Manipulating files and directories in C#
1. Class hierarchy
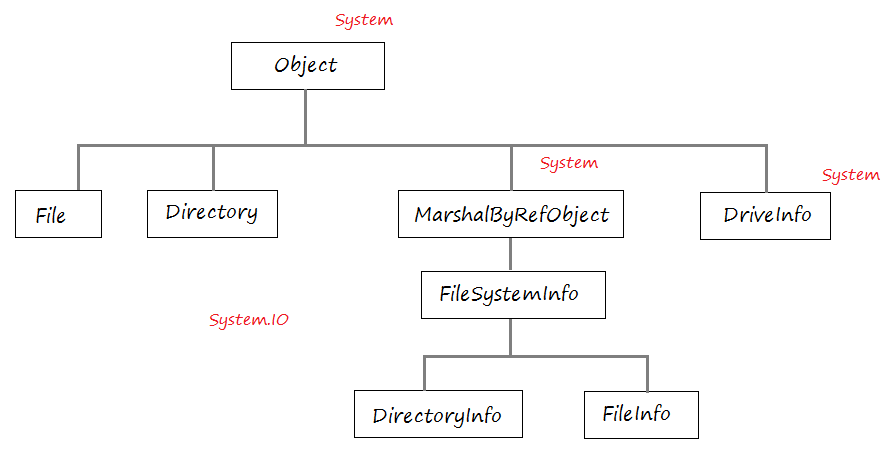
Class | Description |
File | File is a utility class. It provides static methods for the creation, copying, deletion, moving, and opening of a single file, and aids in the creation of FileStreamobjects. |
Directory | Directory is a utility class, It provides static methods for creating, moving, and enumerating through directories and subdirectories. This class cannot be inherited. |
FileInfo | FileInfo is a class that represents a file. It provides properties and instance methods for the creation, copying, deletion, moving, and opening of files, and aids in the creation of FileStream objects. This class cannot be inherited. |
DirectoryInfo | DirectoryInfo is a class representing a directory. It provides methods for creating, moving, and enumerating through directories and subdirectories. This class cannot be inherited. |
DriveInfo | DirveInfo is a class, it provides access to information on a drive. |
2. File
File is a utility class. It provides methods for the creation, copying, deletion, moving, and opening of a single file, and aids in the creation of FileStreamobjects.
The example below check if a file path exists or not, delete this file if it exists.
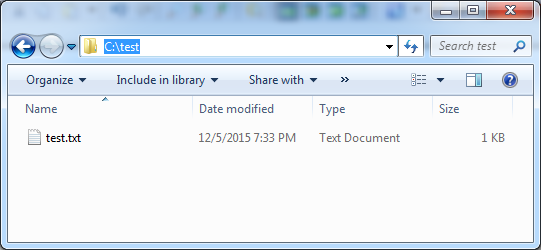
DeleteFileDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DeleteFileDemo
{
public static void Main(string[] args)
{
string filePath = "C:/test/test.txt";
// Check if the path exists or not
if (File.Exists(filePath))
{
// Delete file
File.Delete(filePath);
// Check again
if (!File.Exists(filePath))
{
Console.WriteLine("File deleted...");
}
}
else
{
Console.WriteLine("File test.txt does not yet exist!");
}
Console.ReadKey();
}
}
}
Running the example:
File deleted...
Rename the file may include moving the file to another folder and renaming the file. In case of moving files to another folder to make sure that this folder exists.
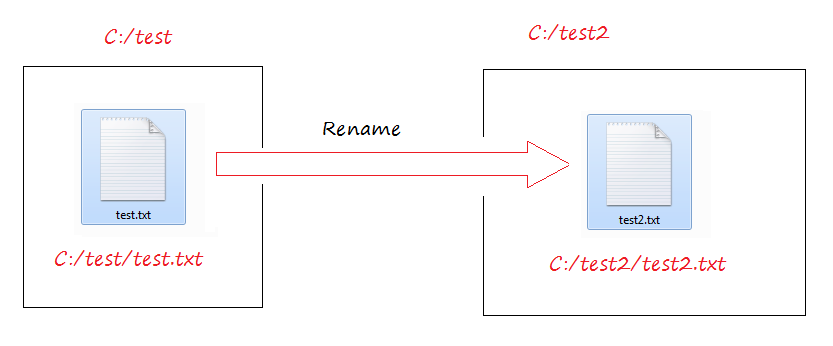
RenameFileDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameFileDemo
{
public static void Main(string[] args)
{
String filePath = "C:/test/test.txt";
if (File.Exists(filePath))
{
Console.WriteLine(filePath + " exist");
Console.WriteLine("Please enter a new name for this file:");
// A String that user enters.
// Example: C:/test/test2.txt
string newFilename = Console.ReadLine();
if (newFilename != String.Empty)
{
// Rename the file:
// May include moving the file to another folder and renaming the file.
// You must ensure that the destination folder exists.
// (Else, DirectoryNotFoundException will be thrown).
File.Move(filePath, newFilename);
if (File.Exists(newFilename))
{
Console.WriteLine("The file was renamed to " + newFilename);
}
}
}
else
{
Console.WriteLine("Path " + filePath + " does not exist.");
}
Console.ReadLine();
}
}
}
Running the example:
C:/test/test.txt exist
Please enterr a new name for this file:
C:/test/test2.txt
The file was renamed to C:/test/test2.txt
3. Directory
Directory is a utility class, It provides static methods for creating, moving, and enumerating through directories and subdirectories. This class cannot be inherited.
For example, check if a directory path exists or not, if it does not exist, create that directory, print out creating time, last write time, ....
DirectoryInformationDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DirectoryInformationDemo
{
public static void Main(string[] args)
{
String dirPath = "C:/test/CSharp";
// Check if a directory path exists or not.
bool exist = Directory.Exists(dirPath);
// If not exist, create it
if (!exist)
{
Console.WriteLine(dirPath + " does not exist.");
Console.WriteLine("Create directory: " + dirPath);
// Create Directory
Directory.CreateDirectory(dirPath);
}
Console.WriteLine("Directory Information " + dirPath);
// Print out infos
// Creation time
Console.WriteLine("Creation time: "+ Directory.GetCreationTime(dirPath));
// Last Write time.
Console.WriteLine("Last Write Time: " + Directory.GetLastWriteTime(dirPath));
// Parent directory
DirectoryInfo parentInfo = Directory.GetParent(dirPath);
Console.WriteLine("Parent directory: " + parentInfo.FullName);
Console.Read();
}
}
}
Running the example:
Directory Information C:/test/CSharp
Creation time: 12/5/2015 9:46:10 PM
Last Write Time: 12/5/2015 9:46:10 PM
Parent directory: C:\test
Rename the folder:
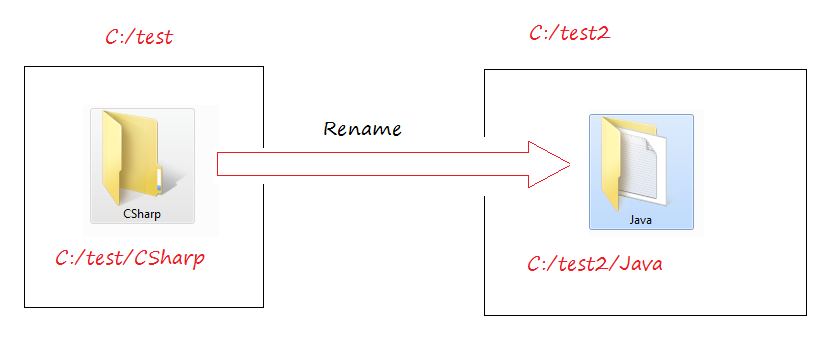
You can change folder name. It can make that folder to move from existing parent directory. But you must ensure that the new parent directory have been already existed. The example below illustrates how to rename folder:
RenameDirectoryDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameDirectoryDemo
{
public static void Main(string[] args)
{
// A directory path.
String dirPath = "C:/test/CSharp";
// If this path exists.
if (!Directory.Exists(dirPath))
{
Console.WriteLine(dirPath + " does not exist.");
Console.Read();
return;
}
Console.WriteLine(dirPath + " exist");
Console.WriteLine("Please enter a new name for this directory:");
// String that the user enters.
// example: C:/test2/Java
string newDirname = Console.ReadLine();
if (newDirname == String.Empty)
{
Console.WriteLine("You not enter new directory name. Cancel rename.");
Console.Read();
return;
}
// If the path entered by the user exists.
if (Directory.Exists(newDirname))
{
Console.WriteLine("Cannot rename directory. New directory already exist.");
Console.Read();
return;
}
// Parent directory.
DirectoryInfo parentInfo = Directory.GetParent(newDirname);
// Create parent folder of the new folder that the user enters.
Directory.CreateDirectory(parentInfo.FullName);
// You can change the path of a directory.
// but make sure parent path of new path is exists.
// (Else, DirectoryNotFoundException will be thrown)
Directory.Move(dirPath, newDirname);
if (Directory.Exists(newDirname))
{
Console.WriteLine("The directory was renamed to " + newDirname);
}
Console.ReadLine();
}
}
}
Running the example:
C:/test/CSharp exist
Please enter a new name for this directory:
C:/test2/Java
The directory was renamed to C:/test2/Java
The example below recursion to print out all the subdirectories, grandchildren, ... of a folder
EnumeratingDirectoryDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class EnumeratingDirectoryDemo
{
public static void Main(string[] args)
{
string dirPath = "C:/Windows/System32";
PrintDirectory(dirPath);
Console.Read();
}
// Recursive method to list the subfolders of a folder.
public static void PrintDirectory(string dirPath)
{
try
{
// You do not have sufficient access to the folder 'dirPath',
// UnauthorizedAccessException exception will be thrown.
IEnumerable<string> enums = Directory.EnumerateDirectories(dirPath);
List<string> dirs = new List<string>(enums);
foreach (var dir in dirs)
{
Console.WriteLine(dir);
// Recursive to search subdirectories.
// Đệ quy (recursive) để tìm kiếm các thư mục con.
PrintDirectory(dir);
}
}
// Security error when accessing the directory where you have no permission.
catch (UnauthorizedAccessException e)
{
Console.WriteLine("Can not access directory: " + dirPath);
Console.WriteLine(e.Message);
}
}
}
}
Running the example:
...
C:/Windows/System32\wbem\en-US
C:/Windows/System32\wbem\Logs
C:/Windows/System32\wbem\Repository
C:/Windows/System32\wbem\tmf
C:/Windows/System32\wbem\eml
C:/Windows/System32\WCN
C:/Windows/System32\WCN\en-US
...
4. FileInfo
FileInfo is a class that represents a file. It provides properties and instance methods for the creation, copying, deletion, moving, and opening of files, and aids in the creation of FileStream objects. This class cannot be inherited.
The difference between File and FileInfo is that File is an utility class and its methods are static, FileInfo represents a specific file.
FileInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class FileInfoDemo
{
static void Main(string[] args)
{
// An object that represents a file.
FileInfo testFile = new FileInfo("C:/test/test.txt");
if (testFile.Exists)
{
Console.WriteLine(testFile.FullName + " exist.");
// Creation time.
Console.WriteLine("Creation time: " + testFile.CreationTime);
// Last Write Time
Console.WriteLine("Last Write Time " + testFile.LastWriteTime);
// Name of parent Directory.
Console.WriteLine("Directory Name: " + testFile.DirectoryName);
}
else
{
Console.WriteLine(testFile.FullName + " does not exist.");
}
Console.Read();
}
}
}
Running the example:
C:\test\test.txt exist.
Creation time: 12/5/2015 7:47:40 PM
Last Write Time 12/5/2015 10:17:29 PM
Directory Name: C:\test
Rename the file may include moving the file to another folder and renaming the file. In case of moving files to another folder to make sure that this folder exists.
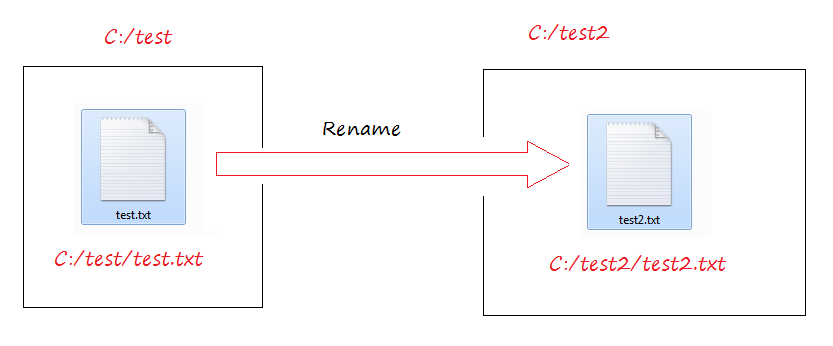
RenameFileInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class RenameFileInfoDemo
{
public static void Main(string[] args)
{
FileInfo fileInfo = new FileInfo("C:/test/test.txt");
if (!fileInfo.Exists)
{
Console.WriteLine(fileInfo.FullName + " does not exist.");
Console.Read();
return;
}
Console.WriteLine(fileInfo.FullName + " exist");
Console.WriteLine("Please enter a new name for this file:");
// A String that user enters
// example: C:/test/test2.txt
string newFilename = Console.ReadLine();
if (newFilename == String.Empty)
{
Console.WriteLine("You not enter new file name. Cancel rename");
Console.Read();
return;
}
FileInfo newFileInfo = new FileInfo(newFilename);
// If 'newFileInfo' exist (Can not rename).
if (newFileInfo.Exists)
{
Console.WriteLine("Can not rename file to " + newFileInfo.FullName + ". File already exist.");
Console.Read();
return;
}
// Create parent directory of 'newFileInfo'.
newFileInfo.Directory.Create();
// Rename
fileInfo.MoveTo(newFileInfo.FullName);
// Refresh.
newFileInfo.Refresh();
if (newFileInfo.Exists)
{
Console.WriteLine("The file was renamed to " + newFileInfo.FullName);
}
Console.ReadLine();
}
}
}
Running the example:
C:\test\test.txt exist
Please enter a new name for this file:
C:/test2/test2.txt
The file was renamed to C:\test2\test2.txt
5. DirectoryInfo
DirectoryInfo is a class representing a directory. It provides methods for creating, moving, and enumerating through directories and subdirectories. This class cannot be inherited.
The difference between Directory và DirectoryInfo class is that a utility Directory is utility class and its methods are static, while DirectoryInfo represents a particular directory.
DirectoryInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DirectoryInfoDemo
{
static void Main(string[] args)
{
// An object representing a folder.
DirectoryInfo dirInfo = new DirectoryInfo("C:/Windows/System32/drivers");
// Write out creation time.
Console.WriteLine("Creation time: " + dirInfo.CreationTime);
// Last write time
Console.WriteLine("Last Write Time " + dirInfo.LastWriteTime);
// Full name
Console.WriteLine("Directory Name: " + dirInfo.FullName);
// Array of subfolders.
DirectoryInfo[] childDirs = dirInfo.GetDirectories();
// An array of files in the folder.
FileInfo[] childFiles = dirInfo.GetFiles();
foreach(DirectoryInfo childDir in childDirs ){
Console.WriteLine(" - Directory: " + childDir.FullName);
}
foreach (FileInfo childFile in childFiles)
{
Console.WriteLine(" - File: " + childFile.FullName);
}
Console.Read();
}
}
}
Running the example:
Creation time: 7/14/2009 10:20:14 AM
Last Write Time 11/21/2010 2:06:51 PM
Directory Name: C:\Windows\System32\drivers
- Directory: C:\Windowws\System32\drivers\en-US
- File: C:\Windowws\System32\gm.dls
- File: C:\Windowws\System32\gmreadme.txt
- File: C:\Windowws\System32\winmount.sys
6. DriveInfo
DirveInfo is a class, it provides access to information on a drive.
DriveInfoDemo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace FileDirectoryTutorial
{
class DriveInfoDemo
{
static void Main(string[] args)
{
DriveInfo[] drives = DriveInfo.GetDrives();
foreach (DriveInfo drive in drives)
{
Console.WriteLine(" ============================== ");
// Name of Drive (C, D, ..)
Console.WriteLine("Drive {0}", drive.Name);
// Drive type (Removable,..)
Console.WriteLine(" Drive type: {0}", drive.DriveType);
// If drive is ready.
if (drive.IsReady)
{
Console.WriteLine(" Volume label: {0}", drive.VolumeLabel);
Console.WriteLine(" File system: {0}", drive.DriveFormat);
Console.WriteLine(
" Available space to current user:{0, 15} bytes",
drive.AvailableFreeSpace);
Console.WriteLine(
" Total available space: {0, 15} bytes",
drive.TotalFreeSpace);
Console.WriteLine(
" Total size of drive: {0, 15} bytes ",
drive.TotalSize);
}
}
Console.Read();
}
}
}
Running the example:
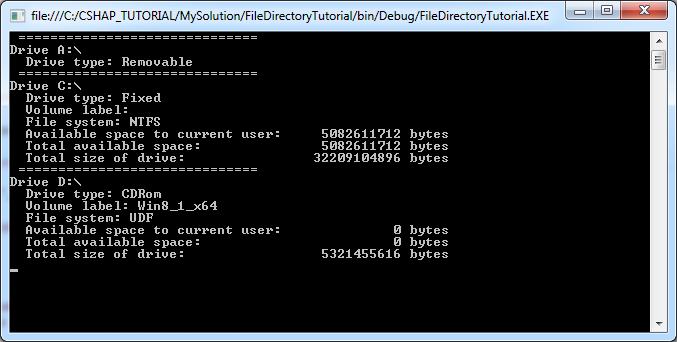
C# Programming Tutorials
- Inheritance and polymorphism in C#
- What is needed to get started with C#?
- Quick learning C# for Beginners
- Install Visual Studio 2013 on Windows
- Abstract class and Interface in C#
- Install Visual Studio 2015 on Windows
- Compression and decompression in C#
- C# Multithreading Programming Tutorial with Examples
- C# Delegates and Events Tutorial with Examples
- Install AnkhSVN on Windows
- C# Programming for Team using Visual Studio and SVN
- Install .Net Framework
- Access Modifier in C#
- C# String and StringBuilder Tutorial with Examples
- C# Properties Tutorial with Examples
- C# Enums Tutorial with Examples
- C# Structures Tutorial with Examples
- C# Generics Tutorial with Examples
- C# Exception Handling Tutorial with Examples
- C# Date Time Tutorial with Examples
- Manipulating files and directories in C#
- C# Streams tutorial - binary streams in C#
- C# Regular Expressions Tutorial with Examples
- Connect to SQL Server Database in C#
- Work with SQL Server database in C#
- Connect to MySQL database in C#
- Work with MySQL database in C#
- Connect to Oracle Database in C# without Oracle Client
- Work with Oracle database in C#
Show More