with React and Redux on the client side
- React-Transition-Group API Tutorial with Examples
- Quickstart with ReactJS in NodeJS Environment
- Undertanding ReactJS Router with a basic example (NodeJS)
- React-Transition-Group Transition Example (NodeJS)
- React-Transition-Group CSSTransition Example (NodeJS)
- Introduction to ReactJS
- Install React Plugin for Atom Editor
- Create a Simple HTTP Server with NodeJS
- Quickstart with ReactJS - Hello ReactJS
Show More