C# Structures Tutorial with Examples
1. What is Struct?
In C#, Struct (structure) is a special type of value, it creates a variable, to store multiple values, but the values are related to each other.
For example, information about an employee include:
- Employee number
- Employee name
- Position
You can create 3 variables to store the information above of employee. However you can create a Struct to store all three information on in a single variable.
C # uses struct keyword to declare a Struct.
Employee.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
struct Employee
{
public string empNumber;
public string empName;
public string position;
public Employee(string empNumber, string empName, string position)
{
this.empNumber = empNumber;
this.empName = empName;
this.position = position;
}
}
}
For example, use Struct:
EmployeeTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
class EmployeeTest
{
public static void Main(string[] args)
{
Employee john = new Employee("E01","John", "CLERK");
Console.WriteLine("Emp Number: " + john.empNumber);
Console.WriteLine("Emp Name: " + john.empName);
Console.WriteLine("Emp Position: " + john.position);
Console.Read();
}
}
}
Running the example:
Emp Number: E01
Emp Name: John
Emp Position: CLECK
2. Struct vs Class
Struct is commonly used to create an object to store the value, while the class is using more diversely.
- Struct does not allow inherit, it can not expand from a certain class or a struct.
- Struct does not allow implement Interface.
One of the struct frequently used in C# that is DateTime, a struct describing the date and time. You can see more about using date and time in C# at:
If struct appears as a parameter in a method, it is passed as a value. Meanwhile, if the class appears as a parameter in a method it is passed as a reference.
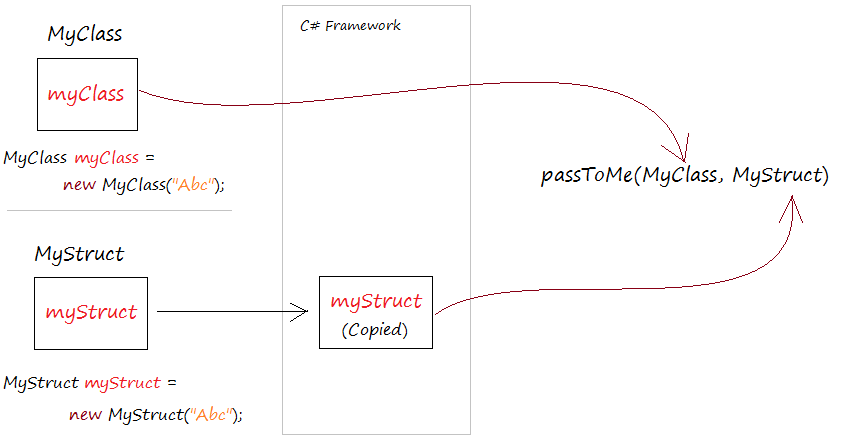
MyClass.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
class MyClass
{
public string name = "Abc";
public MyClass(string name)
{
this.name = name;
}
}
}
MyStruct.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
struct MyStruct
{
public string name;
public MyStruct(string name)
{
this.name = name;
}
}
}
MyTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
class MyTest
{
private static void passToMe(MyClass myClass, MyStruct myStruct)
{
// Change value for name field.
myClass.name = "New Name";
// Change value for name field.
myStruct.name = "New Name";
}
public static void Main(string[] args)
{
MyClass myClass = new MyClass("Abc");
MyStruct myStruct = new MyStruct("Abc");
Console.WriteLine("Before pass to method");
Console.WriteLine("myClass.name = " + myClass.name);// Abc
Console.WriteLine("myStruct.name = " + myStruct.name);// Abc
Console.WriteLine("Pass to method");
// 'myStruct' passed to the method is a copy.
// (Not the original object).
passToMe(myClass, myStruct);
Console.WriteLine("myClass.name = " + myClass.name); // New Name
Console.WriteLine("myStruct.name = " + myStruct.name);// Abc
Console.Read();
}
}
}
Running the example:
Before pass to method
myClass.name = Abc
myStruct.name = Abc
Pass to method
myClass.name = New Name
myStruct.name = Abc
3. Constructor of Struct
Struct can have Constructors but do not have Destructor
Here are few notes for the Constructor:
- You can not write a default constructor (no parameters) for struct.
- In the constructor you must assign values to all fields that don't have value.
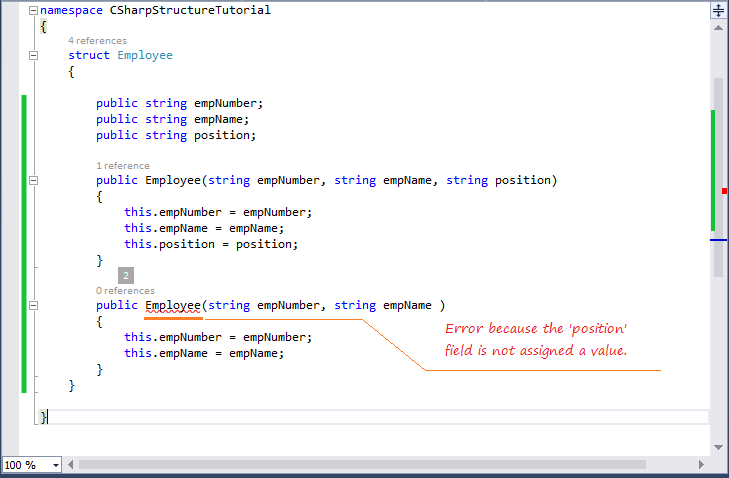
4. Methods and properties of struct
Struct can have methods and properties, and because Struct do not inherit, therefore, all its modes and properties are not allowed to be abstract.
Book.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharpStructureTutorial
{
struct Book
{
private string title;
private string author;
// Property
public string Title
{
get
{
return this.title;
}
set
{
this.title = value;
}
}
// Property
public string Author
{
get
{
return this.author;
}
}
// Constructor.
public Book(string title, string author)
{
this.title = title;
this.author = author;
}
// Method.
public string GetInfo()
{
return "Book Title: " + this.title + ", Author: " + this.author;
}
}
}
C# Programming Tutorials
- Inheritance and polymorphism in C#
- What is needed to get started with C#?
- Quick learning C# for Beginners
- Install Visual Studio 2013 on Windows
- Abstract class and Interface in C#
- Install Visual Studio 2015 on Windows
- Compression and decompression in C#
- C# Multithreading Programming Tutorial with Examples
- C# Delegates and Events Tutorial with Examples
- Install AnkhSVN on Windows
- C# Programming for Team using Visual Studio and SVN
- Install .Net Framework
- Access Modifier in C#
- C# String and StringBuilder Tutorial with Examples
- C# Properties Tutorial with Examples
- C# Enums Tutorial with Examples
- C# Structures Tutorial with Examples
- C# Generics Tutorial with Examples
- C# Exception Handling Tutorial with Examples
- C# Date Time Tutorial with Examples
- Manipulating files and directories in C#
- C# Streams tutorial - binary streams in C#
- C# Regular Expressions Tutorial with Examples
- Connect to SQL Server Database in C#
- Work with SQL Server database in C#
- Connect to MySQL database in C#
- Work with MySQL database in C#
- Connect to Oracle Database in C# without Oracle Client
- Work with Oracle database in C#
Show More