C# String and StringBuilder Tutorial with Examples
1. Hierarchical Inheritance
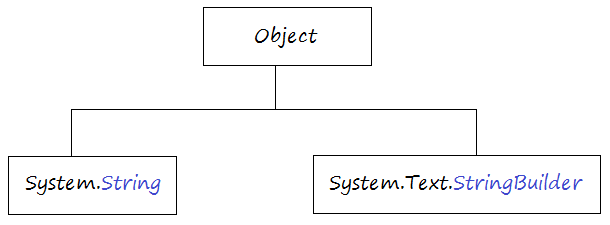
When working with text data, CSharp provides you with three classes including StringandStringBuilder. When working with big data, you should use StringBuilder to optimize the efficiency. Basically, 2 classes have many similarities.
- String is immutable (More details of this concept are in the document). It does not allow the existence of subclass.
- StringBuilder is mutable.
2. Mutable and immutable concept
Consider an illustration:
MutableClassExample.cs
namespace StringTutorial
{
// This is a class has one field: 'Value'.
// If you have an object of this class.
// You can assign new value to 'Value' field
// via SetNewValue(int) method.
// So this is a mutable class.
class MutableClassExample
{
private int Value;
public MutableClassExample(int value)
{
this.Value = value;
}
public void SetNewValue(int newValue)
{
this.Value = newValue;
}
}
}
ImmutableClassExample.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace StringTutorial
{
// This is a class has 2 fields: 'Value' & 'Name'.
// If you have an object of this class,
// You cannot asign new value to 'Value','Name' field from outside,
// It means this class is immutable.
class ImmutableClassExample
{
private int Value;
private String Name;
public ImmutableClassExample(String name, int value)
{
this.Value = value;
this.Name = name;
}
public String GetName()
{
return Name;
}
public int GetValue()
{
return Value;
}
}
}
String is a immutable class. String includes various fields like length, but values in these fields can not change
3. String and string
In C #, sometimes you see String and string are used in parallel. In fact, they do not have any difference, string can be seen as an alias for System.String (full name including the namespace of the String class).
The table below describes the full list of aliases of the common class.
The table below describes the full list of aliases of the common class.
Alias | Class |
object | System.Object |
string | System.String |
bool | System.Boolean |
byte | System.Byte |
sbyte | System.SByte |
short | System.Int16 |
ushort | System.UInt16 |
int | System.Int32 |
uint | System.UInt32 |
long | System.Int64 |
ulong | System.UInt64 |
float | System.Single |
double | System.Double |
decimal | System.Decimal |
char | System.Char |
4. String
String is one of the most important class in CSharp and anyone who starts with CSharp programming uses String to print something on console by using famous Console.WriteLine() statements. Many C# beginners not aware that String is immutable and sealed (Do not allow subclasses). In C# and every modification in String result creates a new String object.
** String **
[SerializableAttribute]
[ComVisibleAttribute(true)]
public sealed class String : IComparable, ICloneable, IConvertible,
IEnumerable, IComparable<string>, IEnumerable<char>, IEquatable<string>
The methods of the String
You can look at the methods of String:
Below is a list of some common methods of String.
Some String methods
public bool EndsWith(string value)
public bool EndsWith(string value, StringComparison comparisonType)
public bool Equals(string value)
public int IndexOf(char value)
public int IndexOf(char value, int startIndex)
public int IndexOf(string value, int startIndex, int count)
public int IndexOf(string value, int startIndex, StringComparison comparisonType)
public int IndexOf(string value, StringComparison comparisonType)
public string Insert(int startIndex, string value)
public int LastIndexOf(char value)
public int LastIndexOf(char value, int startIndex)
public int LastIndexOf(char value, int startIndex, int count)
public int LastIndexOf(string value)
public int LastIndexOf(string value, int startIndex)
public int LastIndexOf(string value, int startIndex, int count)
public int LastIndexOf(string value, int startIndex, int count, StringComparison comparisonType)
public int LastIndexOf(string value, int startIndex, StringComparison comparisonType)
public int LastIndexOf(string value, StringComparison comparisonType)
public int LastIndexOfAny(char[] anyOf)
public int LastIndexOfAny(char[] anyOf, int startIndex)
public int LastIndexOfAny(char[] anyOf, int startIndex, int count)
public int IndexOf(string value, int startIndex, int count, StringComparison comparisonType)
public string Replace(char oldChar, char newChar)
public string Replace(string oldValue, string newValue)
public string[] Split(params char[] separator)
public string[] Split(char[] separator, int count)
public string[] Split(char[] separator, int count, StringSplitOptions options)
public string[] Split(char[] separator, StringSplitOptions options)
public string[] Split(string[] separator, StringSplitOptions options)
public bool StartsWith(string value)
public bool StartsWith(string value, bool ignoreCase, CultureInfo culture)
public bool StartsWith(string value, StringComparison comparisonType)
public string Substring(int startIndex)
public string Substring(int startIndex, int length)
public char[] ToCharArray()
public char[] ToCharArray(int startIndex, int length)
public string ToLower()
public string ToLower(CultureInfo culture)
public string ToLowerInvariant()
public override string ToString()
public string ToUpper()
public string ToUpper(CultureInfo culture)
public string ToUpperInvariant()
public string Trim()
public string Trim(params char[] trimChars)
public string TrimEnd(params char[] trimChars)
public string TrimStart(params char[] trimChars)
5. StringBuilder
In C# and every modification in String result creates a new String object. Meanwhile StringBuilder contains in it an array of characters, this array will automatically replaced by a larger array if necessary, and copy the characters from old array. If you must concatenate multiple strings, you should use the StringBuilder, it helps to increase the efficiency of the program. But it is not necessary if you only concatenate a few strings, you should not abuse StringBuilder in that case.
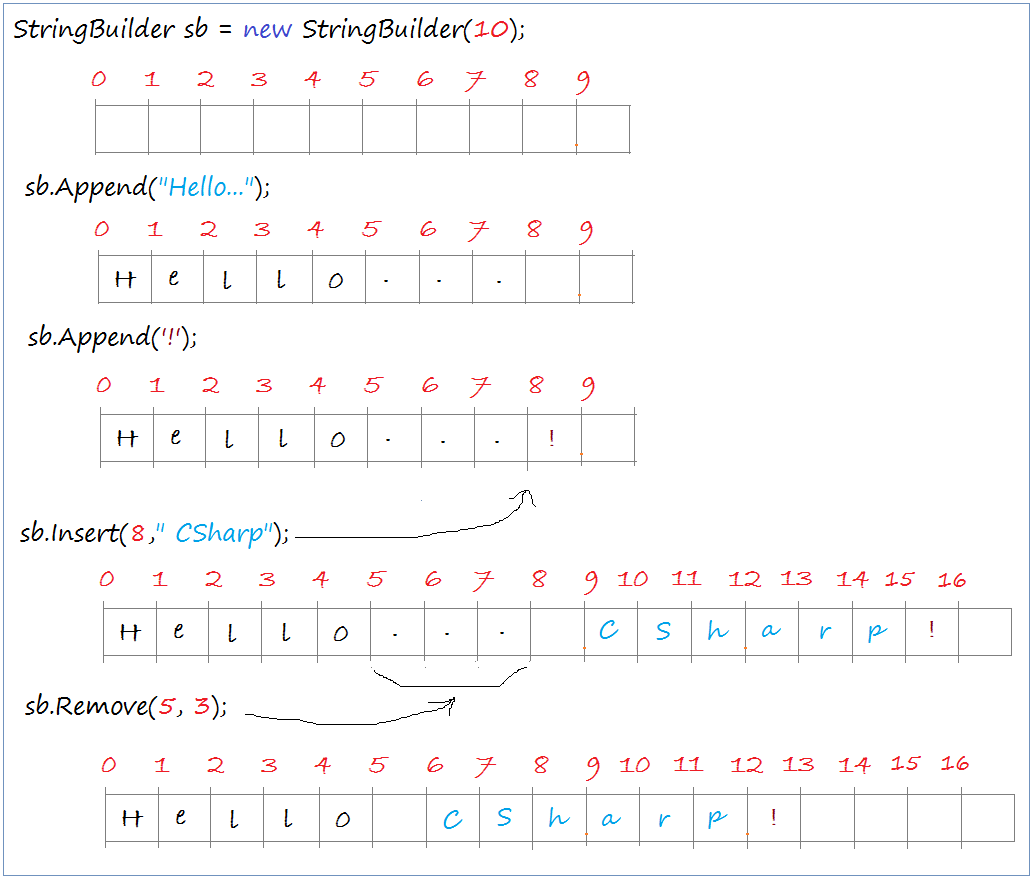
StringBuilderDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace StringTutorial
{
class StringBuilderDemo
{
public static void Main(string[] args)
{
// Create a StringBuilder object with a capacity of 10 characters.
StringBuilder sb = new StringBuilder(10);
// Append the string Hello ...
sb.Append("Hello...");
Console.WriteLine("- sb after appends a string: " + sb);
// Append a character
char c = '!';
sb.Append(c);
Console.WriteLine("- sb after appending a char: " + sb);
// Insert a string at index 5
sb.Insert(8, " CSharp");
Console.WriteLine("- sb after insert string: " + sb);
// Remove substring at index 5 and 3 characters.
sb.Remove(5, 3);
Console.WriteLine("- sb after remove: " + sb);
// Get string in StringBuilder.
String s = sb.ToString();
Console.WriteLine("- String of sb: " + s);
Console.Read();
}
}
}
Results of running the example:
- sb after appends a string: Hello...
- sb after appending a char: Hello...!
- sb after insert string: Hello... CSharp!
- sb after remove: Hello CSharp!
- String of sb: Hello CSharp!
C# Programming Tutorials
- Inheritance and polymorphism in C#
- What is needed to get started with C#?
- Quick learning C# for Beginners
- Install Visual Studio 2013 on Windows
- Abstract class and Interface in C#
- Install Visual Studio 2015 on Windows
- Compression and decompression in C#
- C# Multithreading Programming Tutorial with Examples
- C# Delegates and Events Tutorial with Examples
- Install AnkhSVN on Windows
- C# Programming for Team using Visual Studio and SVN
- Install .Net Framework
- Access Modifier in C#
- C# String and StringBuilder Tutorial with Examples
- C# Properties Tutorial with Examples
- C# Enums Tutorial with Examples
- C# Structures Tutorial with Examples
- C# Generics Tutorial with Examples
- C# Exception Handling Tutorial with Examples
- C# Date Time Tutorial with Examples
- Manipulating files and directories in C#
- C# Streams tutorial - binary streams in C#
- C# Regular Expressions Tutorial with Examples
- Connect to SQL Server Database in C#
- Work with SQL Server database in C#
- Connect to MySQL database in C#
- Work with MySQL database in C#
- Connect to Oracle Database in C# without Oracle Client
- Work with Oracle database in C#
Show More